Why Scala Outperforms Java in Scalability Challenges
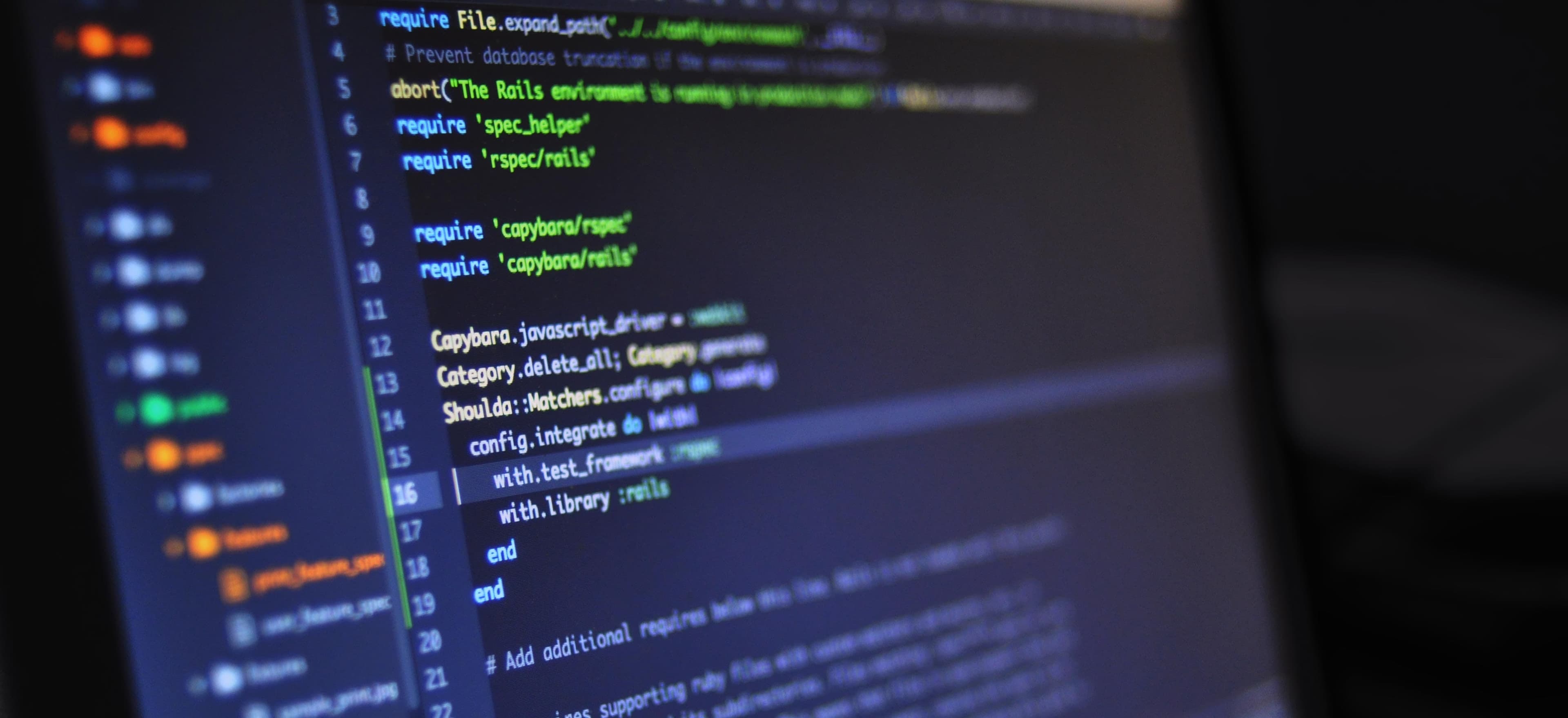
- Published on
Why Scala Outperforms Java in Scalability Challenges
In the ever-evolving landscape of software development, scalability remains one of the fundamental cornerstones of efficient programming. Both Java and Scala have garnered acclaim as robust and versatile programming languages, primarily in the realm of enterprise solutions. However, when it comes to scalability, Scala consistently demonstrates certain advantages over Java. In this blog post, we’ll delve into the nuances of scalability, explore the core differences between these two languages, and discuss why Scala can often outperform Java in addressing scalability challenges.
A Brief Overview to Scalability
Scalability refers to the capability of a system to handle a growing amount of workload or its potential to accommodate growth. It is crucial for applications that expect to grow in terms of user traffic, data processing, or computational demands over time.
Why Scalability Matters
Scalability is essential for several reasons:
- Cost Efficiency: A scalable system can handle increased loads without a complete overhaul, saving costs over time.
- User Satisfaction: A smooth user experience is maintained even during peak loads.
- Competitive Edge: Businesses can respond to growth or demand spikes swiftly.
Java’s Foundation in Scalability
Java, with its robust object-oriented principles and long-standing enterprise usage, provides a solid foundation for scalable applications. Its Write Once, Run Anywhere philosophy enables developers to build applications that can run on various platforms without significant rework. However, the traditional Java platform comes with certain limitations:
- Verbosity: Java's verbose syntax can lead to longer development times, making it less agile in responding to changing requirements.
- Concurrency: The multi-threading model in Java can sometimes be cumbersome, making it harder to implement complex concurrent tasks efficiently.
The Rise of Scala
Scala, a programming language that combines functional programming and object-oriented paradigms, was designed to address many of these constraints. It offers concise syntax, which enhances developer productivity, and inherent support for concurrency.
Key Features Making Scala Superior for Scalability
1. Concise Syntax and Expressiveness
Scala’s concise syntax allows developers to write less code while maintaining clarity. This expressiveness not only reduces the amount of boilerplate code but also accelerates the development process.
For example, consider a simple case of filtering even numbers from a list:
val numbers = List(1, 2, 3, 4, 5, 6)
val evenNumbers = numbers.filter(_ % 2 == 0)
println(evenNumbers) // Output: List(2, 4, 6)
In this example, the use of a lambda function simplifies the filtering process. In Java, the equivalent code would require more lines and be less intuitive:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Example {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6]
}
}
Here, both languages accomplish the same task, but Scala offers a more concise approach, leading to less room for errors and easier readability.
2. First-Class Functions
Scala treats functions as first-class citizens, allowing developers to pass functions as parameters, return them from other functions, and store them in variables. This paradigm simplifies code organization and enhances reusability.
def applyFunction(f: Int => Int, x: Int): Int = f(x)
val square: Int => Int = x => x * x
println(applyFunction(square, 5)) // Output: 25
This capability allows for powerful abstractions which can lead to more manageable and testable codebases.
3. Immutability by Default
In Scala, variables are immutable by default. This immutability ensures that data does not change, which greatly simplifies the challenges associated with concurrency. Faced with simultaneous operations, immutable data structures help prevent unpredictable states and race conditions.
Here’s a demonstration of using an immutable List in Scala:
val originalList = List(1, 2, 3)
val newList = originalList :+ 4
println(originalList) // Output: List(1, 2, 3)
println(newList) // Output: List(1, 2, 3, 4)
As you can see, the original list remains unchanged, emphasizing the benefits of functional programming principles. In Java, mutable structures can lead to fragile code in multi-threaded environments.
4. Advanced Concurrency Model
Scala’s actor model via the Akka framework provides a highly-effective mechanism for handling concurrency. Rather than relying on traditional threading, the actor model allows each actor to process messages independently:
import akka.actor.Actor
import akka.actor.ActorSystem
import akka.actor.Props
class MyActor extends Actor {
def receive = {
case message: String => println(s"Received message: $message")
}
}
object Main extends App {
val system = ActorSystem("MySystem")
val myActor = system.actorOf(Props[MyActor], "myActor")
myActor ! "Hello, Actor"
}
This model abstracts the complexity of thread management, enabling developers to write more scalable code that remains easy to understand and maintain. For further insight, you might check out Akka's official documentation.
5. Powerful Collection Libraries
Scala comes with a rich set of collections that facilitate functional programming. Its collection library provides immutable and mutable collections, facilitating seamless operations on large datasets:
val data = List("apple", "banana", "cherry")
val lengths = data.map(_.length)
println(lengths) // Output: List(5, 6, 6)
With high-level abstractions, processing large datasets becomes more intuitive, reducing the cognitive load on developers.
Summary: When to Choose Scala Over Java for Scalability
While Java remains an excellent choice for numerous applications, Scala's advanced features significantly simplify scalability challenges. Key advantages include:
- Concise syntax reduces development complexity.
- First-class functions enhance reusability.
- Immutability fosters safe concurrent programming.
- Advanced concurrency support through the actor model.
- Rich collection libraries for better data handling.
Choosing Scala over Java for scalable applications can lead to improved agility, reduced cost of development, and a more efficient overall architecture.
Lessons Learned
As technology continues to advance, the demand for scalable solutions remains higher than ever. Scala's distinctive features render it particularly suitable for addressing the complexities of scalability inherent in modern applications. Java has undoubtedly paved the way and still holds significant value, but Scala is emerging as a formidable contender, especially in large enterprise applications.
For further reading on the importance of choosing the right programming language, be sure to check out this insightful article on scalability challenges and how to approach them effectively.
Explore the advantages of Scala to elevate your next project to new heights. Happy coding!