Struggling to Extract Common Name from X.509 in Java?
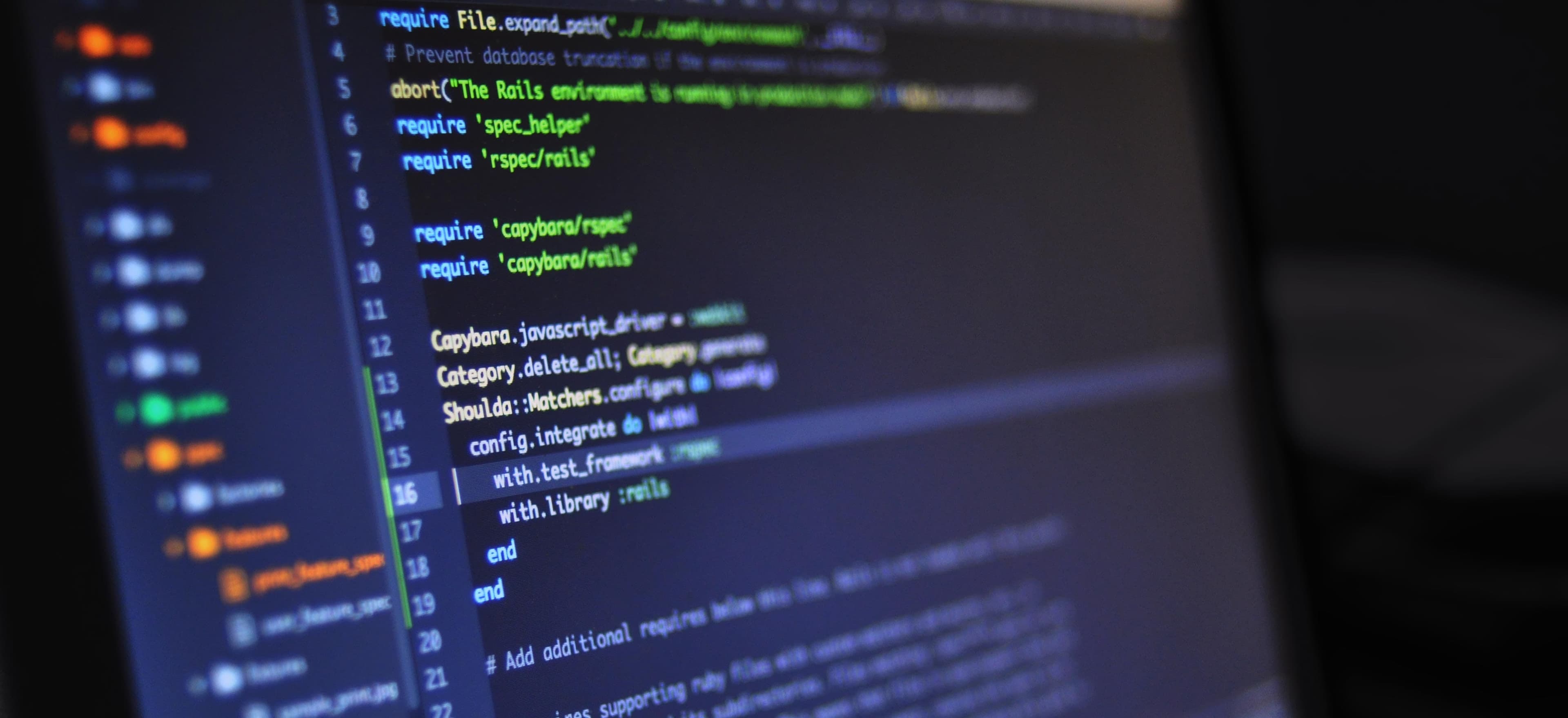
- Published on
Struggling to Extract Common Name from X.509 in Java?
When working with X.509 certificates in Java, one common task arises: extracting the Common Name (CN). The Common Name typically represents the entity the certificate represents, such as a server or user. Whether you're implementing secure communications or validating users, knowing how to retrieve this information is crucial. This post will guide you through the process step by step, showcasing code snippets along the way.
What is an X.509 Certificate?
An X.509 certificate is a digital certificate that uses the X.509 public key infrastructure standard to verify that a public key belongs to the individual, organization, or device it claims to represent. These certificates are widely used in various contexts, including SSL/TLS for secure web communication.
Why Do You Need to Extract the Common Name?
Extracting the Common Name can be essential for:
- Validating server identity in SSL connections.
- Ensuring the correct configuration in applications that rely on a specific certificate.
- Auditing and logging, where you might need to display the entity represented by the certificate.
How to Extract Common Name from X.509 in Java
Here's how you can extract the Common Name from an X.509 certificate in Java. You’ll need the following:
- Java Development Kit (JDK): Ensure you have it installed.
- An X.509 certificate for testing purposes.
Step 1: Loading the Certificate
First, let's load the certificate. You can load it from a file or a network location. Here, we will show how to load it from a .cer
file.
import java.io.FileInputStream;
import java.security.cert.Certificate;
import java.security.cert.CertificateFactory;
public class CertificateLoader {
public static Certificate loadCertificate(String filePath) throws Exception {
FileInputStream fis = new FileInputStream(filePath);
CertificateFactory cf = CertificateFactory.getInstance("X.509");
return cf.generateCertificate(fis);
}
public static void main(String[] args) {
try {
Certificate cert = loadCertificate("path/to/your/certificate.cer");
System.out.println("Certificate Loaded Successfully!");
} catch (Exception e) {
System.out.println("Failed to Load Certificate: " + e);
}
}
}
Why This Code Works:
- CertificateFactory enables the reading of the certificate in X.509 format.
- A FileInputStream is used to read the file containing the certificate.
Step 2: Extracting the X.509 Certificate
Now that we've loaded the certificate, let's extract it into a more usable format.
import java.security.cert.X509Certificate;
public class CertificateExtractor {
public static X509Certificate getX509Certificate(Certificate certificate) throws Exception {
return (X509Certificate) certificate;
}
public static void main(String[] args) {
try {
Certificate cert = CertificateLoader.loadCertificate("path/to/your/certificate.cer");
X509Certificate x509Cert = getX509Certificate(cert);
System.out.println("X.509 Certificate Extracted Successfully!");
} catch (Exception e) {
System.out.println("Failed to Extract X.509 Certificate: " + e);
}
}
}
Why This Code Works:
- The method casts the generic
Certificate
toX509Certificate
, allowing us to access more specific methods related to X.509.
Step 3: Extracting the Common Name
The Common Name can be found in the subject of the certificate. This is usually stored in a specific format within a Distinguished Name (DN)
.
import java.security.cert.X509Certificate;
import java.security.Principal;
public class CommonNameExtractor {
public static String extractCommonName(X509Certificate x509Certificate) {
Principal principal = x509Certificate.getSubjectDN();
String subject = principal.getName();
// Split the subject string and find CN
for (String part : subject.split(",")) {
if (part.trim().startsWith("CN=")) {
return part.substring(3).trim();
}
}
return null; // Return null if CN not found
}
public static void main(String[] args) {
try {
Certificate cert = CertificateLoader.loadCertificate("path/to/your/certificate.cer");
X509Certificate x509Cert = getX509Certificate(cert);
String commonName = extractCommonName(x509Cert);
System.out.println("Common Name: " + commonName);
} catch (Exception e) {
System.out.println("Failed to Extract Common Name: " + e);
}
}
}
Why This Code Works:
- The
getSubjectDN()
method retrieves the subject distinguished name of the certificate. - The
subject
string is split into its components, and we check for theCN=
prefix to retrieve the common name.
Complete Example in One Runnable Class
To simplify the process, here’s a complete example that puts everything together:
import java.io.FileInputStream;
import java.security.cert.Certificate;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import java.security.Principal;
public class X509CommonNameExtractor {
public static Certificate loadCertificate(String filePath) throws Exception {
FileInputStream fis = new FileInputStream(filePath);
CertificateFactory cf = CertificateFactory.getInstance("X.509");
return cf.generateCertificate(fis);
}
public static X509Certificate getX509Certificate(Certificate certificate) throws Exception {
return (X509Certificate) certificate;
}
public static String extractCommonName(X509Certificate x509Certificate) {
Principal principal = x509Certificate.getSubjectDN();
String subject = principal.getName();
for (String part : subject.split(",")) {
if (part.trim().startsWith("CN=")) {
return part.substring(3).trim();
}
}
return null; // Return null if CN not found
}
public static void main(String[] args) {
try {
Certificate cert = loadCertificate("path/to/your/certificate.cer");
X509Certificate x509Cert = getX509Certificate(cert);
String commonName = extractCommonName(x509Cert);
System.out.println("Common Name: " + commonName);
} catch (Exception e) {
System.out.println("Error: " + e);
}
}
}
Why This Code Works:
- It encapsulates certificate loading, extraction, and common name retrieval into a single efficient workflow.
- Console outputs are useful for debugging while developing the application.
A Final Look
Extracting the Common Name from an X.509 certificate in Java is a straightforward process. By following the steps outlined in this blog post, you can confidently handle certificate operations in your applications.
For further reading on certificates, you may find the following resources useful:
By mastering certificate management, you not only improve the security posture of your applications but also enhance your overall programming proficiency. Happy coding!
Checkout our other articles