Mastering CopyOnWriteArraySet: Avoiding ConcurrentModificationExceptions
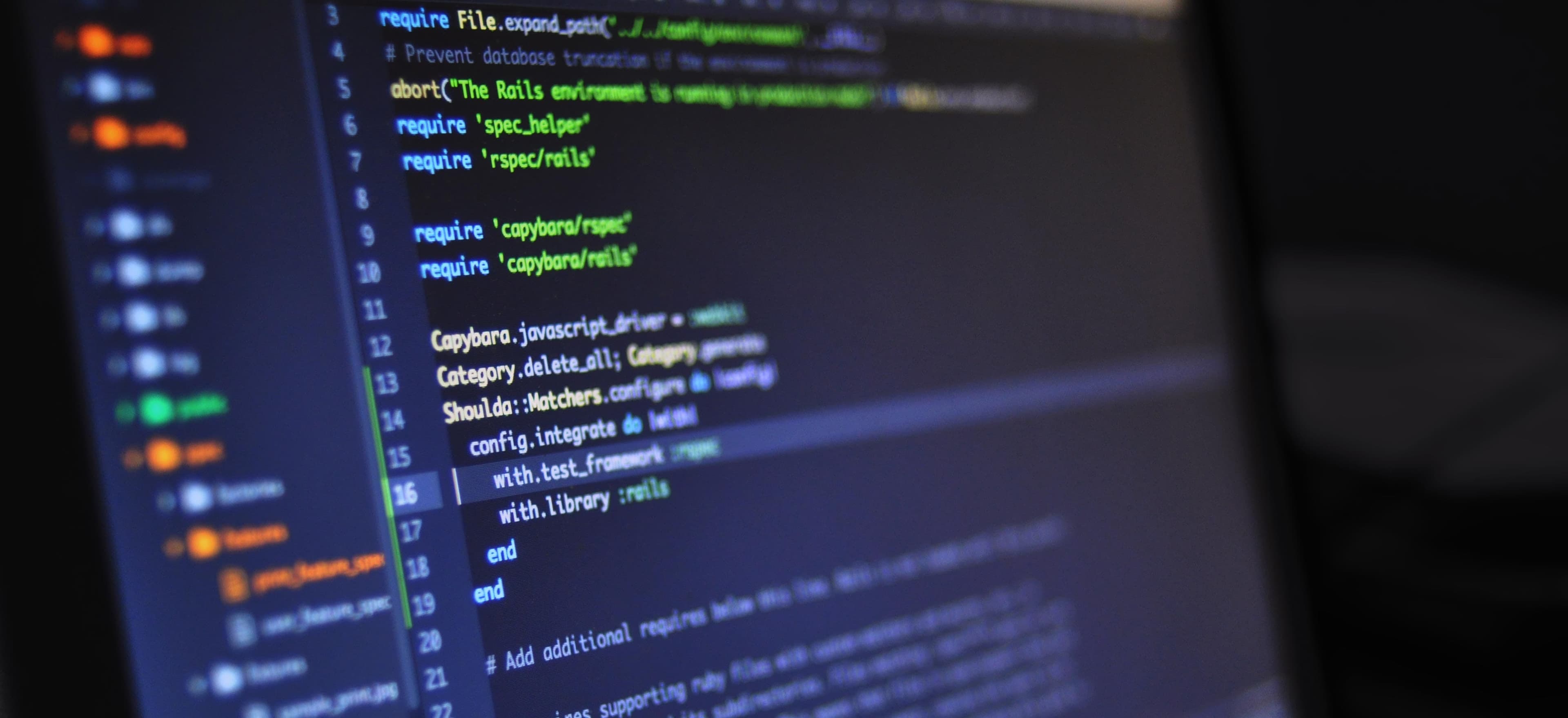
- Published on
Mastering CopyOnWriteArraySet: Avoiding ConcurrentModificationExceptions
In the ever-evolving landscape of Java's concurrency utilities, there's a plethora of collections designed to handle a variety of multi-threaded scenarios. Among these, CopyOnWriteArraySet
stands out for its unique approach to managing concurrent modifications while maintaining data integrity. In this blog post, we will delve into CopyOnWriteArraySet, emphasizing its design philosophy, use cases, and how it gracefully avoids ConcurrentModificationExceptions.
What is CopyOnWriteArraySet?
CopyOnWriteArraySet
is part of the Java Collections Framework and is found in the java.util.concurrent
package. It is an implementation of the Set
interface, backed by a CopyOnWriteArrayList
. This means that whenever the set is modified, a fresh copy of the underlying array is created. This architectural decision yields a set that is very efficient in navigating read operations, while still maintaining thread safety.
The Internal Mechanics
CopyOnWriteArraySet is designed on the foundation of the Copy-On-Write (COW) strategy. This fundamental mechanism allows you to read from the set without locking, thus ensuring that even when elements are concurrently added or removed, readers will not face visibility issues.
Here’s a brief outline of how it operates:
- Copy on Write: When modifications (like add, remove) are performed, the entire array is copied, making the write operation relatively resource-heavy.
- Reader-Writer Separation: Read operations can occur concurrently with modifications, thus allowing a high level of concurrent performance.
This leads to minimal contention among threads during read operations while ensuring safety during writes.
Why Use CopyOnWriteArraySet?
While traditional collections like HashSet
would throw a ConcurrentModificationException
when accessed simultaneously by multiple threads, CopyOnWriteArraySet
sidesteps this issue beautifully. But the question arises – when should you leverage CopyOnWriteArraySet? Here are several scenarios:
- Frequent Reads, Infrequent Writes: If your application primarily reads data but only occasionally modifies it. This scenario perfectly leverages the strengths of CopyOnWriteArraySet.
- Event Handling: In situations where events are published and consumed by multiple listeners, each listener may be reading from the set, while events may only add or remove listeners infrequently.
- Immutable Data: When the data included in your set is essentially static but may occasionally change.
Example: Using CopyOnWriteArraySet
Let’s dig deeper with some code to illustrate basic operations with CopyOnWriteArraySet
.
import java.util.concurrent.CopyOnWriteArraySet;
public class CopyOnWriteArraySetExample {
public static void main(String[] args) {
CopyOnWriteArraySet<String> set = new CopyOnWriteArraySet<>();
// Adding elements
set.add("Element 1");
set.add("Element 2");
System.out.println("Initial Set: " + set);
// Concurrent modification simulation
Thread writerThread = new Thread(() -> {
set.add("Element 3");
System.out.println("Added Element 3");
});
// Start a writer thread that modifies the set
writerThread.start();
// Reading from the set
for (String element : set) {
System.out.println("Reading: " + element);
try {
// Simulate some processing time
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
// Ensure writer thread completes execution
try {
writerThread.join();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Final Set: " + set);
}
}
Commentary
In the above code snippet:
- We start by initializing a
CopyOnWriteArraySet
. - We add a couple of elements to it and print the initial contents.
- A separate thread simulates a write operation by adding another element.
- Simultaneously, the main thread reads elements from the set.
This example underscores the beauty of CopyOnWriteArraySet
: even as modifications are occurring in another thread, the main thread can read the elements without running into ConcurrentModificationExceptions.
Performance Considerations
While CopyOnWriteArraySet
shines in read-heavy scenarios, it is vital to understand its performance trade-offs:
- Memory Overhead: Each write operation incurs a significant memory cost due to the need to create a copy of the underlying array. In scenarios where modifications are more frequent, alternative collections, like
ConcurrentHashMap
, may be more efficient. - Latency for Writes: Because write operations involve copying the array, they can introduce latency. Thus, it's less suited for scenarios where data changes frequently.
When NOT to Use
- High Write Frequency: If your application demands numerous write operations, consider using alternatives like
ConcurrentHashMap
or other synchronized collections. - Low Read Operations: If reading is infrequent, the overhead of copying the set becomes unnecessary.
Key Takeaways
In conclusion, CopyOnWriteArraySet
is a powerful tool for concurrent programming in Java, particularly in scenarios emphasizing read operations over writes. Its design effectively provides a solution to manage concurrent access without running into ConcurrentModificationExceptions
, thereby offering a safe and reliable container.
To further explore Java concurrency topics, consider visiting Oracle's Concurrency Tutorial and Java's Collection Framework Documentation.
By understanding CopyOnWriteArraySet
, Java developers can make informed decisions that enhance performance, reliability, and maintainability in their multi-threaded applications. Happy coding!