Common Pitfalls When Learning ANTLR for Beginners
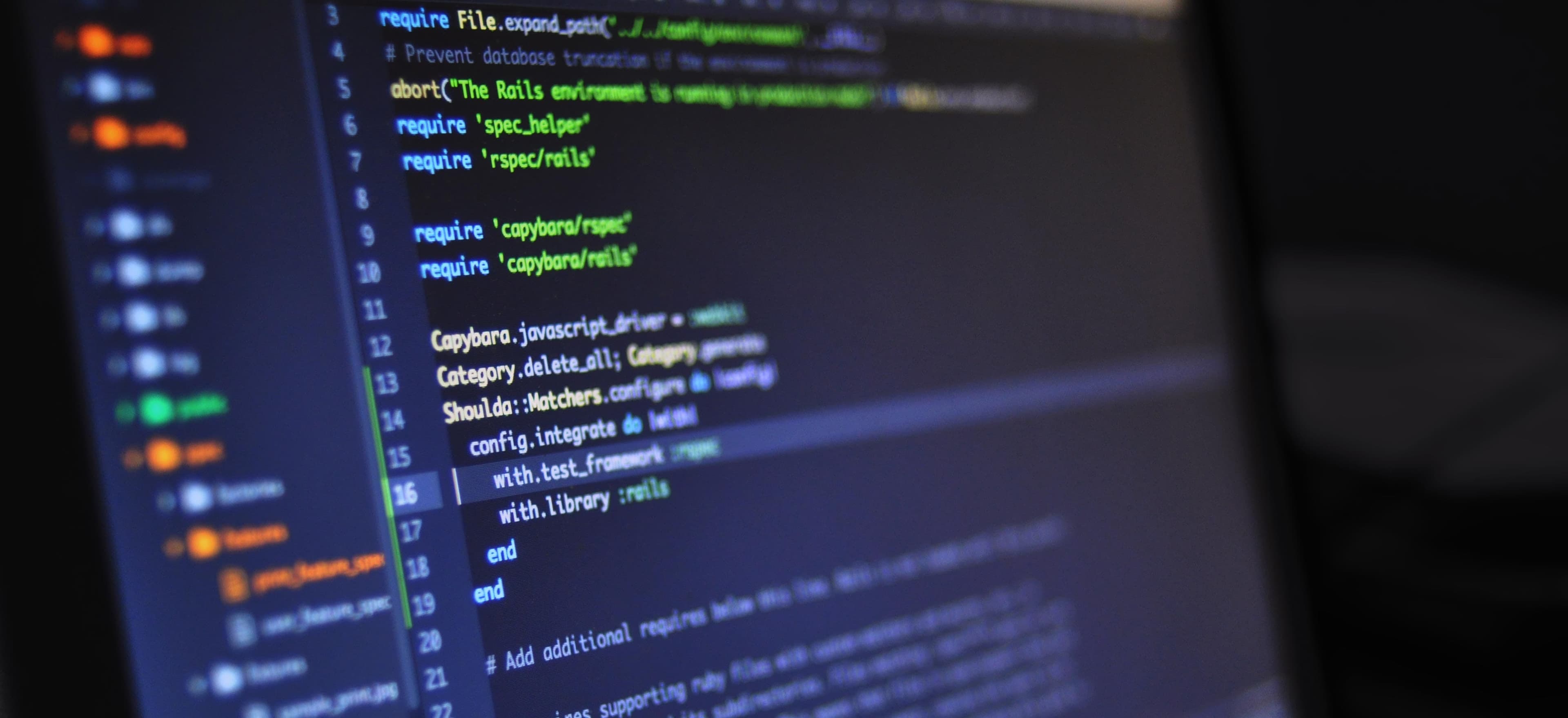
- Published on
Common Pitfalls When Learning ANTLR for Beginners
ANTLR, short for ANother Tool for Language Recognition, is a powerful tool used to create parsers for processing structured text. It allows developers to define a grammar for a language and generate code that will analyze that language. While ANTLR is an excellent tool for creating domain-specific languages or parsing data formats, beginners often run into several common pitfalls. In this blog post, we will explore these pitfalls, providing code examples and tips on how to overcome them.
1. Ignoring Grammar Design Principles
One of the first challenges a beginner faces is writing a suitable grammar. ANTLR requires a well-structured grammar file that accurately reflects the language being processed. Ignoring basic grammar design principles can lead to ambiguous or erroneous parser behavior.
Example
grammar SimpleExpression;
// The entry point of the grammar
expr: term (('+'|'-') term)* ;
// Define what a term is
term: factor (('*'|'/') factor)* ;
// Define what a factor is
factor: INT | '(' expr ')' ;
// Integer definition
INT: [0-9]+;
// Skip whitespace
WS: [ \t\r\n]+ -> skip;
Commentary
In this grammar, the use of operators to define expressions is straightforward. One common mistake is to create overly complex rules without understanding how they interact. Each rule should be modular and comprehensive, ensuring clarity and ease of understanding.
Tip: Start simple. Once you have a working grammar for a small subset of your language, you can expand gradually.
2. Neglecting Error Handling
As a beginner, it’s easy to overlook error handling in your grammar. ANTLR provides several mechanisms to deal with errors. Neglecting these can lead to frustrating experiences when debugging.
Example
expr: term (('+'|'-') term)* EOF;
term: factor (('*'|'/') factor)* ;
factor: INT | '(' expr ')' ;
INT: [0-9]+;
WS: [ \t\r\n]+ -> skip;
// Custom error handling
@members {
void recover(RecognitionException e) {
System.err.println("Error: " + e.getMessage());
}
}
// Throwing custom messages
fragment ERROR: . ;
Commentary
This example includes custom error handling to capture exceptions during parsing. Handling errors gracefully can save you countless debugging hours, as users will receive meaningful feedback instead of a generic stack trace.
Tip: Implement error recovery methods specific to your grammar rules, providing informative messages when failures occur.
3. Underestimating the Importance of Context
Another common pitfall involves misunderstandings of context-sensitive features of grammar. Beginners sometimes implement grammar rules that work well in isolation but conflict when parsed sequentially.
Example
grammar ContextExample;
program: statement+;
statement: assignment | functionCall;
assignment: ID '=' expr ';';
functionCall: ID '(' argList? ')' ';';
argList: expr (',' expr)*;
ID: [a-zA-Z_][a-zA-Z0-9_]*;
expr: INT | ID;
INT: [0-9]+;
WS: [ \t\r\n]+ -> skip;
Commentary
In this example, the context in which an ID
appears affects its meaning (whether it is part of an assignment or a function call). Beginners might mistakenly assume that ID
can only refer to one type of entity, leading to lack of clarity in how identifiers are resolved.
Tip: Pay close attention to how identifiers are used and defined in different contexts; using clear rule delineations helps avoid ambiguities.
4. Failing to Utilize ANTLR Toolset
ANTLR comes with sophisticated tools for debugging and visualizing grammar. New users may overlook these, opting instead to rely solely on print statements.
Visualization Example
You can visualize your grammar using ANTLRWorks or ANTLR’s built-in visualization capabilities. This interface helps you see how rules relate to each other.
!ANTLRworks Visualization
Commentary
Using tools like ANTLRWorks can help you track state transitions, making it easier to spot flaws in your grammar or understand the flow of parsing. It's much more efficient than manually tracing it through code.
Tip: Invest time in learning and utilizing the tools associated with ANTLR to enhance your understanding and productivity.
5. Misunderstanding Lexer vs. Parser Roles
Another frequent misstep for newcomers is conflating the lexer and parser responsibilities. The lexer is responsible for breaking input text into tokens, while the parser constructs a parse tree based on these tokens.
Example
In a grammar, maintaining separation of concerns is vital:
grammar HelloWorld;
// Lexer rules
HELLO: 'hello';
WORLD: 'world';
WS: [ \t\r\n]+ -> skip;
// Parser rules
greeting: HELLO WORLD;
Commentary
Here, the tokens HELLO
and WORLD
are managed separately from the primary grammar rules. Misunderstanding this separation can result in unintended errors or incomplete parsing.
Tip: Make sure to grasp the difference between lexer and parser rules to structure your grammar appropriately.
6. Not Writing Comprehensive Tests
Finally, beginners often neglect to test their grammars thoroughly. Just as you wouldn’t deploy software without testing, you should not assume your grammar works perfectly without rigorous validation.
Example
Create a simple test case to validate your grammar:
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.tree.*;
public class TestParser {
public static void main(String[] args) {
String input = "hello world"; // Test input
ANTLRInputStream inputStream = new ANTLRInputStream(input);
HelloWorldLexer lexer = new HelloWorldLexer(inputStream);
CommonTokenStream tokens = new CommonTokenStream(lexer);
HelloWorldParser parser = new HelloWorldParser(tokens);
ParseTree tree = parser.greeting(); // Initiating parse
System.out.println(tree.toStringTree(parser)); // Verify parse tree
}
}
Commentary
In this code snippet, you create a simple test case that executes the parser against the input string "hello world". Running such tests frequently helps catch issues early in the development phase.
Tip: Develop a set of unit tests for your grammar early on. Consider edge cases as well as typical inputs.
In Conclusion, Here is What Matters
Learning ANTLR can be challenging but incredibly rewarding. By being aware of common pitfalls such as ignoring grammar design principles, misunderstanding the lexer and parser distinction, neglecting error handling, and failing to visualize processes, you will save time and improve your learning curve.
As you gain experience with ANTLR, consider reading the solid foundations of ANTLR grammar to further deepen your skills. With practice and diligence, you will harness the full potential of ANTLR, making language parsing a breeze.
By embracing these tips and avoiding these pitfalls, you will be well on your way to mastering ANTLR and creating powerful parsers for your own projects. Happy parsing!