Struggling with Android Scrollable Layout? Here's the Fix!
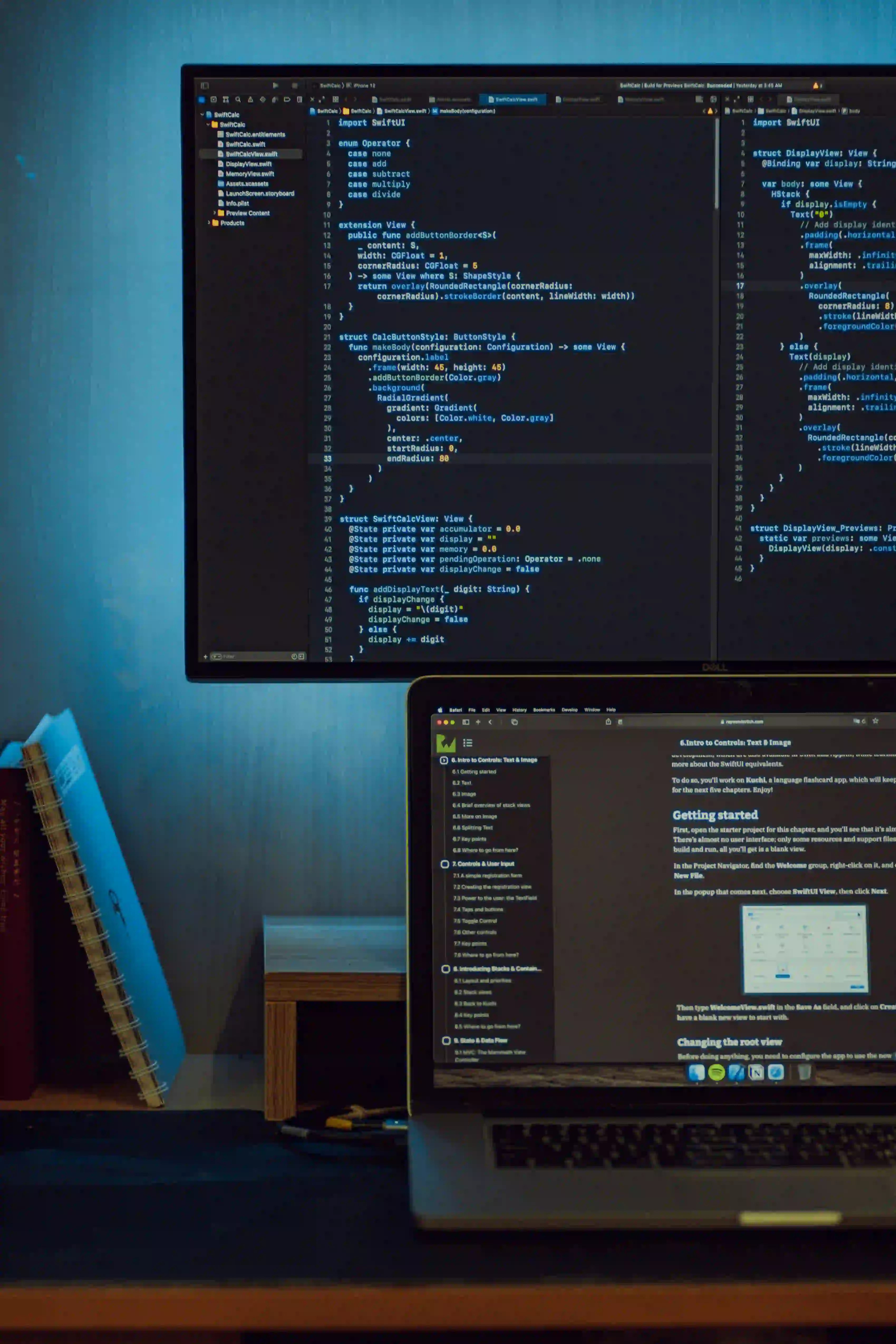
Struggling with Android Scrollable Layout? Here's the Fix!
Creating a user-friendly interface is paramount in Android development. A common challenge developers face involves implementing scrollable layouts effectively. Whether it's a long list of information or dynamic content, managing scrollable views can be a daunting task. In this blog post, we will delve into common pitfalls and provide practical solutions to ensure your Android app delivers a smooth scrolling experience.
Understanding Scrollable Layouts in Android
In Android development, scrollable layouts allow users to navigate through views when they exceed the screen's dimensions. The two most commonly used scrollable layouts are ScrollView
and RecyclerView
.
- ScrollView: A simple way to make a single view scrollable. Ideal when you want to have a vertically scrolling list of flexible layouts.
- RecyclerView: A more advanced and flexible version, designed for performance, especially with large datasets. It is essential for lists where each item may have a different size.
When to Use Which?
- Use
ScrollView
when you have a small number of views and you require a straightforward vertical scrolling. - Opt for
RecyclerView
for lists that may grow large, where you want to leverage an adapter to manage view recycling and improve performance.
Creating a ScrollView Example
Let’s start by creating a basic example of a ScrollView
. Below is how you can implement a simple layout:
<!-- res/layout/activity_main.xml -->
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Welcome to Scrollable Layout"
android:textSize="24sp"
android:padding="16dp"/>
<!-- Add more views here -->
</LinearLayout>
</ScrollView>
Why This Works: The ScrollView
allows for vertical scrolling of LinearLayout
, enabling all child views to be accessible, even if they exceed the screen size.
Handling the Nested Scollable Issue
Sometimes a tricky scenario arises when you have a ScrollView
nested within another scrollable view. This can lead to poor user experience and scrolling issues. Instead, consider using RecyclerView
for a more robust solution.
Working with RecyclerView
If you are handling data that can be numerous and unpredictable, RecyclerView
is your best friend. Follow these steps to incorporate RecyclerView
effectively:
Step 1: Add Dependencies
If you haven't already added the RecyclerView dependency, add the following line to your build.gradle
file:
dependencies {
implementation 'androidx.recyclerview:recyclerview:1.2.1'
}
Step 2: Create RecyclerView Layout
Here’s an example layout for using RecyclerView
.
<!-- res/layout/activity_main.xml -->
<androidx.recyclerview.widget.RecyclerView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
Step 3: Create an Adapter
An adapter bridges the data to the RecyclerView's layout. Below is a concise implementation:
// MyAdapter.java
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.ViewHolder> {
private List<String> dataList;
public MyAdapter(List<String> dataList) {
this.dataList = dataList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_view, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
holder.textView.setText(dataList.get(position));
}
@Override
public int getItemCount() {
return dataList.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
public TextView textView;
public ViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(R.id.textView);
}
}
}
Why Use an Adapter?: The adapter manages the recycling of views, which is critical for performance. Instead of creating a new view for each item, it reuses the existing ones, making scrolling smoother.
Step 4: Set Up the RecyclerView in Your Activity
// MainActivity.java
public class MainActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private MyAdapter myAdapter;
private List<String> dataList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recyclerView);
dataList = new ArrayList<>();
// Add data to the list
for (int i = 0; i < 100; i++) {
dataList.add("Item " + i);
}
myAdapter = new MyAdapter(dataList);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(myAdapter);
}
}
Why a Layout Manager?: The LayoutManager
defines how elements are laid out within the RecyclerView, crucial for efficient scrolling.
Troubleshooting Common Issues
-
Nested ScrollView with RecyclerView: Avoid using ScrollView with Finder classes like RecyclerView. If you must, ensure you utilize the appropriate measures to manage touch events.
-
Slow scrolling: Ensure that item layouts in your RecyclerView are as lightweight as possible. Utilize view holders properly.
-
Dynamic Height of RecyclerView: If you notice your
RecyclerView
isn't scrolling, make sure that its height isn't set towrap_content
in the layout file.
Additional Resources
For a deeper dive into RecyclerView
, consider checking out official documentation or [Android Developers YouTube Channel](https://www.youtube.com/c/Android Developers).
The Closing Argument
Navigating through scrollable layouts in Android doesn’t have to be a struggle. By leveraging RecyclerView
, you can create scalable, efficient, and smooth scrolling experiences. Remember to keep your designs clean and lightweight to prevent any performance issues down the line.
Whether you're integrating a simple ScrollView
or a complex RecyclerView
, understanding when to use each is vital. Embrace these practices, and your users will thank you for an improved experience. Happy coding!