Overcoming Microservices Complexity in Tech Stacks
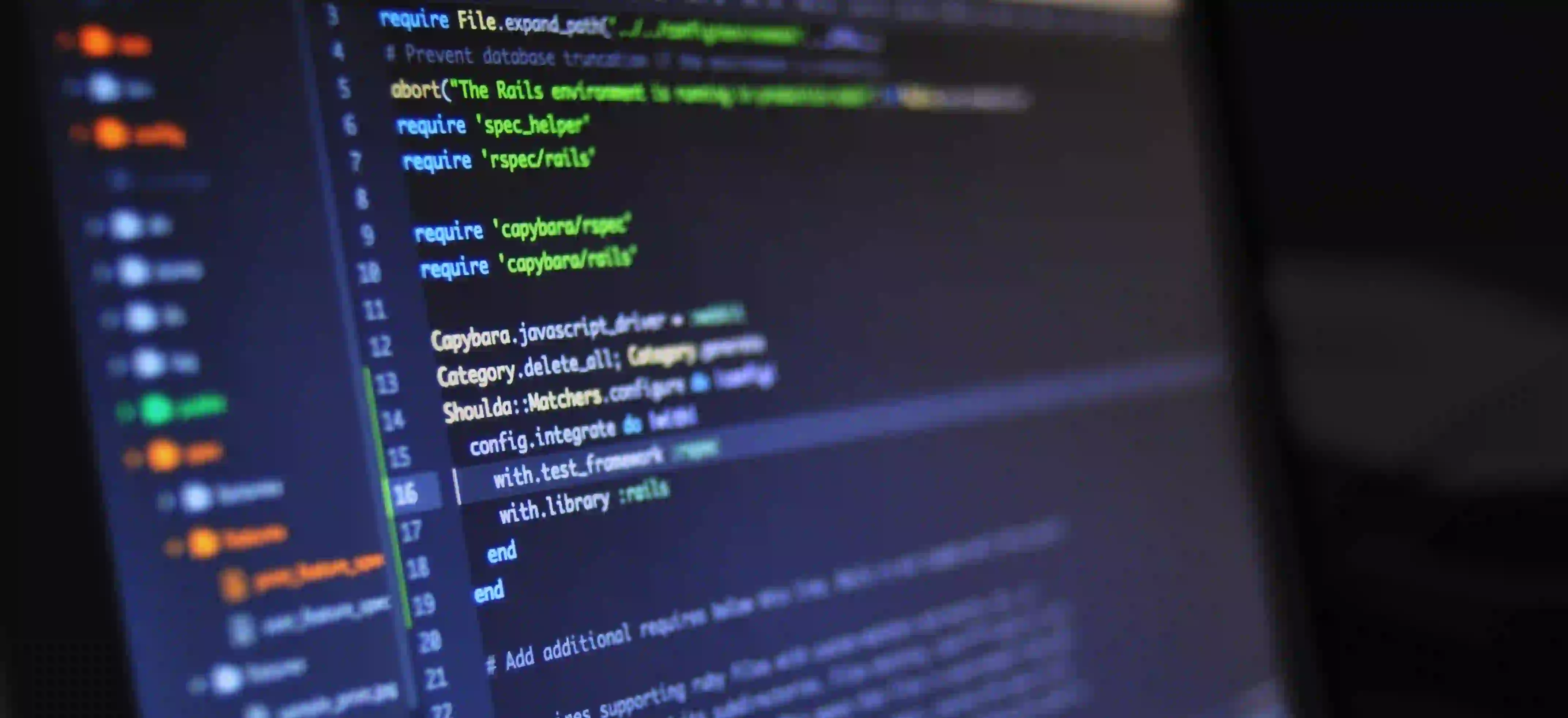
Overcoming Microservices Complexity in Tech Stacks
In today's fast-paced tech landscape, the microservices architecture has emerged as a revolutionary approach to developing robust and scalable applications. However, as with any transformative technology, it brings its set of complexities. In this blog post, we will explore the intricacies of microservices, practical solutions to manage the associated challenges, and best practices for implementing microservices in your tech stack.
Understanding Microservices Architecture
Microservices architecture breaks down a monolithic application into smaller, self-contained services. Each service is responsible for a specific business capability and communicates with others through APIs (Application Programming Interfaces). While this modular approach promotes scalability, fault tolerance, and independent deployment, it can lead to complexity.
Advantages of Microservices
- Scalability: Each service can be scaled independently based on demand.
- Technology Agnostic: Teams can choose the best technology stack for each service without imposing restrictions on other teams.
- Resilience: Failure in one service does not crash the entire application.
Despite these advantages, organizations can find themselves grappling with the following challenges:
Common Challenges in Microservices
-
Service Communication and Data Management: Ensuring reliable communication between services can be cumbersome. With multiple services communicating over the network, latency, and failure become significant concerns.
-
Deployment and Orchestration: Coordinating the deployment of multiple microservices is more complex than managing a single monolithic application. Tools like Kubernetes can help, but they come with their learning curve.
-
Monitoring and Logging: Tracking the performance and health of numerous microservices requires advanced monitoring solutions. Without proper observability, debugging and issue resolution can become time-consuming.
-
Data Consistency: Unlike monolithic applications that use a single database, microservices often need to manage data consistency across multiple databases.
-
Security Concerns: Each service introduces security vulnerabilities. Properly securing APIs and managing authentication and authorization become more complex.
Strategies to Overcome Microservices Complexity
1. Adopt Service Discovery
Using a service discovery tool can help automate the detection of service instances in a microservices architecture. Rather than hardcoding the service locations, a service discovery tool like Netflix Eureka or Consul enables dynamic routing and load balancing.
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@EnableEurekaClient
public class MyServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MyServiceApplication.class, args);
}
}
Why: This code snippet shows how to enable Eureka Client in a Spring Boot application, allowing it to register with the Eureka server for seamless service discovery.
2. Utilize API Gateway
An API Gateway acts as a single entry point for all client requests. It simplifies management by handling authentication, load balancing, caching, and routing requests to the appropriate microservices.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class ApiGatewayController {
@Autowired
private MyService myService;
@GetMapping("/data")
public ResponseEntity<String> getData() {
return ResponseEntity.ok(myService.getData());
}
}
Why: This simple API Gateway snippet shows how to create an endpoint that routes client requests to the corresponding microservice. By consolidating requests, an API Gateway streamlines communication, reducing complexity.
3. Implement Centralized Logging and Monitoring
Monitoring solutions like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus can centralize logs and metrics from different services. This provides a unified view of the system, enhancing observability.
For additional context, check this comprehensive guide for setting up ELK Stack.
{
"log_level": "INFO",
"services": {
"user-service": "active",
"order-service": "active"
}
}
Why: Centralized logging allows you to quickly gather logs from various services, facilitating easier troubleshooting.
4. Use Database Per Service Pattern
Avoid sharing a single database across microservices to maintain autonomy. Instead, implement a database per service pattern to reduce coupling and enhance data ownership.
CREATE TABLE users (
id INT PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(100) UNIQUE
);
Why: Each service can manage its database schema independently, which is critical for ensuring that services can evolve without impacting others.
5. Secure APIs
Implement robust security mechanisms, such as OAuth2, for API authentication. This will help protect sensitive data and secure communications between services.
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("client-id")
.secret("{noop}client-secret")
.authorizedGrantTypes("password", "authorization_code")
.scopes("read", "write");
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.authenticationManager(authenticationManager);
}
}
Why: This configuration effectively secures your microservices with OAuth2, reducing the risk of unauthorized access.
Best Practices for Microservices Adoption
-
Start Small: Don’t migrate everything to microservices at once. Start with one or two services, and gradually evolve your architecture.
-
Define Clear Boundaries: Ensure that each microservice has a well-defined purpose. This helps maintain the autonomy of each service and reduces interdependencies.
-
Automate Testing: Continuous testing is crucial. Utilize CI/CD pipelines to automate the building, testing, and deployment of microservices.
-
Facilitate Communication: Regular communication between teams managing different microservices helps avoid misunderstandings and fosters collaboration.
-
Document Everything: Clear documentation is essential to manage complexity and onboard new team members effectively.
Key Takeaways
Microservices architecture has the potential to revolutionize how applications are built and managed. While it introduces a layer of complexity, these challenges can be effectively managed through thoughtful design choices and practices. By adopting service discovery, utilizing API Gateways, implementing centralized logging, and enforcing robust security mechanisms, organizations can harness the full power of microservices while mitigating the associated risks.
For further reading on building microservices, check out Martin Fowler's article on Microservices, which offers a more in-depth view on architecture principles.
Adopting microservices is a journey, one that will certainly lead you toward better scalability, resilience, and agility in software development. Start embracing this architecture today, and experience the transformation yourself!