Mastering Gradle: Overcoming Common Command Line Pitfalls
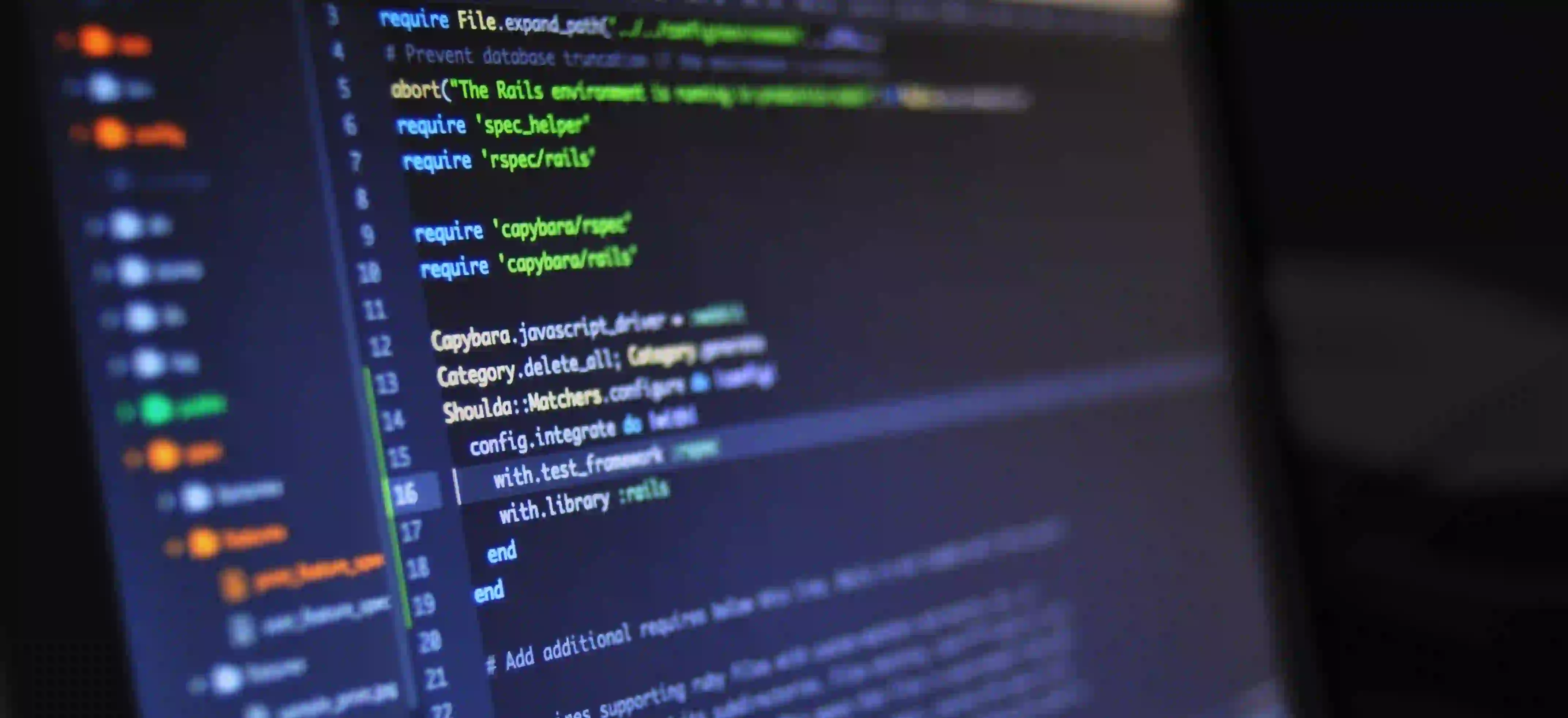
Mastering Gradle: Overcoming Common Command Line Pitfalls
Gradle is a powerful build automation tool that has gained immense popularity among Java developers. As the backbone of many modern Java projects, it simplifies project management and dependency handling. However, mastering Gradle's command line can be daunting due to its vast array of commands and options.
In this blog post, we will discuss common pitfalls encountered when using Gradle from the command line and how to tackle them effectively. We will include practical code snippets and explanations to ensure clarity.
Table of Contents
What is Gradle?
Gradle is an open-source build automation tool that combines the best features of other build systems. It provides flexibility with its build scripts written in Groovy or Kotlin and supports a wide range of languages. You can find more about Gradle on its official documentation page.
Common Command Line Pitfalls
Navigating Gradle's command line might lead to errors or undesired outcomes. Here are some common issues developers face and how to overcome them.
1. Gradle Wrapper vs. Gradle Installation
One of the first traps developers fall into is confusion between the Gradle Wrapper and the locally installed Gradle.
The Wrapper: The Gradle Wrapper allows you to run Gradle tasks without needing a local Gradle installation. It ensures that the correct Gradle version is used for the project.
Usage: To use the Wrapper, you typically run:
./gradlew build
This command invokes the Gradle Wrapper script. It's particularly useful in CI/CD pipelines where you want consistent environments.
Misstep: Using the system-installed Gradle can lead to compatibility issues if the project expects a specific Gradle version.
Correction: Ensure you always use ./gradlew
to avoid potential mismatches between Gradle versions, particularly in collaborative environments. More on the Gradle Wrapper can be found in the official docs.
2. Misunderstanding Build Scopes
Gradle manages different build configurations, which can be confusing for new users. Often developers set tasks or configurations in the wrong scope.
Example: A common mistake is defining a task within task
block instead of in project.afterEvaluate
for tasks depending on project properties.
task exampleTask {
doLast {
println "Running example task"
}
}
Correct Approach: Depending on the project evaluation phase, you might use:
project.afterEvaluate {
task exampleTask {
doLast {
println "Task run after project evaluation"
}
}
}
Why This Matters: Using project.afterEvaluate
ensures that your task has access to all the project properties, preventing undefined issues when the task is run. Understanding the lifecycle of a build can help mitigate these issues.
3. Ignoring Dependency Management
Dependency management is a core feature of Gradle. New developers often overlook this, leading to incorrect builds or missing libraries.
Misunderstanding Transitive Dependencies: You may declare dependencies, but if you don't understand how they propagate, you might end up with version conflicts.
dependencies {
implementation 'org.springframework:spring-core:5.3.0'
implementation 'org.springframework:spring-context:5.3.0'
}
Concern: What if a transitive dependency is causing an error?
Solution: Use the dependency insight command:
./gradlew dependencies --configuration compileClasspath
This command provides a tree view of the dependencies. You can analyze what's being pulled into your project.
Resolution: To manage versions better, consider using a dependency management plugin like Spring Dependency Management Plugin. This allows you to control versions in a more centralized manner.
4. Command Line Arguments
Command line arguments can modify build behavior, but incorrect usage can lead to build failures.
Example: Pass properties via the command line can be done as follows:
./gradlew build -Penv=production
If you forget to define env
in the build.gradle
, it will throw an error.
Solution: Always verify if command line properties are handled correctly in your build script. Check for these properties:
if (project.hasProperty('env')) {
println "Environment: ${project.property('env')}"
}
This will ensure that your script can gracefully handle missing or unexpected properties. For a comprehensive guide on properties in Gradle, refer to the official documentation.
Best Practices
To maximize your productivity with Gradle, consider the following best practices:
-
Use the Wrapper: Always opt for the Gradle Wrapper whenever possible. It ensures consistency in builds across different environments.
-
Break Down Build Scripts: Keep your
build.gradle
clean and modular. Use separate files or apply plugins for organization. -
Leverage Gradle Caching: Gradle's caching capabilities enhance build times significantly. Optimize your builds by enabling caching.
-
Version Control Your Gradle Files: Ensure
gradle-wrapper.properties
andbuild.gradle
are under version control to maintain project integrity. -
Regularly Monitor Dependencies: Use tools like the Gradle Dependency Insight report to catch potential pitfalls early.
The Bottom Line
Mastering Gradle command line is crucial for effective Java development. By understanding its nuances, you can avoid common pitfalls and enhance your productivity. Keep experimenting with Gradle, leverage its extensive features, and you're sure to see improvements in your build management routines.
Gradle is more than just a build tool; itβs the backbone of modern development practices. For a deeper dive into Gradle, explore the official documentation.
Ready to take your Gradle skills to the next level? Start implementing these best practices today and watch your build processes transform. Happy coding!