Troubleshooting Common Java Deployment Issues on Heroku
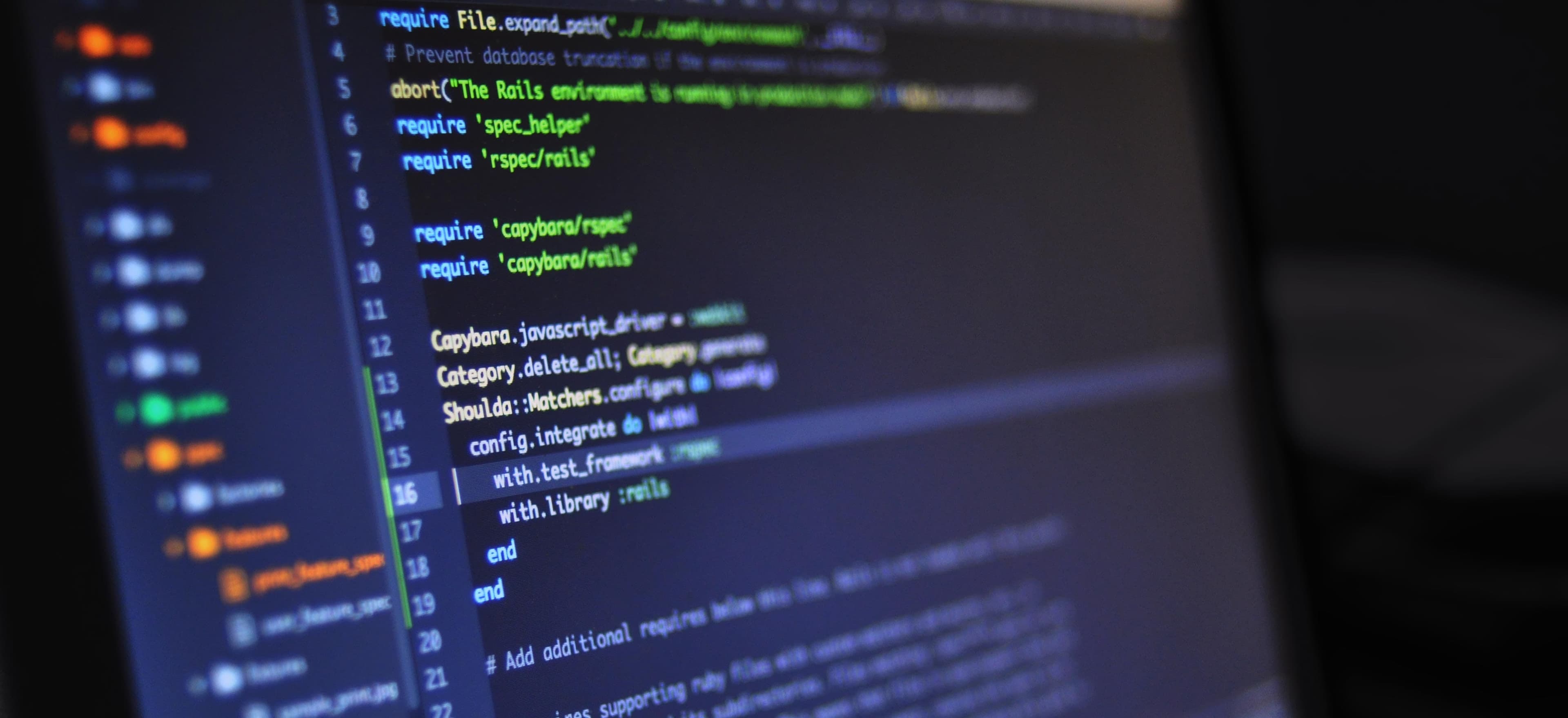
- Published on
Troubleshooting Common Java Deployment Issues on Heroku
Deploying Java applications on Heroku can be a straightforward process, but it is not without its pitfalls. In this article, we will explore common issues developers face when deploying Java applications to Heroku, provide troubleshooting steps, and offer best practices to ensure a smooth deployment. Whether you’re deploying a Spring Boot application or a simple Java servlet, this guide will help you navigate the challenges effectively.
Understanding the Heroku Environment
Before diving into troubleshooting, it is essential to understand the Heroku environment. Heroku abstracts much of the server management away, focusing instead on the application. However, this can sometimes lead to confusion regarding configuration and dependencies.
Heroku runs applications within "dynos"—containers that run your app. This means that any aspect of your application needing configuration must be defined either in the code or through environment settings. Understanding how Heroku manages Java applications will significantly ease the troubleshooting process.
Key Components
-
Procfile: This file tells Heroku how to run your application. A typical
Procfile
for a Spring Boot application would look like this:web: java -jar target/myapp.jar
-
Buildpacks: Buildpacks are scripts that automate the setup of your app’s environment on Heroku. The Java buildpack is usually included by default, but you can specify custom buildpacks if necessary.
-
Environment Variables: Critical for DB connections and API keys, these configuration details must be set correctly in Heroku’s settings.
Common Deployment Issues and How to Fix Them
1. Build Failures
Problem: Build failures can occur due to various issues, such as incorrect configurations in the pom.xml
or missing dependencies.
Solution: Check your build logs for specific error messages. Common issues often relate to:
-
Unresolved Dependencies: Ensure that all dependencies are specified correctly in your
pom.xml
.<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>2.5.4</version> </dependency> </dependencies>
-
Incorrect Java Version: Make sure you specify the correct Java version in your
system.properties
file:java.runtime.version=11
2. Application Crashes on Startup
Problem: If your app crashes immediately upon startup, it often indicates a misconfiguration or missing resource.
Solution: Review your application logs using Heroku CLI:
heroku logs --tail
Look for stack traces in the logs and address the exceptions. Common reasons include:
-
Port Configuration: Heroku dynamically assigns ports, so your app should listen on the port provided by the
PORT
environment variable:public static void main(String[] args) { SpringApplication app = new SpringApplication(MyApp.class); app.addListeners(new ApplicationPidFileWriter("myapp.pid")); app.run(args); } @Override public void run(String... args) { System.setProperty("server.port", System.getenv("PORT")); }
-
Missing Environment Variables: Ensure that all required environment variables are set in Heroku. You can set environment variables using:
heroku config:set MY_VARIABLE=value
3. Database Connection Issues
Problem: Database errors significantly hinder app functionality. These include authentication failures or connection timeouts.
Solution: Confirm your database configuration in the application.properties
file. For example, using PostgreSQL, your file should include:
spring.datasource.url=jdbc:postgresql://<DB_HOST>:<DB_PORT>/<DB_NAME>
spring.datasource.username=<DB_USERNAME>
spring.datasource.password=<DB_PASSWORD>
Make sure Heroku's database URL is retrieved correctly, typically set up by the JDBC connection string.
4. Misconfigured Buildpacks
Problem: Incorrect buildpack usage can lead to various runtime issues or inability to deploy at all.
Solution: Verify that you are using the correct buildpack for Java applications:
heroku buildpacks:set heroku/java
5. Health Check Failures
Problem: Heroku conducts health checks, and if your application doesn’t respond correctly, it will be marked as unhealthy.
Solution: Ensure your application is healthy and responds to HTTP requests. You may need to adjust your health check endpoints or configuration in the application.properties
file:
management.endpoint.health.show-details=always
6. File Not Found Exception
Problem: Customers often face issues when their app cannot locate necessary files (like configuration files).
Solution: Ensure that any files your application needs are included in your build. In Maven projects, for example, ensure relevant files are located in the src/main/resources
folder and configured correctly.
7. Security Settings
Problem: Issues with CORS (Cross-Origin Resource Sharing) can arise for web applications trying to connect to APIs.
Solution: Handle CORS properly. In a Spring Boot application, configure CORS globally as follows:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("*");
}
}
8. Log Management
Problem: Poor visibility into application behavior can complicate troubleshooting.
Solution: Use Heroku logs effectively to monitor your application. You can also integrate logging libraries like Logback or Log4J for better log management.
Best Practices for Heroku Java Deployments
Now that we've addressed common issues, here are some best practices to keep in mind while deploying your Java application on Heroku:
-
Use Environment Variables: Avoid hardcoding sensitive information. Use environment variables for database URLs, API keys, and other sensitive data.
-
Consistent Testing: Always test your application locally before deploying. Test with a similar database setup to minimize discrepancies.
-
Monitor Performance: Utilize Heroku's monitoring tools to analyze performance metrics and catch issues early.
-
Manage Dependencies: Regularly update dependencies to avoid security vulnerabilities.
-
Health Checks: Implement health check endpoints to ensure your application is alive and functioning correctly.
-
Documentation: Maintain thorough documentation for your application, including deployment guidelines.
Key Takeaways
Deploying Java applications on Heroku can often be a seamless experience if best practices are followed. However, being prepared to troubleshoot issues such as build failures, connection problems, and other misconfigurations is crucial for success. By carefully reviewing logs, using environment variables, and following the best practices outlined in this article, you can enhance the reliability and performance of your Java applications on Heroku.
For further reading on Heroku and Java deployments, refer to:
By understanding potential issues and how to address them proactively, you'll ensure a much smoother deployment process. Happy coding!
Checkout our other articles