Troubleshooting Common Spring Integration Session Issues
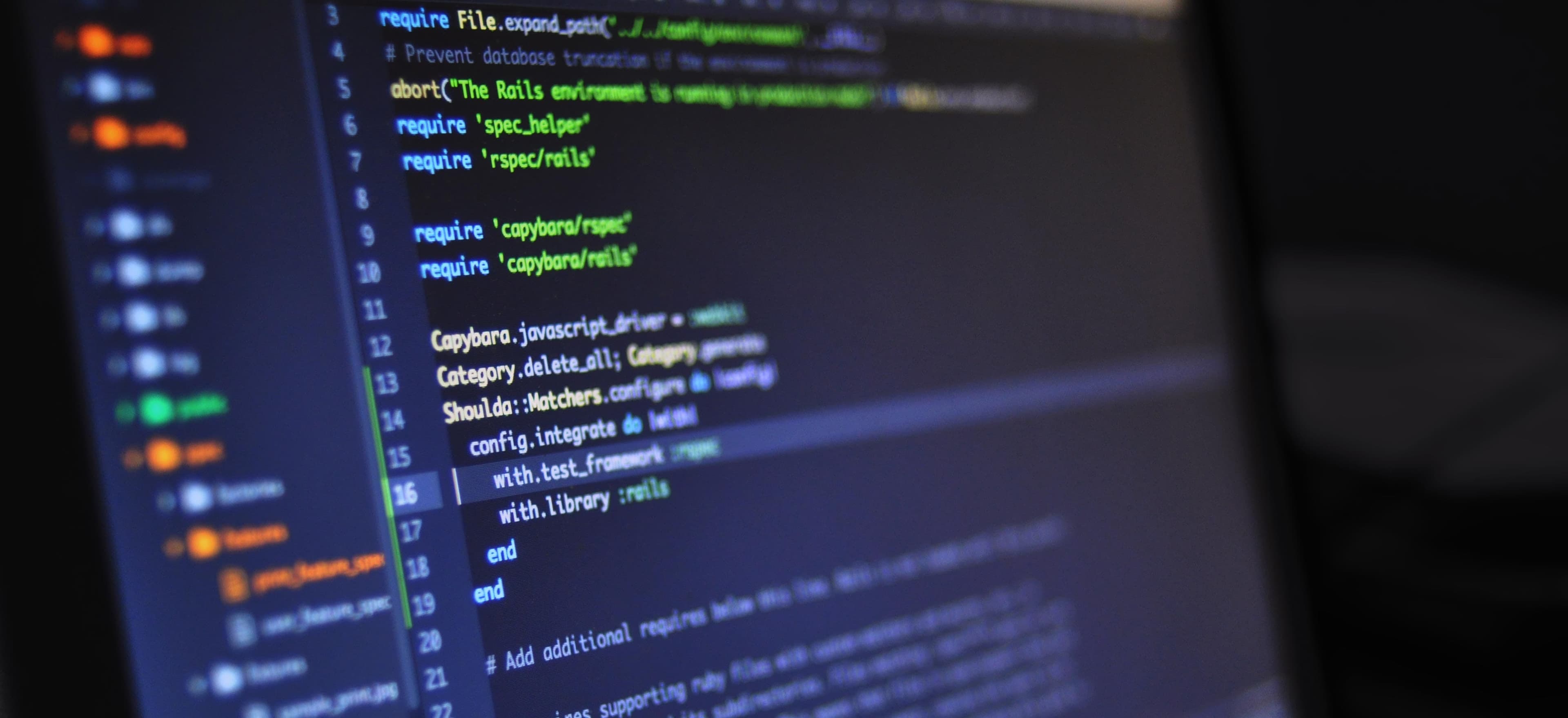
- Published on
Troubleshooting Common Spring Integration Session Issues
Spring Integration is a powerful framework that allows Spring applications to communicate with external systems effectively. It facilitates integration points by handling message routing, transformation, and processing. However, when dealing with session management in Spring Integration, developers often encounter challenges that can impede application performance. In this blog post, we will explore common Spring Integration session issues and propose troubleshooting strategies to help developers overcome these obstacles.
Understanding Spring Integration Sessions
Before diving into troubleshooting, it's crucial to understand what a session is in the context of Spring Integration. A session in Spring Integration typically refers to a context for managing state, which can include both stateful and stateless integration flows. Sessions can involve managing user information, preserving data across multiple requests, or even maintaining transactional states.
Common Session Issues in Spring Integration
-
Session Not Being Created or Persisted
A frequent issue developers face is that the session may not be getting created as expected. This can lead to issues such as null pointer exceptions when trying to access session attributes.Troubleshooting Steps:
- Check Configuration:
Ensure that your Spring integration configuration is properly set up to handle session state. Review your
applicationContext.xml
or Java configuration classes. Make sure that the relevant beans for session management (such asHttpSession
) are defined.
Example Configuration:
<bean id="sessionFactory" class="org.springframework.web.context.request.RequestContextHolder" />
The above bean declaration ensures that the Spring context is aware of the session factory.
- Verify Session Scope:
Ensure that the beans requiring session state are annotated with
@SessionScope
if you're using a recent Spring version:
@Component @SessionScope public class UserSession { private String userId; public String getUserId() { return userId; } public void setUserId(String userId) { this.userId = userId; } }
- Check Configuration:
Ensure that your Spring integration configuration is properly set up to handle session state. Review your
-
Session Timeout Problems
Another common issue is premature session timeouts. This occurs when the session expires before its intended lifecycle.Troubleshooting Steps:
- Adjust Timeout Settings:
Check your web application’s session timeout settings as defined in
web.xml
or configuration class.
<session-config> <session-timeout>30</session-timeout> <!-- In minutes --> </session-config>
Here, ensure that the timeout is sufficient for your application's workloads.
- Handle Session Refresh:
Sometimes long-running tasks may leave the session idle. Implement mechanisms to refresh the session programmatically. For example, you might call
request.getSession().setMaxInactiveInterval(...)
to extend the session timeout before critical operations.
- Adjust Timeout Settings:
Check your web application’s session timeout settings as defined in
-
Concurrent Session Issues
When multiple concurrent requests try to access or modify session data, conflicts can occur. This can lead to inconsistent read states or agent deadlocks.Troubleshooting Steps:
- Implement Synchronization: Use synchronized blocks or locks to prevent concurrent access.
public synchronized void updateSessionData(String data) { // your logic here }
- Consider Session Replication: If your application involves multiple nodes, consider using session replication strategies (such as Redis or Memcached). This enables sessions to be shared across nodes and provides a fallback mechanism in case one node goes down.
-
Serialization Issues
Serialization problems can emerge when objects stored in a session fail to serialize properly, leading toInvalidClassException
or other related errors.Troubleshooting Steps:
- Verify Serializable Implementation:
Ensure that all objects placed in the session implement
java.io.Serializable
.
public class UserData implements Serializable { private static final long serialVersionUID = 1L; private String name; private List<String> roles; }
- Test Serialization Independently: Test object serialization and deserialization in isolation to catch issues early, before they propagate to session-related errors.
- Verify Serializable Implementation:
Ensure that all objects placed in the session implement
-
Resource Leaks
Finally, session management often suffers from resource leaks, particularly if sessions are not being cleaned up properly.Troubleshooting Steps:
-
Monitor Session Lifecycle: Utilize profiling and monitoring tools to track active sessions and identify unused sessions. Spring Actuator can provide valuable metrics around session management.
-
Implement Cleanup Logic: If certain sessions no longer need to persist, implement regular cleanup processes. For instance, you could implement a periodic task to invalidate sessions based on specific conditions.
@Scheduled(fixedRate = 60000) // Runs every minute public void cleanupSessions() { sessionRegistry.getAllSessions().forEach(session -> { if (shouldBeInvalidated(session)) { session.invalidate(); } }); }
-
Useful Links for Further Reading
For those who want to delve deeper into the nuances of session management within Spring Integration, here are two useful resources:
Closing Remarks
Dealing with session management in Spring Integration can present several challenges. By understanding the common issues and following a systematic troubleshooting approach, developers can enhance their applications' robustness and reliability. With careful configuration, appropriate synchronization, and a focus on the lifecycle of the session, the integration of external systems will be smooth and efficient.
In this post, we explored critical aspects of session management, provided code examples that highlight best practices, and shared troubleshooting strategies. Now, you are better equipped to tackle common session issues in Spring Integration. Happy coding!
Checkout our other articles