Mastering Annotations: Common Pitfalls in Java Development
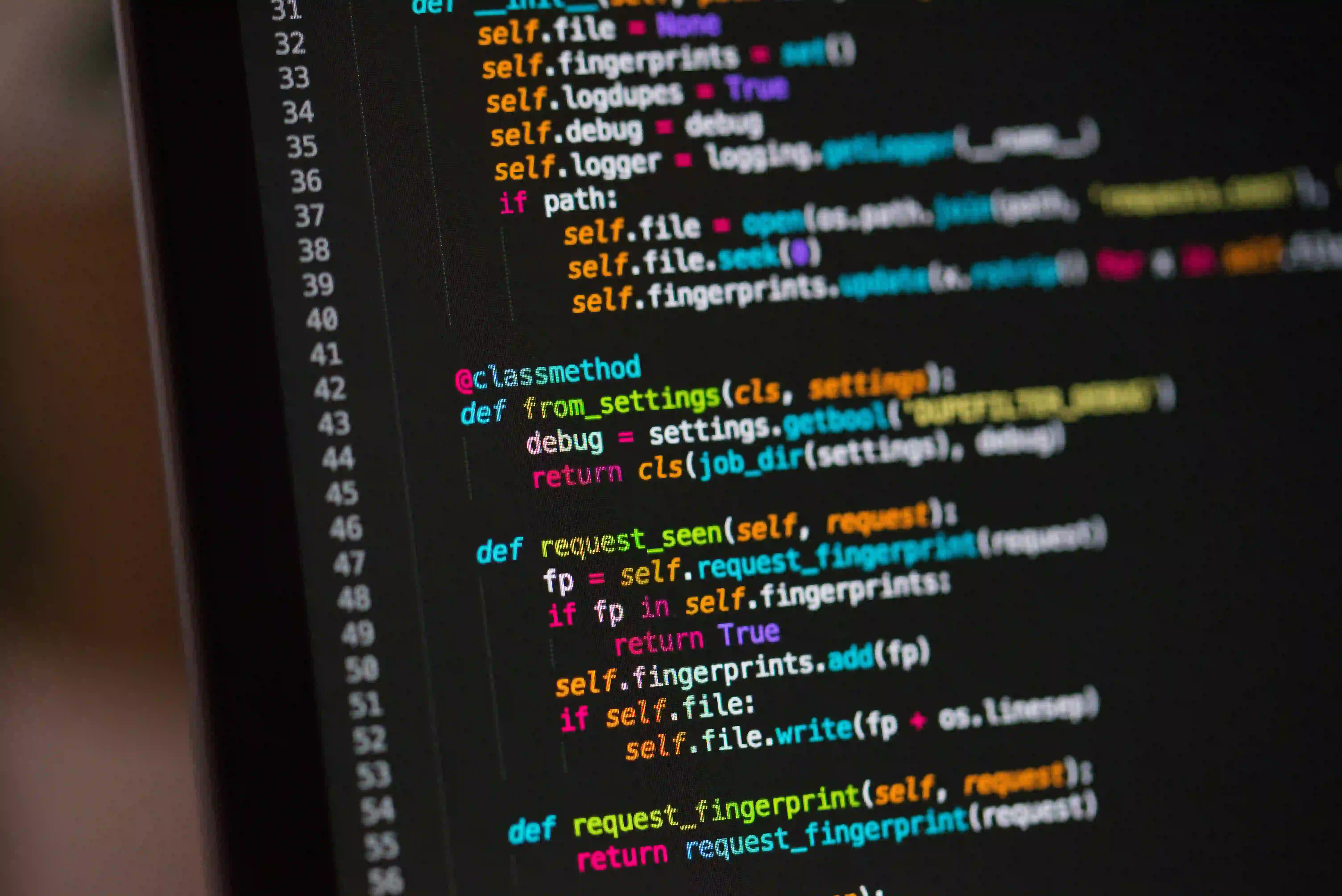
Mastering Annotations: Common Pitfalls in Java Development
Java annotations are a powerful feature that allows developers to add metadata to their code. They can provide information to the compiler, influence program behavior at runtime, or even serve as documentation. Despite their usefulness, many developers encounter pitfalls when working with annotations. This blog post will walk you through common challenges and best practices to help you master Java annotations.
Understanding Java Annotations
Before diving into pitfalls, let’s clarify what Java annotations are. Annotations are essentially markers in your code, defined using the @interface
keyword. They are used to provide information about the code that can then be processed by the compiler or runtime.
Here’s a simple annotation definition:
public @interface MyCustomAnnotation {
String value();
int number() default 1;
}
Usage Example
To use this annotation, you might apply it to a method:
@MyCustomAnnotation(value = "Test Method", number = 5)
public void testMethod() {
// Method implementation
}
This annotation serves as a metadata descriptor for the testMethod
. Now, let’s explore the common pitfalls developers face with annotations.
Common Pitfalls in Java Annotations
1. Not Retaining Annotations at Runtime
Issue: By default, Java annotations can only be detected at compile time. If you want to access annotations at runtime, you must specify the retention policy.
Solution: Use @Retention
to specify that an annotation should be retained in the runtime.
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
public @interface MyRuntimeAnnotation {
String description();
}
This ensures that the annotation will be available for reflection at runtime.
2. Overusing Annotations
Issue: Annotations can make your code cleaner, but overusing them can lead to clutter and confusion. Annotations should serve a meaningful purpose, not be used as mere labels.
Solution: Apply annotations judiciously. Ask yourself if an annotation truly provides value or if it complicates readability.
3. Ignoring Default Values
Issue: Failing to provide default values for annotation elements may lead to boilerplate code.
Solution: Always define sensible default values.
public @interface Config {
String filePath() default "/default/path";
}
By providing a default value (/default/path
), you allow the annotation to be more flexible and reduce the need for explicit definitions when not necessary.
4. Confusing Annotations with Interfaces
Issue: Some developers mistakenly treat annotations as interfaces. Unlike interfaces, annotations do not support method implementations.
Solution: Remember that annotations can only contain method signatures (elements) and cannot have method bodies.
Example of incorrect usage:
public @interface NotAnInterface {
void myMethod(); // This will cause a compilation error
}
5. Complex Nested Annotations
Issue: Nesting multiple annotations can make the code less readable and harder to maintain.
Solution: Keep nested annotations to a minimum. If you find your annotations are becoming complex, consider refactoring into simpler, more manageable constructs.
6. Failing to Document Annotations
Issue: Annotations that lack documentation can be unclear to anyone who uses them.
Solution: Document each annotation clearly. Explain what the annotation does, why it exists, and provide usage examples.
/**
* This annotation is used to mark methods that require a transaction.
*/
@Retention(RetentionPolicy.RUNTIME)
public @interface Transactional {
String value() default "default";
}
7. Using Annotations Without Processing
Issue: Applying annotations without a processing mechanism can lead to unused metadata.
Solution: Always use an annotation processor (like APT
or javax.annotation.processing
) to ensure that the annotations have a purpose.
Here’s a simple demonstration of a processor:
import javax.annotation.processing.*;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
@SupportedAnnotationTypes("MyCustomAnnotation")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class MyAnnotationProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element element : roundEnv.getElementsAnnotatedWith(MyCustomAnnotation.class)) {
// Processing logic
System.out.println("Processing: " + element.getSimpleName());
}
return true;
}
}
8. Not Considering Inheritance
Issue: Annotations may behave differently when applied to classes and their subclasses.
Solution: Understand that annotations can be inherited using the @Inherited
annotation.
import java.lang.annotation.Inherited;
@Inherited
@Retention(RetentionPolicy.RUNTIME)
public @interface BaseAnnotation {
}
Subsequently, any class extending a class annotated with @BaseAnnotation
will also inherit this annotation.
9. Ignoring Performance Implications
Issue: Using a large number of annotations can have performance implications, especially if they are processed at runtime.
Solution: Be mindful of performance and only utilize necessary annotations. Evaluate your application’s performance if you're using annotations heavily.
Closing Remarks
Java annotations are a multifaceted tool that, when used correctly, can enhance your code's clarity and functionality. By avoiding the pitfalls discussed in this article, you can maximize the benefits of annotations in your Java development. Always prioritize readability, maintainability, and performance when working with annotations.
For further reading on Java annotations, you may find the following resources valuable:
- Java Documentation on Annotations
- The Complete Guide to Java Annotations
Engage and Learn More
If you’ve encountered any other pitfalls or have best practices you would like to share, feel free to leave a comment below. Happy coding!