Resolving Common Errors When Parsing JSON with Moshi
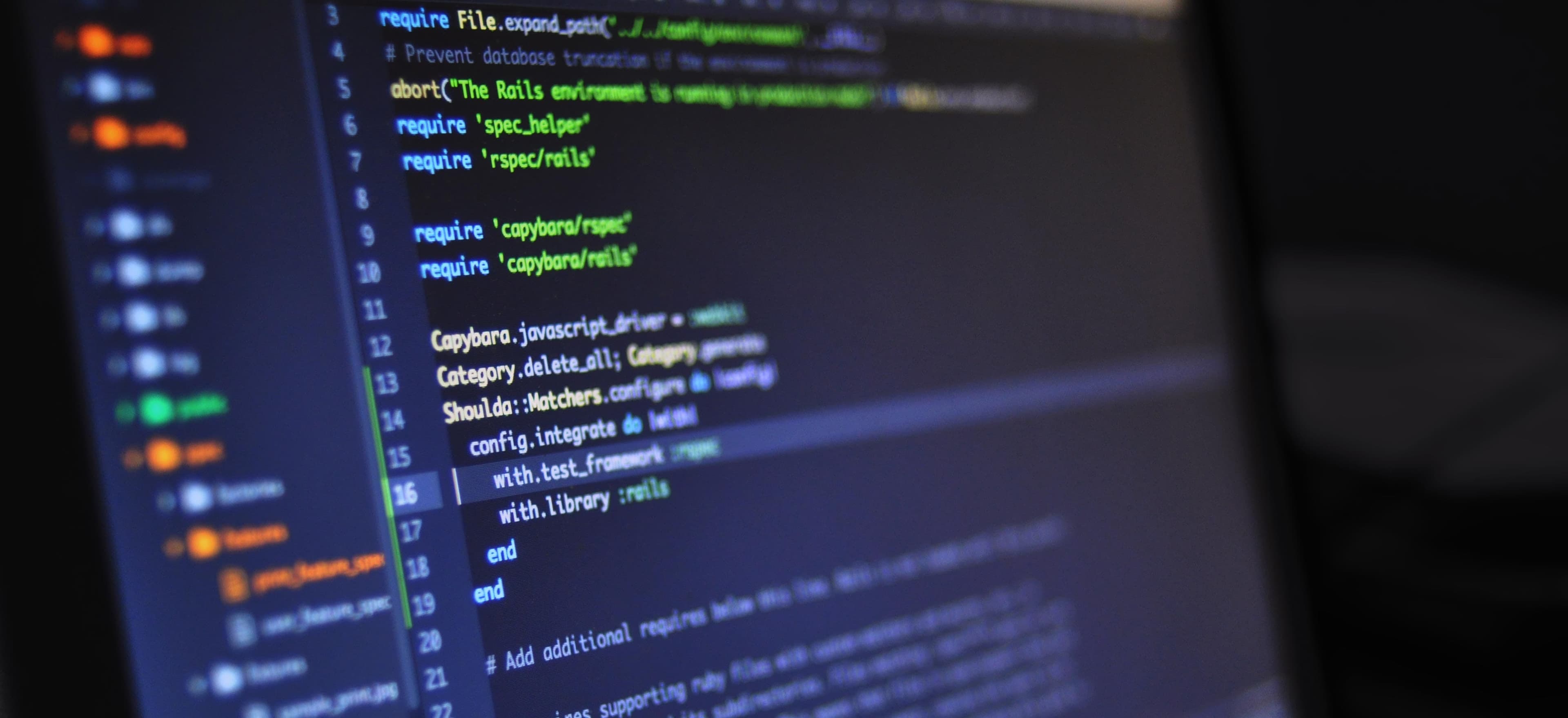
- Published on
Resolving Common Errors When Parsing JSON with Moshi
When it comes to working with JSON in Android development, Moshi is one of the most popular libraries for converting JSON into Java objects. Its simplicity and efficiency make it a perfect choice for handling JSON data in your applications. However, developers often encounter errors while parsing JSON, which can be frustrating and time-consuming to resolve. In this blog post, we will explore some common errors associated with JSON parsing using Moshi and how to effectively address them.
Table of Contents
- What is Moshi?
- Setting Up Moshi
- Common JSON Parsing Errors
- 3.1. No adapter for this type
- 3.2. Unexpected JSON token
- 3.3. Nested data parsing issues
- Best Practices for Using Moshi
- Conclusion
What is Moshi?
Moshi is a modern JSON library for Android and Java, developed by Square. It is user-friendly, lightweight, and powerful, making it an excellent choice for parsing complex JSON data structures. Moshi’s ability to generate type-safe data models and handle Kotlin coroutines effectively is a significant advantage for developers.
For more details about Moshi, you can check its official documentation.
Setting Up Moshi
To start using Moshi in your project, first, add Maven dependencies to your build.gradle
file:
dependencies {
implementation "com.squareup.moshi:moshi:1.13.0"
implementation "com.squareup.moshi:moshi-kotlin:1.13.0"
implementation "com.squareup.retrofit2:converter-moshi:2.9.0"
}
This will include Moshi along with its Kotlin adapter and Retrofit support. Now let's look at how we can parse JSON data with Moshi.
Example: Basic Parsing with Moshi
import com.squareup.moshi.Moshi;
import com.squareup.moshi.JsonAdapter;
import com.squareup.moshi.Types;
public class User {
public String name;
public int age;
}
Moshi moshi = new Moshi.Builder().build();
JsonAdapter<User> jsonAdapter = moshi.adapter(User.class);
String json = "{\"name\":\"John\", \"age\":30}";
User user = jsonAdapter.fromJson(json);
System.out.println("Name: " + user.name + ", Age: " + user.age);
This code snippet demonstrates how to define a basic User
class and parse the corresponding JSON string into a User
object.
Common JSON Parsing Errors
Even with Moshi’s efficiency, you may run into the following errors during JSON parsing:
3.1. No adapter for this type
This error occurs when Moshi doesn’t have a registered adapter to handle the type you are trying to parse.
Resolution:
Ensure you have registered the appropriate Moshi adapters for your custom classes. If you are parsing a list or a more complex object, Moshi needs to know how to handle it.
Type userType = Types.newParameterizedType(List.class, User.class);
JsonAdapter<List<User>> adapter = moshi.adapter(userType);
3.2. Unexpected JSON token
Moshi throws an IOException
when it encounters a JSON token that does not match the expected format of your class. This often happens when the JSON structure does not align with your class attributes.
Resolution:
Verify the structure of your JSON against your class definition. Also, consider using optional fields or default values in your class to handle missing data gracefully.
public class User {
public String name;
public int age = 25; // Default value
}
3.3. Nested data parsing issues
If your JSON contains nested objects, you may end up with parsing errors if those nested objects do not have their corresponding classes set up.
Resolution:
Define data classes for nested structures and ensure proper annotations if necessary. For instance, suppose your JSON looks like this:
{
"user": {
"name": "John",
"age": 30
},
"isActive": true
}
You would need to create another class to handle the user object:
public class Response {
public User user;
public boolean isActive;
}
Now you can parse it as follows:
JsonAdapter<Response> responseAdapter = moshi.adapter(Response.class);
String responseJson = "{\"user\":{\"name\":\"John\", \"age\":30}, \"isActive\":true}";
Response response = responseAdapter.fromJson(responseJson);
Best Practices for Using Moshi
To enhance your experience with Moshi and avoid common errors, here are some recommended best practices:
-
Keep JSON Structures Simple: If you can simplify your JSON structure, it will make parsing much easier and less error-prone.
-
Utilize Data Classes: Use data classes whenever possible, especially when using Kotlin, for simple boilerplate-free class definitions.
-
Read the Moshi Documentation: Familiarize yourself with Moshi’s documentation for best practices, usage examples, and advanced features.
-
Extensive Testing: Create comprehensive unit tests to cover various scenarios, especially edge cases regarding data parsing.
-
Error Handling: Implement proper error handling when parsing JSON to preemptively catch and manage potential issues.
The Last Word
Working with JSON can sometimes be challenging, especially when parsing complex data structures. However, with Moshi's simplicity and power, you can effectively manage your JSON needs in Android development. By understanding common errors and following best practices, you can improve the reliability and maintainability of your code.
Remember that debugging and error resolution are part of the coding experience. By implementing the solutions discussed in this blog post, you can minimize parsing errors and enjoy a smoother development workflow.
For further reading, consider exploring other JSON parsing libraries like Gson and Jackson, as they provide different approaches and features that might suit your project needs.
Happy coding!
Checkout our other articles