Troubleshooting AMX Boot Issues in Glassfish 3 with Groovy
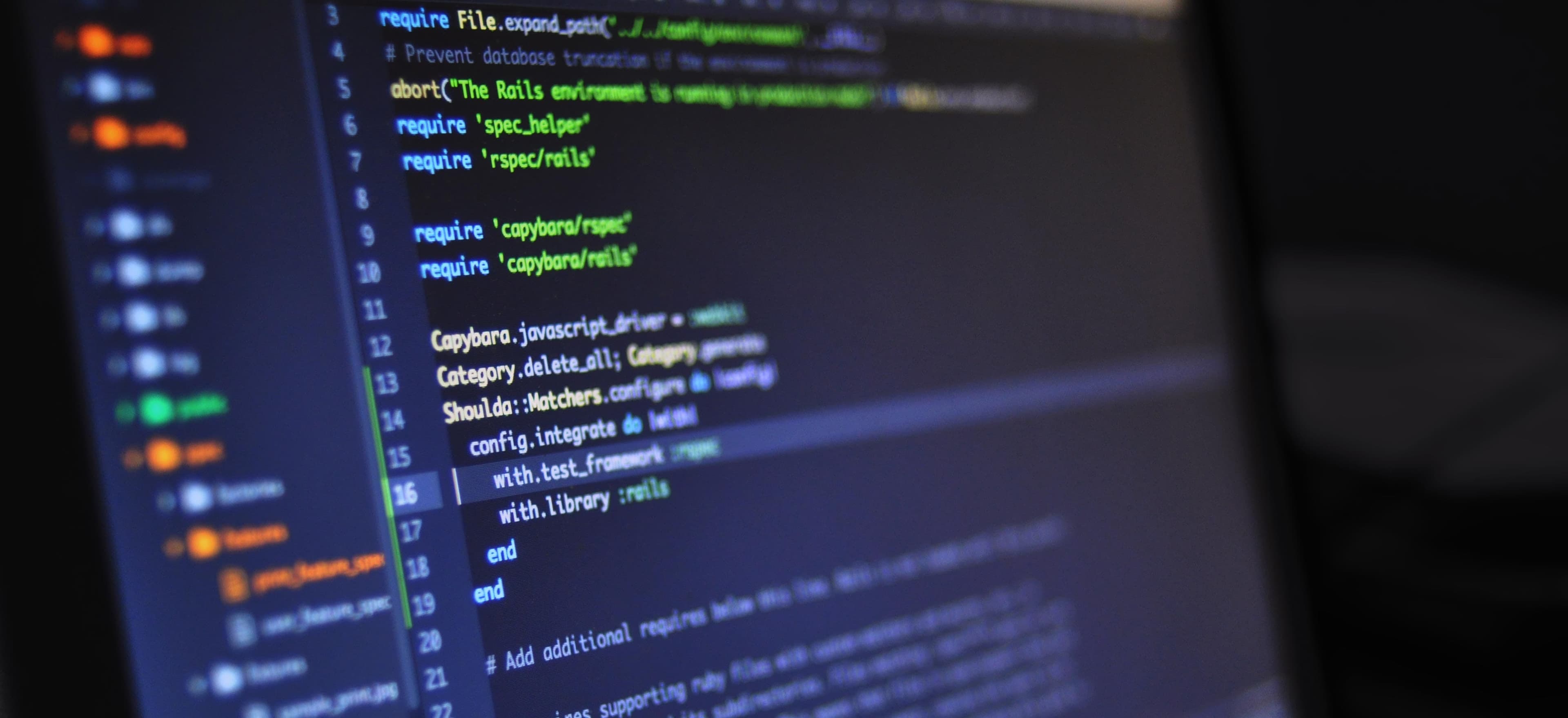
- Published on
Troubleshooting AMX Boot Issues in Glassfish 3 with Groovy
Setting the Stage
GlassFish is a powerful open-source application server that supports multiple Java EE technologies, providing a robust environment for enterprise applications. However, developers sometimes encounter challenges, especially when working with the AMX (Admin Mun infrastructure eXtension) features in GlassFish 3. One such challenge is boot issues that can arise due to various configuration and environmental factors. In this blog post, we will explore common AMX boot issues and how to troubleshoot them using Groovy, a dynamic language for the Java platform.
Understanding AMX and GlassFish
Before diving into troubleshooting, it's crucial to understand what AMX is and how it integrates with GlassFish. The AMX is a JMX implementation (Java Management Extensions) that enhances management capabilities in GlassFish, enabling developers to interact programmatically with different components of the application server.
For more information on GlassFish and AMX architecture, visit Oracle.
Common AMX Boot Issues
- Configuration Errors: Misconfiguration within the GlassFish domain or application can lead to boot issues.
- Classpath Issues: Missing or incorrect classes in the classpath can disrupt the AMX initialization process.
- Network Connectivity: In cases where GlassFish needs to access resources over the network, connectivity issues can lead to AMX failing to boot properly.
- Resource Constraints: Low memory or CPU resources can hinder AMX's ability to function.
- Plugin Mismatches: Incompatibilities between various GlassFish plugins and libraries may also cause AMX boot problems.
Utilizing Groovy for Troubleshooting
Groovy, with its concise syntax and dynamic nature, can be instrumental in troubleshooting AMX boot issues. Here are some examples of how you can leverage Groovy scripts to identify and potentially resolve these issues.
Checking GlassFish Status
Before digging deeper, it’s essential to know the status of the GlassFish server. Using Groovy, you can quickly check whether the server is running and if AMX is available.
// Import necessary classes
import org.glassfish.api.ActionReport
import org.glassfish.api.server.ServerEnvironment
import com.sun.enterprise.util.SystemPropertyConstants
// Function to check GlassFish status
def checkServerStatus() {
def server = ServerEnvironment.instance
def running = server.isRunning()
if (running) {
println "GlassFish server is running."
} else {
println "GlassFish server is not running."
}
}
checkServerStatus()
Analyzing Log Files
Log files provide vital information regarding the server's internal operations. Often, the logs contain error messages that can point you right to the issue at hand. A Groovy script can read through these logs for critical error keywords.
def logFilePath = "/path/to/glassfish/domains/domain1/logs/server.log"
def errorKeywords = ["AMX", "error", "fatal"]
def checkLogs() {
new File(logFilePath).eachLine { line ->
if (errorKeywords.any { line.contains(it) }) {
println line
}
}
}
checkLogs()
Verifying Classpath
Classpath issues are a common viewpoint when dealing with startup problems. Here is a Groovy script that lists all classpath elements, making it easier to determine for irregularities.
def printClasspath() {
def classpath = System.getProperty("java.class.path")
classpath.split(":").each { entry ->
println entry
}
}
printClasspath()
Troubleshooting Resource Constraints
If you suspect resource issues, it's helpful to assess memory and CPU usage. Below is an example of how to observe system memory status using Groovy.
def checkMemory() {
def runtime = Runtime.getRuntime()
long totalMemory = runtime.totalMemory()
long freeMemory = runtime.freeMemory()
println "Total Memory: ${totalMemory / (1024 * 1024)} MB"
println "Free Memory: ${freeMemory / (1024 * 1024)} MB"
}
checkMemory()
Resolving Issues
With common issues identified and potential fixes brainstormed through Groovy scripts, let’s discuss some approaches to resolving these boot issues.
Configuration Fixes
- Ensure that your
domain.xml
file is properly configured. Improper settings here often lead to boot issues. - Use the GlassFish Admin Console to validate configurations visually.
Classpath Correctness
- Review your Java classpath and make sure all necessary libraries and plugins are included.
- Consider checking replacement libraries that may cause conflicting versions.
Network Checks
- Perform simple ping tests to ensure all required services are reachable.
- Check firewall settings that might block necessary communication.
Enhancing Resources
- If memory constraints are present, consider increasing the JVM heap size.
- Scale up your server resources if required, particularly in high-load environments.
Closing the Chapter
Troubleshooting AMX boot issues in GlassFish 3 can be an arduous task, but employing Groovy scripts for diagnostics can streamline the process. This blog post aimed to guide you through identifying common pitfalls, using Groovy for checking server status, analyzing logs, verifying classpath, and assessing resource availability.
For continuous improvement in your troubleshooting practices, always refer to the official GlassFish documentation for the latest updates. Understanding the AMX boot processes will improve not only your problem-solving skills but will also enhance the performance of enterprise applications in which you work.
Implement these strategies, and you will reduce the frustrations associated with boot issues to pave the way for a smooth approach to deploying applications on GlassFish. Happy coding!