Mastering Spring 4 Conditional Annotations Made Easy
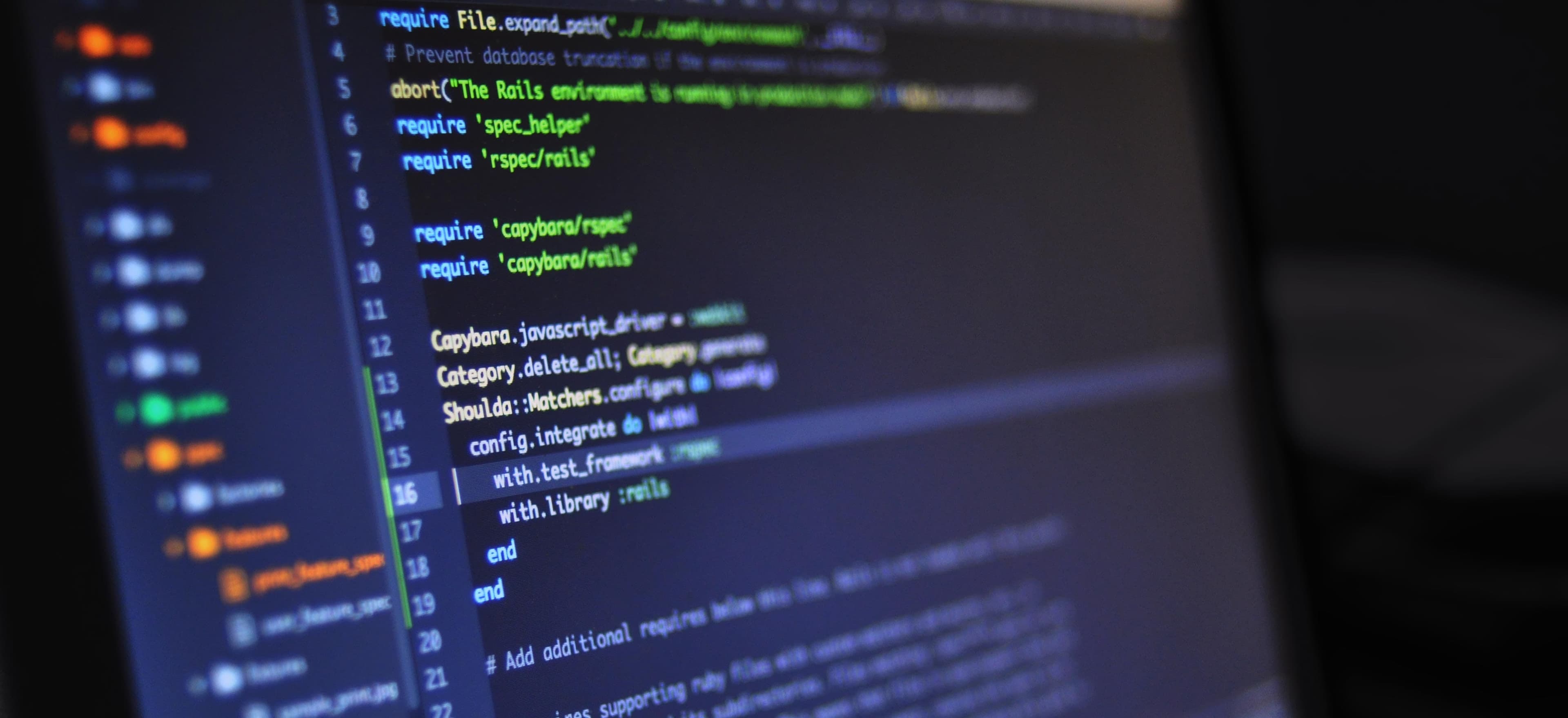
- Published on
Mastering Spring 4 Conditional Annotations Made Easy
Spring 4 introduced a feature that revolutionized the way developers configure their applications: Conditional Annotations. This powerful mechanism allows beans to be created based on certain conditions, which enhances flexibility and minimizes unnecessary configuration in Java applications. In this blog post, we'll explore the various types of conditional annotations, their practical applications, and code snippets that illustrate their usage.
Understanding Conditional Annotations
Conditional annotations are a suite of annotations that can modify the behavior of your Spring application configuration based on specific conditions. The primary annotations introduced in Spring 4 include:
- @Conditional
- @ConditionalOnProperty
- @ConditionalOnClass
- @ConditionalOnBean
- @ConditionalOnMissingBean
These annotations allow you to define conditions under which a specific bean will be registered within the Spring Application Context. Let's dive deeper into each one.
1. @Conditional
The @Conditional
annotation is a foundational annotation that allows you to create custom conditions to determine whether a bean should be registered. You can implement the Condition
interface and define your logic within it.
Code Example
import org.springframework.context.annotation.Conditional;
import org.springframework.context.annotation.Configuration;
@Configuration
@Conditional(CustomCondition.class)
public class CustomConditionConfig {
// Define your bean here
}
Why This Matters: By implementing CustomCondition
, you gain complete control over when and why your configurations are activated. Here's a simple implementation:
import org.springframework.context.annotation.Condition;
import org.springframework.context.annotation.ConditionContext;
import org.springframework.core.type.AnnotatedTypeMetadata;
public class CustomCondition implements Condition {
@Override
public boolean matches(ConditionContext context, AnnotatedTypeMetadata metadata) {
String property = context.getEnvironment().getProperty("my.custom.property");
return "true".equalsIgnoreCase(property);
}
}
In this example, if "my.custom.property"
is set to "true"
, the CustomConditionConfig
will be instantiated.
2. @ConditionalOnProperty
The @ConditionalOnProperty
annotation is a convenient way to conditionally load beans based on the presence or value of a specific property in your application's configuration.
Code Example
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
@ConditionalOnProperty(name = "feature.enabled", havingValue = "true")
public MyFeature myFeatureBean() {
return new MyFeature();
}
}
Why This Matters: This feature allows you to toggle features based on configuration. This is particularly useful for enabling or disabling features in production versus development environments.
3. @ConditionalOnClass
The @ConditionalOnClass
annotation can be used to check for the presence of a specific class on the classpath. This is useful if your bean is only relevant in certain environments, such as when specific libraries are in use.
Code Example
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
@ConditionalOnClass(name = "com.example.ExternalLibrary")
public class ExternalLibraryConfig {
@Bean
public ExternalLibraryBean externalLibraryBean() {
return new ExternalLibraryBean();
}
}
Why This Matters: This helps avoid ClassNotFoundException
at runtime in environments where the expected library isn't present.
4. @ConditionalOnBean
With @ConditionalOnBean
, you can specify that your bean should only be created if a specific bean, or beans, are already present in the application context.
Code Example
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class ParentConfig {
@Bean
public ParentBean parentBean() {
return new ParentBean();
}
}
@Configuration
@ConditionalOnBean(ParentBean.class)
public class ChildConfig {
@Bean
public ChildBean childBean(ParentBean parentBean) {
return new ChildBean(parentBean);
}
}
Why This Matters: By using @ConditionalOnBean
, you can ensure that ChildBean
is only created if ParentBean
exists. This creates a tighter coupling between related beans.
5. @ConditionalOnMissingBean
Conversely, @ConditionalOnMissingBean
allows you to define a bean that will only be registered if a certain bean is missing from the application context.
Code Example
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfig {
@Bean
@ConditionalOnMissingBean
public DefaultService defaultService() {
return new DefaultService();
}
}
Why This Matters: This annotation is useful for setting up default implementations, ensuring that your application can operate as expected with minimal configuration.
Practical Use Cases
Conditional annotations come in handy in various scenarios, such as:
- Feature Flags: You can easily toggle features for A/B testing by managing feature flags through configuration.
- Environment-Specific Configurations: Conditional annotations allow having different beans based on the active profile.
- Optional Dependencies: Load beans based on the presence of optional libraries, thus keeping your application lightweight.
Key Takeaways
Mastering Spring 4 Conditional Annotations can significantly streamline your application's configuration and improve its maintainability. By utilizing annotations like @Conditional
, @ConditionalOnProperty
, and others, you can ensure that your Spring beans are registered only when certain conditions are met, ultimately leading to a cleaner and more efficient application.
For more detailed documentation, check the official Spring documentation on Conditional Annotations.
By employing these powerful conditional mechanisms, you can build responsive Java applications that adapt to their environments effortlessly. Happy coding!