Mastering Picocli: Overcoming Common Command Line Pitfalls
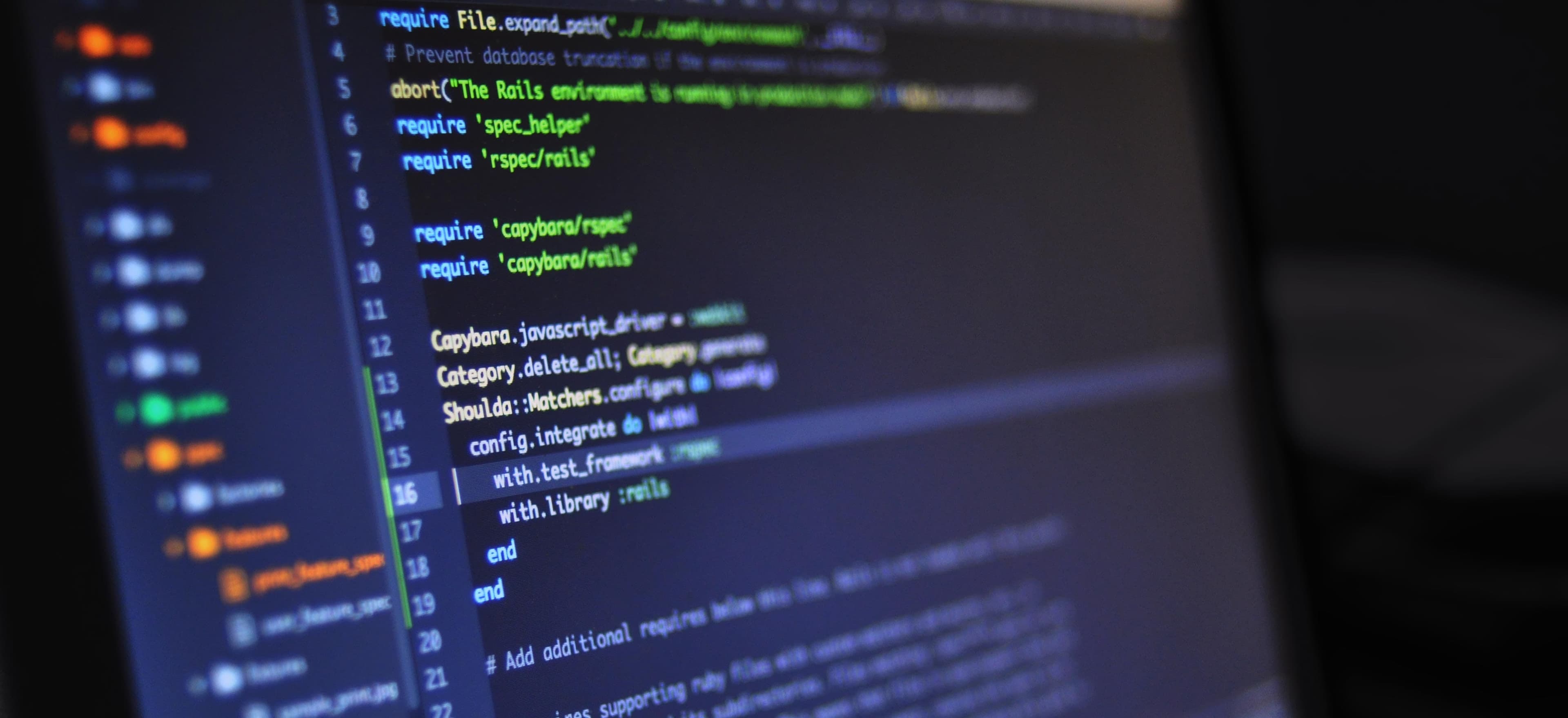
- Published on
Mastering Picocli: Overcoming Common Command Line Pitfalls
Command line interfaces (CLIs) are essential for many applications. They provide powerful tools for users to interact with software directly. However, creating a robust CLI can be challenging, especially when accounting for user input and command structure. Picocli is a popular Java library that simplifies the process of building command line applications. This article will guide you through common pitfalls in CLI development and show how you can use Picocli to create a powerful command line utility.
What is Picocli?
Picocli is a feature-rich library designed for building command line applications in Java. It allows you to define command-line options, parameters, and subcommands with minimal boilerplate code. Some key features include:
- Automatic help generation: Picocli automatically generates usage information.
- Support for nested commands: You can create a sophisticated command structure.
- Type conversion: Picocli supports various data types and automatically converts command line arguments.
- Custom validation: It allows you to enforce constraints on user inputs.
With these features in mind, let’s delve into some common command line pitfalls and see how to overcome them using Picocli.
Common Command Line Pitfalls
1. Poor Error Handling
CLI applications often fail silently or provide unhelpful error messages. Users can become frustrated when they encounter issues that do not clearly describe the problem or suggest possible solutions.
Solution: Use Picocli’s built-in error handling mechanisms to provide clear feedback.
Example Code:
import picocli.CommandLine;
import picocli.CommandLine.Command;
import picocli.CommandLine.Option;
@Command(name = "example", mixinStandardHelpOptions = true, version = "1.0",
description = "An example command")
public class ExampleCommand implements Runnable {
@Option(names = {"-n", "--name"}, required = true,
description = "The name of the user")
private String name;
public void run() {
try {
validateName(name);
System.out.println("Hello, " + name + "!");
} catch (IllegalArgumentException e) {
System.err.println(e.getMessage());
CommandLine.usage(this, System.err);
}
}
private void validateName(String name) {
if (name.length() < 3) {
throw new IllegalArgumentException("Name must be at least 3 characters.");
}
}
public static void main(String[] args) {
CommandLine.run(new ExampleCommand(), args);
}
}
In the above code, we ensure that the user provides a valid name. If not, an exception is thrown, and a clear message is displayed along with the command's usage information.
2. Neglecting User Experience
When developing a command line application, it is crucial to prioritize user experience. This means designing a CLI that is intuitive and provides users with the information they need.
Solution: Use Picocli’s annotations to provide helpful descriptions and examples.
Example Code:
@Command(name = "file-manager", mixinStandardHelpOptions = true,
version = "file-manager 1.0",
description = "A command-line file manager")
public class FileManager implements Runnable {
@Option(names = {"-s", "--source"}, required = true,
description = "Source file to operate on")
private String source;
@Option(names = {"-d", "--destination"}, description = "Destination for the file")
private String destination;
public void run() {
System.out.println("Managing file from: " + source +
(destination != null ? " to: " + destination : ""));
}
public static void main(String[] args) {
CommandLine.run(new FileManager(), args);
}
}
In this example, the command has well-defined options, and when the user invokes --help
, they receive complete and informative descriptions.
3. Inconsistent Command Parsing
Users often expect commands to behave consistently. If similar commands are treated differently, it can lead to confusion.
Solution: Use Picocli’s support for subcommands to maintain a consistent command structure.
Example Code:
@Command(name = "cli-app", subcommands = { UploadCommand.class, DownloadCommand.class })
public class CLIApp implements Runnable {
public void run() {
System.out.println("Use --help for available commands.");
}
public static void main(String[] args) {
CommandLine.run(new CLIApp(), args);
}
}
@Command(name = "upload", description = "Upload a file")
class UploadCommand implements Runnable {
@Option(names = {"-f", "--file"}, required = true, description = "File to upload")
String file;
@Override
public void run() {
System.out.println("Uploading " + file);
}
}
@Command(name = "download", description = "Download a file")
class DownloadCommand implements Runnable {
@Option(names = {"-f", "--file"}, required = true, description = "File to download")
String file;
@Override
public void run() {
System.out.println("Downloading " + file);
}
}
This example demonstrates how to structure commands using subcommands. Users can intuitively access both upload and download functionalities, maintaining a consistent experience.
4. Failing to Enforce Constraints
Invalid data input can lead to unpredictable behavior. Without constraints, users might input incorrect parameters, causing your application to crash.
Solution: Use Picocli to validate input before execution.
Example Code:
@Command(name = "calculate", description = "Perform mathematical operations")
public class Calculator implements Runnable {
@Option(names = {"-a", "--add"}, split = ",",
description = "Numbers to add (comma-separated)", required = true)
private List<Integer> numbers;
public void run() {
int sum = numbers.stream().mapToInt(Integer::intValue).sum();
System.out.println("Sum: " + sum);
}
public static void main(String[] args) {
CommandLine.run(new Calculator(), args);
}
}
Here, we require a list of numbers separated by commas. Picocli handles parsing, and users can only input valid integers.
5. Complex Code Structure
Complex command line applications can quickly become difficult to maintain. Keeping logic and commands well-organized is essential.
Solution: Leverage classes and modular code design.
Example Code:
@Command(name = "main-app", subcommands = { UserCommand.class, AdminCommand.class })
public class MainApp implements Runnable {
public void run() {
System.out.println("Welcome to MainApp! Use --help for options.");
}
public static void main(String[] args) {
CommandLine.run(new MainApp(), args);
}
}
By breaking down complex functionality into separate classes (e.g., UserCommand and AdminCommand), you achieve a more maintainable codebase.
Key Takeaways
Mastering Picocli can significantly enhance your command line application's usability, flexibility, and maintainability. By addressing common pitfalls such as poor error handling, neglecting user experience, inconsistent command parsing, failure to enforce constraints, and complex code structure, you ensure a more robust CLIs.
As you dive deeper into using Picocli, consider exploring its official documentation for more advanced features and optimizations. Writing a well-structured and user-friendly command line utility will not only improve the end-user experience but also make your software more resilient to user errors.
Whether you are building a small utility or a complex system, Picocli provides all the tools you need to overcome common command line pitfalls effectively. Get started today and watch your CLI application flourish!