Overcoming Common Play Framework Pitfalls
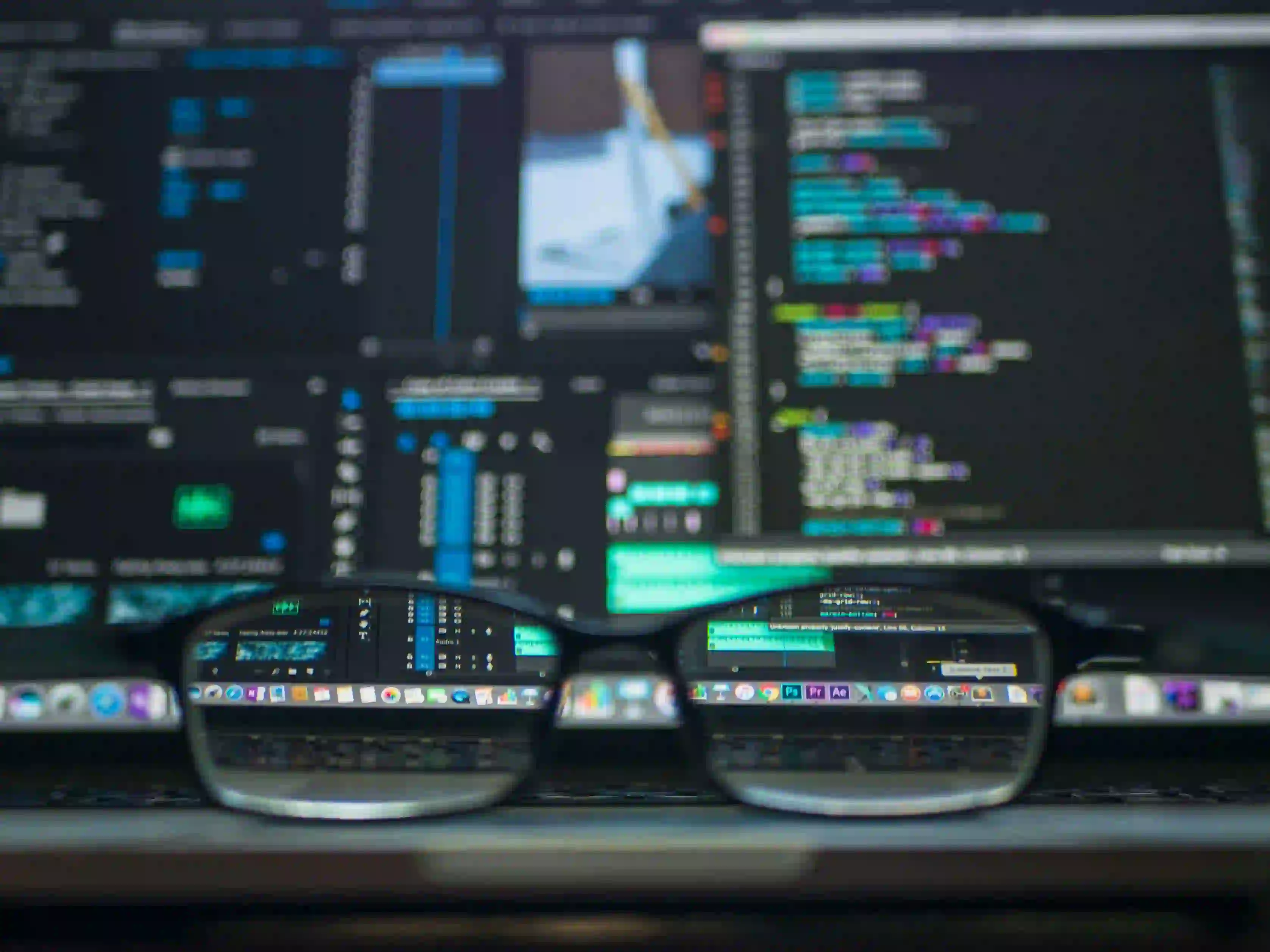
Overcoming Common Play Framework Pitfalls
The Play Framework is a powerful tool for building web applications in Java and Scala. Known for its ease of use and responsiveness, it allows developers to focus on application development instead of server configuration. However, like any framework, Play has its own set of commonly encountered pitfalls. In this blog post, we'll explore these challenges and provide strategies to overcome them.
The Importance of Understanding the Play Framework
Before diving into the pitfalls, it’s essential to grasp why the Play Framework is a go-to choice for many developers. Its reactive architecture allows for efficient handling of concurrent users, which is critical in modern web applications. Moreover, it employs a concise syntax and promotes the use of design patterns like MVC (Model-View-Controller), making it easier to maintain.
That said, understanding the Play Framework's foundations is crucial to circumvent pitfalls effectively. Let's discuss these pitfalls and how we can navigate them.
1. Managing Dependencies Properly
Pitfall
One common issue developers face is mismanaging dependencies, particularly when it comes to libraries and modules. Using outdated libraries can lead to compatibility issues, security vulnerabilities, and more.
Solution
Always keep your dependencies updated. Play Framework supports the use of a build tool called SBT (Simple Build Tool), which allows you to manage and specify your project's dependencies effectively.
Here's how you can do this in build.sbt
:
libraryDependencies += "com.typesafe.play" %% "play" % "2.8.8"
This line specifies which version of Play to use. When newer versions are released, update this line to the latest.
Why
Keeping dependencies current avoids issues related to deprecated methods, ensures compatibility with the latest features, and strengthens security measures by using libraries with fewer vulnerabilities.
For more details on managing dependencies, you can check the Play Framework Documentation.
2. Misconfiguring Routes
Pitfall
Routes define how HTTP requests are handled in a Play application. A common mistake is misconfiguring these routes, leading to unexpected behaviors or application crashes.
Solution
Be meticulous when defining your routes in the conf/routes
file. Ensure each path is unique and correctly associated with the desired action. Here’s an example of a correctly configured simple route:
GET /home controllers.HomeController.index
POST /submit controllers.FormController.submitForm
Why
Misconfiguring routes can lead to confusing errors. By organizing your routes clearly, you maintain high readability for yourself and your team, leading to faster debugging and code maintenance processes.
3. Failing to Implement Error Handling
Pitfall
Another common oversight is overlooking error handling. Not implementing adequate error handling can lead to a poor user experience and expose sensitive information.
Solution
Play Framework provides excellent mechanisms for handling errors, primarily through its controller base methods. Using the play.api.mvc.Results
class, you can handle exceptions effectively.
Here's a sample controller method demonstrating error handling:
public Result index() {
try {
// Business logic here
return ok("Success!");
} catch (Exception e) {
// Log the exception
Logger.error("Error while processing", e);
return internalServerError("An unexpected error occurred.");
}
}
Why
Implementing error handling enhances application robustness. It ensures appropriate actions are taken when an error occurs, thus safeguarding user experience and maintaining application integrity.
4. Not Utilizing Asynchronous Processing
Pitfall
A common mistake is running blocking operations on the main thread, which can lead to performance bottlenecks. This is particularly problematic in high-load situations.
Solution
Leverage Play's built-in asynchronous capabilities. Use the CompletableFuture
or its reactive counterparts. Here’s an example of how to manage an asynchronous database call:
public CompletionStage<Result> getUserData(String userId) {
return userService.findUserById(userId).thenApply(user -> {
if (user != null) {
return ok(Json.toJson(user));
} else {
return notFound("User not found");
}
});
}
Why
Asynchronous programming enables better resource management, allowing your application to handle multiple requests simultaneously without blocking the main thread.
For more information on asynchronous processing in Play, you can explore Asynchronous Actions in Play Framework.
5. Ignoring Testing
Pitfall
Skipping unit and integration tests can severely impact the reliability of your application. Inadequate testing can lead to unnoticed bugs and complications in the long run.
Solution
Take full advantage of Play Framework’s testing capabilities. The framework allows for easy integration of libraries like JUnit and Mockito, making it straightforward to write tests for your application.
Here's a simple unit test example using JUnit:
import org.junit.Test;
import static org.junit.Assert.*;
public class HomeControllerTest {
@Test
public void testIndex() {
HomeController controller = new HomeController();
Result result = controller.index();
assertEquals("Success!", contentAsString(result));
}
}
Why
Testing ensures stability and security. By implementing tests, you can catch issues early, making your application more reliable and easier to maintain.
A Final Look
The Play Framework is a robust tool for building web applications, but it is not without its challenges. By understanding these common pitfalls and implementing the strategies discussed, you can enhance your development process and create a smoother, more efficient coding experience.
Continuous learning and a proactive approach to problem-solving will keep your applications robust, maintainable, and scalable. For further exploration, you might find Play Framework's official site a great resource.
Understanding and overcoming these pitfalls in the Play Framework involves careful planning, attention to detail, and a commitment to following best practices. With practice, your expertise will grow, leading to more efficient and successful Play applications.