Common JavaFX Game Development Mistakes to Avoid
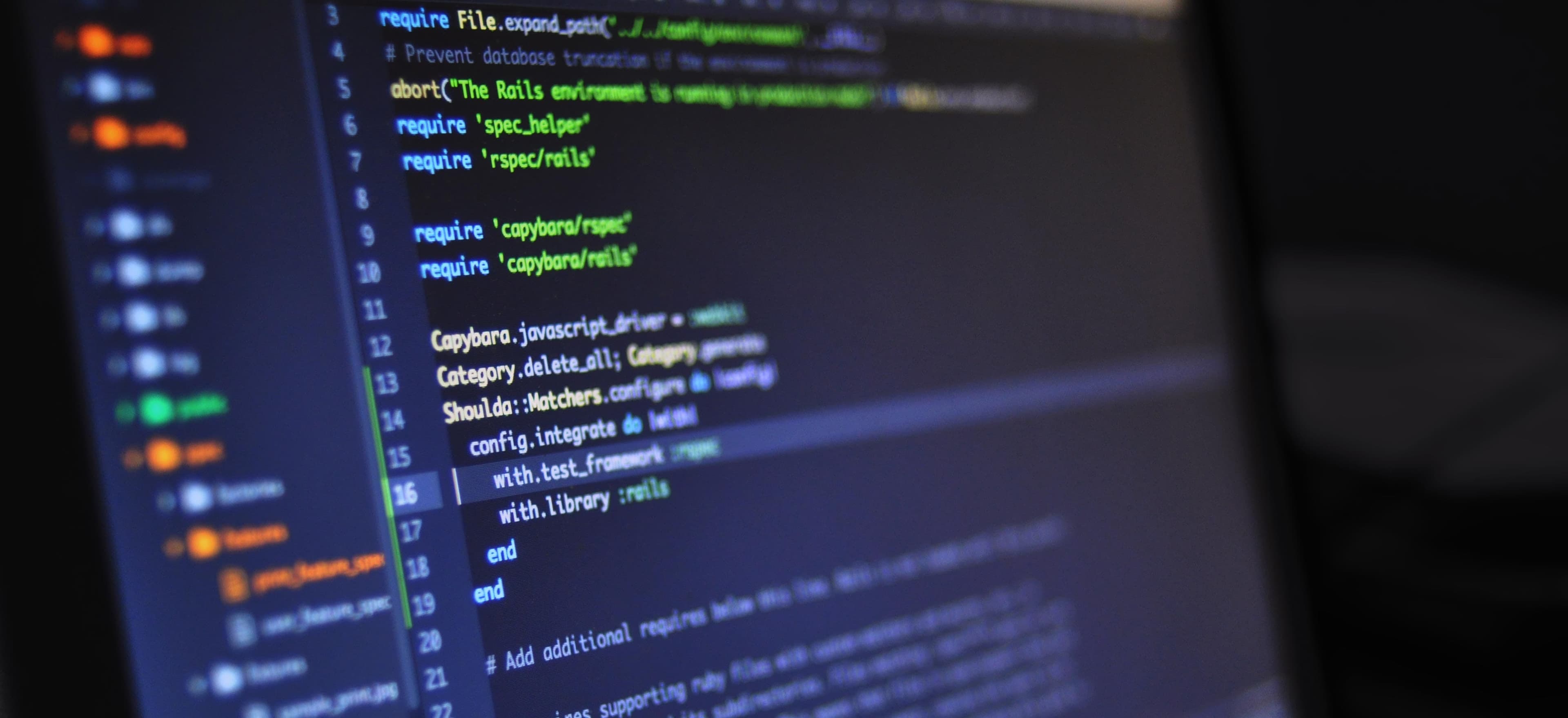
- Published on
Common JavaFX Game Development Mistakes to Avoid
Game development can be a thrilling yet challenging endeavor. JavaFX, recognized for its rich GUI capabilities, provides a robust foundation for game development. However, even seasoned developers can stumble upon hurdles. This blog post will explore common JavaFX game development mistakes and how to avoid them, ensuring a smoother journey in your game development adventure.
1. Ignoring the JavaFX Animation Framework
One of the greatest strengths of JavaFX is its animation framework. However, many developers neglect this feature, opting instead for manual frame updates.
Why This is a Mistake
The manual approach often results in inefficient code, which can cause frame rate drops or stuttering animations. JavaFX’s built-in animation classes help streamline this process and leverage hardware acceleration.
Solution: Use JavaFX Animation Classes
Instead of re-implementing animation logic, use existing classes like Timeline
, AnimationTimer
, and Transition
. Here's a basic example of a bouncing ball:
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class BouncingBall extends Application {
private double dx = 2, dy = 2; // Speed
private Circle ball;
@Override
public void start(Stage primaryStage) {
Pane pane = new Pane();
ball = new Circle(20, Color.BLUE);
ball.setCenterX(50);
ball.setCenterY(50);
pane.getChildren().add(ball);
Scene scene = new Scene(pane, 600, 400);
primaryStage.setScene(scene);
primaryStage.setTitle("Bouncing Ball");
primaryStage.show();
new AnimationTimer() {
public void handle(long currentNanoTime) {
if (ball.getCenterX() >= scene.getWidth() - ball.getRadius() || ball.getCenterX() <= ball.getRadius()) {
dx *= -1; // Reverse horizontal direction
}
if (ball.getCenterY() >= scene.getHeight() - ball.getRadius() || ball.getCenterY() <= ball.getRadius()) {
dy *= -1; // Reverse vertical direction
}
ball.setCenterX(ball.getCenterX() + dx);
ball.setCenterY(ball.getCenterY() + dy);
}
}.start();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary
This code snippet demonstrates the use of AnimationTimer
to update the ball's position on each frame. Notice how we simply reverse the direction of the ball when it hits the bounds. This abstraction allows developers to focus on game logic instead of frame management.
2. Overlooking Event Handling
Many developers underestimate the importance of effective event handling. Improper handling can lead to a sluggish interface and a poor user experience.
Common Pitfall
A frequent mistake is to attach too many event listeners or process heavyweight logic during event handling.
Solution: Optimize Event Handling
Use event handlers sensibly and keep operations lightweight. For instance:
scene.setOnKeyPressed(event -> {
switch (event.getCode()) {
case LEFT:
// Move player left
break;
case RIGHT:
// Move player right
break;
// Add more cases as needed
}
});
Commentary
The example above uses a simple switch-case structure for key events. This is efficient and neatly organizes the logic for handling user inputs. For heavy operations, consider delegating them to a separate method or using JavaFX's concurrency features, like Task
or Service
.
3. Failing to Manage Resources Properly
Resource management is crucial in game development. Developers often neglect the efficient use of images, sounds, and other assets, leading to increased memory usage and potential crashes.
Why This Should Matter
Not managing resources can result in slow performance or excessive memory consumption.
Solution: Use Resource Caching
JavaFX provides options for caching resources. For instance, instead of loading an image every time it's needed:
Image image = new Image("path/to/image.png");
ImageView imageView = new ImageView(image);
Commentary
This code snippet shows how to efficiently load an image from a resource path. By storing the image in a variable, it prevents the overhead of repeated loading, thus optimizing memory usage.
4. Hardcoding Values
Hardcoding values directly into your game logic can lead to inflexibility and difficulties in modifying the game.
Why This is a Problem
Changing game parameters can become tedious with hardcoded values, and experimenting with different gameplay settings becomes impractical.
Solution: Use Configurations
Consider separating your configurations from game logic, potentially loading them from external files. For example, you could use a properties file:
player.speed=5
enemy.speed=3
You can read the configuration in your Java code like this:
Properties properties = new Properties();
properties.load(new FileInputStream("config.properties"));
int playerSpeed = Integer.parseInt(properties.getProperty("player.speed"));
Commentary
Using a properties file allows you to modify game settings without diving into the codebase. This approach promotes flexibility and scalability, especially during the development and testing phases.
5. Neglecting Game Loop
A well-structured game loop is the backbone of any game, yet many developers overlook it in favor of simpler structures.
Risk of Overlooking the Game Loop
Without a properly defined game loop, game state management can become convoluted, leading to glitches and erratic behavior.
Solution: Define a Game Loop
Always implement your game loop effectively. Here's a simple structure:
void gameLoop() {
long lastTime = System.nanoTime();
final double amountOfTicks = 60.0;
double ns = 1000000000 / amountOfTicks;
double delta = 0;
while (running) {
long now = System.nanoTime();
delta += (now - lastTime) / ns;
lastTime = now;
while (delta >= 1) {
update(); // Update game state
delta--;
}
render(); // Render game
}
}
Commentary
The above loop structure allows you to update the game state at a fixed time rate while rendering it as fast as possible. This ensures smoother gameplay while keeping your game logic organized.
6. Forgetting to Optimize Performance
JavaFX is generally efficient, but unoptimized code can lead to severe performance issues, hampering the gameplay experience.
Why Optimization Matters
Slow performance can frustrate players, leading to poor reviews and loss of interest.
Solution: Profile Your Game
Use Java profiling tools like VisualVM to analyze and identify bottlenecks in your game. Start with small changes:
- Use appropriate data structures
- Minimize object creations in loops
- Use
StringBuilder
for concatenations
Pitfalls to Avoid
Avoid excessive use of reflection or unnecessarily complex algorithms that don't fit the context. Always measure performance before and after optimizations to ensure they are effective.
The Closing Argument
Developing a game in JavaFX can be rewarding when approached with care and consideration. By avoiding common pitfalls such as inefficient event handling, poor resource management, or neglecting the game loop, you will pave the way for a smoother development process and a more enjoyable experience for players.
Remember, continuous learning and adapting are key. Every game developed provides invaluable insights that can help hone your craft. For more in-depth JavaFX resources, feel free to check out the official JavaFX documentation and Oracle's JavaFX tutorials.
Happy developing!
Checkout our other articles