Mastering Proximity Alerts: Common Pitfalls and Solutions
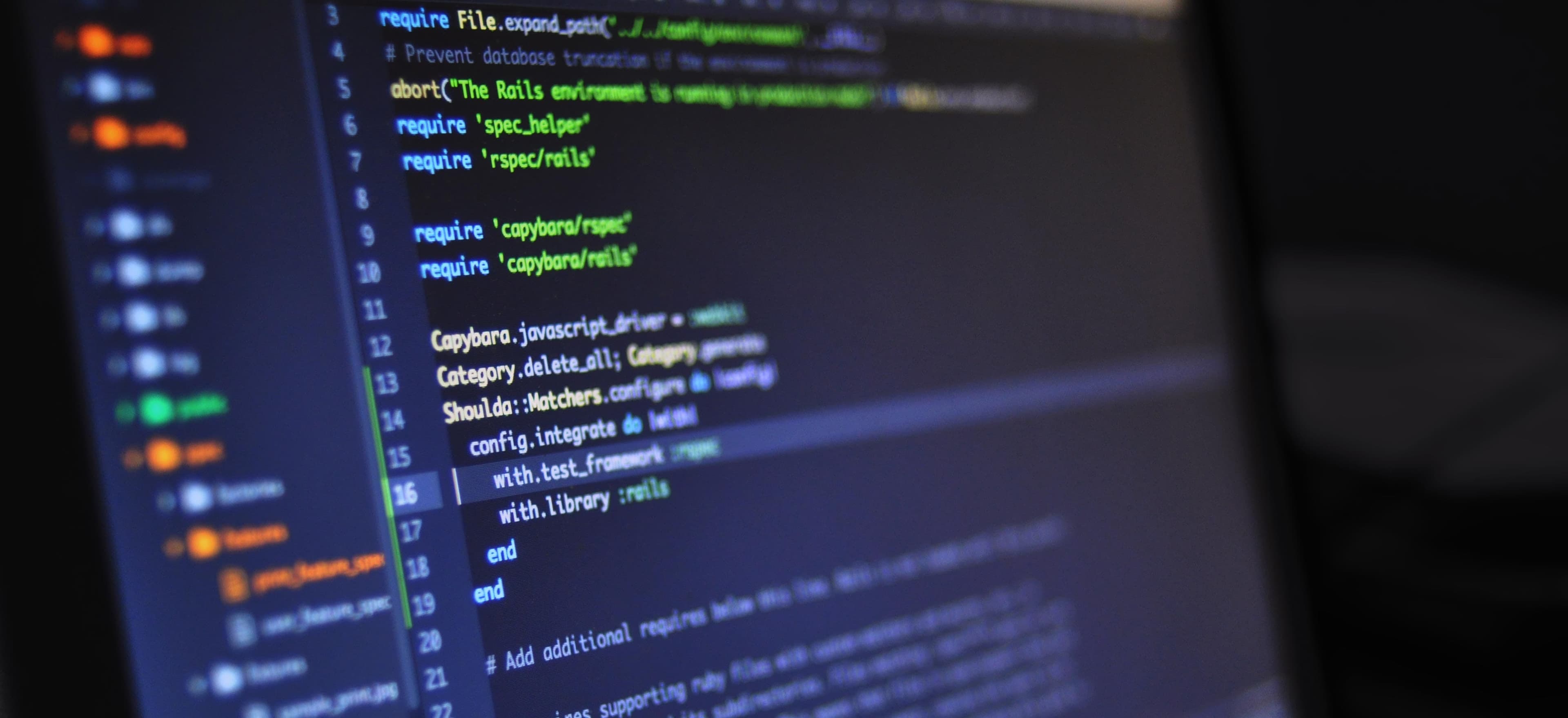
- Published on
Mastering Proximity Alerts: Common Pitfalls and Solutions
In today's rapidly evolving tech landscape, proximity alerts serve as an invaluable feature for a variety of applications, from location-based services to smart home systems. However, implementing these alerts comes with its own set of challenges. In this blog post, we will delve into the common pitfalls encountered while coding proximity alerts in Java applications and provide actionable solutions to overcome them.
What Are Proximity Alerts?
Proximity alerts are notifications triggered based on the physical proximity of an object, such as a user or device, to a specific location. These alerts rely on location services and sensors to operate efficiently. Understanding how to implement this feature correctly can greatly enhance user experience.
For more details on location services, visit this Android Developer Guide on Location Services.
Key Pitfalls in Implementing Proximity Alerts
1. Poor Location Accuracy
The Issue
One of the most common issues in proximity alerts is relying on location data that is not accurate. A small GPS error can lead to false alerts or missed notifications.
Solution
To improve location accuracy:
- Use a combination of GPS, Wi-Fi, and cellular data to obtain the best possible location fix.
- Implement a location filtering algorithm that smooths out the readings.
import android.location.Location;
import android.location.LocationManager;
public class LocationService {
private LocationManager locationManager;
public LocationService(LocationManager locationManager) {
this.locationManager = locationManager;
}
public Location getBestLocation() {
Location bestLocation = null;
// Getting last known location
Location lastKnownLocation = locationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
if (lastKnownLocation != null && (bestLocation == null || lastKnownLocation.getAccuracy() < bestLocation.getAccuracy())) {
bestLocation = lastKnownLocation;
}
// Continue checking Wi-Fi, cellular, etc., to improve accuracy...
return bestLocation;
}
}
In this code snippet, we check the last known location from the GPS provider to ensure that we are starting from the best available data. Fine-tuning your approach to getting location data is crucial for enhancing alert accuracy.
2. Battery Drain
The Issue
Constantly accessing location data can lead to rapid battery drain. Users are likely to disable your app if it consumes too much power.
Solution
- Use Android's "Fused Location Provider" which intelligently manages location updates, ensuring minimal battery usage.
- Set appropriate update intervals based on user requirements.
import com.google.android.gms.location fusedLocationProviderClient;
public class ProximityAlertService {
private FusedLocationProviderClient fusedLocationClient;
public ProximityAlertService(Context context) {
fusedLocationClient = LocationServices.getFusedLocationProviderClient(context);
}
public void requestLocationUpdates(LocationRequest request) {
fusedLocationClient.requestLocationUpdates(request, locationCallback, Looper.getMainLooper());
}
}
Using the FusedLocationProviderClient
, this approach balances performance improvements and battery efficiencies. A well-optimized location request can significantly mitigate battery drain issues.
3. Handling Permissions
The Issue
A frequent pitfall is improperly handling runtime permissions, especially in Android 6.0 (API level 23) and above. If users do not grant location permissions, your proximity alerts will not function.
Solution
- Implement proper checks for permissions and request them where necessary.
if (ContextCompat.checkSelfPermission(context, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(activity, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, LOCATION_PERMISSION_REQUEST_CODE);
}
Always check for permissions before trying to access location data. Be transparent with users about why your app needs these permissions, as this can increase user trust and engagement.
4. Ignoring Geofencing Limitations
The Issue
Many developers improperly configure geofences, ignoring limitations like the maximum number of geofences and the accuracy of the defined areas.
Solution
- Understand and respect geofence constraints. Use appropriate parameters and test them thoroughly.
Geofence geofence = new Geofence.Builder()
.setRequestId("home")
.setCircularRegion(latitude, longitude, radius)
.setExpirationDuration(Geofence.NEVER_EXPIRE)
.setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER | Geofence.GEOFENCE_TRANSITION_EXIT)
.build();
Geofences are crucial for proximity alerts. Make sure to regularly review any limitations set by the platform, especially when considering expanding your app to handle multiple geofences.
5. Incorrect Event Handling
The Issue
When geofence events trigger erroneously or without logic, it can lead to user frustration and app abandonment.
Solution
- Ensure proper logging and event handling mechanisms to respond to changes in location effectively.
@Override
public void onReceive(Context context, Intent intent) {
GeofencingEvent geofencingEvent = GeofencingEvent.fromIntent(intent);
if (geofencingEvent.hasError()) {
Log.e(TAG, "Error receiving geofencing event");
return;
}
int transitionType = geofencingEvent.getGeofenceTransition();
if (transitionType == Geofence.GEOFENCE_TRANSITION_ENTER) {
// Handle entry transition
} else if (transitionType == Geofence.GEOFENCE_TRANSITION_EXIT) {
// Handle exit transition
}
}
In this event handler, you can see how to process geofence transitions efficiently. Proper logging will help you debug issues and enhance the reliability of your proximity alerts.
To Wrap Things Up
Implementing proximity alerts in your Java applications involves navigating several potential pitfalls. From optimizing location accuracy to efficiently managing battery life and respecting user permissions, each element plays a significant role in the user experience.
By employing the strategies and code snippets outlined in this post, you can refine your applications and provide reliable, effective proximity alerts that your users will appreciate.
For additional insights into location-based services and proximity alerts, visit the Google Developer Documentation.
FAQs
What should I do if my app won't receive location updates?
Check your permissions, ensure that the settings are correct for your location services, and optimize your Fused Location Provider settings.
How can I improve battery consumption in my location-based app?
Use Fused Location Provider for location requests, set reasonable intervals, and avoid continuous location updates when unnecessary.
Feel free to comment below if you have any questions or if there are other aspects of proximity alerts you would like to explore further!