Simplifying Exception Handling in JUnit Tests
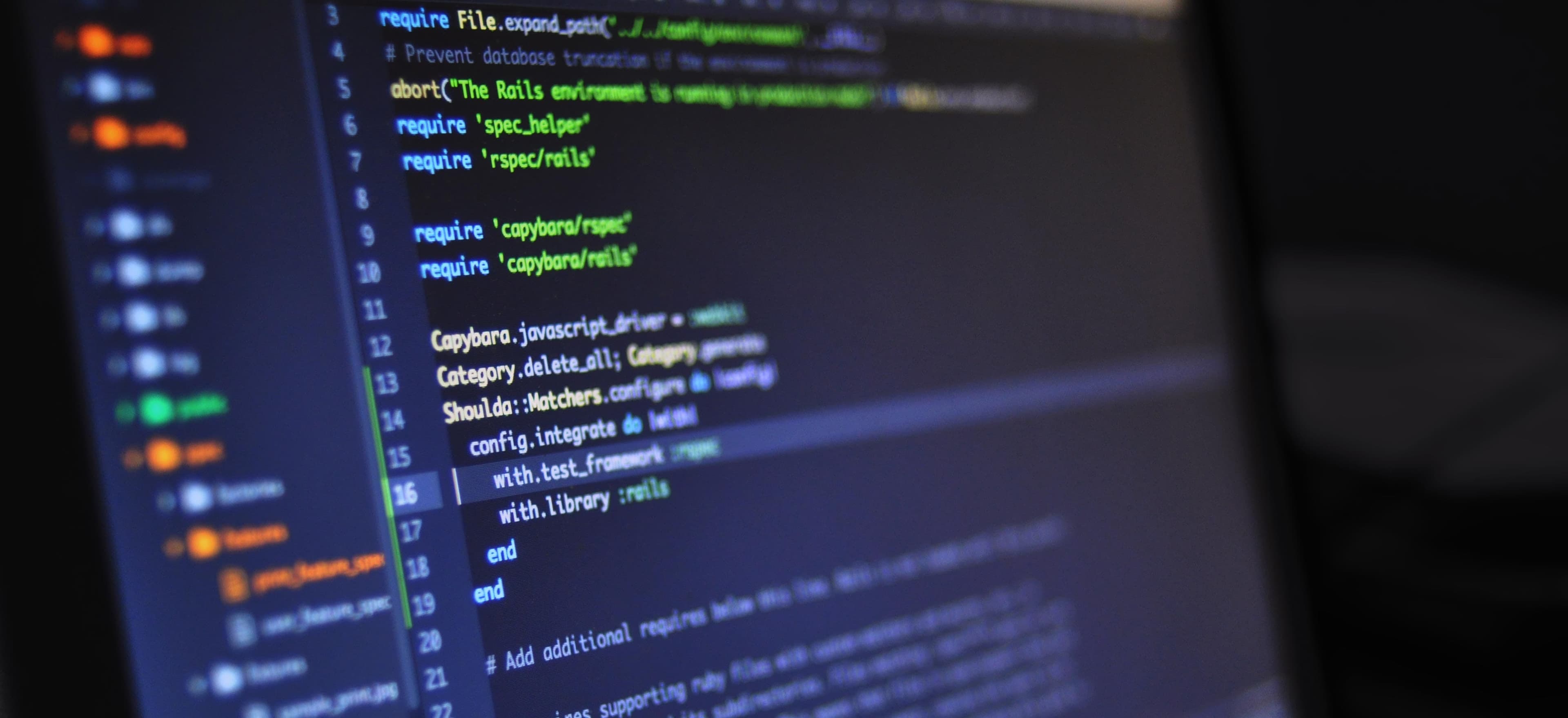
- Published on
Simplifying Exception Handling in JUnit Tests
Testing is a fundamental part of software development, and ensuring that your code handles exceptions appropriately is crucial for delivering robust applications. In Java, JUnit is the go-to framework for unit testing, and it offers powerful features for exception handling. However, developers often grapple with how to effectively manage exceptions in their tests. In this blog post, we’ll explore some best practices and techniques to simplify exception handling in JUnit tests.
What Are JUnit Tests?
JUnit is an open-source testing framework for Java programmers. It provides annotations, assertions, and test runners to facilitate test-driven development (TDD). Writing effective JUnit tests not only helps you verify that your code works as intended but also guarantees that your exception handling logic is robust and reliable.
Why is Exception Handling Important?
Exception handling is vital for any application that interacts with external resources, such as databases, file systems, or web services. Properly handling exceptions ensures that your application doesn't crash unexpectedly and can provide meaningful feedback to users and developers alike.
In a JUnit context, testing how your code handles exceptions helps ensure that:
- Errors Are Managed Gracefully: Your application remains stable and functional, even when an unexpected condition occurs.
- User Experience Is Enhanced: Users receive informative error messages rather than cryptic stack traces.
- Regression Issues Are Minimized: New changes don't introduce bugs related to exception handling.
Basic Exception Handling in JUnit
JUnit provides built-in tools to assert that exceptions are thrown. The traditional approach involves using the @Test(expected = …)
annotation. Below is an example illustrating this method.
Example 1: Using @Test(expected = ...)
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
Calculator calculator = new Calculator();
calculator.divide(10, 0);
}
}
In this example, we test a division operation that should produce an ArithmeticException
when we attempt to divide by zero. This approach is simple, effective, but has its limitations, especially when you want to perform additional assertions on the exception.
Using ExpectedException
Rule
While the @Test(expected = …)
annotation is simple, it prevents additional assertions on the exception object. To overcome this limitation, we can utilize the ExpectedException
rule.
Example 2: Using ExpectedException
Rule
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
public class CalculatorTest {
@Rule
public ExpectedException thrown = ExpectedException.none();
@Test
public void testDivisionByZero() {
thrown.expect(ArithmeticException.class);
thrown.expectMessage("Division by zero");
Calculator calculator = new Calculator();
calculator.divide(10, 0);
}
}
In this example, we have extended our test by asserting the specific exception message as well. This not only confirms the type of exception thrown but also checks if it conveys the correct message, adding depth to your test cases.
Using AssertJ for More Robust Assertions
JUnit works seamlessly with libraries like AssertJ, which provide fluent assertions and can simplify the syntax of your tests. AssertJ makes it easier to write readable and maintainable tests.
Example 3: Using AssertJ
import static org.assertj.core.api.Assertions.assertThatThrownBy;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testDivisionByZero() {
Calculator calculator = new Calculator();
assertThatThrownBy(() -> calculator.divide(10, 0))
.isInstanceOf(ArithmeticException.class)
.hasMessage("Division by zero");
}
}
This example uses assertThatThrownBy()
, which provides a more readable way to express your expectations. The chaining methods allow you to clearly specify what you're asserting about the exception.
How to Handle Multiple Exceptions
Sometimes, a single method might throw various exceptions based on its input parameters. It's important to write tests that can validate these conditions without clutter. One approach to handle multiple exceptions elegantly is by creating a parameterized test.
Example 4: Parameterized Tests
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import static org.junit.Assert.assertEquals;
@RunWith(Parameterized.class)
public class CalculatorTest {
private final int a;
private final int b;
private final Class<? extends Exception> expectedException;
public CalculatorTest(int a, int b, Class<? extends Exception> expectedException) {
this.a = a;
this.b = b;
this.expectedException = expectedException;
}
@Parameterized.Parameters
public static Object[][] data() {
return new Object[][] {
{10, 0, ArithmeticException.class},
{10, -1, IllegalArgumentException.class}
};
}
@Test
public void testDivisionThrowsException() {
try {
Calculator calculator = new Calculator();
calculator.divide(a, b);
} catch (Exception e) {
assertEquals(expectedException, e.getClass());
}
}
}
In this parameterized test, we can test various input combinations without duplicating code. Each test case feeds a different set of parameters into the test method. This is a clean way to verify that your code behaves as expected under various conditions.
Final Thoughts
Exception handling in JUnit tests doesn't have to be complex. By leveraging built-in features, additional libraries like AssertJ, and writing parameterized tests, we can simplify our approach and maintain clarity. Ensuring robust exception management will result in cleaner code and improved application stability.
By following these best practices, your unit tests will not only cover typical success cases but also gracefully handle exceptions, which is crucial in developing a resilient application. For more in-depth reading on JUnit and exception handling, check out JUnit 5 User Guide and Effective Java - Exception Handling.
With these strategies, you can improve exception handling in your tests and ultimately build better Java applications. Happy testing!