Simplifying Spring Integration with JMS: A Step-by-Step Guide
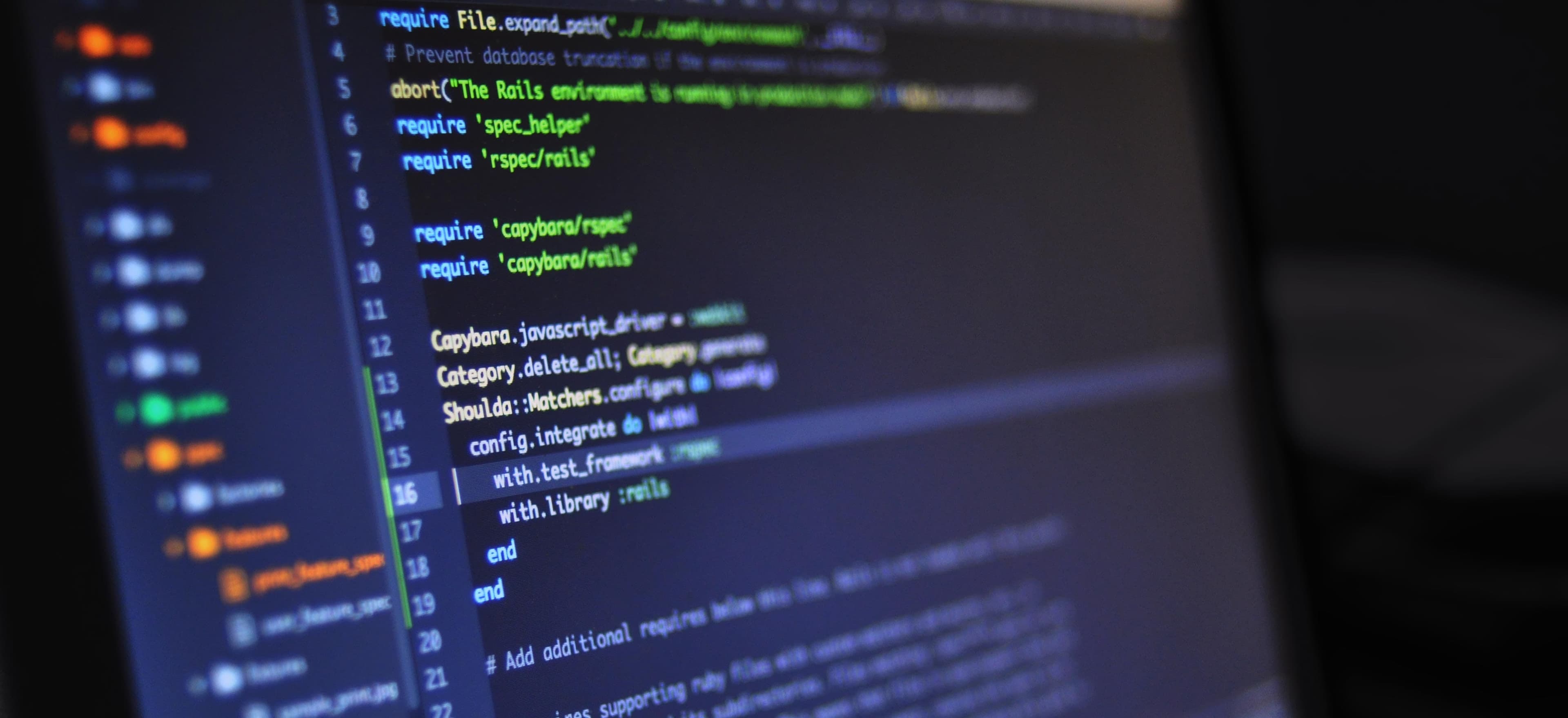
- Published on
Simplifying Spring Integration with JMS: A Step-by-Step Guide
Spring Integration is a powerful framework for building enterprise integration solutions within the Spring ecosystem. When combined with JMS (Java Message Service), it allows seamless communication between different applications. In this blog post, we will walk you through the process of simplifying Spring Integration with JMS, providing clear steps and practical examples along the way.
Table of Contents
- Understanding Spring Integration and JMS
- Dependencies and Setup
- Defining the Message Flow
- Implementing the JMS Messaging Configuration
- Creating Message Listeners
- Testing Your Integration
- Conclusion
Understanding Spring Integration and JMS
Spring Integration provides an abstraction layer over messaging systems. It allows you to define message-driven architectures with ease. When you integrate JMS, you're essentially enabling your application to communicate asynchronously through messages, promoting decoupling and scalability.
Why Use JMS with Spring Integration?
- Asynchronous Processing: JMS facilitates asynchronous communication, resulting in improved application performance.
- Decoupling Systems: Applications can run independently, allowing for easier changes and maintenance.
- Integration with Legacy Systems: JMS can help incorporate existing systems without major overhauls.
To understand how to implement this, it's important to first set up your development environment.
Dependencies and Setup
To build a Spring Integration application with JMS, you'll need to include relevant dependencies in your pom.xml
if you are using Maven. Here’s a simplified setup:
<dependencies>
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-jms</artifactId>
<version>5.5.3</version>
</dependency>
<dependency>
<groupId>org.apache.activemq</groupId>
<artifactId>activemq-spring-boot-starter</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
</dependencies>
Here, we have included both Spring Integration and ActiveMQ (a popular JMS provider). Make sure you adapt the versions to match your project needs.
Defining the Message Flow
Next, we need to define the message flow in the application. A classic flow might consist of a message producer, channel, and consumer. This can be set up in an XML configuration or Java-based configuration, but we will go with Java here.
Example:
- Message Producer: This component will send a message to a defined channel.
- Message Channel: A point of communication between the producer and consumer.
- Message Consumer: This component listens for incoming messages from the channel and processes them.
Here is a simple definition using Java:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.annotation.IntegrationComponentScan;
import org.springframework.integration.annotation.ServiceActivator;
import org.springframework.integration.channel.DirectChannel;
import org.springframework.integration.jms.JmsOutboundGateway;
import org.springframework.jms.annotation.EnableJms;
@Configuration
@EnableJms
@IntegrationComponentScan
public class JmsConfig {
@Bean
public DirectChannel inputChannel() {
return new DirectChannel();
}
@Bean
@ServiceActivator(inputChannel = "inputChannel")
public JmsOutboundGateway jmsOutboundGateway() {
JmsOutboundGateway gateway = new JmsOutboundGateway();
gateway.setDestinationName("myQueue");
return gateway;
}
}
Why This Matters: The above configuration sets up a direct channel for messages. We then create a JmsOutboundGateway
that sends messages to a JMS destination named myQueue
.
Implementing the JMS Messaging Configuration
Next, we configure the ActiveMQ connection. This step is crucial, as it enables the application to connect to the messaging provider.
import org.apache.activemq.ActiveMQConnectionFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.annotation.EnableJms;
import org.springframework.jms.config.DefaultJmsListenerContainerFactory;
import org.springframework.jms.core.JmsTemplate;
import javax.jms.ConnectionFactory;
@Configuration
@EnableJms
public class MessagingConfig {
@Bean
public ConnectionFactory connectionFactory() {
return new ActiveMQConnectionFactory("tcp://localhost:61616");
}
@Bean
public JmsTemplate jmsTemplate() {
return new JmsTemplate(connectionFactory());
}
@Bean
public DefaultJmsListenerContainerFactory myFactory() {
DefaultJmsListenerContainerFactory factory = new DefaultJmsListenerContainerFactory();
factory.setConnectionFactory(connectionFactory());
return factory;
}
}
Why Configure a Connection Factory?: This allows the Spring application to connect to the ActiveMQ broker, handling all connection pooling and threading behind the scenes.
Creating Message Listeners
Once you've set up the configuration, it's time to implement listeners. Listeners are components that handle incoming messages. Below is an example of a simple message listener:
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class MyMessageListener {
@JmsListener(destination = "myQueue")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
}
Explaining the Code: The @JmsListener
annotation marks the method that will process incoming messages from the queue. This way, your application automatically listens to the myQueue
and picks up any messages.
Testing Your Integration
Testing your message flow is essential before going to production. Here is a simple way to test it:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.jms.core.JmsTemplate;
import org.springframework.stereotype.Component;
@Component
public class MessageSender implements CommandLineRunner {
@Autowired
private JmsTemplate jmsTemplate;
@Override
public void run(String... args) throws Exception {
jmsTemplate.convertAndSend("myQueue", "Hello, JMS!");
System.out.println("Message sent to the queue!");
}
}
Testing the Flow: This component sends a test message to the queue when the application starts. To verify the integration, you should see the received message printed out by your listener.
The Bottom Line
Integrating Spring with JMS simplifies handling messaging with clear abstraction and configuration. Throughout this guide, we've covered:
- Understanding the integration of Spring and JMS.
- Setting up dependencies and configuration.
- Creating message producers, consumers, and channels.
- Testing the integration effectively.
By following these steps, you create an efficient asynchronous architecture that improves performance and maintainability in your applications. With the right integration strategy, your systems can become more flexible and responsive, paving the way for future growth.
For more information on Spring Integration, visit the official documentation: Spring Integration Documentation.
Feel free to ask questions or share your experiences below!