Mastering File and Directory Attributes in Java SE
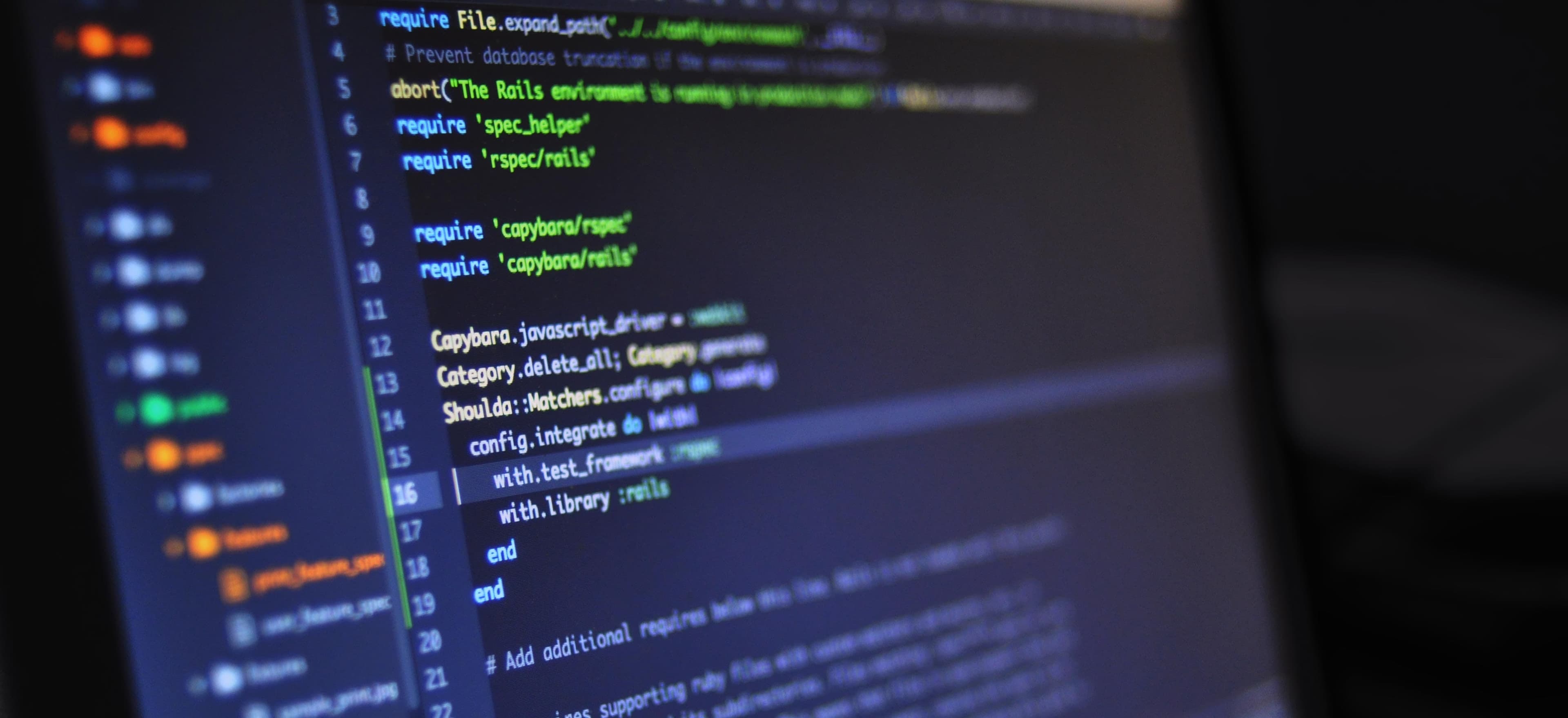
- Published on
Mastering File and Directory Attributes in Java SE
Java, a well-established programming language, provides powerful APIs for managing files and directories. In this post, we delve into the nuances of file and directory attributes in Java SE, explaining how to access and manipulate these attributes effectively. This understanding is paramount for tasks such as maintaining file integrity, checking file properties, or even handling user permissions.
Table of Contents
- Introduction to File Attributes
- Getting Started with NIO.2
- Accessing Basic File Attributes
- Modifying File Attributes
- Advanced File Attributes
- Conclusion
- References
A Quick Look to File Attributes
In Java, file attributes refer to metadata about files and directories. These attributes include information such as file size, last modified date, owner, permissions, and more. Manipulating these attributes can prove useful in file management, enhancing security, and ensuring the application behaves as intended.
Java SE introduced the NIO.2 (New Input/Output) package in Java 7, which significantly improved file handling capabilities compared to the original IO package. The key classes in NIO.2, namely Path
, Files
, and FileSystems
, allow developers to perform operations efficiently and intuitively.
Getting Started with NIO.2
To work with NIO.2, we need to use the following core classes:
Path
: Represents the file path in the file system.Files
: Contains static methods for file manipulation.LinkOption
: Indicates how symbolic links are handled.
Here's a simple example demonstrating how to create a Path
object:
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileAttributesExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
System.out.println("Path: " + path.toString());
}
}
In this code, we utilize Paths.get()
to create a Path
object representing example.txt
. Understanding how to create and manipulate Path
objects lays the groundwork for accessing file attributes.
Accessing Basic File Attributes
Java provides the Files
class, which is equipped with methods to retrieve basic attributes of files. To access attributes like size
, creation time
, and last modified time
, we utilize the readAttributes
method.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.BasicFileAttributes;
import java.io.IOException;
public class BasicFileAttributesExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
// Read Basic File Attributes
BasicFileAttributes attrs = Files.readAttributes(path, BasicFileAttributes.class);
// Displaying the attributes
System.out.println("Size: " + attrs.size() + " bytes");
System.out.println("Creation Time: " + attrs.creationTime());
System.out.println("Last Modified Time: " + attrs.lastModifiedTime());
System.out.println("Is Directory: " + attrs.isDirectory());
} catch (IOException e) {
System.err.println("Error reading file attributes: " + e.getMessage());
}
}
}
In this snippet, we read the basic file attributes of example.txt
. The BasicFileAttributes
class provides convenient methods to get essential file details.
Why Access Basic Attributes?
Understanding file properties allows developers to conditionally execute operations based on the file states, such as checking if a file has been modified before taking action.
Modifying File Attributes
In addition to reading file attributes, we may need to modify them as well. However, not all attributes can be changed.
One common update is modifying the last modified time. To do this, we utilize the setLastModifiedTime
method from the Files
class:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.FileTime;
import java.io.IOException;
public class ModifyFileAttributesExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
// Set Last Modified Time to the current time
FileTime now = FileTime.fromMillis(System.currentTimeMillis());
Files.setLastModifiedTime(path, now);
System.out.println("Last modified time updated to now.");
} catch (IOException e) {
System.err.println("Error updating file attributes: " + e.getMessage());
}
}
}
This code sets the last modified time of example.txt
to the current system time. This can be essential for cache management, backup systems, or synchronization tasks.
Why Modify Attributes?
Updating file metadata without changing the file content can simplify time-based operations such as logging changes, version controls, and other auditing processes.
Advanced File Attributes
Apart from basic attributes, Java allows access to more advanced attributes. These include ownership and permission-related attributes provided by classes such as PosixFileAttributes
(Linux/Unix) and AclFileAttributes
(Windows).
Here’s how to access advanced POSIX attributes:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.PosixFileAttributes;
import java.io.IOException;
public class PosixAttributesExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
// Read POSIX File Attributes
PosixFileAttributes attrs = Files.readAttributes(path, PosixFileAttributes.class);
// Display Owner
System.out.println("Owner: " + attrs.owner().getName());
// Display Group
System.out.println("Group: " + attrs.group().getName());
// Display Permissions
System.out.println("Permissions: " + attrs.permissions());
} catch (IOException e) {
System.err.println("Error reading POSIX file attributes: " + e.getMessage());
}
}
}
Why Advanced Attributes Matter?
Advanced attributes, especially ownership and permission details, are essential for applications requiring precise control over access rights. This is particularly important in server-side applications where security is of utmost concern.
My Closing Thoughts on the Matter
Mastering file and directory attributes in Java SE is a crucial skill for any Java developer. By leveraging the capabilities of the NIO.2 package, developers can efficiently manage files, access critical metadata, and perform necessary modifications with ease.
As we move into an increasingly file-driven world, understanding these concepts becomes integral to writing robust Java applications. Dive deeper into file operations, explore error handling, and put your knowledge into practice to truly solidify these concepts.
For more information on file I/O in Java, check out the official documentation on Java NIO.2.
References
- Java Documentation - NIO.2
- Understanding File and Directory Attributes
- Java File Management Basics
Now go ahead, experiment with what you learned, and leverage file and directory attributes effectively in your Java applications!