Understanding the Challenges of Dismantling InvokeDynamic
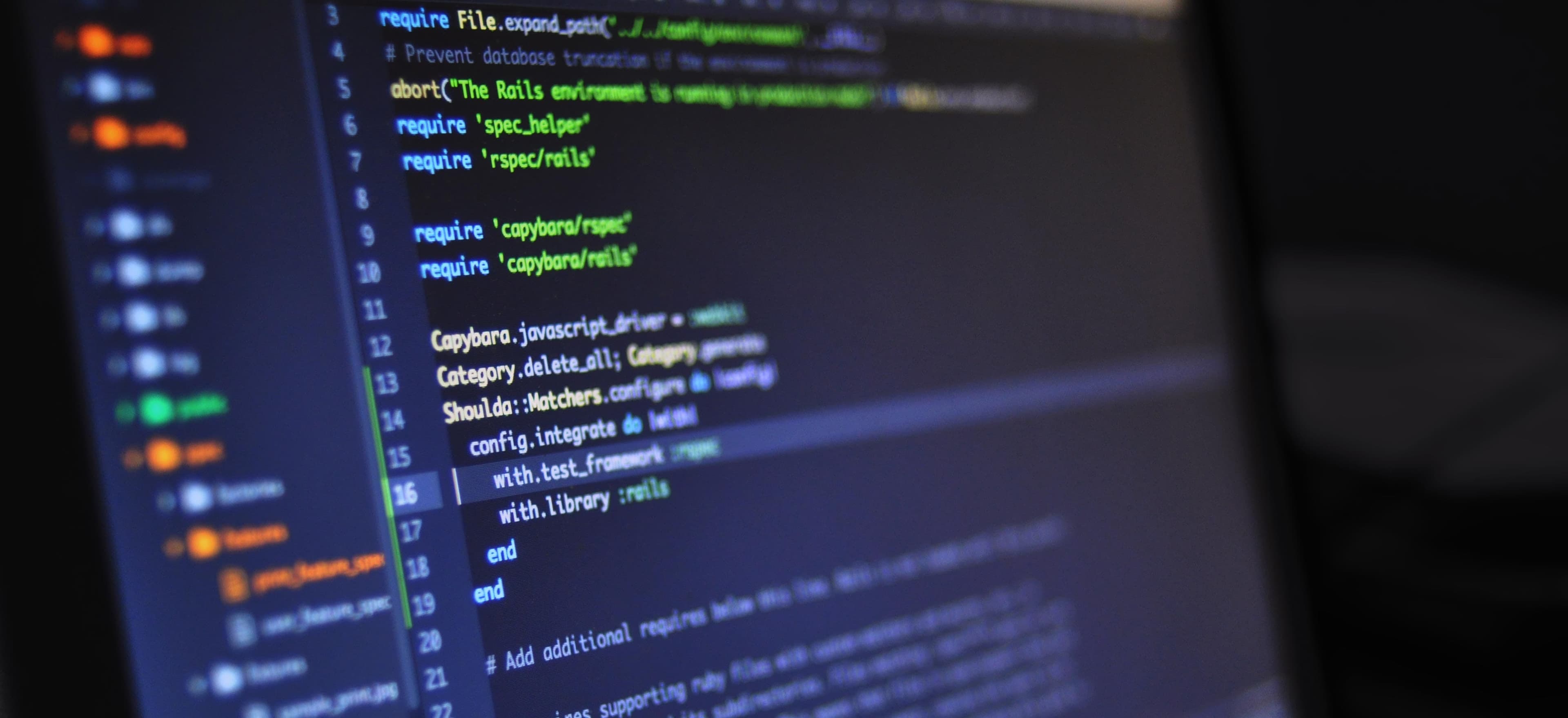
- Published on
Understanding the Challenges of Dismantling InvokeDynamic
InvokeDynamic is a powerful feature introduced in Java 7 as part of the JVM's effort to improve support for dynamically typed languages on the Java platform. However, this flexibility comes with a set of challenges, especially when it comes to dismantling or understanding its mechanics. In this blog post, we'll dive deep into the intricacies of InvokeDynamic, explore why it is essential, and discuss the challenges developers may face when attempting to manipulate or dismantle its functionality.
What is InvokeDynamic?
InvokeDynamic is a bytecode instruction that allows the Java Virtual Machine (JVM) to dynamically determine the method to be invoked at runtime. This instruction is primarily designed to support languages that operate outside the static typing system Java enforces.
The Significance of InvokeDynamic
One may wonder: why was InvokeDynamic introduced? The answer lies primarily with the need for better performance and versatility when running various languages on the JVM. Prior to InvokeDynamic, languages like Groovy or JRuby struggled with performance because they relied on reflection and dynamic method lookup, which are relatively slow compared to static calls.
InvokeDynamic streamlines this process by enabling dynamic linking of methods, which allows for optimizations that static languages cannot offer.
The Basic Syntax
To understand InvokeDynamic functionality more thoroughly, let's look at a simple example.
import java.lang.invoke.*;
public class InvokeDynamicExample {
public static void main(String[] args) throws Throwable {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodHandle handle = lookup.findStatic(InvokeDynamicExample.class, "sayHello", MethodType.methodType(void.class));
// Invoke the method dynamically
handle.invokeExact();
}
public static void sayHello() {
System.out.println("Hello, from InvokeDynamic!");
}
}
Code Explanation
MethodHandles.Lookup
: This is used to perform dynamic lookups of methods.lookup.findStatic(...)
: This locates the static method we want to call dynamically.handle.invokeExact()
: This calls the method through theMethodHandle
, enabling us to invoke the method dynamically.
The primary advantage of using MethodHandle
is the performance gain it provides over reflection. The run-time performance is closer to direct method invocations, leading to improved efficiency.
Challenges of Dismantling InvokeDynamic
While InvokeDynamic presents numerous advantages, dismantling it for understanding or modification purposes can be complex. Here are some notable challenges:
1. Complexity of Dynamic Typing
Because InvokeDynamic supports dynamic typing, the code becomes inherently more complex. Unlike static typing, where types are known at compile time, dynamic types are resolved at runtime.
Implication
This complexity can introduce runtime errors that could have been caught at compile time. Debugging these issues can be tedious, especially in a large codebase.
2. Performance Overhead
While InvokeDynamic offers performance improvements, there is still associated overhead due to the dynamic nature of method resolution. The initial call to the method handle may take longer than a direct method invocation.
Example
public static void dynamicCall(int iterations) throws Throwable {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodHandle handle = lookup.findStatic(InvokeDynamicExample.class, "sayHello", MethodType.methodType(void.class));
for (int i = 0; i < iterations; i++) {
// Calling the method dynamically
handle.invokeExact();
}
}
In this example, even if every invocation is optimized eventually, the first few invocations may take longer due to the overhead of establishing the method handle.
3. Increased Boilerplate Code
When using InvokeDynamic, the amount of boilerplate code can increase significantly. Developers often find themselves writing additional code to handle method lookups and manage method handles.
Solution Suggestions
One way to mitigate this issue is by encapsulating frequently used patterns in utility classes. This can help reduce redundancy throughout your codebase and streamline the use of InvokeDynamic.
4. Limitations with Cross-Platform Compatibility
Not all features supported by InvokeDynamic are available across different JVM implementations. Some languages built on top of the JVM may not fully utilize the InvokeDynamic capabilities.
Consideration
Before migrating projects or integrating third-party libraries, ensure that the InvokeDynamic features you plan to use are supported across your targeted platforms.
Dealing with InvokeDynamic Challenges
Here are some strategies to effectively manage the challenges associated with using InvokeDynamic in your Java projects:
1. Profiling and Optimization
Use profiling tools to identify performance bottlenecks when using InvokeDynamic. The Java Flight Recorder and tools like VisualVM can be invaluable for monitoring how your application performs at runtime and where improvements can be made.
2. Abstraction Layers
Develop abstractions or libraries that encapsulate common usage patterns for InvokeDynamic. This not only reduces boilerplate code but also makes it easier for other developers to work with without needing in-depth knowledge of InvokeDynamic.
3. Comprehensive Testing
Adopt a robust testing framework to ensure that dynamic features work as intended. Since dynamic typing may lead to runtime errors, implementing full test coverage for your dynamic calls will help catch issues early.
4. Documentation
Provide clear documentation regarding the usage of InvokeDynamic in your codebase. This should include how to set up method handles, expected performance behavior, and any nuances involved in dynamic method calls.
Key Takeaways
InvokeDynamic is a powerful tool for enabling dynamic language support within the JVM environment, allowing developers to utilize methods dynamically rather than through the traditional static means. However, this flexibility also brings specific challenges such as complexity, performance overhead, and increased boilerplate code.
By understanding these challenges and applying appropriate strategies, you can take full advantage of what InvokeDynamic has to offer. The key is to strike a balance between leveraging its capabilities while maintaining a clear, manageable codebase.
Additional Resources
- [Oracle Documentation on InvokeDynamic](https://docs.oracle.com/javase/specs/jvms/se11/html/jvms- invokedynamic.html)
- Java Tutorials on Method Handles
- Performance Tuning with Java Flight Recorder
By addressing the complexities inherent in InvokeDynamic, developers can enhance the performance and maintainability of their Java applications while fully utilizing the JVM’s capabilities.