Mastering Mockito: Mocking ResultSet Like a Pro
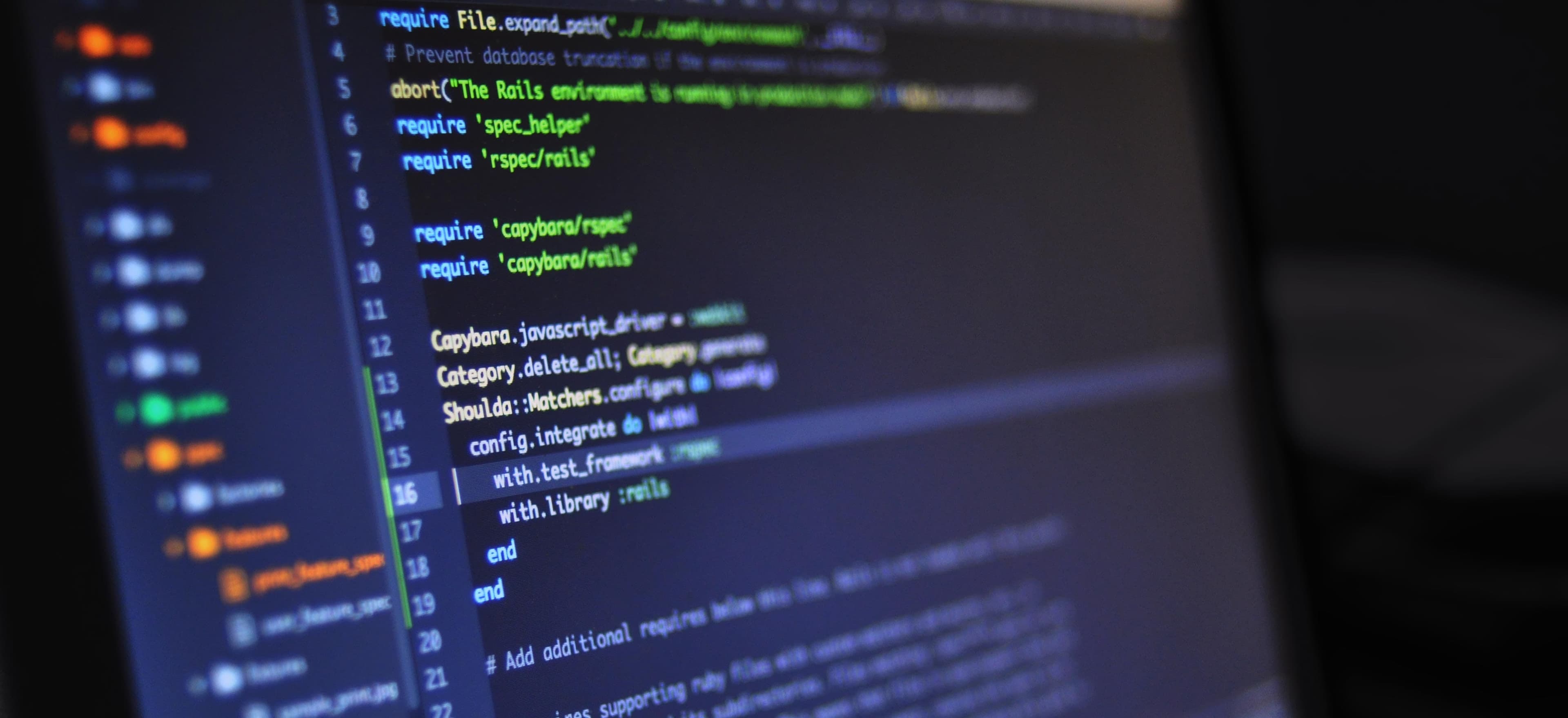
- Published on
Mastering Mockito: Mocking ResultSet Like a Pro
In the world of Java development, unit testing plays a pivotal role in ensuring that the code behaves as expected. One of the critical dependencies in many applications is the JDBC ResultSet. Mocking this dependency can be a bit challenging, especially when you want to validate how your business logic reacts to various states of the data returned from the database. In this blog post, we will explore how to effectively mock a ResultSet
using Mockito, a powerful mocking framework for Java.
Table of Contents
- Introduction to Mockito
- The Importance of Mocking in Unit Testing
- Overview of ResultSet
- Setting Up Your Environment
- Mocking ResultSet with Mockito
- Basic Mocking
- Mocking Different Scenarios
- Conclusion and Best Practices
Before We Begin to Mockito
Mockito is a popular framework that allows developers to create test doubles for Java objects. With Mockito, you can easily mock, stub, and verify behaviors of classes that interact with external systems, such as databases or APIs. Its intuitive API makes writing tests simpler and more readable.
Learn more about Mockito here.
The Importance of Mocking in Unit Testing
Mocking is essential in unit testing because it allows you to isolate units of work. This isolation is particularly important when those units interact with external systems. Mocking helps to:
- Reduce dependencies: Avoid integration tests can lead to slower execution.
- Control the test environment: You can simulate various scenarios.
- Focus on behavior: Verifying interactions rather than the actual implementations.
Overview of ResultSet
In JDBC, ResultSet
is used to access data returned by executing a SQL query. It represents a table of data, each with rows and columns. When working with a ResultSet, you often rely on methods like next()
, getString()
, and getInt()
to retrieve data.
Here’s a simple illustration:
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT id, name FROM users");
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
// Process the data
}
In unit tests, you usually do not want to connect to the actual database. Hence, mocking ResultSet
becomes crucial.
Setting Up Your Environment
To get started with Mockito, ensure you have the following dependencies added to your pom.xml
if you are using Maven:
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>5.0.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
With these dependencies in place, you can begin writing your tests that utilize Mockito.
Mocking ResultSet with Mockito
Once your environment is set up, we can dive into mocking the ResultSet.
Basic Mocking
Here is how to create a simple mocked ResultSet
:
import org.junit.Test;
import org.mockito.Mockito;
import java.sql.ResultSet;
import java.sql.SQLException;
import static org.mockito.Mockito.*;
public class UserDAOTest {
@Test
public void testGetUserById() throws SQLException {
// Create a mock ResultSet
ResultSet mockResultSet = Mockito.mock(ResultSet.class);
// Stub methods to return desired values
when(mockResultSet.next()).thenReturn(true).thenReturn(false);
when(mockResultSet.getInt("id")).thenReturn(1);
when(mockResultSet.getString("name")).thenReturn("John Doe");
// Your Business Logic that uses ResultSet
User user = new UserDAO().getUser(mockResultSet);
// Verify results
assertEquals("John Doe", user.getName());
assertEquals(1, user.getId());
}
}
Why This Approach?
- Controlled Testing Environment: By mocking
ResultSet
, you eliminate the need for a database connection. - Flexibility: You can dictate the flow by controlling what
next()
returns and what values are retrieved.
Mocking Different Scenarios
Mocking different scenarios is one of the strengths of Mockito. You can simulate various database states easily. Here are a few examples:
- Handling Empty Results:
when(mockResultSet.next()).thenReturn(false);
This simulates a scenario where no user exists in the database.
- Throwing Exceptions:
when(mockResultSet.getInt("id")).thenThrow(new SQLException("Database error"));
This tests how your code handles database errors.
Complete Example
Let's wrap all the above concepts into a complete test case.
import org.junit.Test;
import org.mockito.Mockito;
import java.sql.ResultSet;
import java.sql.SQLException;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.*;
public class UserDAOTest {
@Test
public void testGetUserFromResultSet() throws SQLException {
// Simulating a ResultSet
ResultSet mockResultSet = Mockito.mock(ResultSet.class);
// Configuring ResultSet to return data
when(mockResultSet.next()).thenReturn(true).thenReturn(false);
when(mockResultSet.getInt("id")).thenReturn(1);
when(mockResultSet.getString("name")).thenReturn("Jane Doe");
UserDAO userDAO = new UserDAO();
User user = userDAO.getUser(mockResultSet);
// Assertions to verify expected outcomes
assertEquals("Jane Doe", user.getName());
assertEquals(1, user.getId());
}
@Test
public void testEmptyResultSet() throws SQLException {
ResultSet mockResultSet = Mockito.mock(ResultSet.class);
// Configure ResultSet to simulate no records found
when(mockResultSet.next()).thenReturn(false);
UserDAO userDAO = new UserDAO();
User user = userDAO.getUser(mockResultSet);
// Expected user should be null
assertNull(user);
}
@Test(expected = SQLException.class)
public void testResultSetThrowsSQLException() throws SQLException {
ResultSet mockResultSet = Mockito.mock(ResultSet.class);
// Configure to throw an exception
when(mockResultSet.getInt("id")).thenThrow(new SQLException("Database error"));
UserDAO userDAO = new UserDAO();
userDAO.getUser(mockResultSet);
}
}
In this example, we demonstrate how to mock a ResultSet
while covering normal, empty, and exception scenarios.
Final Considerations and Best Practices
Mocking a ResultSet
with Mockito may seem challenging but provides a powerful tool in your unit testing arsenal. Here are some best practices to follow:
- Keep tests isolated: Make sure your tests don’t depend on any state outside of the method they test.
- Group related scenarios: Use @Before and @After setups to reduce redundancy.
- Be explicit: When mocking, ensure it is clear what behavior you intend to simulate.
Ultimately, mastering Mockito can elevate your testing strategy considerably while enhancing code quality and reliability. For a deeper dive into Mockito, consider reading the Mockito documentation.
Happy testing!