Common SLF4J Logging Mistakes to Avoid in Your Code
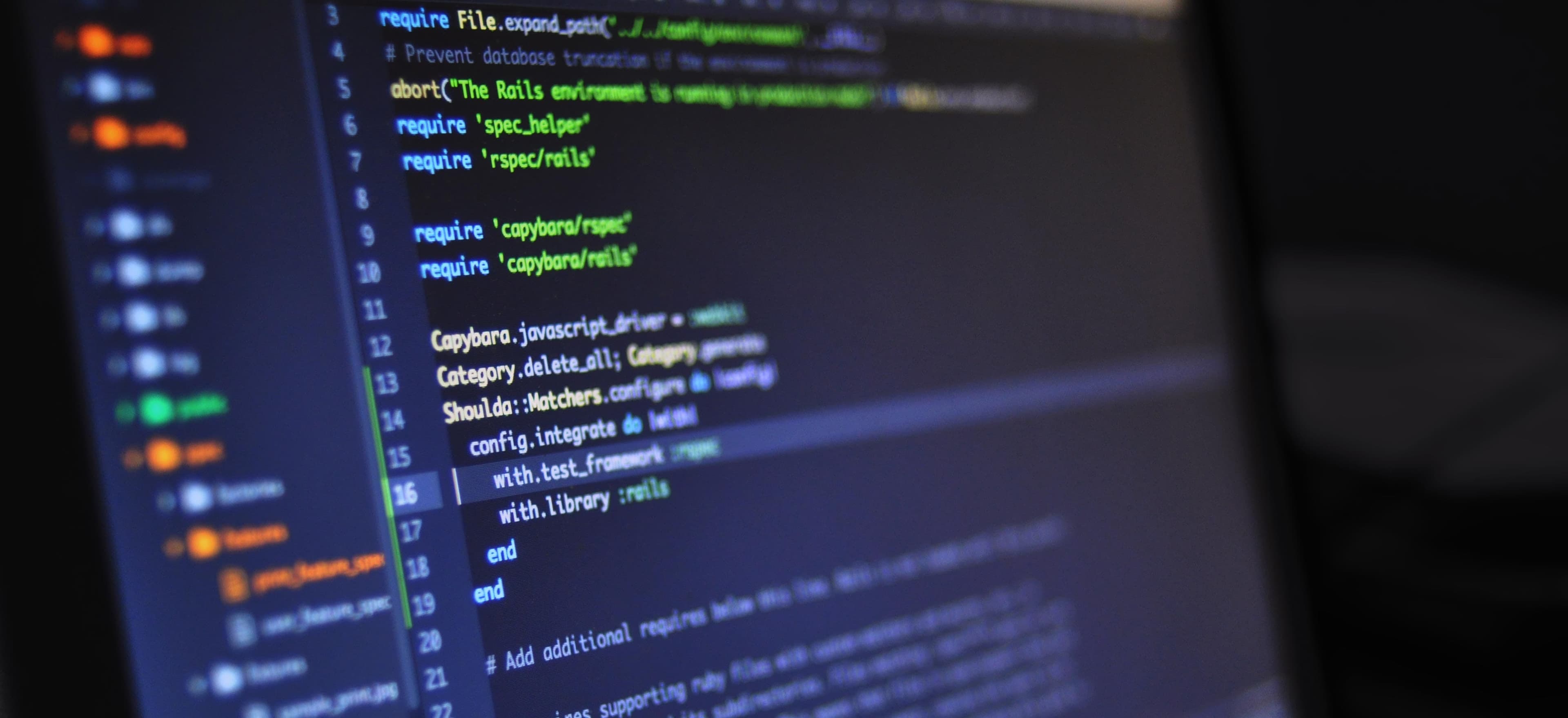
- Published on
Common SLF4J Logging Mistakes to Avoid in Your Code
Logging is an essential aspect of software development. It allows developers to gain insight into their application’s behavior, making debugging easier and enhancing overall maintainability. The Simple Logging Facade for Java (SLF4J) is a popular choice among Java developers. It provides a simple abstraction for various logging frameworks, promoting consistent logging practices across different parts of your application. However, even experienced developers can make common mistakes when implementing SLF4J. In this blog post, we’ll explore these pitfalls and how to avoid them.
Understanding SLF4J
Before diving into mistakes, let's quickly recap what SLF4J is. The SLF4J API allows you to plug in any desired logging framework at deployment time. This means you can switch between frameworks like Logback, Log4J, or java.util.logging without changing your application code.
Key Features of SLF4J
- Parameterization: Avoids the performance penalty of string concatenation.
- Multiple Bindings: Easily switch between logging implementations.
- SLF4J API: Offers a clean, flexible API for logging.
Common SLF4J Logging Mistakes
1. Failing to Use Parameterized Logging
One of the primary mistakes developers make is failing to utilize SLF4J's parameterized logging features. Instead of using string concatenation, SLF4J provides a way to log messages with placeholders.
Example of Poor Practice
logger.info("User {} has logged in.", username);
In the above example, using string concatenation is not optimal.
Improved Practice
logger.info("User {} has logged in", username);
Why This Matters
When you use parameterized logging, SLF4J will handle string interpolation efficiently. It will only create the log message string if the log level is enabled. This means less unnecessary string processing and improved performance, especially in high-frequency logging scenarios.
2. Incorrect Log Levels Usage
Different log levels (TRACE, DEBUG, INFO, WARN, ERROR) serve specific purposes in logging. A frequent mistake is misusing these levels, leading to confusion and cluttered log files.
Example of Poor Practice
logger.debug("User {} has logged in", username);
Using DEBUG
level for important messages can detract from the information that should be genuinely at the INFO
level.
Improved Practice
logger.info("User {} has logged in", username);
Why This Matters
Correct log level usage allows developers and system operators to filter logs effectively. It also aids in conveying the application state clearly. Remember, ERROR
should indicate critical issues, while INFO
should convey significant events that are normal.
3. Ignoring Exceptions
Another common mistake is not logging exceptions correctly. Updating the log level should not simply mean printing the exception stack trace with a simple message.
Example of Poor Practice
catch (Exception e) {
logger.error("An error occurred: " + e.getMessage());
}
This code snippet only records the exception message and not the stack trace.
Improved Practice
catch (Exception e) {
logger.error("An error occurred", e);
}
Why This Matters
Logging the exception alongside its stack trace allows developers to pinpoint where errors occur without needing to reproduce the problem. It provides context, making it easier to understand what went wrong and why.
4. Over-Logging or Under-Logging
Finding the right balance between too much and too little logging is crucial. Over-logging can lead to performance issues and overwhelm log files, making it challenging to find relevant information. Conversely, under-logging can leave you with a lack of visibility into critical application behaviors.
Tips to Maintain Balance
- Use logging judiciously for critical operations.
- Avoid logging every single method call.
- Strike a balance: log important events and errors while maintaining performance.
Why This Matters
Optimizing log verbosity can streamline troubleshooting and system monitoring. Developers can focus on relevant events without sifting through excessive noise.
5. Not Configuring Logback or Other Binds Properly
When using SLF4J with Logback (or any other logging framework), not properly configuring your logging framework can lead to a failure in logging.
Example of Poor Configuration
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
While this may look okay, the configuration is minimal.
Improved Configuration
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
Why This Matters
A structured log format enhances readability and helps in performance monitoring. Adding timestamps, thread names, and log levels improves log file analysis by providing essential context and making it more human-readable.
Best Practices for SLF4J Logging
1. Use a Consistent Log Format
Adopt a uniform logging format across your application, making it easier for developers to understand logs quickly.
2. External Configuration
Always externalize your logging configuration. This makes it simple to adjust logging levels without code changes—a significant advantage in production environments.
3. Log Context-Dependent Data
Logging useful contextual information (such as user IDs or transaction IDs) helps diagnose issues faster and provides crucial operational insights.
Wrapping Up
Proper logging with SLF4J can significantly impact application maintenance, debugging, and performance. Avoiding the common mistakes discussed in this article will empower you to write cleaner, more effective logging statements.
By following best practices and being mindful of how you log events, errors, and exceptions, you establish a reliable logging strategy that serves both developers and system operators effectively.
Don't forget to check out the official SLF4J documentation and Logback documentation for further insights and detailed configurations.
Happy logging!