Solving Memory Leaks in Spring Boot Applications
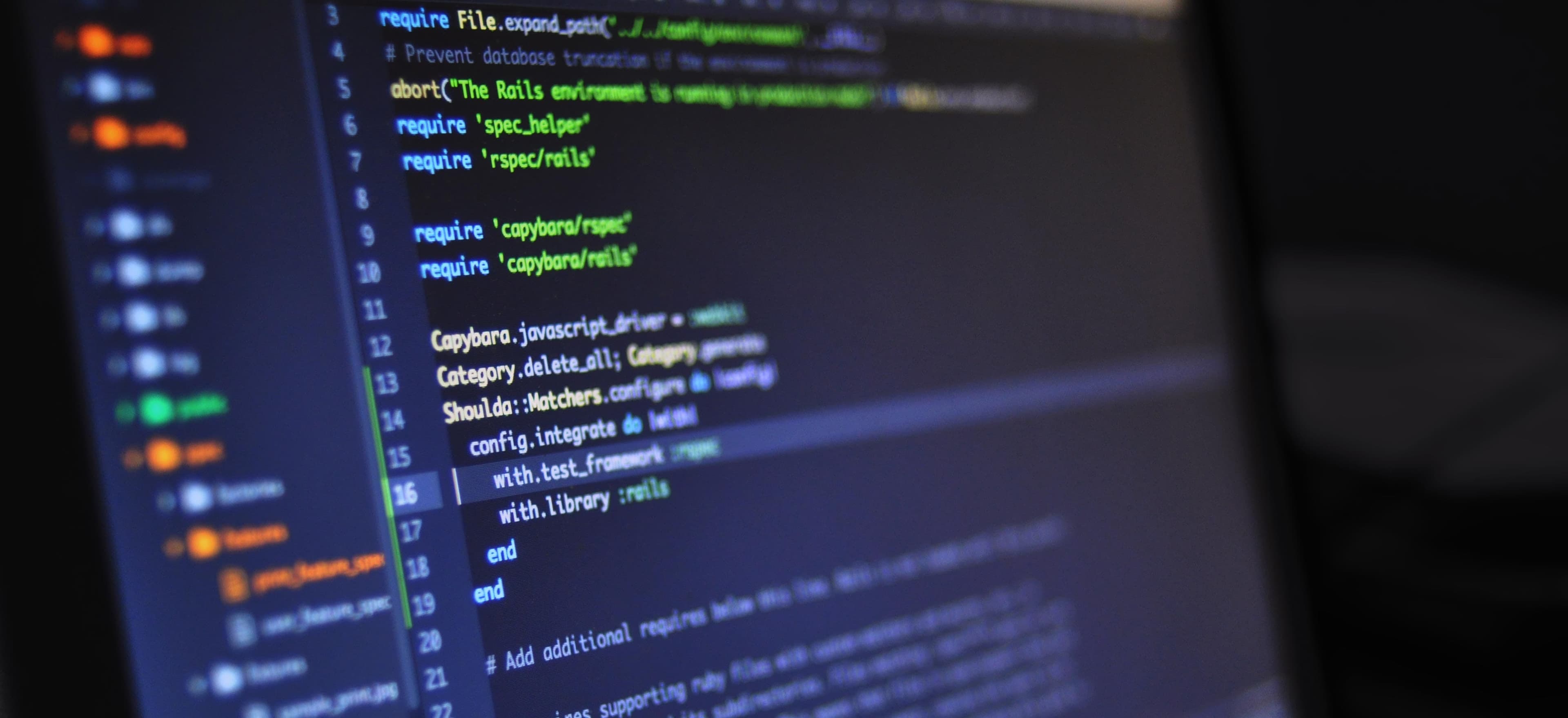
- Published on
Solving Memory Leaks in Spring Boot Applications
Memory leaks can cripple the performance of any application, especially in long-running services like those built using Spring Boot. Identifying and resolving these pesky issues is crucial for maintaining a responsive and stable application. In this blog post, we'll explore what memory leaks are, their causes, how to diagnose them in Spring Boot applications, and provide practical solutions.
What is a Memory Leak?
A memory leak occurs when an application inadvertently retains references to objects that are no longer needed. As a result, the garbage collector cannot free up the memory associated with those objects, leading to increased memory usage over time. Ultimately, this could cause the application to run out of memory, throw OutOfMemoryError
, and crash.
The Impact of Memory Leaks
- Performance Degradation: As memory usage increases, applications can slow down, increasing response times significantly.
- Application Failures: Eventually, if enough memory is consumed, the application may fail entirely, leading to downtime and potential data loss.
- Increased Resource Costs: Running a Java application on cloud infrastructures may incur additional costs due to higher resource consumption.
Common Causes of Memory Leaks in Spring Boot
Understanding common sources of memory leaks is the first step toward preventing them. Here are several prevalent causes:
1. Static References
Static references hold onto objects regardless of their lifecycle, leading to memory leaks.
public class UserService {
private static List<User> users = new ArrayList<>();
public void addUser(User user) {
users.add(user); // User objects never get removed from memory
}
}
Why this matters: Static variables persist for the lifetime of the application. If they hold references to objects, they prevent those objects from being garbage collected even when they are no longer needed.
2. Unreleased Resources
Failing to close connections, streams, and other resources can lead to memory leaks.
import java.sql.Connection;
public void fetchData() {
Connection connection = null;
try {
connection = dataSource.getConnection();
// Perform database operations
} catch (SQLException e) {
e.printStackTrace();
}
// Connection is not closed here
}
Why this matters: Resources, if not closed, keep references to other objects, preventing cleanup.
3. Long-lived Threads
Using long-lived threads for background tasks can lead to memory accumulation, especially if they hold references to application context objects.
public class NotificationTask implements Runnable {
private final ApplicationContext context = ...; // Can lead to memory leaks
@Override
public void run() {
// Do background processing
}
}
Why this matters: If the thread is not released, it can hold onto context or component references, leading to memory retention.
4. Event Listeners
Event listeners that remain registered longer than necessary can keep references alive inadvertently.
@Component
public class MyEventListener {
@EventListener
public void handleEvent(MyEvent event) {
// Logic
}
}
Why this matters: If the event listener is not properly deregistered, it can maintain references to the event source.
Diagnosing Memory Leaks
Identifying memory leaks requires careful observation and analysis. Here are some effective tools and techniques:
Using JVisualVM
JVisualVM is a monitoring tool built into the JDK that helps to visualize memory consumption.
-
Start your Spring Boot application with the following command:
java -jar your-app.jar
-
Run JVisualVM:
jvisualvm
-
Attach to your application and observe the memory usage over time.
Pro Tip: Take heap dumps periodically to analyze memory state and identify objects that are being retained unnecessarily.
Using Heap Dumps
Heap dumps can be triggered manually or automatically.
To manually dump the heap:
jmap -dump:live,format=b,file=heap_dump.hprof <pid>
Analyze the generated file using tools like Eclipse MAT. Identify classes with significant instances that should have been garbage collected.
Fixing Memory Leaks
Now that we understand the causes, it is essential to focus on solutions.
1. Avoid Static References
To mitigate issues from static references, use singleton beans sparingly and clean up after use.
2. Proper Resource Management
Ensure that all resources are properly released. Implement the try-with-resources statement, which automatically closes resources.
try (Connection connection = dataSource.getConnection()) {
// Use connection
} catch (SQLException e) {
e.printStackTrace();
}
Why this matters: This pattern guarantees that resources are closed, reducing the risk of leaks.
3. Use Executors for Background Tasks
Instead of long-lived threads, use ExecutorService
for handling background tasks that can terminate and release resources.
ExecutorService executor = Executors.newSingleThreadExecutor();
executor.submit(() -> {
// Background task
});
executor.shutdown(); // Releases resources
Why this matters: Managing threads helps prevent leaks since they have a shorter lifecycle.
4. Deregister Event Listeners
Ensure that any event listeners are deregistered when they are no longer needed. This might involve removing listeners in your application's shutdown processes.
context.getEventListenerList().removeListener(myEventListener);
Why this matters: This actively releases references, enabling cleanup by the garbage collector.
5. Using Weak References
Using WeakReference
or SoftReference
allows objects to be collected during garbage collection while still enabling a cache-like behavior.
Map<Long, WeakReference<User>> cache = new HashMap<>();
cache.put(userId, new WeakReference<>(user)); // Object can be collected
Why this matters: The garbage collector can reclaim memory for weakly referenced objects, reducing memory pressure.
The Bottom Line
Memory leaks in Spring Boot applications can have debilitating effects. By recognizing common issues, diagnosing effectively, and implementing robust solutions, developers can ensure their applications run smoothly with optimal performance.
Continue exploring:
- Spring Boot Official Documentation
- Java Memory Management
By maintaining good coding practices and an awareness of potential pitfalls, you can mitigate memory leaks and enhance the reliability of your Spring Boot applications. Happy coding!