Common Mistakes When Designing Your Regular API
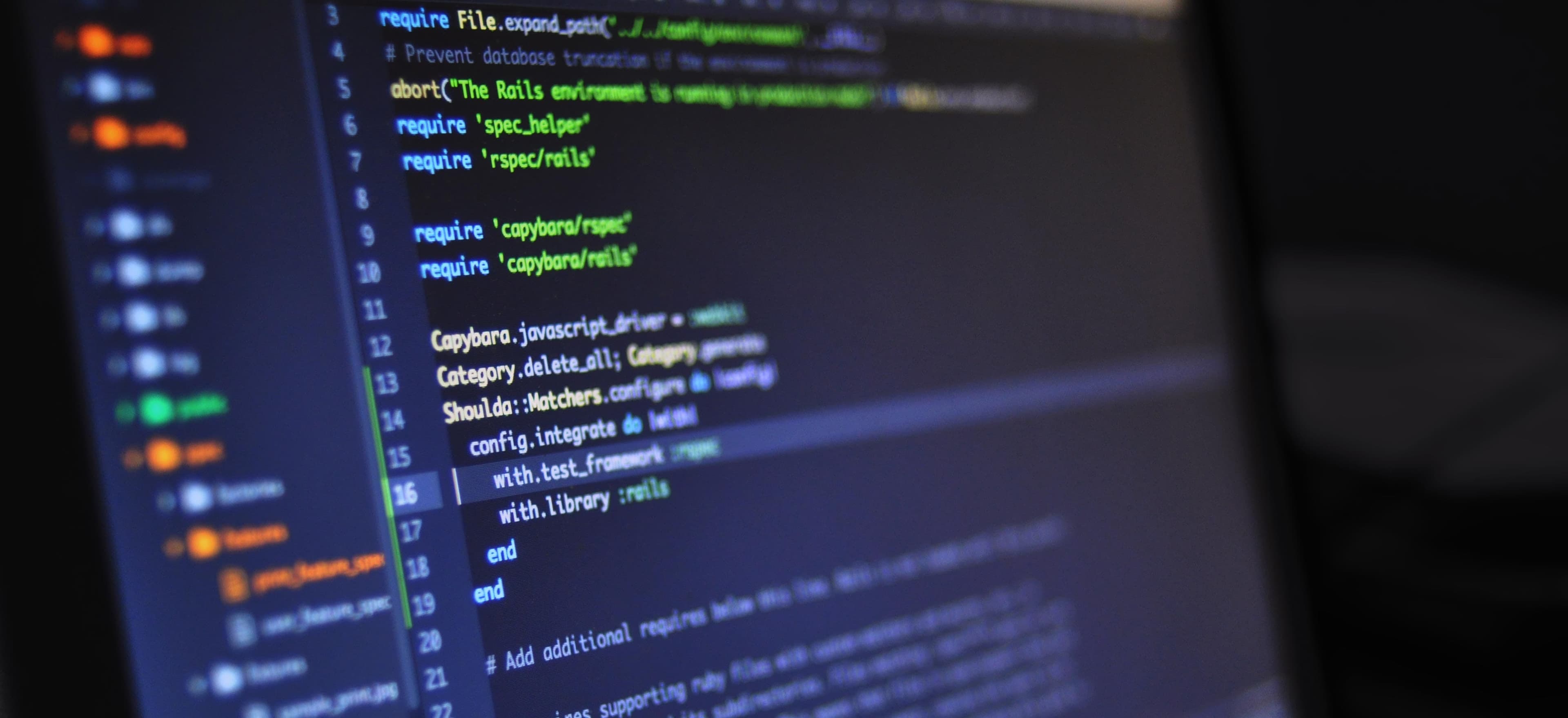
- Published on
Common Mistakes When Designing Your Regular API
Designing a robust API is a crucial step in software development. A well-structured API can significantly enhance the user experience, improve performance, and maintain scalability. However, many developers make common mistakes that can lead to issues down the line. In this blog post, we will delve into the most common errors made when designing regular APIs and provide best practices to avoid them.
1. Ignoring API Versioning
API versioning can be the difference between a smoothly running application and a chaotic one. Ignoring it can lead to broken integrations and unhappy users.
Why Versioning Matters
When you introduce new features, change existing functionality, or, worse, remove features, you must ensure that your API does not break existing clients. Versioning your API allows you to maintain backward compatibility.
Example of Versioning
Here is a simple illustration of versioning:
@GetMapping("/v1/users")
public List<User> getUsersV1() {
// Returns a list of users for version 1
return userService.getUserListV1();
}
@GetMapping("/v2/users")
public List<User> getUsersV2() {
// Returns a list of users with additional info for version 2
return userService.getUserListV2();
}
In this example, different versions of the users endpoint allow consumers to adapt to changes without breaking their existing applications. Read more on API versioning strategies.
2. Lack of Proper Error Handling
Error handling is not merely about returning a status code. It involves providing meaningful feedback.
The Importance of Meaningful Errors
When consumers of your API make a request and encounter an error, they need actionable information to troubleshoot what went wrong. An unclear error message can lead to confusion and wasted time.
Example of Error Handling
Consider this Java controller method:
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable long id) {
Optional<User> user = userService.findUserById(id);
if (user.isPresent()) {
return ResponseEntity.ok(user.get());
} else {
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body("User with ID " + id + " not found.");
}
}
This code not only returns a 404 status code but also provides a meaningful message about the issue. For more information, see Best Practices in API Error Handling.
3. Not Following RESTful Principles
RESTful APIs have specific guidelines that developers often overlook.
The Importance of REST Principles
Not adhering to REST principles can make your API less predictable and less intuitive. Consistency in resource naming, HTTP methods, and status codes is key to a user-friendly API.
Example of RESTful URL Structure
Using descriptive and consistent URLs leads to better understanding:
// Good practice
GET /api/v1/users/123
GET /api/v1/users/123/friends
// Bad practice
GET /api/v1/findFriendForUser?id=123
GET /api/v1/userInfo?uid=123
Using clear, resource-based URLs instead of action-based URLs keeps your API RESTful. For further reading, check out this RESTful API Tutorial.
4. Overcomplicating API Requests
Simplicity is key in API design. Developers sometimes make the mistake of crafting overly complex requests.
How Complexity Hurts Usability
Complexity can make an API difficult to use for developers and could lead to misuse.
Example of Complex Request vs. Simple Request
// Complex: Multiple parameters crammed into one.
POST /api/v1/createUser?name=John&age=29&email=john@example.com
// Simple: JSON Object
POST /api/v1/createUser
{
"name": "John",
"age": 29,
"email": "john@example.com"
}
Using JSON objects for requests keeps the API cleaner and more readable. Check out JSON Best Practices for more information.
5. Not Secure Enough
Security cannot be an afterthought. APIs are frequent targets for attacks.
Security Measures to Implement
After a well-designed API, the next step is ensuring that it remains secure. Common practices include:
- Authentication: Use OAuth2 for secure authorization.
- Data Validation: Prevent SQL injection by validating and sanitizing input data.
- Rate Limiting: Protect your API from abuse by limiting the number of requests per user.
Example Using Spring Security
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest()
.authenticated()
.and()
.oauth2Login(); // Uses OAuth2 for secure login
}
}
By implementing these measures, you protect both your data and your users. For more on API security strategies, refer to OWASP API Security Project.
6. Poor Documentation
API documentation is often neglected. Without clear guidance, developers will struggle to use your API effectively.
The Need for Clear Documentation
Having well-structured documentation can significantly reduce the onboarding time for developers. It should offer clear guidelines, endpoint descriptions, request/response formats, and examples.
Tool for Documentation
Use tools like Swagger or Postman to create interactive documentation. Here is how you can use Swagger annotations:
@RestController
@RequestMapping("/api/v1")
@Api(value="User Management System", description="Operations pertaining to user management")
public class UserController {
@ApiOperation(value = "Get a user by Id")
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable long id) {
//...
}
}
Swagger enables easy understanding of API interactions, making it immensely beneficial for developers. You can find more on Swagger Documentation.
7. Not Allowing for Future Changes
API design is often short-sighted. While current features may function well, the API should be designed with potential growth in mind.
Forward-thinking Design
Designing your API layout with future enhancements can save time and effort. This includes planning for additional fields, new resource types, and scaling options.
Example of Flexible Schema
Instead of using fixed fields, you might consider:
// Flexible data structure
{
"data": {
"name": "John",
"attributes": {
"age": 29,
"email": "john@example.com",
"additionalInfo": { /* future extensible fields */ }
}
}
}
This structure allows the API to grow without hot-fixing its structure. For more on API design principles, read Martin Fowler's API Design.
Closing Remarks
API design is a complex but rewarding process. Avoiding common mistakes such as ignoring versioning, failing to handle errors effectively, neglecting RESTful principles, and overlooking security measures can significantly enhance the quality and usability of your API.
By prioritizing clear documentation and allowing for future changes, you position your API for longevity and user satisfaction. As you embark on your API design journey, remember that a robust API stands not just on code but on thoughtful architecture and user-centric design.
For comprehensive insights into API design practices, consider exploring resources such as API University and various community forums on software architecture.
Happy coding, and may your APIs serve their purpose effectively!
Checkout our other articles