Optimize Your Java REST API: GZIP Compression Issues
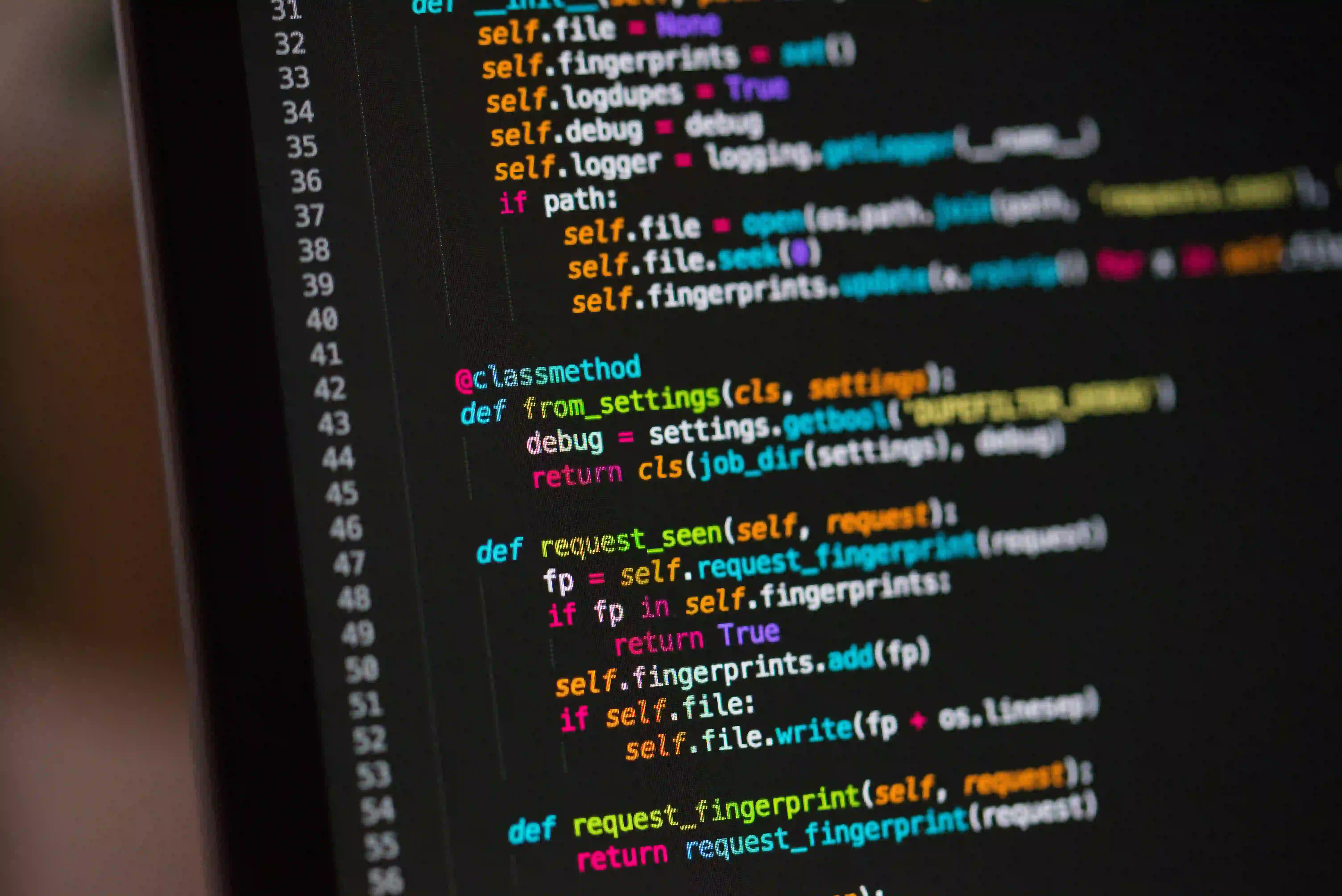
Optimize Your Java REST API: GZIP Compression Issues
In today's digital landscape, performance optimization is crucial for any REST API. GZIP compression is one of the most effective techniques to minimize the size of HTTP responses, thus enhancing the speed and efficiency of data transfer. This article will explore GZIP compression in Java REST APIs, highlight potential issues that can arise, and provide practical code snippets to mitigate these concerns.
Understanding GZIP Compression
GZIP is a compression algorithm that reduces the size of files or data to make transmission over the internet faster. It works by removing redundancies within the data stream. When enabled, a web server compresses data before sending it to a client, and the client decompresses it upon receipt.
For Java REST APIs, enabling GZIP compression can lead to significant performance improvements, especially when dealing with large payloads. According to various studies, GZIP can reduce the size of response bodies by 70-90% in some cases.
Benefits of GZIP Compression
- Reduced Bandwidth Usage: Smaller response sizes lead to lower bandwidth consumption.
- Faster Load Times: Compressed files download faster, improving the overall user experience.
- SEO Benefits: Faster websites contribute to better search engine rankings.
Enabling GZIP Compression in Java
Most frameworks, including Spring Boot and JAX-RS, provide built-in support for GZIP compression. Below are steps to enable GZIP compression in a Spring Boot application, one of the most popular Java frameworks.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ApiApplication {
public static void main(String[] args) {
SpringApplication.run(ApiApplication.class, args);
}
}
Application Properties Configuration
Enable GZIP compression by configuring your application.properties
file:
server.compression.enabled=true
server.compression.mime-types=text/html,text/xml,text/plain,text/css,text/javascript,application/json
server.compression.min-response-size=1024 # in bytes
This configuration tells Spring Boot to enable GZIP compression, specifying the MIME types that should be compressed, along with the minimum response size to apply compression.
Practical Example
Let's consider a simple REST API producing JSON responses. Below is a controller that serves data.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ApiController {
@GetMapping("/data")
public String getData() {
return "{ \"message\": \"Hello, World!\" }"; // A simple JSON response
}
}
The response for this endpoint will be compressed if it exceeds the defined minimum response size.
Common Issues with GZIP Compression
While enabling GZIP compression offers numerous benefits, there are challenges and issues to watch out for:
1. Compression Overhead
The CPU will work harder to compress files, which can introduce latency. This is particularly vital for smaller responses where the compression overhead may outweigh the benefits.
2. Browser Compatibility
Most modern browsers support GZIP, but some older or niche users might not. Although rare, it's good practice to ensure your API can handle such cases gracefully.
3. Security Concerns
Be cautious as GZIP can inadvertently expose sensitive data. An attacker might exploit flaws related to decompression. Be sure to validate and sanitize inputs properly.
4. Nested Resources
If your API retrieves nested resources, you'll need to consider how GZIP handles these larger payloads. Compression might not apply uniformly across nested responses.
Debugging GZIP Compression Issues
When issues arise, debugging is key. Use these techniques to identify and resolve GZIP compression problems.
Use Command-Line Tools
To check if your API responses are compressed, you can use tools like curl
with the --compressed
option:
curl -H "Accept-Encoding: gzip" --compressed http://localhost:8080/data
If the response is GZIP-compressed, you will notice reduced payload size.
Reviewing Response Headers
Inspect HTTP headers to ensure responses are being compressed. Look for the Content-Encoding
header in your API responses:
Content-Encoding: gzip
Code Snippet: Handling Compression Manually
In some cases, you may want to handle GZIP compression manually, especially when you have very specific requirements. Below is a Java example that demonstrates how to manually compress a response using GZIP:
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.OutputStream;
import java.util.zip.GZIPOutputStream;
public class GzipResponseWrapper extends HttpServletResponseWrapper {
private HttpServletResponse response;
private GZIPOutputStream gzipOutputStream;
private OutputStream outputStream;
public GzipResponseWrapper(HttpServletResponse response) throws IOException {
super(response);
this.response = response;
this.outputStream = response.getOutputStream();
this.gzipOutputStream = new GZIPOutputStream(outputStream);
response.setHeader("Content-Encoding", "gzip");
}
@Override
public PrintWriter getWriter() throws IOException {
return new PrintWriter(gzipOutputStream);
}
@Override
public void flushBuffer() throws IOException {
gzipOutputStream.finish();
super.flushBuffer();
}
}
Why This Code Matters
- Custom Control: The
GzipResponseWrapper
gives you granular control over your response handling. - Header Management: It manages the
Content-Encoding
header properly, ensuring the client knows the response is compressed.
Use this wrapper in your servlet or filter to ensure GZIP compression is applied as needed.
To Wrap Things Up
Enabling GZIP compression in your Java REST API can significantly enhance performance while reducing bandwidth usage. Despite some common issues like compression overhead, browser compatibility, and security, awareness and proper management can mitigate these risks.
To further enhance your API's performance, consider reviewing Spring's latest documentation or learning more about HTTP/2 and its native support for multiplexing and server push, which can also optimize performance.
By following the guiding principles discussed here, you’ll not only improve load times for your users but also ensure that your REST API meets modern web standards effectively.
Feel free to share this article with your development team or refer back to it when optimizing your Java REST API!