Streamline Your Spring Lookup Method: Common Pitfalls Explained
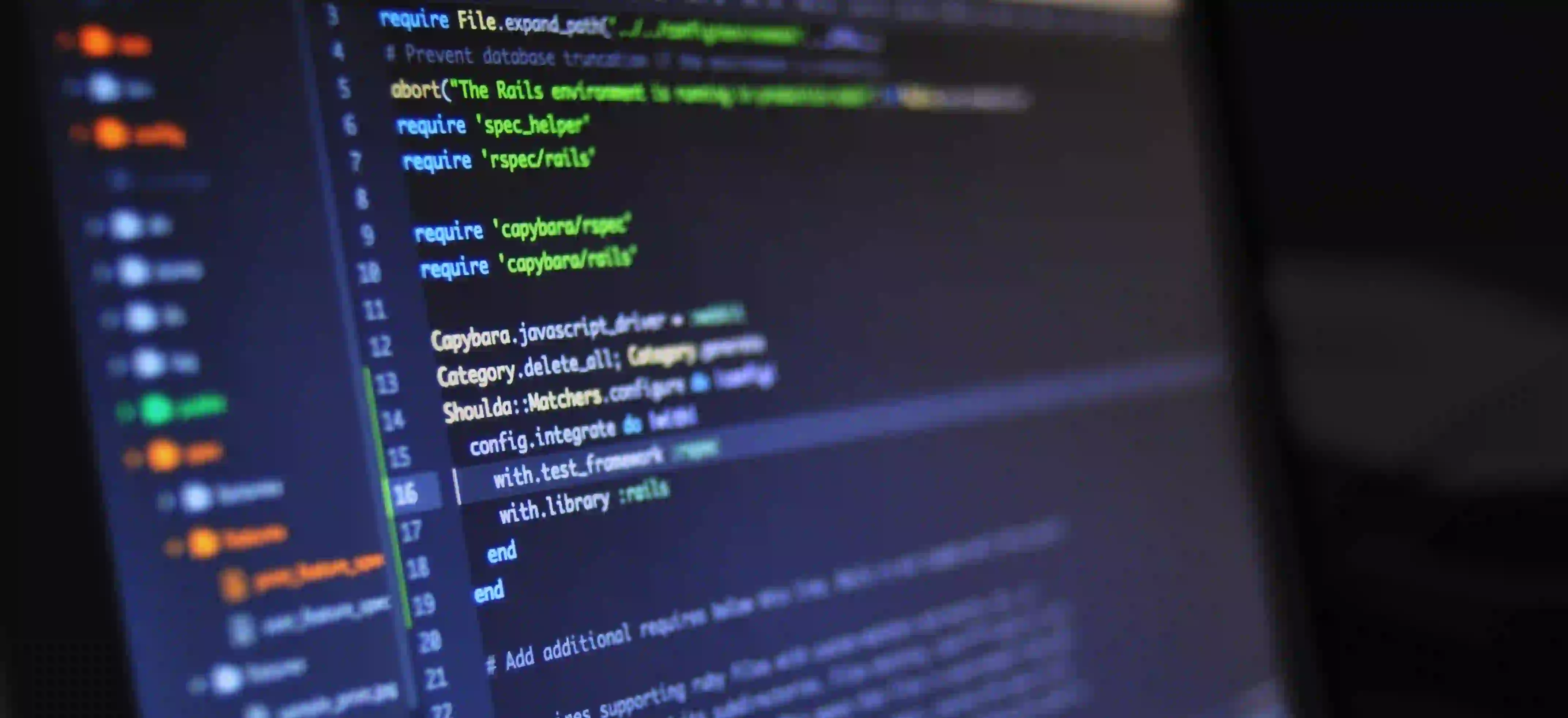
Streamline Your Spring Lookup Method: Common Pitfalls Explained
When working with the Spring Framework, developers often need to create bean instances dynamically at runtime. This is commonly accomplished using the lookup method injection feature. While this approach offers flexibility, there are several common pitfalls that can lead to performance issues or unexpected behaviors. In this blog post, we will explore these pitfalls, explain how to avoid them, and provide code snippets that illustrate each point.
Understanding Lookup Method Injection
Lookup method injection involves defining a method in a Spring bean that indicates a dependency that should be retrieved from the application context at runtime rather than at the time of bean instantiation. This is particularly useful in scenarios where you need to create prototype-scoped beans or when you're dealing with complex object relationships.
To define a lookup method, you can simply annotate a method with @Lookup
. Here’s a simple example:
import org.springframework.beans.factory.annotation.Lookup;
import org.springframework.stereotype.Component;
@Component
public class LookupExample {
@Lookup
public SomePrototypeBean getSomePrototypeBean() {
// The actual method body is not implemented, Spring will override this.
return null;
}
}
In the code above, Spring will automatically override the getSomePrototypeBean
method at runtime. Each call to this method will return a new instance of SomePrototypeBean
. This approach streamlines and simplifies the creation of prototype beans. However, several pitfalls can occur if not handled properly.
Common Pitfalls in Lookup Method Injection
1. Overusing Lookup Methods
One common pitfall is excessive reliance on lookup methods. While they provide convenience, overusing them can lead to scattered code and maintenance difficulties.
Best Practice: Only use lookup methods in scenarios where it is truly necessary. Consider applying design patterns like the Factory or Prototype patterns for better clarity and structure.
2. Not Understanding Bean Scope
Another significant issue arises from misunderstanding the concept of bean scope. By default, Spring beans are singleton-scoped, but lookup methods create new prototype instances. Developers sometimes expect the prototype instance to behave like a singleton, leading to persistent state issues.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Lookup;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("singleton")
public class SingletonBean {
@Lookup
public PrototypeBean getPrototypeBean() {
return null; // Spring provides implementation
}
public void performAction() {
PrototypeBean bean = getPrototypeBean();
// Each call creates a new instance of PrototypeBean
bean.doSomething();
}
}
@Component
@Scope("prototype")
class PrototypeBean {
public void doSomething() {
System.out.println("Doing something");
}
}
In the above example, the SingletonBean
has a method performAction
that retrieves a new PrototypeBean
instance every time it's called, thus avoiding shared mutable state.
3. Using Non-Generic Types
In some instances, developers may try to use raw types instead of generic types in their lookup methods. This may lead to ClassCastExceptions at runtime.
Here's an example to demonstrate this point:
import org.springframework.beans.factory.annotation.Lookup;
import org.springframework.stereotype.Component;
@Component
public class BadLookup {
@Lookup
public List getList() {
return null; // Bad practice. Should be List<SomeType>
}
}
Solution:
Always use generics for better type safety in your code.
4. Lookup Method Caching
Spring does not cache lookup method results – every call instantiates a new prototype bean. This characteristic can be beneficial but also problematic. Depending on your application's logic, repeated calls to a lookup method may lead to performance hits.
If you need to cache certain results, consider implementing caching strategies or using a proper design pattern.
import org.springframework.beans.factory.annotation.Lookup;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Component;
@Component
public class CachingExample {
// This method cannot be cached since it's non-static and is being used for lookup
@Lookup
public PrototypeBean getPrototypeBean() {
return null;
}
@Cacheable("myCache")
public PrototypeBean cacheablePrototypeBean() {
return getPrototypeBean(); // Cache the prototype bean results if this method is called.
}
}
5. Testing Challenges
Testing lookup methods can be tricky due to their dynamic nature. When unit testing, you may want to mock the retrieved prototype beans, which can complicate your test setup.
Example Test Code:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class LookupExampleTest {
@Autowired
private LookupExample lookupExample;
@Test
public void testGetSomePrototypeBean() {
SomePrototypeBean mockBean = mock(SomePrototypeBean.class);
when(lookupExample.getSomePrototypeBean()).thenReturn(mockBean);
SomePrototypeBean retrieved = lookupExample.getSomePrototypeBean();
assertNotNull(retrieved);
}
}
6. Loss of Dependencies
It is essential to ensure that all dependencies for initializations are satisfied. However, in complex setups, a lookup method might fail to provide a required dependency if there are no available beans in the application context.
Strategy: Always verify dependencies in your setup.
Closing Remarks
In conclusion, while Spring Lookup Method Injection can significantly enhance the flexibility and maintainability of your application, rushing into it without understanding its nuances may result in tangible complications. By being aware of the common pitfalls discussed in this blog, you can adopt best practices and avoid unnecessary headaches down the road.
If you want to learn more about Spring and how to enhance your applications, consider exploring the official Spring Framework documentation or taking online courses on platforms like Coursera and Udemy.
Remember, code is not just for machines – it is equally about making our lives easier. Adopt practices that not only lead to cleaner code but also enable easier maintainability and robustness. Happy coding!