Managing Version Fields in DynamoDB with AWS SDK for Java 2
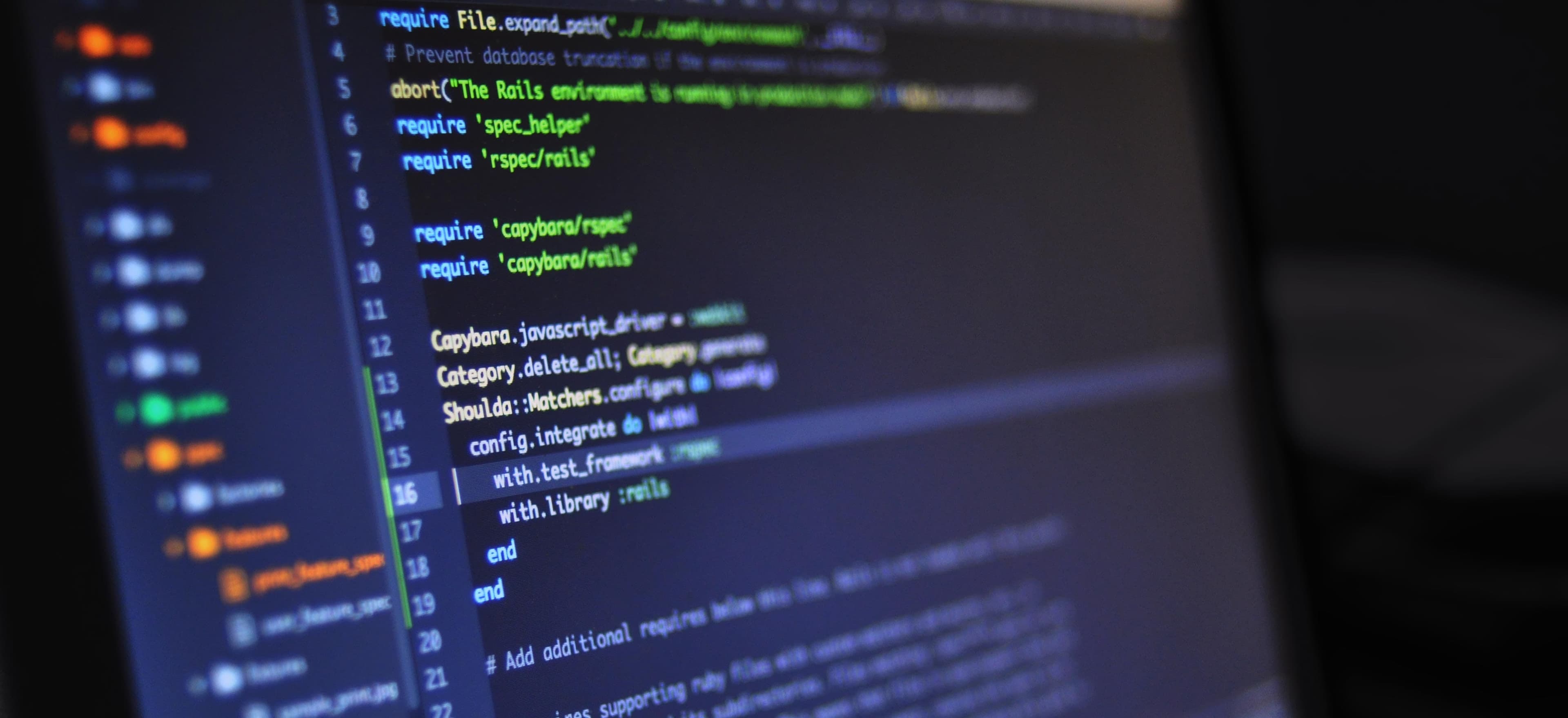
- Published on
Managing Version Fields in DynamoDB with AWS SDK for Java 2
DynamoDB is a fully managed NoSQL database service that provides fast and predictable performance with seamless scalability. One important aspect of data management in DynamoDB is versioning. Implementing version fields allows you to manage and track changes to items effectively. In this blog post, we will explore how to manage version fields in DynamoDB using the AWS SDK for Java 2.
Why Manage Version Fields?
Managing version fields in your DynamoDB tables plays a crucial role in various use cases, such as:
- Optimistic Concurrency Control: Ensure that no overwrites happen without knowledge of a previous state.
- Auditing Changes: Keep track of different versions of an item for rollback or review purposes.
- Data Integrity: Safeguard data against unintended modifications.
Prerequisites
Before we dive into the implementation details, ensure you have:
- An AWS account.
- The AWS SDK for Java 2 installed.
- Basic understanding of Java and DynamoDB.
Setting Up the Project
To start with, create a new Maven project and add the following dependencies in your pom.xml
:
<dependencies>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
<version>2.X.X</version> <!-- Replace with latest version -->
</dependency>
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>auth</artifactId>
<version>2.X.X</version>
</dependency>
</dependencies>
Designing Your DynamoDB Table
Let's assume we need a table to manage user profiles, including a version field. Here’s an example schema:
- Table Name: Users
- Primary Key: UserID (String)
- Attributes:
- Name (String)
- Email (String)
- Version (Number)
Creating the Table
You can create a DynamoDB table using the following code snippet:
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.services.dynamodb.model.*;
public class CreateTable {
public static void main(String[] args) {
DynamoDbClient dynamoDbClient = DynamoDbClient.create();
CreateTableRequest createTableRequest = CreateTableRequest.builder()
.tableName("Users")
.keySchema(KeySchemaElement.builder()
.attributeName("UserID")
.keyType(KeyType.HASH) // Partition key
.build())
.attributeDefinitions(AttributeDefinition.builder()
.attributeName("UserID")
.attributeType(ScalarAttributeType.S)
.build(),
AttributeDefinition.builder()
.attributeName("Version")
.attributeType(ScalarAttributeType.N)
.build())
.provisionedThroughput(ProvisionedThroughput.builder()
.readCapacityUnits(5L)
.writeCapacityUnits(5L)
.build())
.build();
dynamoDbClient.createTable(createTableRequest);
System.out.println("Table created successfully.");
}
}
Commentary:
- We first create a
DynamoDbClient
to interact with DynamoDB. - The
CreateTableRequest
specifies the key schema, including the primary key and attributes. - For
Version
, we are choosing a Number type, since it will hold numeric version identifiers.
Inserting an Item with Versioning
When inserting an item, we must ensure the version field is set correctly. We initialize the version to 1 for new entries.
import software.amazon.awssdk.services.dynamodb.model.*;
public void insertUser(DynamoDbClient dynamoDbClient, String userId, String name, String email) {
Map<String, AttributeValue> item = new HashMap<>();
item.put("UserID", AttributeValue.builder().s(userId).build());
item.put("Name", AttributeValue.builder().s(name).build());
item.put("Email", AttributeValue.builder().s(email).build());
item.put("Version", AttributeValue.builder().n("1").build()); // Initial version
PutItemRequest putItemRequest = PutItemRequest.builder()
.tableName("Users")
.item(item)
.build();
dynamoDbClient.putItem(putItemRequest);
System.out.println("User inserted successfully with initial version.");
}
Commentary:
- An item is constructed using a HashMap.
- The Version is initialized to 1, indicating the first version of the entry.
Updating an Item with Version Control
When updating an existing item, we need to check the current version, increment it, and ensure that no conflicting updates have occurred. This is where version management plays a pivotal role.
public void updateUser(DynamoDbClient dynamoDbClient, String userId, String name, String email, int currentVersion) {
// Try to get the current version from the database
GetItemRequest getItemRequest = GetItemRequest.builder()
.tableName("Users")
.key(Collections.singletonMap("UserID", AttributeValue.builder().s(userId).build()))
.build();
Map<String, AttributeValue> currentItem = dynamoDbClient.getItem(getItemRequest).item();
if (currentItem != null && currentItem.get("Version").n().equals(String.valueOf(currentVersion))) {
// Prepare for update
int newVersion = currentVersion + 1;
Map<String, AttributeValueUpdate> updates = new HashMap<>();
updates.put("Name", AttributeValueUpdate.builder()
.value(AttributeValue.builder().s(name).build())
.action(AttributeAction.PUT)
.build());
updates.put("Email", AttributeValueUpdate.builder()
.value(AttributeValue.builder().s(email).build())
.action(AttributeAction.PUT)
.build());
updates.put("Version", AttributeValueUpdate.builder()
.value(AttributeValue.builder().n(String.valueOf(newVersion)).build())
.action(AttributeAction.PUT)
.build());
UpdateItemRequest updateItemRequest = UpdateItemRequest.builder()
.tableName("Users")
.key(Collections.singletonMap("UserID", AttributeValue.builder().s(userId).build()))
.attributeUpdates(updates)
.build();
dynamoDbClient.updateItem(updateItemRequest);
System.out.println("User updated successfully to version " + newVersion);
} else {
System.out.println("Version conflict detected. Please retry.");
}
}
Commentary:
- A
GetItemRequest
validates the current version against the one provided. - The
UpdateItemRequest
performs the update, incrementing the version appropriately. - This ensures that concurrent modifications do not overwrite changes without awareness.
Deleting an Item
Managing versions also involves providing a mechanism to safely delete items. Once an item is deleted, it cannot be recovered directly. Implementing a soft delete mechanism where you mark an item as deleted can be beneficial.
public void deleteUser(DynamoDbClient dynamoDbClient, String userId) {
// Soft delete: update a deleted flag or similar
Map<String, AttributeValueUpdate> updates = new HashMap<>();
updates.put("Deleted", AttributeValueUpdate.builder()
.value(AttributeValue.builder().bool(true).build())
.action(AttributeAction.PUT)
.build());
UpdateItemRequest deleteItemRequest = UpdateItemRequest.builder()
.tableName("Users")
.key(Collections.singletonMap("UserID", AttributeValue.builder().s(userId).build()))
.attributeUpdates(updates)
.build();
dynamoDbClient.updateItem(deleteItemRequest);
System.out.println("User marked as deleted.");
}
Commentary:
- In this code snippet, we use an "isDeleted" flag implemented via a soft delete approach.
- To achieve actual deletion, you can also implement hard delete using the
DeleteItemRequest
.
The Closing Argument
Implementing version fields in DynamoDB with the AWS SDK for Java 2 empowers you with a robust method for managing concurrency and maintaining data integrity. By effectively utilizing version control, you can prevent data loss, facilitate rollback, and maintain a verifiable history of changes.
For further reading on managing databases with AWS SDK, check out the AWS SDK for Java documentation and DynamoDB documentation.
Embark on your journey of data management with DynamoDB and AWS SDK for Java, and ensure that your applications are resilient against data conflicts and modifications. Happy coding!
Checkout our other articles