How to Handle Global Errors in Spring MVC Integration Tests
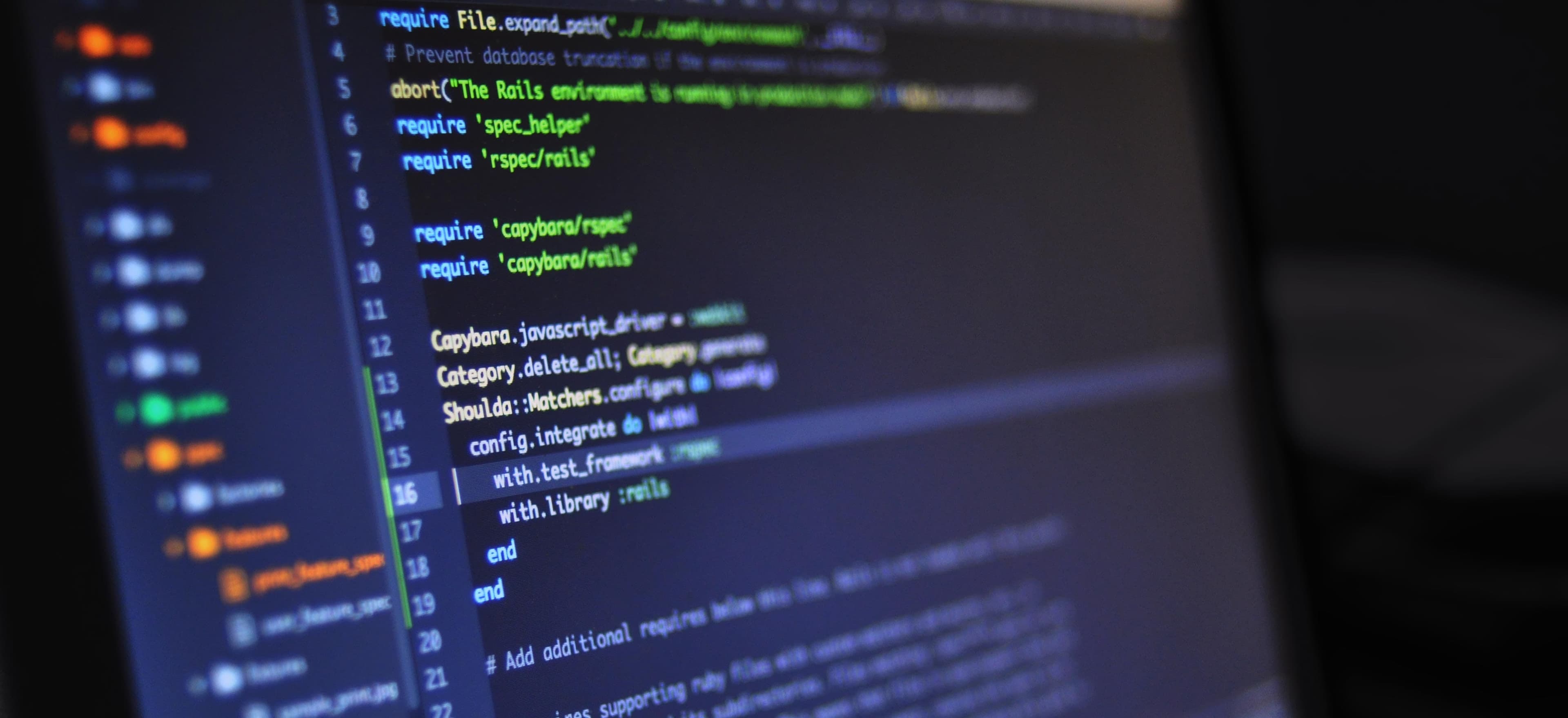
- Published on
How to Handle Global Errors in Spring MVC Integration Tests
Spring MVC is a powerful framework that simplifies the development of web applications in Java. One crucial aspect of building resilient applications is the ability to handle errors effectively, especially during integration testing. In this blog post, we will explore how to handle global errors in Spring MVC integration tests, ensuring our application behaves predictably and gracefully handles exceptional situations.
Understanding Spring MVC Error Handling
Error handling in a Spring MVC application can typically be managed in a few key areas:
- Controller-level error handling: You can annotate methods with
@ExceptionHandler
to define how to handle specific exceptions at the controller level. - Global error handling: Utilizing
@ControllerAdvice
, Spring can provide a centralized approach to exception handling across different controllers. - Error view resolution: Customize the rendered views or responses when an error occurs.
With the focus on integration tests, we will cover the global error handling mechanism using @ControllerAdvice
and ensure proper assertions in our tests.
Setting Up the Project
Before diving into code, ensure you have a Spring Boot project set up. If you haven't already, you can create a simple Spring Boot application by following the instructions on the official Spring Boot website.
Add the required dependencies in your pom.xml
for Spring Web and Spring Test:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
Implementing Global Error Handling
To handle exceptions globally, you can use the @ControllerAdvice
annotation to create an error handler class.
Example Global Error Handler
Here's a simple implementation of a GlobalExceptionHandler
to catch all exceptions globally:
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGlobalException(Exception ex) {
// Log the exception (omitted for brevity)
return new ResponseEntity<>("An unexpected error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why Use Global Exception Handling?
- Centralized Control: It simplifies maintaining and updating error responses.
- Consistency: Every controller in the application responds uniformly to errors.
- Separation of Concerns: Keeps error handling logic separate from business logic in controllers.
Writing Integration Tests
Now that we have our global error handler, the next step is to write integration tests to verify that our error handling mechanism works correctly. We will use Spring MockMvc to simulate requests and validate the response.
Example Controller
Let’s first create a simple controller that may throw exceptions:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SampleController {
@GetMapping("/error")
public void triggerError() {
throw new RuntimeException("This is a runtime exception");
}
}
Creating Integration Tests
Now we can write integration tests to ensure that our error handler catches the exception thrown by the controller.
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@SpringBootTest
@AutoConfigureMockMvc
public class GlobalErrorHandlerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGlobalErrorHandling() throws Exception {
mockMvc.perform(get("/error")
.accept(MediaType.APPLICATION_JSON))
.andExpect(status().isInternalServerError())
.andExpect(content().string(org.hamcrest.Matchers.containsString("An unexpected error occurred: This is a runtime exception")));
}
}
Explanation of Integration Test
In the above test case:
- Setting Up MockMvc: The
@Autowired
annotation injects theMockMvc
instance, which is our main entry point to the testing of MVC controllers. - Testing the Error Endpoint: The
perform(get("/error"))
call simulates a GET request to the/error
endpoint, which, as designed, throws aRuntimeException
. - Assertions: We validate that the HTTP status is
500 INTERNAL SERVER ERROR
and that the response body contains the expected error message.
Further Considerations for Error Handling
While the basic implementation works well, consider these enhancements:
- Custom Exception Classes: Create your own exceptions like
ResourceNotFoundException
and handle them specifically within the global error handler. - Different Response Types: Depending on the request type (e.g., JSON or HTML), you may want different response formats.
- Logging: Use a Logger (e.g., SLF4J) to log errors for tracking and monitoring.
Custom Exception Example
Here’s an example of creating a custom exception and handling it:
public class CustomNotFoundException extends RuntimeException {
public CustomNotFoundException(String message) {
super(message);
}
}
And update the GlobalExceptionHandler
to handle CustomNotFoundException
:
@ExceptionHandler(CustomNotFoundException.class)
public ResponseEntity<String> handleNotFoundException(CustomNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
A Final Look
Handling global errors effectively in Spring MVC is essential for providing a good user experience and maintaining a clean architecture. By leveraging Spring's @ControllerAdvice
, you can centralize your error handling logic, making your application more robust and easier to maintain.
We emphasized writing integration tests with Spring MockMvc to ensure our application behaves as expected under error conditions. Always remember, effective error handling tools are essential in building resilient applications, and with Spring MVC, doing it is straightforward and powerful.
For more on testing in Spring, refer to the Spring Testing Documentation.
Happy coding!