Debugging Common Issues with Spring Boot Actuator
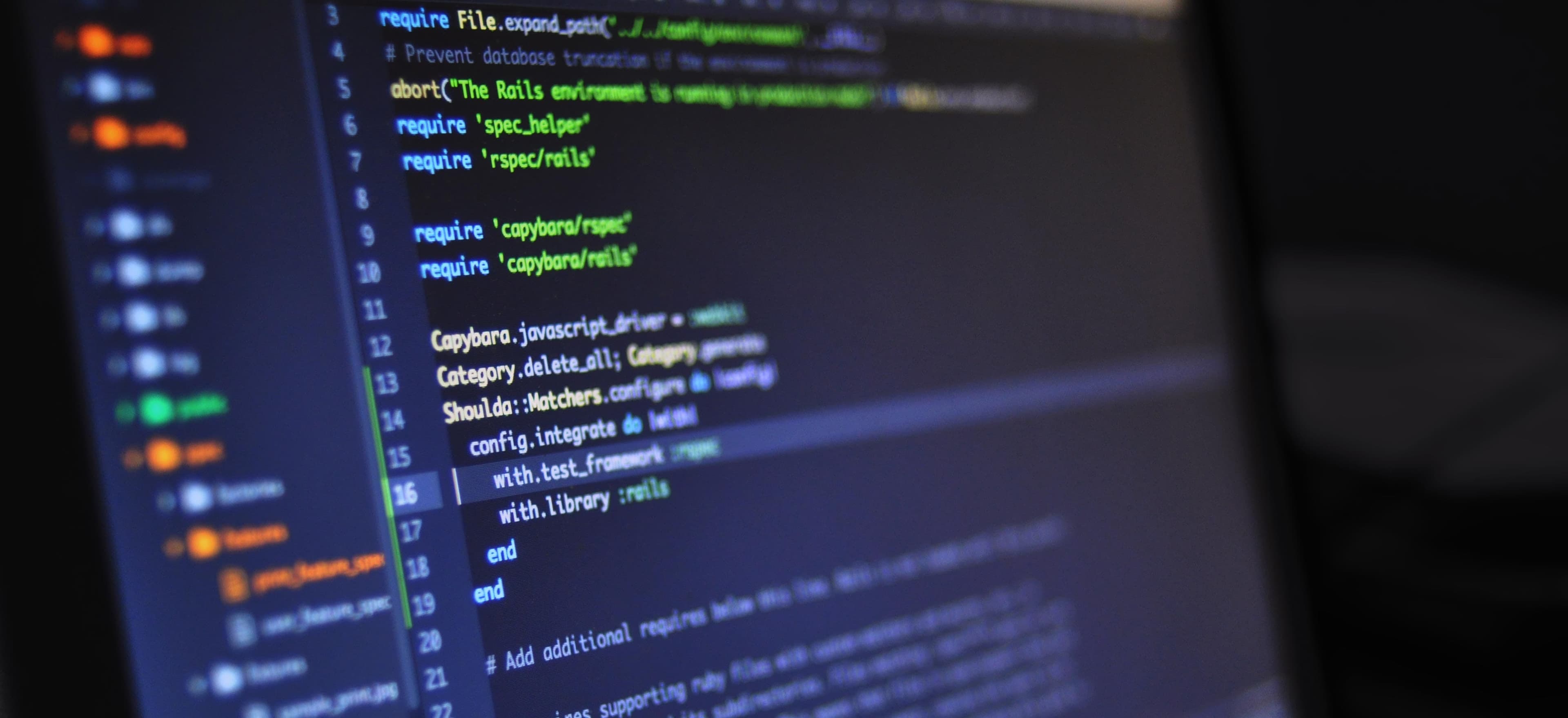
- Published on
Debugging Common Issues with Spring Boot Actuator
Spring Boot Actuator is a powerful tool designed to monitor and manage Spring Boot applications. It provides endpoints that reveal details about your application's health, metrics, environment properties, and much more. However, like any tool, it can run into common issues that might be perplexing for developers. In this post, we will explore debugging these common issues with Spring Boot Actuator, offering solutions that empower you to get the most out of your applications.
Table of Contents
- What is Spring Boot Actuator?
- Common Issues with Spring Boot Actuator
- 2.1. Endpoint Security Issues
- 2.2. Application Health Check Failures
- 2.3. Metrics Not Being Collected
- 2.4. Missing Endpoints
- Best Practices for Using Actuator
- Conclusion
What is Spring Boot Actuator?
Spring Boot Actuator is an extension of Spring Boot that provides built-in endpoints for monitoring and managing your application. These endpoints can expose internal information about the application and diagnose common problems.
With Actuator, you get insights such as:
- Health checks (are your dependencies up and running?)
- Metrics (how many requests were served?)
- Environment properties (what configuration parameters are being used?)
By default, many endpoints are disabled for security reasons. Therefore, understanding how to enable and use Actuator endpoints is essential for any developer.
Common Issues with Spring Boot Actuator
Here, we will discuss the common issues you may encounter while using Spring Boot Actuator.
2.1. Endpoint Security Issues
One of the most common issues developers face is related to security. By default, many Actuator endpoints are secured, meaning they require authentication for access. If you find that your endpoints are returning 403 Forbidden errors, here is a path to resolution:
Solution
You need to configure the security settings in your application.properties
(or application.yml
). Here is how to allow access to all Actuator endpoints without any security for development purposes.
management.endpoints.web.exposure.include=*
management.endpoint.health.show-details=always
spring.security.user.name=admin
spring.security.user.password=password
This configuration opens all Actuator endpoints and allows you to view complete health details. Please remember to avoid using this on production environments.
2.2. Application Health Check Failures
When the health endpoint is hit (/actuator/health), you may encounter an unexpected status, such as 'DOWN'. There could be several causes for this, including database connectivity issues or service dependencies not starting correctly.
Solution
To troubleshoot health check failures, first, view the logs to identify the exact problem. You can configure more details in your application properties:
management.endpoint.health.show-details=always
management.endpoint.health.show-components=always
This configuration will enhance the output you receive from the health endpoint, allowing you to debug effectively. Below is an illustrative example of what you might see:
{
"status": "DOWN",
"components": {
"diskSpace": {
"status": "UP",
"details": {
"total": 499763080192,
"free": 257261250560,
"threshold": 10485760
}
},
"db": {
"status": "DOWN",
"details": {
"error": "Database connection failed"
}
}
}
}
With this detailed information, you can see that the database component is causing the application health to be reported as DOWN.
2.3. Metrics Not Being Collected
Sometimes, you may notice that metrics are not being published or collected as expected. This can occur if the metrics endpoint is not enabled or if there are issues with the configuration.
Solution
To ensure that metrics are collected correctly, check your application properties:
management.metrics.enable.auto= true
management.endpoints.web.exposure.include=metrics
Metrics can be accessed via the endpoint /actuator/metrics
. If metrics still seem absent, verify the dependencies in your pom.xml
or build.gradle
for libraries that register metrics. For instance, including Micrometer can greatly enhance your application's ability to gather metrics:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
</dependency>
2.4. Missing Endpoints
Another issue you may encounter is the absence of expected Actuator endpoints. If your application confronts an issue where some endpoints do not appear in the response from /actuator
, it may mostly relate to configuration problems.
Solution
Check your configuration settings to ensure those endpoints are exposed correctly. You can specify which endpoints to expose in your application.properties
:
management.endpoints.web.exposure.include=health,info,metrics
For example, if you want to include the info endpoint but are not seeing it, adjust your settings accordingly. After implementing fixes to your configuration, restart your application and check the /actuator
endpoint for the new endpoints.
Best Practices for Using Actuator
-
Secure Your Endpoints: Always secure your Actuator endpoints in a production environment to prevent unauthorized access.
-
Environment-Specific Configurations: Use profiles to manage separate configurations for different environments (dev, test, prod).
-
Monitor Performance: Integrate Actuator metrics into your application performance monitoring tools, such as Prometheus or Grafana.
-
Use Private Health Checks: For custom health checks, implement health indicators to encapsulate business logic, ensuring accurate health reporting.
-
Documentation: Regularly refer to the Spring Boot Actuator documentation for updates and new features.
Lessons Learned
Debugging issues related to Spring Boot Actuator may at first seem daunting, but by understanding common pitfalls and their solutions, you can significantly ease the process. The key to effective debugging lies in understanding your environment, customizing configuration appropriately, and utilizing the tools available to create a transparent window into your application's health.
By integrating Actuator correctly, maintaining good security practices, and leveraging its rich set of endpoints, you can ensure that your Spring Boot applications are resilient and well-managed. As you continue your journey in building and debugging Spring Boot applications, remember that proactive monitoring is crucial to maintain application health and performance.
Feel free to explore additional resources such as this guide to Spring Boot Actuator features for further insights into best practices and advanced configurations. Happy coding!