Struggling with Monads? Simplifying with Scoped Continuations
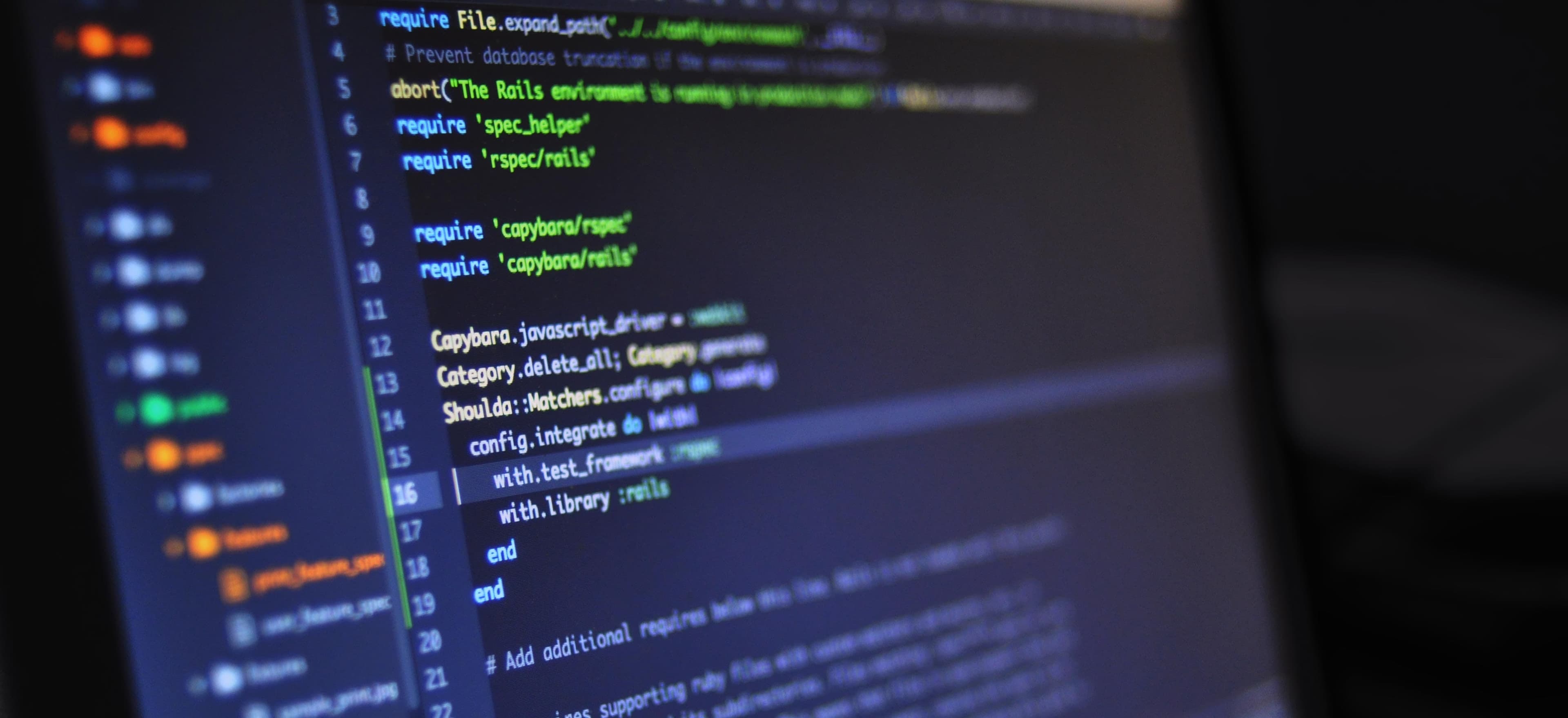
- Published on
Struggling with Monads? Simplifying with Scoped Continuations
Monads are a powerful concept in functional programming, allowing for things like handling state, errors, and side effects. However, many developers find themselves bewildered when trying to grasp how to effectively implement and use them. If you are among the puzzled, fear not—this article intends to illuminate the complexities of monads and offer a simplification through the concept of scoped continuations.
What are Monads?
First, let's define what a monad is. In programming, particularly in languages like Haskell, a monad can be seen as a design pattern used to handle program-wide concerns such as side effects. Essentially, a monad is a type class defined by three components:
- Type Constructor: This takes a type and wraps it into a monadic context.
- Unit Function (or Return): This function takes a value and embeds it in a monad.
- Bind Function: This is typically represented as the
>>=
operator, which is used to chain operations within the monad.
In Java, while we don't have native support for monads, we can achieve similar patterns using functional interfaces and lambda expressions introduced in Java 8.
A Simple Monad Example in Java
import java.util.function.Function;
// Define a simple monad class
public class Box<T> {
private T value;
public Box(T value) {
this.value = value;
}
// The 'unit' function
public static <U> Box<U> of(U value) {
return new Box<>(value);
}
// The 'bind' function
public <R> Box<R> bind(Function<T, Box<R>> mapper) {
return mapper.apply(value);
}
public T getValue() {
return value;
}
}
Why Use This Monad?
The Box
class encapsulates a value along with methods to create a new Box
and to transform its contents. This pattern neatly illustrates the monad structure, allowing you to chain operations on the value it contains (as seen in the bind
method).
The Complexity of Monads
While the concept of monads is relatively straightforward, their implementation can get convoluted. This complexity often stems from the need to conform to monadic laws:
- Left Identity: Wrapping a value in a monad and then applying a function should yield the same result as applying the function directly.
- Right Identity: If you apply a
return
to a value within a monad and then bind it, you should get that value back. - Associativity: The order in which you apply functions should not matter.
When you become aware of these laws, you may feel even more overwhelmed. That's where scoped continuations come into play.
Understanding Scoped Continuations
Scoped continuations provide a means to control the flow of computation, simplifying certain monadic processes. They allow programmers to express complex flows of control in a clearer manner. By leveraging continuations, we can create more manageable abstractions, which can make implementations of structures similar to monads more understandable.
Continuations can be thought of as first-class functions representing "the rest of the computation". To explore this concept further, let's examine a simple continuation in Java using functional interfaces.
A Simple Continuation Example
import java.util.function.Consumer;
// Define a continuation type
public class Continuation<T> {
private Consumer<T> continuation;
public Continuation(Consumer<T> continuation) {
this.continuation = continuation;
}
// Call the continuation with a value
public void run(T value) {
continuation.accept(value);
}
// Map function for chaining
public <R> Continuation<R> map(Function<T, R> mapper) {
return new Continuation<>(value -> continuation.accept(mapper.apply(value)));
}
}
Why Use Continations?
The Continuation
class allows you to encapsulate and manage control flow without the full complexity of monadic bindings. You can easily map transformations over your value, leading to a more flexible approach to managing state and encapsulating side-effects.
Bridging Monads and Continations
Using scoped continuations, we can effectively simplify the use of monads in Java. Consider how we can utilize the Continuation
to represent a simple asynchronous operation.
Asynchronous Example
public class AsyncExample {
public static void asyncOperation(Continuation<String> continuation) {
// Simulate an asynchronous task
new Thread(() -> {
try {
Thread.sleep(1000); // Simulating delay
continuation.run("Result from async operation");
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
public static void main(String[] args) {
Continuation<String> continuation = new Continuation<>(result -> {
System.out.println("Got result: " + result);
});
asyncOperation(continuation);
System.out.println("Asynchronous operation started...");
}
}
Why This Matters
In this example, the asyncOperation
method accepts a Continuation
. Once the asynchronous operation completes, the continuation is called with the result. The beauty here lies in the clear separation of the asynchronous task and the logic that operates once it concludes, reducing confusion and making the code easier to follow.
In Conclusion, Here is What Matters
Monads can undoubtedly be a source of confusion, particularly for those new to functional programming. However, by simplifying their principles with scoped continuations, developers can manage control flow in a more intuitive way.
By judiciously employing the techniques outlined in this article, Java developers can harness the power of monads without getting lost in their complexities.
If you seek to dive deeper into functional programming and the monadic paradigm, resources like Modern Java in Action and Functional Programming in Java may offer more contextual learning.
Keep experimenting, and you'll soon find that some concepts once considered complex can become second nature with the right approach. Happy coding!