Troubleshooting Spring Boot Service Connection Issues
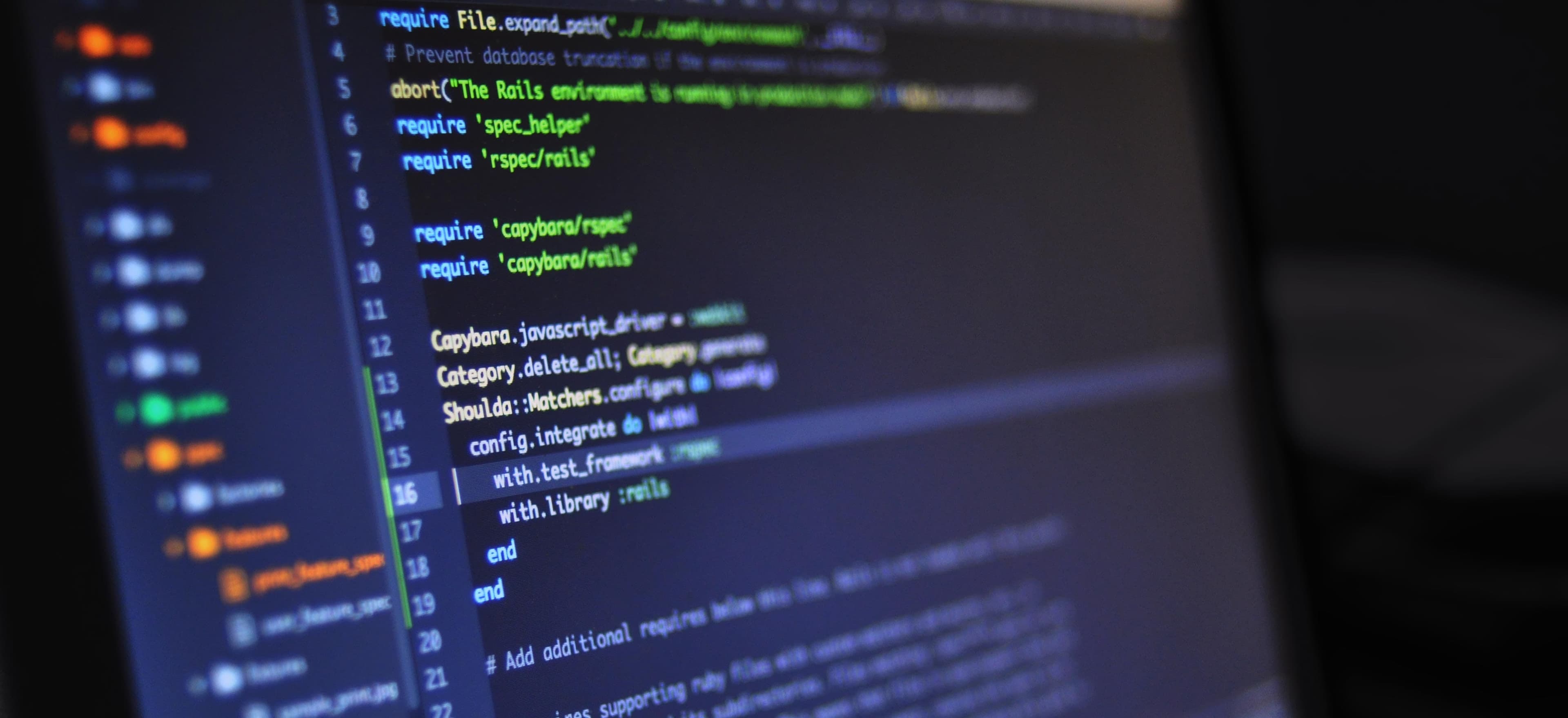
- Published on
Troubleshooting Spring Boot Service Connection Issues
Spring Boot has revolutionized the way Java applications are developed, making it easier to create stand-alone, production-grade Spring applications. However, like any technology, you may encounter connection issues while setting up or running a Spring Boot service. This blog post will cover potential connection problems, their common causes, and strategies to troubleshoot them effectively.
Understanding Service Connections in Spring Boot
Spring Boot services can connect to various components, such as databases, message brokers, and other REST APIs. The connection can fail for numerous reasons, ranging from misconfiguration, network issues, to authentication failures.
Common Causes of Connection Issues
Identifying the cause of connection problems requires a systematic approach. Here are some common sources of issues:
- Wrong Configuration: Misconfigured settings in
application.properties
orapplication.yml
. - Firewall Rules: Network security settings can block necessary ports.
- Database Driver Issues: Missing or incompatible database drivers.
- DNS Resolution Failures: Unable to resolve host names to IP addresses.
- Service Unavailability: The service being connected to is down or unreachable.
Step-by-Step Troubleshooting
Step 1: Check Configuration Settings
The first thing you should do is verify your configuration files. In a Spring Boot application, connectivity settings are usually assigned in the src/main/resources/application.properties
or application.yml
file. Here’s a simple example for a PostgreSQL database:
spring.datasource.url=jdbc:postgresql://localhost:5432/mydb
spring.datasource.username=myuser
spring.datasource.password=mypass
spring.jpa.hibernate.ddl-auto=update
Why is this important? Ensuring that the URL, username, and password are correct is fundamental. If these values don't match your database server settings, you’ll face connection errors.
Step 2: Validate Database Driver Dependency
If you are connecting to a database, ensure that the relevant driver dependency is included in your pom.xml
or build.gradle
file. For instance, for PostgreSQL:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.5</version>
</dependency>
Commentary: Always ensure your driver version is compatible with your database version. Invalid driver versions can lead to connectivity issues.
Step 3: Networking Configuration and Firewall
Ensure that your application has network access to the service. If you are running Spring Boot locally while trying to connect to a remote database or service, check firewall settings:
- Locally: Ensure that the local firewall (like Windows Firewall on Windows or iptables on Linux) allows traffic over the port you're using (such as 5432 for PostgreSQL).
- Remotely: Make sure that the server allows inbound traffic on the specified port.
Why this matters: Without the right firewall configuration, your application will not be able to communicate with the service, leading to "connection refused" errors.
Step 4: Review Service and Server Logs
Logs are your best friends when troubleshooting. Check the following logs:
- Spring Boot application logs
- Database or external service logs
- Network logs
When you run your Spring Boot application, you can access logs in the console, or if you configure logging to a file, you can check the contents there. Look for error messages or exceptions that can give you insights into what's wrong.
Example of a typical error message:
Caused by: org.springframework.transaction.CannotCreateTransactionException: Failed to obtain transaction-scoped EntityManager for transaction
This indicates an issue with obtaining a database connection.
Step 5: Test Network Connectivity
Verify you can reach the target server using command-line tools:
ping your-database-hostname
telnet your-database-hostname 5432
The ping
command will check for network connectivity, while the telnet
command will check if the specific port is open and accessible.
Why should you do this? This helps determine if the problem lies within your network or application configuration.
Step 6: Debugging Code
Sometimes, the issue might arise from the code itself. Debuggers in IDEs can help trace where connectivity fails. It is advisable to log a detailed stack trace using Spring’s logging capabilities.
Here's an example of logging during an attempt to connect to the database:
@Autowired
private DataSource dataSource;
@Override
public void run(String... args) throws Exception {
try (Connection connection = dataSource.getConnection()) {
logger.info("Successfully connected to the database!");
} catch (SQLException e) {
logger.error("Failed to connect to the database", e);
// Additional handling logic
}
}
Commentary: Logging provides clarity and helps understand which part of your code is causing issues.
Step 7: Consult Documentation
Each database and third-party service typically has documentation on common connection issues. For example, the PostgreSQL documentation offers insights on debug connections, which can be helpful.
Step 8: Reach Out for Help
If all else fails, don’t hesitate to ask for help. Forums like Stack Overflow and the Spring Community are great places to seek guidance. Provide clear context, share error messages, and describe what you have already tried.
In Conclusion, Here is What Matters
Troubleshooting connection issues in a Spring Boot application can seem daunting, but by following a systematic approach, you can identify and resolve problems faster. Always ensure your configuration files, network settings, and dependencies are set up correctly.
If you apply the troubleshooting steps outlined in this post, you'll be well on your way to fixing any connection issues that arise and getting your Spring Boot application up and running smoothly.
Remember: logging, documentation, and community support are invaluable resources in your journey of mastering Spring Boot. Happy coding!
Feel free to leave your comments or questions below!