Streamline Validation: Ditch the Excessive If-Else Blocks
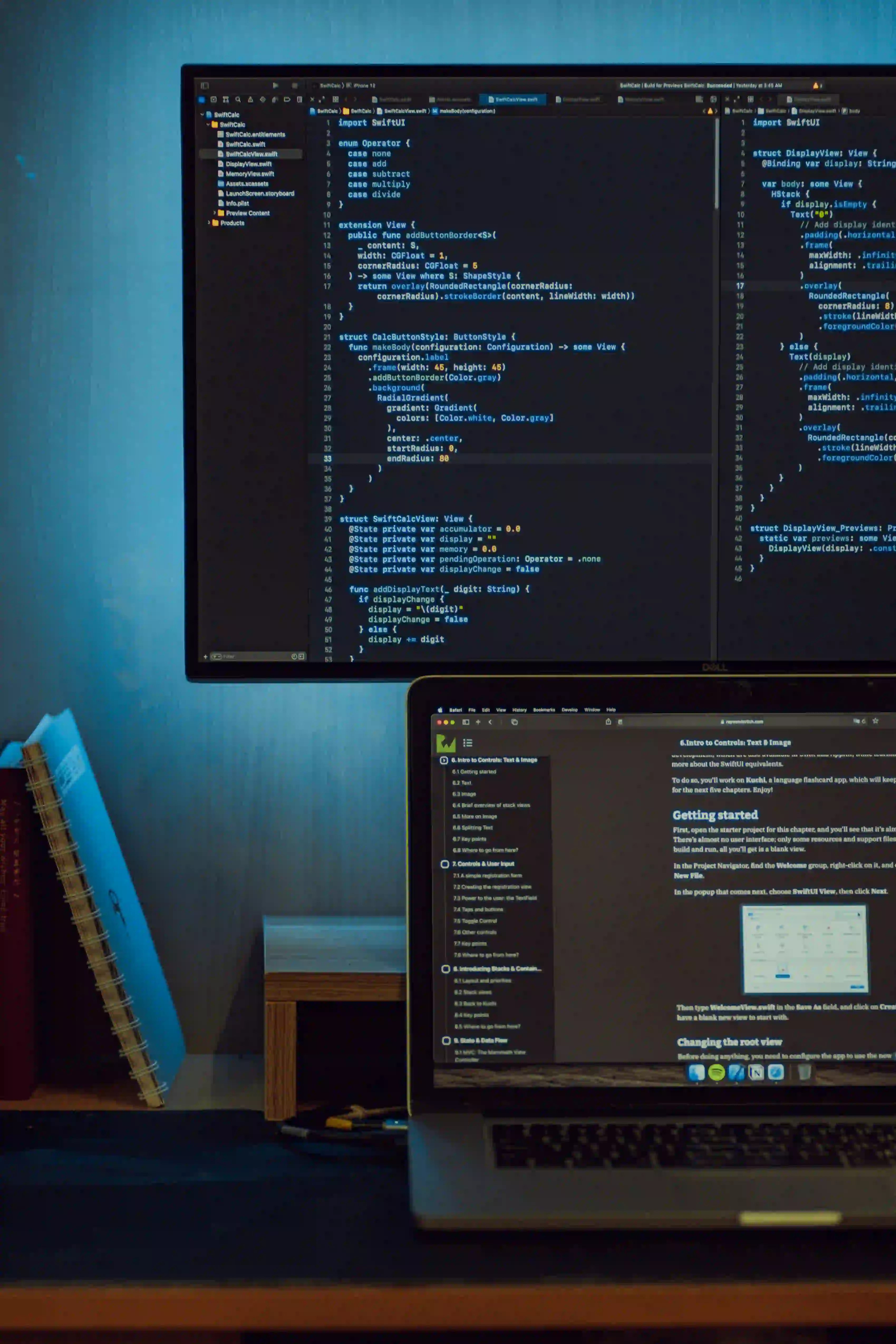
Streamline Validation: Ditch the Excessive If-Else Blocks in Java
Java developers often find themselves knee-deep in intense validation logic. If you are like many of us, you may have relied heavily on if-else blocks to manage complex validation scenarios. While this approach can work for simple checks, using an abundance of if-else statements can lead to code that is hard to read, maintain, and test. In this blog post, we will explore ways to streamline validation in Java, substituting redundant if-else constructs with cleaner, more maintainable solutions.
The Problem with Excessive If-Else Blocks
When writing validation logic, one of the first things developers gravitate toward is the if-else construct. It's familiar and straightforward. However, as validations grow, these blocks start to stack up, leading to the infamous "pyramid of doom" or "arrow anti-pattern". Here’s a simple example:
public String validateInput(String input) {
if (input == null) {
return "Input cannot be null.";
} else if (input.isEmpty()) {
return "Input cannot be empty.";
} else if (input.length() < 5) {
return "Input must be at least 5 characters.";
} else if (!input.matches("[a-zA-Z]+")) {
return "Input must contain only alphabetic characters.";
}
return "Input is valid!";
}
In this example, we start seeing a long chain of conditions that can quickly spiral out of control. It's not only difficult to read, but adding more validations further complicates the logic.
Benefits of Refactoring Validation Logic
Refactoring your validation logic offers several advantages:
- Readability: Code becomes easier to read and understand.
- Maintainability: Adding or changing validation rules will be significantly simpler.
- Testing: Isolated and clear logic can be tested in smaller units without needing to simulate entire condition trees.
- Reusability: Makes it easier to reuse validation logic across different parts of your application.
Let's look at several alternative approaches to improve our validation strategy in Java.
1. Use Guard Clauses
Guard clauses can simplify your validation by returning early if a condition is not met. This avoids the nested logic associated with traditional if-else statements.
public String validateInput(String input) {
if (input == null) {
return "Input cannot be null.";
}
if (input.isEmpty()) {
return "Input cannot be empty.";
}
if (input.length() < 5) {
return "Input must be at least 5 characters.";
}
if (!input.matches("[a-zA-Z]+")) {
return "Input must contain only alphabetic characters.";
}
return "Input is valid!";
}
2. Leverage Java 8 Optional
With the introduction of Java 8, the Optional
class can provide an elegant solution for handling cases where values may or may not be present.
Here is a revised version of your validation logic that uses Optional
:
import java.util.Optional;
public String validateInput(Optional<String> input) {
return input.map(value -> {
if (value.isEmpty()) {
return "Input cannot be empty.";
}
if (value.length() < 5) {
return "Input must be at least 5 characters.";
}
if (!value.matches("[a-zA-Z]+")) {
return "Input must contain only alphabetic characters.";
}
return "Input is valid!";
}).orElse("Input cannot be null.");
}
In this example, we handle null values gracefully using Optional
, which can help avoid NullPointerExceptions
.
3. Create Validation Rules as Separate Methods
Consider creating individual methods for each validation rule and returning an error message if a validation fails. This simplifies your main validation method:
public String validateInput(String input) {
if (input == null) {
return "Input cannot be null.";
}
return validateNotEmpty(input)
.or(() -> validateMinLength(input))
.or(() -> validateAlphabetic(input))
.orElse("Input is valid!");
}
private Optional<String> validateNotEmpty(String input) {
return input.isEmpty() ? Optional.of("Input cannot be empty.") : Optional.empty();
}
private Optional<String> validateMinLength(String input) {
return input.length() < 5 ? Optional.of("Input must be at least 5 characters.") : Optional.empty();
}
private Optional<String> validateAlphabetic(String input) {
return !input.matches("[a-zA-Z]+") ? Optional.of("Input must contain only alphabetic characters.") : Optional.empty();
}
This modular approach not only enhances readability but also allows you to add or remove rules with minimal impact.
4. Use a Validation Framework
For complex use cases, consider employing a validation framework such as Hibernate Validator (part of the Bean Validation specification). This approach centralizes validation logic and employs declarative annotations for rules, leading to clean code.
Example using Hibernate Validator:
import javax.validation.constraints.*;
public class UserInput {
@NotNull(message = "Input cannot be null.")
@NotEmpty(message = "Input cannot be empty.")
@Size(min = 5, message = "Input must be at least 5 characters.")
@Pattern(regexp = "[a-zA-Z]+", message = "Input must contain only alphabetic characters.")
private String input;
// Getters and Setters
}
5. Functional Programming with Streams
Using streams can allow you to handle collection validations fluidly, especially if you need to check multiple inputs:
public List<String> validateInputs(List<String> inputs) {
return inputs.stream()
.map(this::validateInput)
.filter(result -> !result.equals("Input is valid!"))
.collect(Collectors.toList());
}
In this case, validate every input in the list and collect any validation errors in a new list.
Final Considerations
In this post, we explored how to streamline validation in Java by minimizing excessive if-else blocks. From using guard clauses and Java 8's Optional
to leveraging modular methods and even frameworks, the options are plentiful for improving your code.
In a world where clean, maintainable code is essential, adopting these strategies can significantly enhance both the quality of your code and the ease with which you can modify it as your applications evolve.
For more insights on Java best practices, check out Oracle's Official Java Tutorials and further your skills.
Remember, less is more. By reducing complexity in your validation logic, you are paving the way for cleaner, more robust Java applications. Happy coding!