Common Java Mistakes Beginners Make and How to Avoid Them
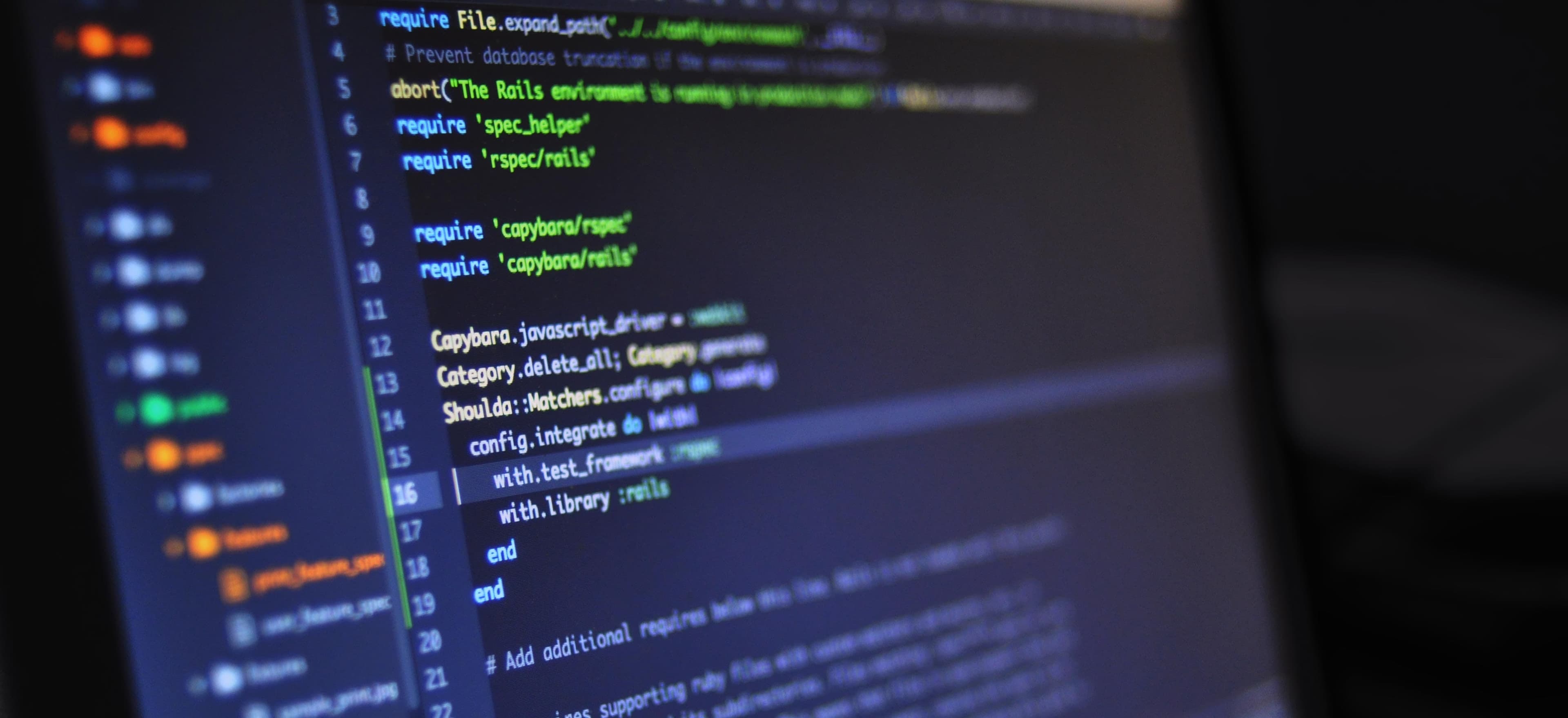
- Published on
Common Java Mistakes Beginners Make and How to Avoid Them
Java is a powerful and versatile programming language that is widely used in enterprise applications, mobile apps, and web development. For beginners, the journey into Java can be both exciting and daunting. Mistakes are a natural part of learning, but understanding common pitfalls can help you become a proficient Java developer more quickly. In this blog post, we will explore some of these common Java mistakes and provide tips on how to avoid them.
1. Ignoring Basic Syntax Rules
One of the simplest yet most pervasive mistakes beginners make is overlooking Java's syntax rules. Java is a case-sensitive language, meaning that variable
, Variable
, and VARIABLE
are treated as three distinct identifiers.
Example:
int number = 10;
int Number = 20;
System.out.println(number); // Outputs: 10
Why This Matters:
Using inconsistent naming conventions can lead to confusion and bugs in your code. Always stick to a naming convention, such as camelCase for variables, and remember to be consistent with your case usage.
Avoiding the Mistake:
- Always double-check your variable and method names.
- Use integrated development environments (IDEs) with syntax highlighting features, like IntelliJ IDEA or Eclipse, to catch errors early.
2. Misunderstanding Data Types
Java is statically typed, which means every variable must have a defined data type. Beginners often mix types, leading to compilation errors or unexpected runtime behavior.
Example:
int age = "25"; // Compilation error
Why This Matters:
Each data type has its own set of properties, memory uses, and operational capabilities. Choosing the wrong type can lead to performance issues and bugs.
Avoiding the Mistake:
- Familiarize yourself with Java's data types, such as
int
,double
,char
, andString
. - Use constructors or methods that correctly convert types when necessary, such as
Integer.parseInt()
for string to integer conversion.
3. Forgetting Semicolons and Braces
Java is particular about ending statements with semicolons and using braces {}
for defining code blocks.
Example:
if (condition) // Missing braces
System.out.println("Hello World") // Missing semicolon
Why This Matters:
Omitting these can cause compilation errors and unexpected behavior in your programs. In the above example, the lack of braces could lead to confusion regarding which statements are conditioned by the if
.
Avoiding the Mistake:
- Get into the habit of adding semicolons at the end of each statement.
- Use braces for all conditional statements and loops, even if they contain a single statement.
4. Misusing the 'Null' Value
Beginners often overlook how Java handles null
values, leading to NullPointerException
, one of the most common exceptions in Java.
Example:
String str = null;
System.out.println(str.length()); // Throws NullPointerException
Why This Matters:
Null checks are essential in order to avoid crashes or unexpected behaviors in your applications.
Avoiding the Mistake:
- Always check if an object is null before accessing its methods or properties.
- Use the Optional class (available since Java 8) to better handle potentially null values.
Optional<String> optionalStr = Optional.ofNullable(str);
optionalStr.ifPresent(s -> System.out.println(s.length()));
5. Not Understanding Object-Oriented Principles
Java is an object-oriented programming (OOP) language. Beginners sometimes struggle to grasp concepts such as inheritance, encapsulation, and polymorphism.
Example:
class Animal {
void makeSound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Bark");
}
}
Why This Matters:
Not leveraging OOP principles can lead to code that is poorly organized and difficult to manage. Understanding these basics is critical for writing scalable applications.
Avoiding the Mistake:
- Study the four main pillars of OOP: abstraction, encapsulation, inheritance, and polymorphism.
- Implement OOP principles in your projects to improve code quality.
6. Excessive Use of Static Variables and Methods
While static variables and methods have their place in Java, beginners often misuse them, thinking they are the only way to manage state and data.
Example:
class Counter {
static int count = 0; // Shared across all instances
public static void increment() {
count++;
}
}
Why This Matters:
Excessive use of static members can lead to unexpected behavior, especially in multi-threaded environments where shared state can be modified concurrently.
Avoiding the Mistake:
- Use static only when necessary, typically for utility classes or methods.
- Manage state through instance variables whenever appropriate.
7. Inefficient Use of Loops and Conditions
Beginners often write redundant loops and conditions, leading to inefficient code that is harder to read and maintain.
Example:
for (int i = 0; i < 10; i++) {
if (i < 5) {
System.out.println(i);
}
}
Why This Matters:
Clear and efficient code is easier to read, maintain, and optimize. Excessive nesting or unnecessary loops can cause performance issues.
Avoiding the Mistake:
- Simplify your loops and conditions by using methods like
Enhanced For Loop
and leveraging Java's Stream API for better readability and performance.
IntStream.range(0, 5).forEach(System.out::println);
My Closing Thoughts on the Matter
Learning Java is a fulfilling endeavor full of challenges and rewards. By recognizing and avoiding these common mistakes, you can gain a solid foundational understanding of the language.
Always remember that coding is as much about logic as it is about structure and style. As you practice, don’t forget to explore resources like the Java Documentation and communities like Stack Overflow for help.
By continuously improving your skills and knowledge, you will not only avoid common pitfalls but also become a more effective and efficient Java programmer. Happy coding!