Debugging JVM: Common Misprints in Array Outputs
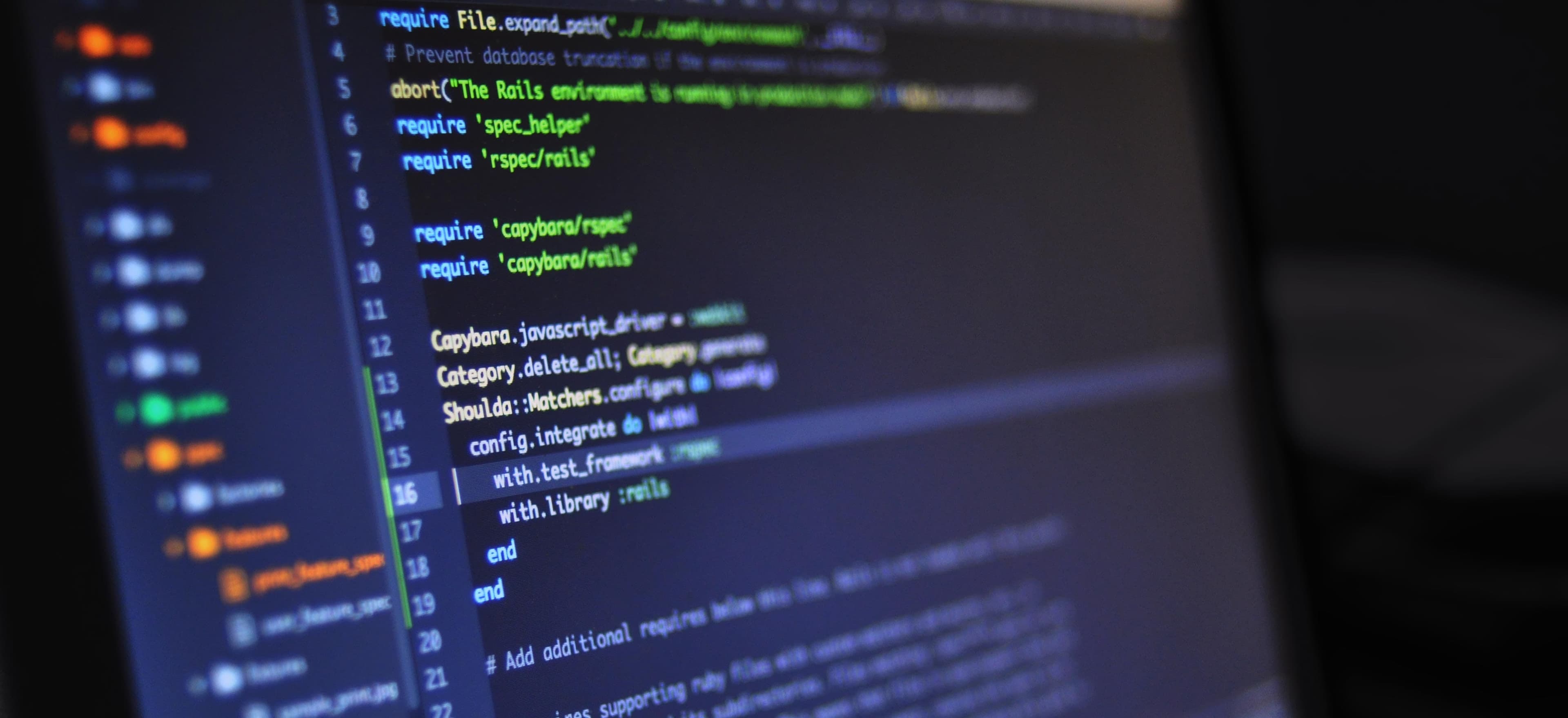
- Published on
Debugging JVM: Common Misprints in Array Outputs
The Java Virtual Machine (JVM) is a powerful component of the Java programming language. It allows developers to run their Java programs on any device with the appropriate JVM installed. However, when dealing with arrays in Java, misunderstandings and misprints can occur that may lead to bugs or unexpected behaviors. In this blog post, we will discuss some common issues related to array outputs in Java, explore the reasons behind them, and provide solutions to effectively debug these problems.
Understanding Java Arrays
Before diving into common misprints, it is essential to understand how arrays work in Java. An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created and cannot be changed dynamically.
Example of Array Initialization
int[] numbers = new int[5]; // Creating an array of integers with 5 elements
While you can initialize an array with different values directly, remember that accessing out-of-bounds indices may lead to an ArrayIndexOutOfBoundsException
.
Example of Array Initialization with Values
int[] numbers = {1, 2, 3, 4, 5}; // Initializing an array with values
Common Misprints and Debugging Techniques
Despite its straightforwardness, working with arrays can lead to unexpected output. Below are some common misprints along with debugging techniques.
1. Inaccurate Array Length
A frequent error occurs when developers miscalculate the length of an array. The length property of an array in Java can be accessed using arrayName.length
. Misprints arise when developers mistakenly use arrayName.length()
(which is a method call, leading to a compiler error) or misinterpret the length value.
String[] fruits = {"Apple", "Banana", "Cherry"};
System.out.println("Length of fruits array: " + fruits.length); //Correct
// System.out.println("Length of fruits array: " + fruits.length()); //Incorrect
Debugging Technique
To avoid confusion, always ensure you are familiar with how to access array properties and methods. Implement defensive coding practices, such as ensuring you check for null or empty arrays before accessing their length.
2. Printing Array Contents Incorrectly
When printing an array directly using System.out.println()
, it will not output the contents as expected. Instead, it will print the type and hash code of the array.
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers); // Will print something like [I@15db9742
Example of Correctly Printing Array Contents
To print the contents of an array, you can either loop through the elements or use Arrays.toString()
.
import java.util.Arrays;
int[] numbers = {1, 2, 3, 4, 5};
// Correctly printing array contents
System.out.println(Arrays.toString(numbers)); // Outputs: [1, 2, 3, 4, 5]
Debugging Technique
Make use of java.util.Arrays
which provides utility methods for array manipulation and diagnostics. Always remember to format your output for better readability.
3. Misusing Arrays in Loops
When iterating through an array using loops, forgetting to adjust the loop condition can produce unwanted results. A common pitfall is using the length of the array incorrectly.
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i <= numbers.length; i++) {
System.out.println(numbers[i]); // Will throw ArrayIndexOutOfBoundsException at the last iteration
}
Correct Loop Initialization
The condition should be i < numbers.length
instead of i <= numbers.length
.
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]); // Correctly prints each number
}
Debugging Technique
Always use a zero-based index and make sure your loop boundaries do not exceed your array's bounds. For more complex data structures, consider using enhanced for-loops for simplicity.
4. Mutability Misunderstandings: Reference Types
In Java, arrays are reference types. This means if you assign an array to another array variable, both variables will reference the same array object.
int[] original = {1, 2, 3};
int[] copy = original;
copy[0] = 99;
System.out.println(original[0]); // Outputs: 99 due to reference linking
Debugging Technique
To create a true copy of an array, you can use the Arrays.copyOf()
method.
int[] copy = Arrays.copyOf(original, original.length);
copy[0] = 99;
System.out.println(original[0]); // Outputs: 1
Further Resources
For more in-depth understanding, you can refer to the Java Documentation for the Arrays utility class.
Final Thoughts
Debugging array outputs in Java can be straightforward when developers are aware of common pitfalls. By carefully managing array lengths, utilizing proper printing techniques, correctly iterating through arrays, and understanding referential behavior, programmers can eliminate many issues that may arise.
Take these debugging principles into practice as you work with arrays in your applications. The JVM is a robust machine, and with proper debugging and coding practices, you can fully harness its power in your Java development journey.
Implementing efficient debugging techniques not only saves time but also enhances the performance of your code. Happy coding!
References
Feel free to reach out if you have any additional thoughts or questions regarding debugging arrays in Java!
Checkout our other articles