Common Pitfalls in Software Architecture Planning
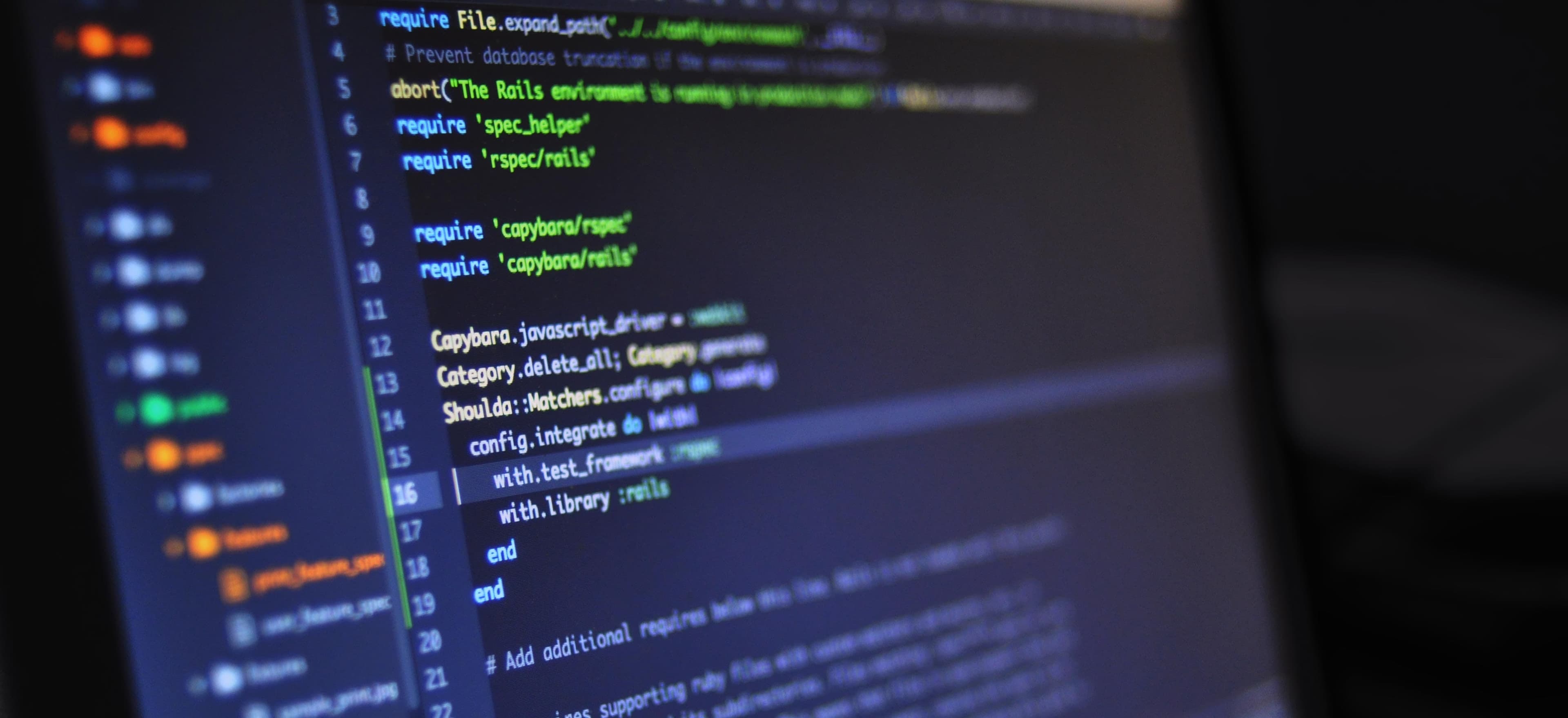
- Published on
Common Pitfalls in Software Architecture Planning
Software architecture is a foundational aspect of any successful software development project. It sets the groundwork for how the software will function, scale, and evolve over time. However, navigating this landscape is fraught with potential pitfalls.
In this blog post, we'll explore some of the most common mistakes made during software architecture planning and how to avoid them.
1. Underestimating Non-Functional Requirements
The Importance of Quality Attributes
Non-functional requirements, often referred to as quality attributes, define how a system performs its tasks. These include performance, security, scalability, and usability. Too often, they are overlooked during the architecture planning phase.
For example, if you are developing a web application, you might focus solely on user features. However, how quickly the application loads or how it handles large user volumes is just as critical.
Why It Matters
Ignoring non-functional requirements can lead to a system that meets user needs initially but fails to perform under real-world conditions. To incorporate these requirements effectively, you can follow this approach:
- Identify Key Requirements: Gather stakeholders to discuss which quality attributes matter most.
- Set Metrics: Define clear metrics for measuring success for each requirement.
- Prototype Early and Often: Build early prototypes that stress test the architecture against non-functional requirements.
// Example: Simple implementation showing a responsiveness metric
class ResponseTime {
private long startTime;
private long responseTime;
public void startTimer() {
startTime = System.currentTimeMillis();
}
public void stopTimer() {
responseTime = System.currentTimeMillis() - startTime;
}
public long getResponseTime() {
return responseTime;
}
}
Here, we measure response time to gauge performance, which is a non-functional requirement. Efficient architecture requires measuring and optimizing these metrics from the get-go.
2. Lack of Documentation
The Document Dilemma
In the early stages of a project, team members are often focused on producing new features rather than documenting their design decisions. This lack of documentation can lead to significant issues later, particularly when team members change or transition.
Why It Matters
Good documentation can serve as a valuable tool for onboarding new team members, ensuring everyone is aligned, and maintaining the architecture over time. Key aspects to focus on include:
- Design Decisions: Document why specific choices were made.
- Architecture Diagrams: Use tools like Lucidchart or Draw.io for visual representation.
- Version Control for Documentation: Keep documentation updated with your codebase in a version control system.
3. Neglecting Scalability
Scalability is Not an Option, It's a Requirement
It is easy to design architecture for current needs, but neglecting scalability can lead to catastrophic failures as systems grow. Think about how your application will manage increased user loads or data volume.
Why It Matters
Without planning for scalability from the beginning, you may face a costly and complex overhaul later. Here are some scalability strategies:
- Load Balancing: Distribute requests among multiple servers to prevent any single server from becoming a bottleneck.
- Microservices: Design your application as a suite of small services (consider reading more about Microservices Architecture).
- Database Sharding: Split your data across multiple databases to improve performance for large datasets.
A basic example of load-shedding using Java could look like this:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class LoadBalancer {
private ExecutorService executorService;
public LoadBalancer(int numberOfServers) {
executorService = Executors.newFixedThreadPool(numberOfServers);
}
public void handleRequest(Runnable task) {
executorService.submit(task);
}
public void shutdown() {
executorService.shutdown();
}
}
Here, using a thread pool allows us to manage load effectively across multiple servers. This snippet illustrates a fundamental principle of not tying requests to a single point of failure.
4. Overengineering
The Temptation of Complexity
In an attempt to create a robust architecture, teams often fall into the trap of overengineering. This can lead to unnecessarily complex systems that become hard to understand and maintain.
Why It Matters
Overengineering usually leads to wasted resources and delayed project timelines. Opt for simplicity and elegance in your designs. Here are a couple of recommendations:
- Stick to MVC Patterns: Keeping your system organized using Model-View-Controller can help maintain a simple architecture.
- Evaluate Third-Party Solutions: Don't reinvent the wheel—leverage established frameworks when possible.
5. Ignoring Maintenance and Evolution
The Empire Strikes Back
A common misconception is that software architecture is a one-time task. In reality, as the application evolves, so must the architecture. This includes accommodating new feature requests, fixing bugs, and adopting emerging technologies.
Why It Matters
Neglecting this evolution leads to technical debt. Here's how to ensure your architecture can adapt over time:
- Code Reviews: Implement regular reviews to identify and address potential technical issues early.
- Keep Learning: Stay informed about emerging technologies and best practices.
- Refactor Regularly: Just because something works doesn't mean it shouldn't be improved. Regular refactoring can keep your codebase healthy.
Example of Refactoring
This example shows a simple Java class that can be refactored to improve readability and maintainability:
public class OrderProcessor {
public void process(Order order) {
validateOrder(order);
chargeCustomer(order);
sendConfirmation(order);
}
private void validateOrder(Order order) {
// validation logic here
}
private void chargeCustomer(Order order) {
// charging logic here
}
private void sendConfirmation(Order order) {
// sending logic here
}
}
By breaking down the process into clear, manageable methods, we can enhance readability and make future changes easier.
Final Thoughts
Software architecture planning is an intricate process riddled with potential pitfalls. By being aware of common mistakes—like underestimating non-functional requirements, neglecting scalability, and overengineering—developers can better prepare for a successful project.
Approach software architecture with the mindset of flexibility, documentation, and continuous learning. Ultimately, each decision should lead to a scalable, maintainable, and efficient system that stands the test of time.
For more insights into software architecture, check out this comprehensive guide on Architectural Patterns that provides deeper insights into effective planning techniques.
Happy coding!
Checkout our other articles