Mastering EJB 3.x: Navigating Concurrency Challenges
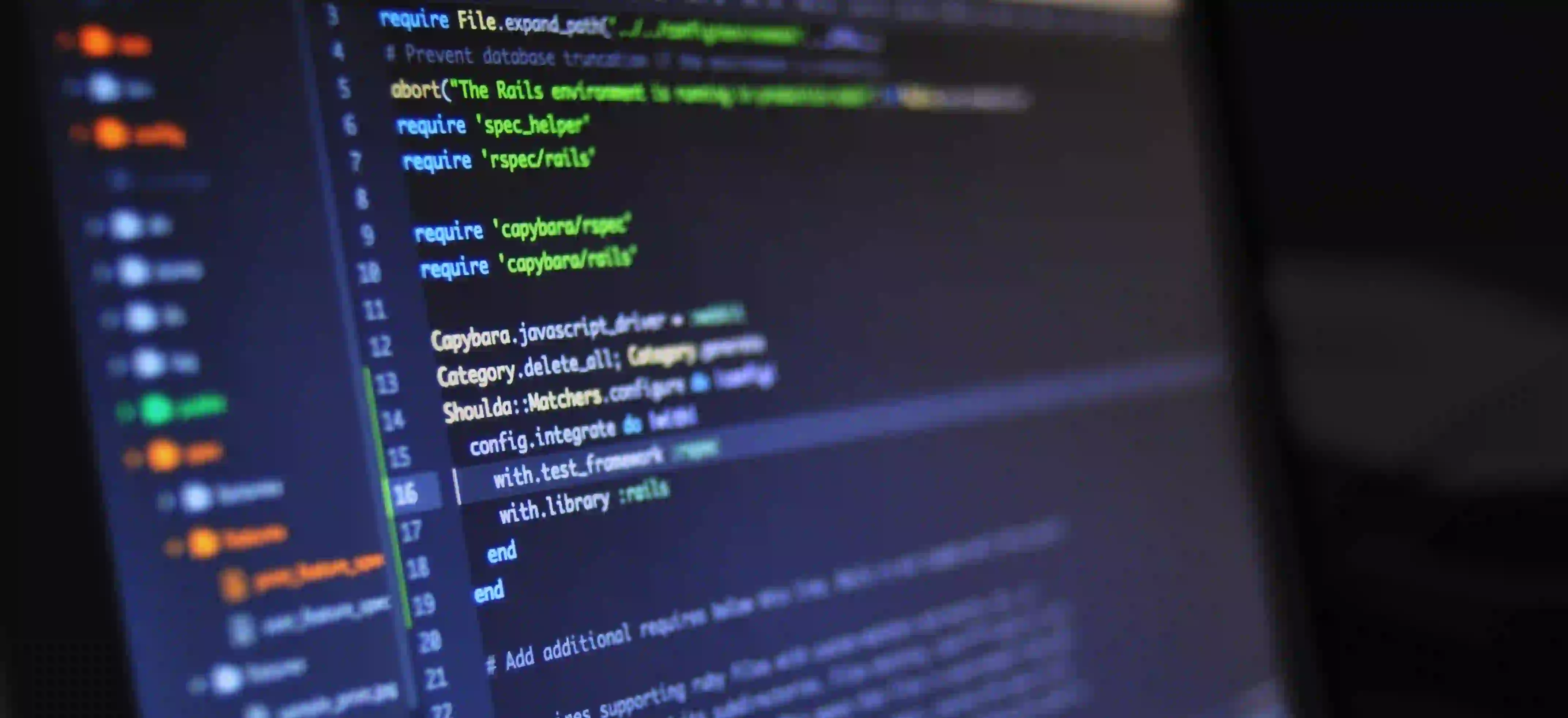
Mastering EJB 3.x: Navigating Concurrency Challenges
Enterprise JavaBeans (EJB) 3.x significantly simplifies the development of distributed, enterprise-level applications. However, one of the most intricate aspects of EJBs is handling concurrency. As applications scale, ensuring that multiple threads do not interfere with one another is vital for maintaining data integrity. In this post, we will explore the concurrency challenges in EJB 3.x and provide strategies to navigate them effectively.
Understanding EJB Concurrency
EJBs can operate in a multi-threaded environment. When multiple clients invoke methods on a singleton bean or when a bean is accessed concurrently, you need to manage their access thoughtfully. Concurrency in EJB revolves around three main aspects:
- Bean Types: EJBs can be stateful, stateless, or singleton, each affecting concurrency management differently.
- Transactional Context: The transaction management plays a vital role in state integrity.
- Concurrency Management Annotations: EJB 3.x introduces annotations that help manage concurrent access.
The Challenge of Stateful Beans
Stateful session beans maintain the state for a client across multiple method calls. Since the same bean instance is shared across multiple clients, ensuring thread safety becomes paramount. If two threads modify the same state's attributes simultaneously, it can lead to unpredictable results.
Solution: Use the @Lock
annotation to manage access.
import javax.ejb.Lock;
import javax.ejb.Singleton;
@Singleton
public class UserSessionBean {
private String username;
@Lock(LockType.WRITE)
public void setUsername(String username) {
this.username = username;
}
@Lock(LockType.READ)
public String getUsername() {
return username;
}
}
Explanation:
- @Lock(LockType.WRITE): This ensures that only one thread can modify
username
at a time. - @Lock(LockType.READ): Allows multiple threads to read
username
simultaneously but prevents them from writing to it. This balance is essential for performance and integrity.
Stateless Beans and Concurrency
Stateless session beans, on the other hand, are inherently thread-safe because they do not maintain any state. Each request is independent and can be handled by a different instance or even a different thread.
Even though there are fewer concerns regarding concurrency with stateless beans, keep in mind that if they invoke access to shared resources, like a database, concurrency issues can still arise.
Example of accessing a database resource in a stateless bean:
import javax.annotation.Resource;
import javax.ejb.Stateless;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
@Stateless
public class OrderServiceBean {
@PersistenceContext
private EntityManager em;
public void createOrder(Order order) {
em.persist(order);
}
}
Explanation:
In this example, the OrderServiceBean
can safely handle multiple clients creating orders simultaneously as it relies on the EntityManager
, which is managed by the application server. However, be cautious as accessing the same database record from different transactions might still pose a problem.
Singleton Beans and Concurrency
Singleton beans are shared across all clients and instances. Therefore, they pose the greatest concurrency challenges. It is important to consider the correct approach to manage shared state.
You can use the @Lock
annotation or even implement your custom synchronization mechanisms.
Here’s an example of implementing a singleton with concurrency control:
import javax.ejb.Lock;
import javax.ejb.Singleton;
@Singleton
public class ConfigurationManager {
private String configValue;
@Lock(LockType.WRITE)
public void updateConfig(String value) {
configValue = value;
}
@Lock(LockType.READ)
public String getConfig() {
return configValue;
}
}
Explanation:
Locking is similar to the previous examples. The singleton allows controlled write access while facilitating multiple readers simultaneously.
Managing Transactions
The transactional context isn’t just about ensuring data integrity; it helps resolve many concurrency issues.
Using @TransactionAttribute
can help dictate how methods handle transactions. For example, if a method has REQUIRES_NEW
, it begins a new transaction, isolating changes from the calling transaction.
import javax.ejb.TransactionAttribute;
import javax.ejb.TransactionAttributeType;
@Stateless
public class PaymentService {
@TransactionAttribute(TransactionAttributeType.REQUIRES_NEW)
public void processPayment(Payment payment) {
// Process payment logic here
}
}
Explanation:
This can prevent issues that arise when a calling method rolls back. By encapsulating payment processing in its transaction, you ensure that the state is consistently maintained without interference.
Advanced Concurrency Patterns
For more complex concurrency challenges, consider implementing advanced patterns like:
-
Optimistic Locking: This pattern allows multiple threads to operate on the same entity without locking it upfront. You can manage concurrency by checking version fields before committing.
-
Pessimistic Locking: This is used when you expect contention. By locking the record in the database until the transaction completes, you prevent other transactions from accessing it.
Example of Optimistic Locking
import javax.persistence.Entity;
import javax.persistence.Version;
@Entity
public class Product {
@Id
private Long id;
@Version
private Long version;
private String name;
// other fields
}
Explanation:
In this entity, the @Version
annotation is used to track changes. When two transactions attempt to modify the same Product
, the version check will ensure that one of them fails unless you reconcile the changes.
Wrapping Up
Concurrency management in EJB 3.x is a multifaceted challenge that requires understanding the behavior of different bean types, proper transaction management, and the appropriate use of locking mechanisms. By carefully analyzing your application's needs and implementing the strategies discussed, you can ensure a robust and scalable enterprise application.
For more in-depth understanding of EJB concurrency and best practices, consider these resources:
- Oracle's EJB Documentation
- Java EE Concurrency Utilities in EJB
By mastering these concepts, you'll enhance your application's performance and reliability. Happy coding!