The Hidden Challenges of Implementing Continuous Testing
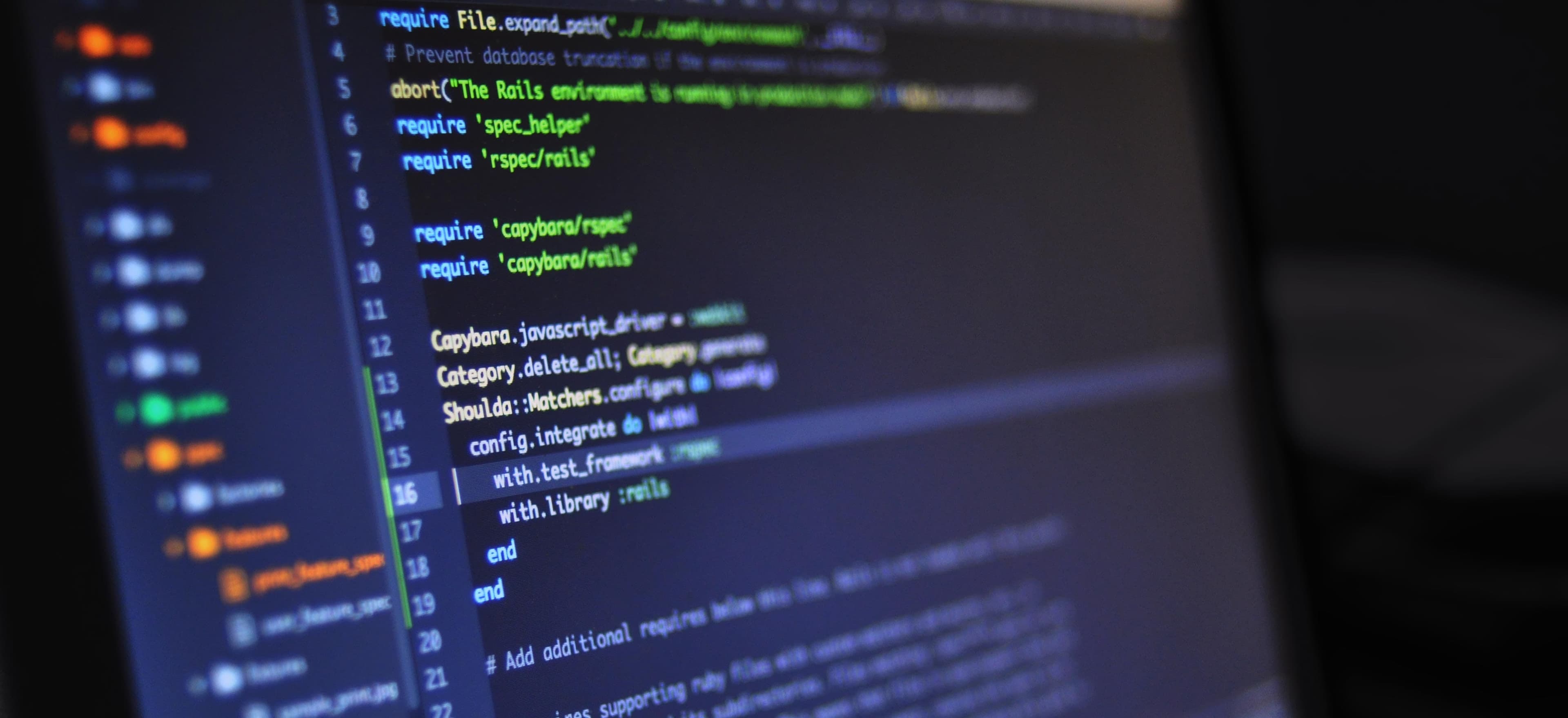
- Published on
The Hidden Challenges of Implementing Continuous Testing
Continuous Testing (CT) has become a critical component of modern software development practices, particularly in the realm of Agile and DevOps. The concept revolves around integrating automatic software testing into the software delivery pipeline, allowing for rapid feedback and enabling teams to detect and fix defects early in the development process. While the benefits of continuous testing are apparent, several hidden challenges can complicate its effective implementation. In this blog post, we will explore these challenges in-depth, providing insight and possible solutions to help you navigate the complexities of continuous testing.
Understanding Continuous Testing
Before diving into the challenges, let’s establish a clear definition of continuous testing. Continuous testing is the practice of executing automated tests as part of the software delivery pipeline to obtain immediate feedback on the business risks associated with a software release candidate. The goal is to ensure that software is always at a high level of quality and ready to deliver, thus accelerating the release cycle.
For a more detailed understanding, refer to the official Continuous Testing in DevOps glossary entry.
Benefits of Continuous Testing
While we will mainly focus on the challenges, it's essential to recognize the positive impacts of adopting continuous testing:
- Faster Release Cycles: Enables quicker feedback loops, facilitating faster releases.
- Increased Quality: More frequent testing helps identify and resolve bugs early in the development lifecycle.
- Reduced Risk: Continuous feedback allows developers to spot issues before they escalate into significant problems.
Despite these benefits, the following challenges often arise, often unnoticed until they significantly impact the testing process.
1. Culture Shift
The Challenge
Implementing continuous testing requires a cultural shift within an organization. It demands collaboration among various roles—developers, QA engineers, and operations teams. Yet, many organizations find these groups work in silos, making effective communication and collaboration difficult.
Why This Matters
Cultural resistance can delay the adoption of practices essential for continuous testing. If team members are not on the same page or invested in the process, the quality and reliability of testing could suffer.
Possible Solutions
- Foster a Collaborative Environment: Encourage open dialogues among team members from different disciplines. Conduct regular meetings to discuss the importance of shared responsibility for quality.
- Integrate DevOps Principles: Emphasize the DevOps philosophy where development and operations work cohesively towards common performance goals.
2. Tooling Challenges
The Challenge
The market is saturated with various testing tools, and selecting the right mix can become overwhelming. Furthermore, integrating various tools into a CI/CD pipeline can introduce additional complexity, leading to higher maintenance overhead.
Why This Matters
Choosing the wrong tools can slow down the process rather than expedite it. Integration issues can result in fragmented workflows, leading to missed tests and delayed feedback.
Possible Solutions
- Conduct Thorough Research: Before jumping into a tool selection, evaluate multiple pieces of software that meet your team’s specific needs. Consider factors like integration capabilities, ease of use, and long-term support.
- Leverage Open Source Tools: Many open-source tools can provide flexibility for teams, reducing licensing costs. Some popular options include Selenium for browser automation and JUnit for unit testing.
Here's a simple example of a unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
Why This Code Matters: This snippet illustrates a basic unit test using JUnit to verify our Calculator
class’s add
method. Unit tests help catch bugs early by confirming the logic of methods in isolation.
3. Flaky Tests
The Challenge
One of the most frustrating challenges in continuous testing is dealing with flaky tests—tests that produce inconsistent results, failing sometimes and passing at other times. Flaky tests can undermine trust in the testing process.
Why This Matters
Flaky tests can lead developers to disregard test results, making it difficult to determine the reliability of the system. This can result in further debugging efforts and wasted time.
Possible Solutions
- Root Cause Analysis: Investigate failed tests to establish whether they are genuinely defective or merely flaky. Address the underlying issues causing flakiness, such as dependencies on external data or timing issues.
- Create Stable Test Environments: Use containerization (like Docker) to create consistent and isolated environments for running tests. Reducing external variables helps achieve stable test outcomes.
4. Test Coverage
The Challenge
Achieving adequate test coverage can be daunting. Many teams struggle to ensure their code is thoroughly tested, often leaving critical paths untested.
Why This Matters
Inadequate test coverage can lead to undetected bugs. When deploys happen frequently without robust end-to-end testing, the software may introduce severe defects in production.
Possible Solutions
- Implement Code Coverage Tools: Use tools that analyze your code's test coverage (e.g., JaCoCo for Java applications) to help identify untested paths.
- Prioritize Critical Logic: Focus on covering vital features and frequently altered code first, reducing risk before incrementally expanding coverage.
// Example of code coverage using JaCoCo
public class UserService {
public boolean isValidUser(String username) {
return username != null && !username.isEmpty();
}
}
In this snippet, the isValidUser
method is a simple validation function. Writing tests to cover this will help ensure that all user input validations are reliable and robust, laying the groundwork for better application security and user experience.
5. The Complexity of Automation
The Challenge
Automating tests can lead to initial complexity. While automation is a vital part of continuous testing, the setup and management of automation scripts can become cumbersome.
Why This Matters
Businesses might become bogged down in automation at the expense of manual testing where necessary. An overreliance on automation without clear strategy can introduce more bugs than it resolves.
Possible Solutions
- Begin Small: Start by automating the most critical tests and gradually expand. Focusing on these areas reduces complexity and offers immediate value.
- Review and Refactor: Regularly revisit automated tests to ensure they remain relevant and high-quality.
Bringing It All Together
Continuous Testing can significantly enhance software quality and speed up delivery timelines. However, recognizing and addressing the hidden challenges is critical to reaping these benefits. By focusing on cultural shifts, diligent tooling choices, handling flaky tests, ensuring adequate test coverage, and managing automation complexity, teams can pave the way for a successful continuous testing strategy.
As you think about implementing continuous testing or refining your approach, consider these challenges and the proposed solutions. Continuous testing is not just about tools and scripts; it's about people, processes, and maintaining a relentless focus on quality.
For more information, feel free to explore these resources:
- Continuous Testing & Shift Left Testing
- The Role of Test Automation in Continuous Testing
Happy testing!
Checkout our other articles