Overcoming Challenges in 3-Tier GIS Application Development
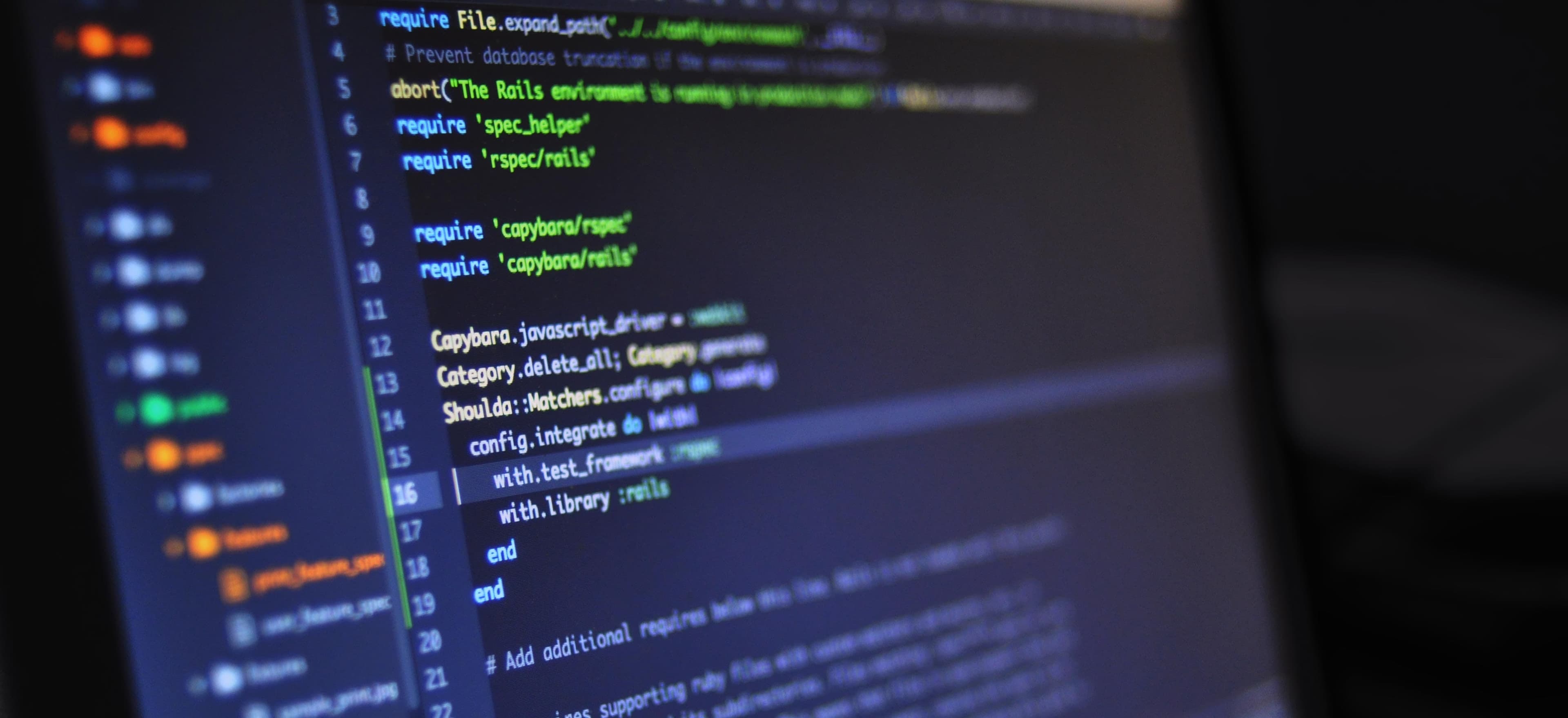
- Published on
Overcoming Challenges in 3-Tier GIS Application Development
Geographic Information Systems (GIS) are essential for managing, analyzing, and visualizing spatial data. When developing a GIS application, the 3-tier architecture—consisting of presentation, application, and data tiers—offers a robust way to build scalable solutions. However, navigating through the complexities of 3-tier GIS application development can be daunting. This blog post delves into the challenges you may encounter while developing a 3-tier GIS application and provides effective strategies for overcoming them.
Understanding the 3-Tier Architecture
Before we dive into challenges, let’s clarify the three tiers of this architecture:
-
Presentation Tier: The user interface of the application. It interacts with users and displays geospatial data.
-
Application Tier: The server-side logic where processing occurs. It's responsible for business rules, processing requests, and interacting with the data tier.
-
Data Tier: The backend database where spatial and non-spatial data is stored.
Each tier has its functionality and technology stack, and they communicate through well-defined interfaces.
Common Challenges in 3-Tier GIS Application Development
1. Data Management Complexity
In GIS, the data can be quite massive, encompassing everything from point data to complex relationships between different geographic entities. Managing this diversity can be a significant challenge.
Solution: Use Geospatial Databases
To efficiently manage spatial data, a relational database with geospatial extensions (like PostgreSQL with PostGIS) is recommended. This type of database allows for efficient storage, retrieval, and querying of spatial data.
-- Create a spatial table in PostgreSQL
CREATE TABLE locations (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
geom GEOGRAPHY(POINT, 4326) -- Using geography type for global coordinates
);
Why: The use of geospatial data types enhances the ability to perform spatial queries and ensures that your application scales as data grows.
2. Integration of Multiple Data Sources
3-tier architectures often need to integrate data from various sources such as REST APIs, external databases, or file systems. This can lead to inconsistencies, data duplication, and synchronization problems.
Solution: Implement a Unified Data Access Layer
Creating a data access layer that abstracts the intricacies of data source integration allows you to manage these systems as a single entity. You can use an Object-Relational Mapping (ORM) tool such as Hibernate for Java.
// Example of using Hibernate for geospatial data
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
Location location = new Location("LocationName", new Point(30.0, 10.0));
session.save(location);
transaction.commit();
session.close();
Why: Abstracting data access through an ORM minimizes potential integration issues, leading to cleaner, more maintainable code.
3. User Experience (UX) Optimization
In GIS applications, users expect an interactive interface that represents data effectively on maps. Poor UX can lead to user frustration and abandonment of the application.
Solution: Leverage Modern Frontend Frameworks
Using a frontend framework like React or Angular along with libraries such as Leaflet or OpenLayers can significantly enhance the user experience.
// Sample code using Leaflet to create a simple map
var map = L.map('map').setView([51.505, -0.09], 13);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
maxZoom: 19,
attribution: '© OpenStreetMap'
}).addTo(map);
Why: Modern frameworks improve interaction speed and responsiveness, ensuring users can explore data seamlessly.
4. Performance Issues
As your application scales up with increasing users, performance can become a crucial factor. GIS applications, in particular, may require rendering large datasets which can slow down performance.
Solution: Implement Caching Strategies
Using caching mechanisms like Redis or in-memory caching helps to reduce load times by storing frequently accessed data in memory.
@Cacheable("locations")
public List<Location> getAllLocations() {
return locationRepository.findAll();
}
Why: Caching reduces database load and response time, enhancing overall performance and user satisfaction.
5. Security Concerns
A GIS application often deals with sensitive geographical data, making it vulnerable to various security threats. Adequate measures must be taken to safeguard both data and user privacy.
Solution: Implement Security Best Practices
Use secure APIs, HTTPS, and authentication mechanisms such as OAuth2 to protect data and service layers. Additionally, role-based access control will ensure that users only have access to data necessary for their roles.
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
Why: Enforcing strict security measures protects your application from unauthorized access and data breaches.
6. Handling Spatial Analysis
Spatial analysis operations can be computationally intensive, often requiring significant resources and specialized algorithms.
Solution: Use Dedicated Libraries for Spatial Analysis
Leveraging libraries like GeoTools or JTS (Java Topology Suite) allows you to perform spatial operations efficiently within your application.
// Example of using JTS for spatial operations
GeometryFactory geometryFactory = new GeometryFactory();
Point point1 = geometryFactory.createPoint(new Coordinate(1, 1));
Point point2 = geometryFactory.createPoint(new Coordinate(2, 2));
double distance = point1.distance(point2);
Why: Utilizing specialized libraries optimizes performance and ensures accurate spatial operations.
Bringing It All Together
Developing a 3-tier GIS application presents unique challenges — from data management to ensuring user satisfaction. By understanding these challenges and implementing the proposed solutions, you can develop a more robust and scalable GIS application.
Make use of modern technologies, adhere to security practices, and continuously optimize both your backend and frontend layers. Remember to keep users at the forefront of your development process, and leverage the community around GIS development for ongoing support and innovation.
For further reading and resources, you can explore:
With the right strategies and tools, your journey in 3-tier GIS application development can indeed be rewarding and successful.
Checkout our other articles