Streamlining JSON Processing in Java: A JSR 353 Guide
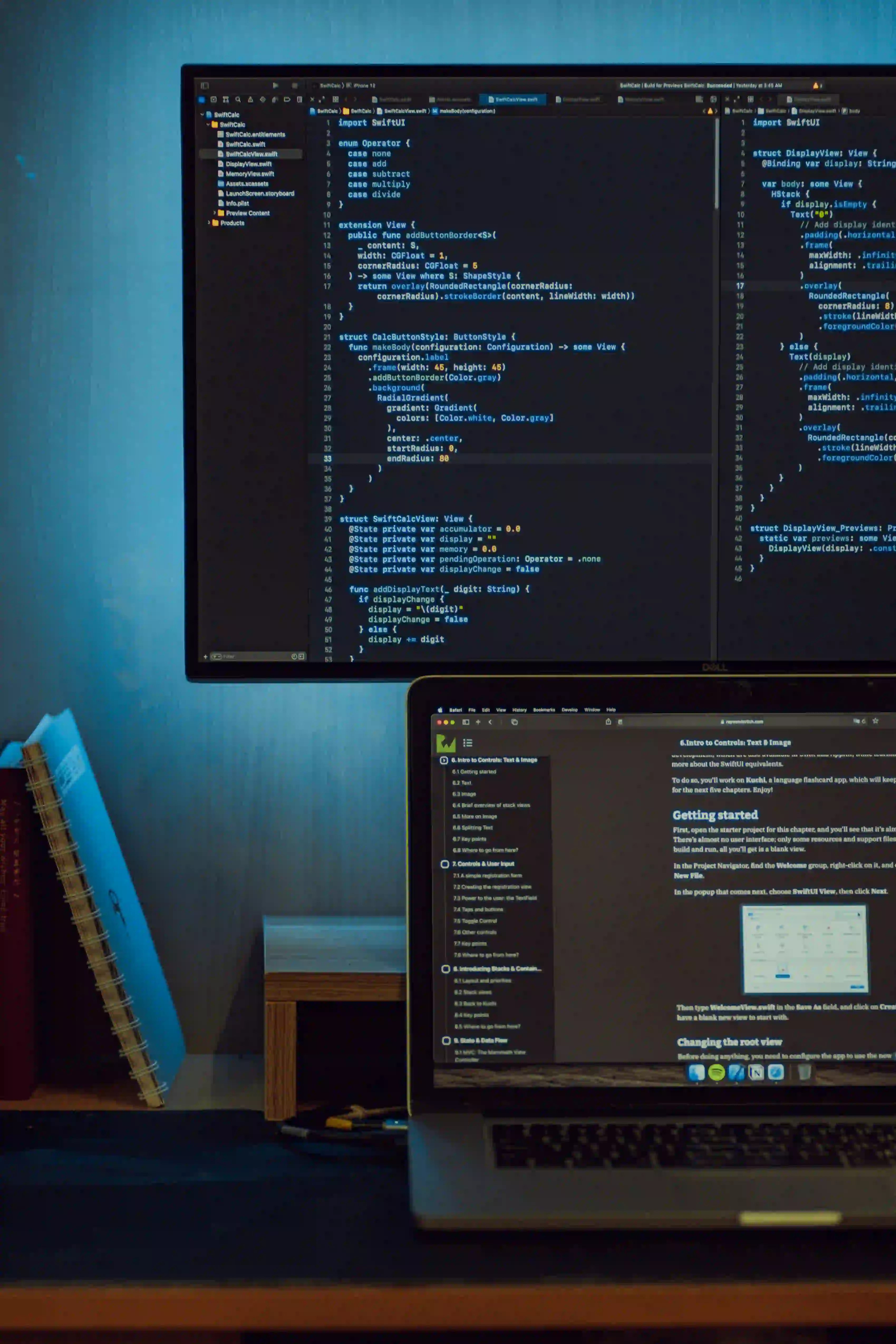
Streamlining JSON Processing in Java: A JSR 353 Guide
In today's data-driven world, JSON (JavaScript Object Notation) has become a popular choice for data interchange formats. Its lightweight nature and ease of use make it ideal for web applications, APIs, and integration across different services. For Java developers, handling JSON has been made simpler with the introduction of JSR 353 (Java API for JSON Processing).
In this blog post, we will explore the essentials of JSON processing in Java using JSR 353, delve into the advantages it provides, and walk through some practical examples. Let's dive in!
What is JSR 353?
JSR 353 is a part of Java EE’s API that specifies how to process JSON data in a standardized way. It allows developers to parse JSON data into Java objects, create JSON structures, and serialize Java objects to JSON efficiently. This unifying API simplifies integrating JSON into Java applications while maintaining the flexibility that developers crave.
Why Use JSON?
Understanding the advantages of JSON helps appreciate why JSR 353 is essential. JSON is:
- Lightweight: JSON is less verbose compared to XML.
- Easy to Read: Its simple structure makes it human-readable.
- Language-agnostic: Supported in multiple programming languages.
- Flexible: Can represent complex data structures with arrays and objects.
Key Components of JSR 353
Before we start implementing JSR 353, let's familiarize ourselves with its critical components. The API consists of several interfaces, including:
- JsonReader: Reads JSON data from a source.
- JsonWriter: Writes JSON data to a destination.
- JsonObject: Represents a JSON object.
- JsonArray: Represents a JSON array.
- JsonParser: Parses JSON data.
- JsonGenerator: Generates JSON data.
Setting Up the Environment
To get started, add the following dependency to your pom.xml
if you're using Maven:
<dependency>
<groupId>javax.json</groupId>
<artifactId>javax.json-api</artifactId>
<version>1.1.4</version>
</dependency>
<dependency>
<groupId>org.glassfish</groupId>
<artifactId>javax.json</artifactId>
<version>1.1.4</version>
</dependency>
This will pull in the necessary JSON Processing libraries for your project.
Basic JSON Operations with JSR 353
Reading JSON Data
Let's start by reading JSON data. Suppose we have a JSON string as follows:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"skills": ["Java", "Python", "JavaScript"]
}
Here's how you can read this data using JsonReader
:
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonReader;
import java.io.StringReader;
public class JsonReaderExample {
public static void main(String[] args) {
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"city\":\"New York\",\"skills\":[\"Java\",\"Python\",\"JavaScript\"]}";
JsonReader reader = Json.createReader(new StringReader(jsonString));
JsonObject jsonObject = reader.readObject();
reader.close();
System.out.println("Name: " + jsonObject.getString("name"));
System.out.println("Age: " + jsonObject.getInt("age"));
System.out.println("City: " + jsonObject.getString("city"));
System.out.println("Skills: " + jsonObject.getJsonArray("skills"));
}
}
Code Explanation
In this code snippet:
- We create a
StringReader
to read the JSON string. - We use
Json.createReader
to create aJsonReader
. readObject()
retrieves the rootJsonObject
.- We can fetch data using methods like
getString
,getInt
, andgetJsonArray
.
Writing JSON Data
Next, let's see how to create JSON data. We'll create a JSON object that reflects the same structure as the one we previously read.
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonWriter;
import java.io.StringWriter;
public class JsonWriterExample {
public static void main(String[] args) {
JsonObject jsonObject = Json.createObjectBuilder()
.add("name", "John Doe")
.add("age", 30)
.add("city", "New York")
.add("skills", Json.createArrayBuilder()
.add("Java")
.add("Python")
.add("JavaScript"))
.build();
StringWriter stringWriter = new StringWriter();
JsonWriter writer = Json.createWriter(stringWriter);
writer.write(jsonObject);
writer.close();
String jsonString = stringWriter.toString();
System.out.println(jsonString);
}
}
Code Explanation
In the JsonWriterExample
:
- We use
Json.createObjectBuilder()
to create a new JSON object. - The builder pattern allows easy chaining of
add
methods to add key-value pairs. - We build the object and write it to a
StringWriter
usingJsonWriter
. - Finally, we print the generated JSON string.
Advanced Features
JSR 353 also offers additional features like JSON-P streaming APIs for efficient processing of large JSON documents, and JsonPointer
for navigating to specific parts of JSON data.
Using JsonPointer
Here’s a brief example of using JsonPointer
to access a specific item in a JSON structure:
import javax.json.JsonPointer;
import javax.json.JsonObject;
import javax.json.Json;
import javax.json.JsonReader;
import java.io.StringReader;
public class JsonPointerExample {
public static void main(String[] args) {
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"city\":\"New York\",\"skills\":[\"Java\",\"Python\",\"JavaScript\"]}";
JsonReader reader = Json.createReader(new StringReader(jsonString));
JsonObject jsonObject = reader.readObject();
JsonPointer pointer = Json.createPointer("/skills/1"); // Access the second skill
String skill = pointer.getValue(jsonObject).toString();
System.out.println("Second Skill: " + skill);
}
}
Code Explanation
In the above code:
- We create a
JsonPointer
instance pointing to the second skill in the JSON array. - The
getValue
method returns the corresponding value.
The Closing Argument
JSR 353 provides a robust API for Java developers to handle JSON data effortlessly, whether reading, writing, or manipulating JSON structures. The API’s intuitive design and method chainability simplify complex data interactions, making it easier to integrate JSON within applications.
Understanding and implementing JSR 353 can position you well in today's job market, where JSON is ubiquitous. For further reading, you can check out the official Java EE Documentation.
Additional Resources
By leveraging JSR 353, you are well on your way to mastering JSON processing in Java and making scalable, efficient applications. Happy coding!