Solving Microservices: Common Pitfalls and Solutions
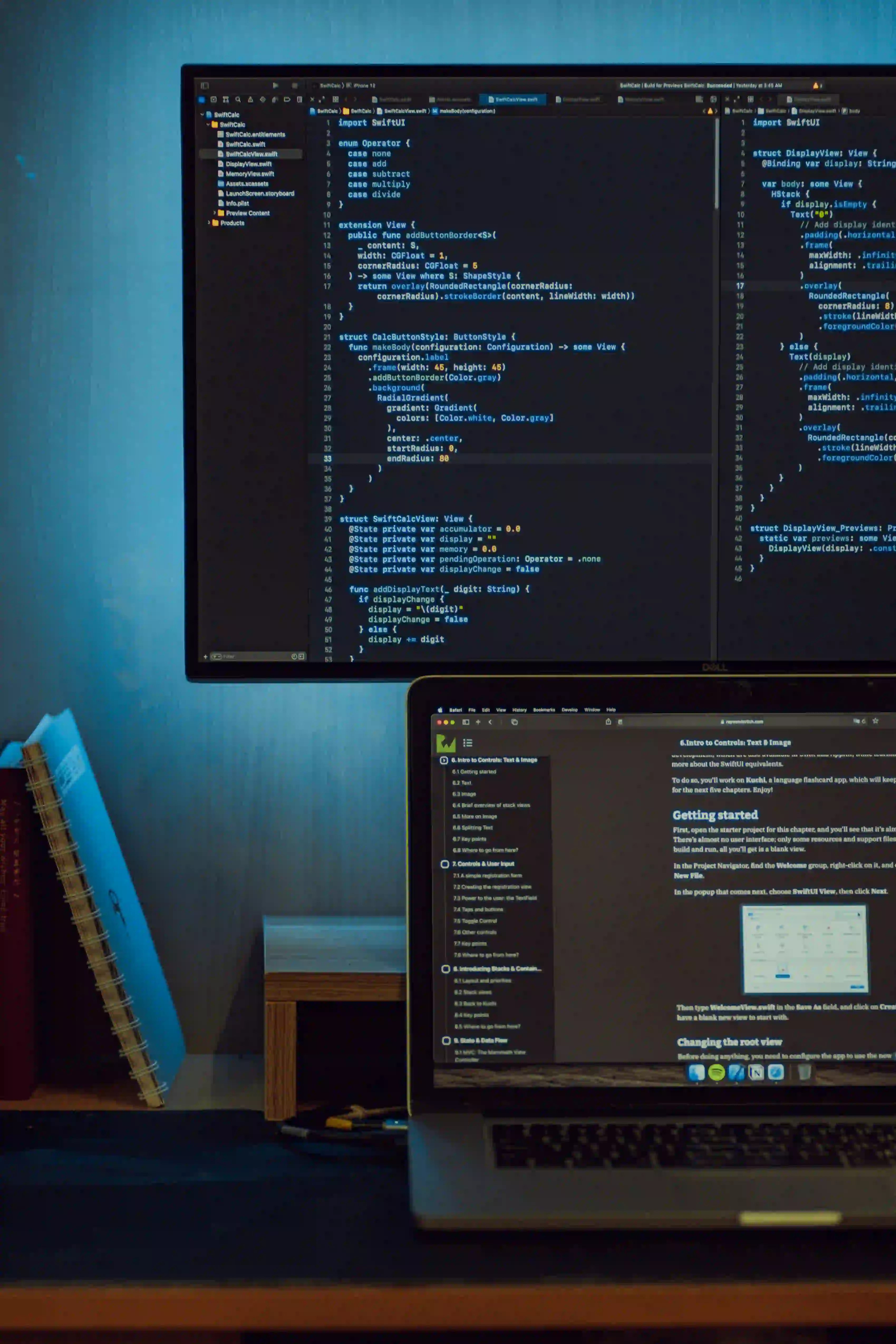
Solving Microservices: Common Pitfalls and Solutions
In recent years, microservices architecture has become a preferred approach for building scalable and resilient applications. While it offers a plethora of advantages, migrating to a microservice-based system can be challenging. In this blog post, we will explore some common pitfalls encountered during microservices implementation and propose actionable solutions.
What Are Microservices?
Microservices are a cloud-native architecture approach that structures an application as a collection of small, independently deployable services. Each service is built around a specific business capability and can communicate with others via APIs. This modularization facilitates continuous development and deployment, enhances scalability, and allows teams to leverage different technologies.
For an in-depth resource on microservices architecture, check out Martin Fowler's article.
Common Pitfalls
1. Over-Engineering
The Issue
One of the first pitfalls teams face with microservices is over-engineering. Developers often attempt to apply microservices even for simple applications that do not require the complexity. This leads to unnecessary overhead in development time and resource management.
Solution
Base the decision to adopt microservices on the application's needs. If the application is relatively small or is a straightforward CRUD operation, it may be more beneficial to stick with a monolith. Always remember: microservices should bring value, not complexity.
2. Communication Overhead
The Issue
Microservices communicate over a network, leading to the communication overhead problem. Latency issues can arise due to the inter-service calls, negatively impacting performance.
Solution
Adopt asynchronous messaging and event-driven architecture using message brokers like RabbitMQ or Apache Kafka. This reduces synchronous API calls that block processes and allows services to operate independently.
Example Code Snippet: Using Spring Boot and RabbitMQ for asynchronous messaging.
// Producer class that sends messages to a queue
@Service
public class MessageProducer {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("messageQueue", message);
System.out.println("Message Sent: " + message);
}
}
In this example, the sendMessage
method sends a message to a RabbitMQ queue asynchronously. This decouples services and minimizes the impact of network delays.
3. Data Consistency
The Issue
In a microservices architecture, each service typically manages its own database. This leads to potential data consistency problems when trying to achieve transactional properties across multiple services.
Solution
Instead of relying on distributed transactions, adopt eventual consistency models. Utilize patterns such as the Saga pattern or Distributed Choreography. These mechanisms allow for a more manageable way to achieve consistency without compromising on the service's independence.
Example Code Snippet: Basic implementation of the Saga pattern.
public class OrderService {
public void placeOrder(Order order) {
// Step 1: Create Order
createOrder(order);
// Step 2: Reserve Stock
reserveStock(order.getProductId(), order.getQuantity());
// Step 3: Make Payment
makePayment(order.getPaymentDetails());
// Handle failures by compensating each step
}
private void createOrder(Order order) {
// Implementation here
}
private void reserveStock(String productId, int quantity) {
// Implementation here
}
private void makePayment(PaymentDetails paymentDetails) {
// Implementation here
}
}
This code serves as a high-level representation of how services can independently participate in a series of actions (a Saga). On failures, compensatory actions can be implemented to maintain consistency.
4. Insufficient Monitoring and Logging
The Issue
With many components communicating via APIs, traditional logging approaches become insufficient. Missing logs can lead to difficulties in debugging and monitoring.
Solution
Implement centralized logging and monitoring systems. Tools such as ELK Stack (Elasticsearch, Logstash, Kibana) or Grafana with Prometheus allow administrators to aggregate and analyze logs from multiple services efficiently.
Utilizing a unique correlation ID across services helps track requests back to their source.
5. Lack of Governance
The Issue
When microservices proliferate, organizations can face governance issues. Different teams might develop services independently, leading to variations in standards, protocols, and technologies.
Solution
Implement a governance model that defines conventions, best practices, and standards across teams. Establish clear guidelines for API design, service communication, and documentation.
Utilizing tools like Swagger/OpenAPI can standardize API documentation across services.
Example Code Snippet: Basic OpenAPI configuration.
import io.swagger.v3.oas.annotations.OpenAPIDefinition;
@OpenAPIDefinition
@RestController
@RequestMapping("/api/orders")
public class OrderController {
// Endpoint declarations with Swagger annotations
}
By decorating your controllers with OpenAPI annotations, you create a standardized approach to API documentation that facilitates communication among teams and curtails discrepancies.
6. Security Gaps
The Issue
With every microservice exposing an API, the attack surface increases, leading to potential security vulnerabilities.
Solution
Adopt a security-first approach:
- Authentication: Implement API gateways to handle authentication.
- Authorization: Use role-based access control (RBAC) for resource access.
- Network Security: Utilize service mesh technologies like Istio to enforce network policies.
Ensure each service validates requests carefully and implements methods to protect against threats such as SQL injections, cross-site scripting (XSS), and denial-of-service attacks.
The Last Word
Microservices can help address complexities in modern application development while providing scalability and flexibility. However, teams must be aware of the common pitfalls that can arise.
From over-engineering to security concerns, understanding these issues is essential for successful microservice implementation. Embracing best practices, leveraging the right tools, and fostering a culture of collaboration can significantly enhance the effectiveness of microservices in your organization.
Arming yourself with the knowledge of both the challenges and solutions will place you on the pathway to microservices success. If you are planning to dive deeper into these practices, consider looking into resources like Jesse Shilty's exploration of microservice design which offers more insights.
By adopting a thoughtful and well-governed approach, you can unlock the full potential of microservices while ensuring a robust application architecture.
Ready for a journey into microservices? Let's build something remarkable!
Feel free to comment below with your experiences, questions, or topics you'd like us to dive into regarding microservices.