Boost Your Test Suite: Custom Assertions Made Easy!
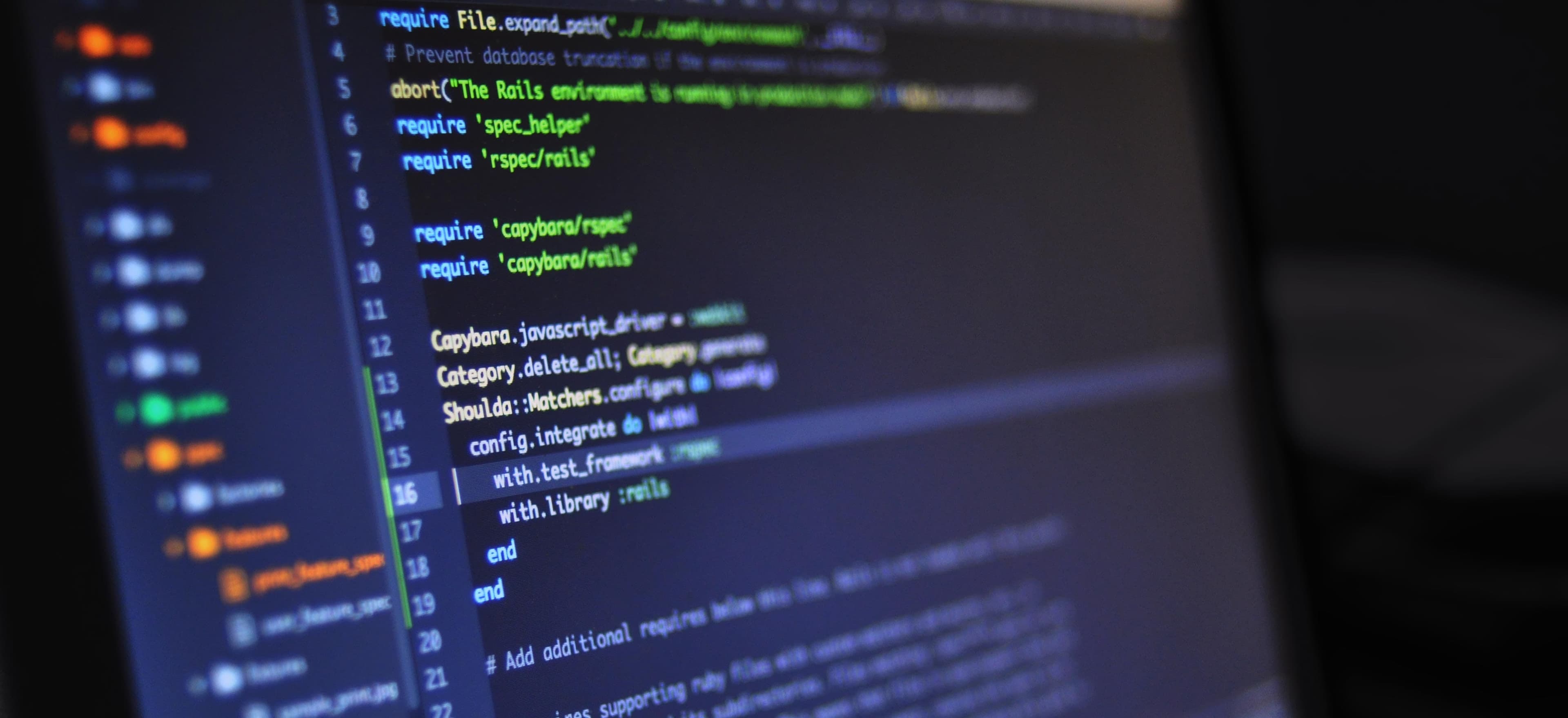
- Published on
Boost Your Test Suite: Custom Assertions Made Easy!
Whether you're a seasoned Java developer or just getting started, writing efficient and comprehensive test cases is crucial for ensuring the reliability and quality of your software. As we push the boundaries of application complexity, custom assertions can significantly streamline this process. In this blog post, we'll dive deep into creating custom assertions in Java, highlighting their importance, benefits, and how to implement them effectively.
Understanding Assertions in Java Testing
Assertions are statements that guarantee a certain condition holds true at a specific point in your code. If this condition evaluates to false, an error is thrown, indicating that something is wrong. This feature is particularly useful in unit testing.
Testing frameworks like JUnit and TestNG provide built-in assertions. However, there are situations where these built-in assertions may fall short. This is where custom assertions come into play. Custom assertions allow you to encapsulate validation logic unique to your application, making your tests cleaner, more expressive, and reusable.
Why Use Custom Assertions?
-
Clarity and Maintainability: Custom assertions make your tests easier to read, enabling developers to quickly understand the intent behind each test case.
-
Reusability: By centralizing your assertion logic, you reduce redundancy and simplify updates when requirements change.
-
Specific Feedback: Custom assertions can provide more informative error messages, which makes debugging a breeze.
-
Convenience: They streamline complex validation logic, reducing boilerplate code in your tests.
Getting Started with Custom Assertions
Let's break down how custom assertions can be created and utilized in a Java testing environment using JUnit as our framework.
Step 1: Set Up Your Project
To follow along, you will need a basic Java project with JUnit. If you haven't already, you can add JUnit to your Maven project by including the following dependency in your pom.xml
:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
Step 2: Creating a Custom Assertion Class
Now, let’s create a custom assertion class. Suppose we're dealing with a user entity where we want to assert that a user's age is valid. We can create a custom assertion that encapsulates this logic.
public class UserAssertions {
public static void assertIsValidUser(User user) {
if (user == null) {
throw new AssertionError("User object should not be null.");
}
if (user.getAge() < 0 || user.getAge() > 120) {
throw new AssertionError("User's age must be between 0 and 120.");
}
// Additional validations can go here
}
}
In this example, the assertIsValidUser
method checks the validity of a User
object. If the user is null or if the age is outside the typical range, it throws an AssertionError
with a descriptive message. This specificity can greatly aid in debugging.
Step 3: Using Custom Assertions in Your Test
Now, you can use the custom assertion in your test cases like so:
import org.junit.Test;
public class UserAssertionsTest {
@Test
public void testValidUser() {
User user = new User("John Doe", 30);
UserAssertions.assertIsValidUser(user); // This should pass without throwing exceptions
}
@Test(expected = AssertionError.class)
public void testNullUser() {
UserAssertions.assertIsValidUser(null); // This should throw AssertionError
}
@Test(expected = AssertionError.class)
public void testInvalidAge() {
User user = new User("John Doe", 130);
UserAssertions.assertIsValidUser(user); // This should throw AssertionError
}
}
In these tests, we invoke our assertIsValidUser
method to check different scenarios. If the assertions fail, the tests will throw an AssertionError
, which is exactly what we want.
Step 4: Enhancing Usability
You can further improve your custom assertions by adding more context or combining them with other validators. For instance, you could create a chain of validation methods for comprehensive user validation.
Here's how you might add a method to check if the user has a valid email:
public class UserAssertions {
public static void assertIsValidUser(User user) {
assertUserNotNull(user);
assertUserAge(user.getAge());
assertUserEmail(user.getEmail());
}
private static void assertUserNotNull(User user) {
if (user == null) {
throw new AssertionError("User object should not be null.");
}
}
private static void assertUserAge(int age) {
if (age < 0 || age > 120) {
throw new AssertionError("User's age must be between 0 and 120.");
}
}
private static void assertUserEmail(String email) {
if (email == null || !email.contains("@")) {
throw new AssertionError("A valid email is required.");
}
}
}
Best Practices for Custom Assertions
-
Descriptive Error Messages: Always include meaningful messages when throwing assertion errors. This will assist developers in quickly identifying problems during test failure.
-
Keep It Simple: Ensure that your custom assertions focus on a specific aspect of test validation. This enhances readability and usability.
-
Document Custom Assertions: Provide documentation or comments to explain the purpose and usage of your custom assertions. This is especially crucial when working in teams.
-
Consider Reusability: If certain assertions may be relevant across multiple classes or modules, consider creating a base assertion class or utility for broader use.
My Closing Thoughts on the Matter
Incorporating custom assertions into your Java testing strategy can significantly enhance the clarity, maintainability, and reliability of your test suite. By writing precise, reusable assertions, you streamline the testing process, leaving behind complex and unwieldy test code.
For further reading on JUnit testing frameworks and best practices, you may refer to the JUnit official documentation and the Effective Java book by Joshua Bloch.
Get started today! Implement custom assertions to boost your test suite and enjoy clearer, more effective tests.
Checkout our other articles