Mastering Java String Manipulation: Top Interview Challenges
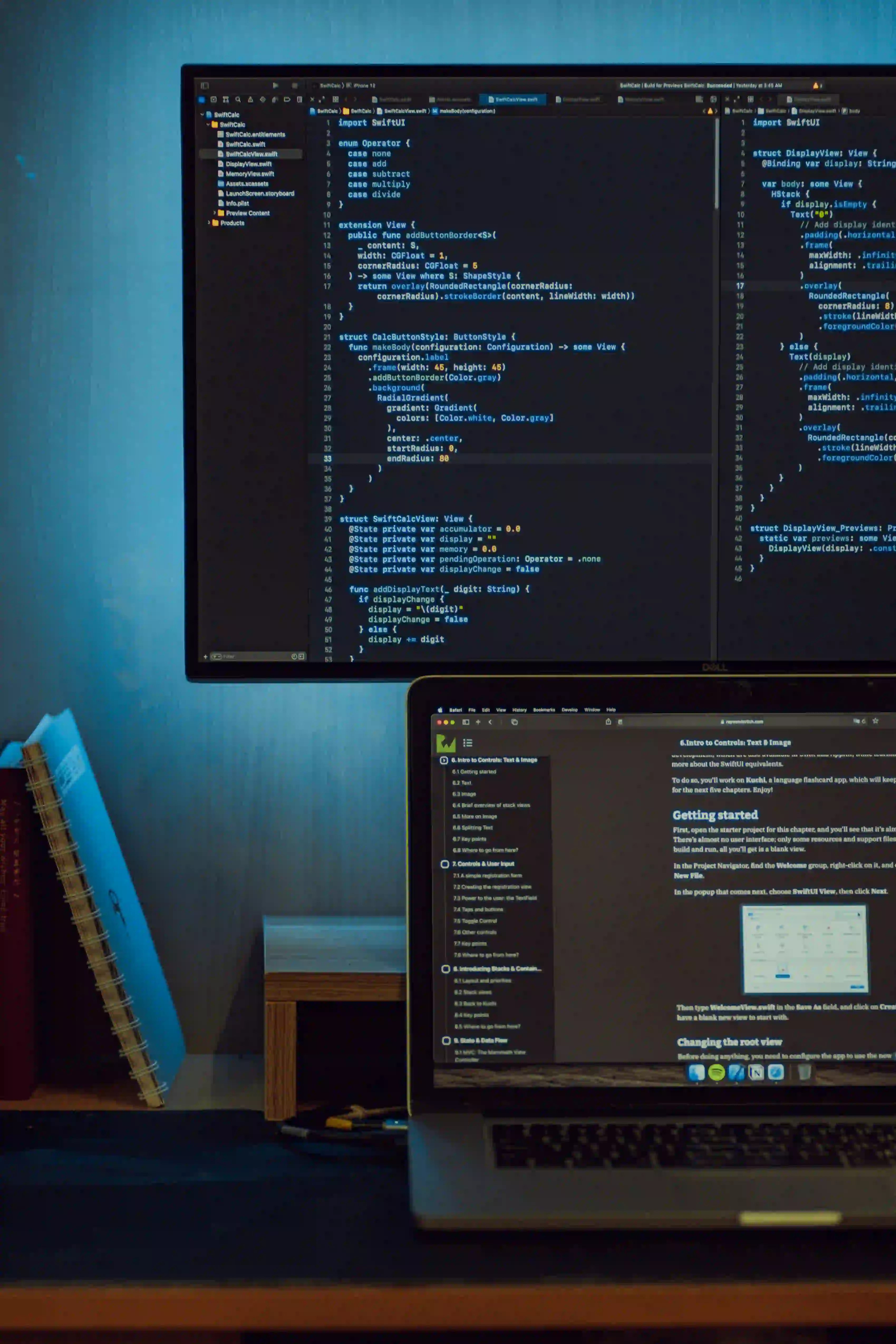
Mastering Java String Manipulation: Top Interview Challenges
Java is a powerful and versatile programming language, renowned for its robustness and simplicity. Among the numerous topics developers will encounter, string manipulation is a fundamental skill that is often tested in technical interviews. Understanding how to efficiently manipulate strings can set you apart as a candidate, showcasing both your knowledge of the Java API and your problem-solving abilities.
In this blog post, we’ll cover some common challenges related to Java string manipulation, providing code snippets accompanied by clear commentary to guide you through the reasoning and logic behind each solution.
Understanding Java Strings
Before diving into challenges, let's clarify what strings are in Java. Strings in Java are immutable, which means once a string object is created, it cannot be modified. This immutability may seem limiting, but it also enhances performance when handling string values.
Key Characteristics of Java Strings:
- Immutability: Strings cannot be changed after creation.
- String Pooling: Java stores string literals to optimize memory usage.
- Built-in Methods: Java provides numerous built-in methods, such as substring(), length(), and more.
Now that you have a solid understanding of strings, let's dive into popular string manipulation challenges often encountered in interviews.
Challenge 1: Reversing a String
One classic challenge is reversing a string. You’re given a string input, and you need to return the reverse of that string.
Sample Code
public class StringReversal {
public static String reverse(String original) {
StringBuilder reversed = new StringBuilder(original);
return reversed.reverse().toString();
}
public static void main(String[] args) {
String input = "Java";
String output = reverse(input);
System.out.println(output); // Outputs: avaJ
}
}
Explanation
- StringBuilder: We utilize StringBuilder because it is mutable. This allows us to reverse the string efficiently without creating multiple intermediate objects.
- reverse() method: The built-in reverse() method of StringBuilder helps to achieve the desired output in a single, elegant line of code.
Challenge 2: Check for Palindrome
Another common task is to determine if a string is a palindrome, meaning it reads the same backward as forward, such as "racecar".
Sample Code
public class PalindromeChecker {
public static boolean isPalindrome(String str) {
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
public static void main(String[] args) {
String testWord = "level";
boolean result = isPalindrome(testWord);
System.out.println(result ? "Palindrome" : "Not a Palindrome"); // Outputs: Palindrome
}
}
Explanation
- Two-Pointer Technique: We employ a two-pointer approach to compare characters from both ends of the string. This optimizes performance by ensuring we only traverse the string half the time.
- Efficiency: This approach runs in O(n) time complexity, making it effective even for longer strings.
Challenge 3: Counting Vowels and Consonants
In this challenge, you’ll write a function to count the number of vowels and consonants in a given string.
Sample Code
import java.util.HashMap;
public class VowelConsonantCounter {
public static HashMap<String, Integer> countVowelsAndConsonants(String str) {
HashMap<String, Integer> counts = new HashMap<>();
int vowels = 0, consonants = 0;
for (char ch : str.toLowerCase().toCharArray()) {
if (Character.isLetter(ch)) {
if ("aeiou".indexOf(ch) != -1) {
vowels++; // Increment vowel count
} else {
consonants++; // Increment consonant count
}
}
}
counts.put("Vowels", vowels);
counts.put("Consonants", consonants);
return counts;
}
public static void main(String[] args) {
String sentence = "Hello World";
HashMap<String, Integer> result = countVowelsAndConsonants(sentence);
System.out.println(result); // Outputs: {Vowels=3, Consonants=7}
}
}
Explanation
- HashMap for Counting: We utilize a HashMap to store the counts of vowels and consonants, offering an efficient way to associate keys (the types of characters) with their respective counts.
- Character Detection: The function converts the string to lowercase to handle case sensitivity easily while checking for vowels.
Challenge 4: Finding the First Non-Repeating Character
This challenge involves identifying the first non-repeating character in a string, which has applications in various real-world scenarios.
Sample Code
import java.util.LinkedHashMap;
public class FirstNonRepeatingChar {
public static Character firstNonRepeating(String str) {
LinkedHashMap<Character, Integer> charCountMap = new LinkedHashMap<>();
for (char ch : str.toCharArray()) {
charCountMap.put(ch, charCountMap.getOrDefault(ch, 0) + 1);
}
for (char ch : charCountMap.keySet()) {
if (charCountMap.get(ch) == 1) {
return ch; // Return the first non-repeating character
}
}
return null; // Return null if all characters repeat
}
public static void main(String[] args) {
String input = "swiss";
Character result = firstNonRepeating(input);
System.out.println(result != null ? result : "All characters repeat"); // Outputs: w
}
}
Explanation
- LinkedHashMap: This collection maintains the insertion order, which is crucial for identifying the first non-repeating character in a string.
- Efficiency: We traverse the string twice (once to build the map, once to check counts), yielding an overall time complexity of O(n).
Challenge 5: String Compression
The final challenge we discuss is to compress a string such that 'aaabb' becomes 'a3b2’.
Sample Code
public class StringCompressor {
public static String compress(String str) {
StringBuilder compressed = new StringBuilder();
int countConsecutive = 0;
for (int i = 0; i < str.length(); i++) {
countConsecutive++;
// If next character is different than current, append this character to the result
if (i + 1 >= str.length() || str.charAt(i) != str.charAt(i + 1)) {
compressed.append(str.charAt(i));
compressed.append(countConsecutive);
countConsecutive = 0; // Reset the counter
}
}
return compressed.toString();
}
public static void main(String[] args) {
String input = "aaabbbcc";
String output = compress(input);
System.out.println(output); // Outputs: a3b3c2
}
}
Explanation
- Run-Length Encoding: The algorithm counts consecutive characters efficiently and appends both the character and the count to the result as needed.
- Performance: The use of StringBuilder also enhances performance by minimizing string concatenation overhead.
Lessons Learned
Mastering string manipulation in Java is not just about knowing how to handle strings; it is about understanding the underlying principles and best practices that can make your code more efficient and maintainable.
These examples illuminate just a fraction of the string manipulation challenges you may encounter during interviews. For further reading, you can explore the Java String API Documentation for in-depth details on string methods and functionalities.
By practicing these challenges, you will not only prepare yourself for technical interviews but also become more proficient in your programming abilities. Happy coding!