Troubleshooting Jetty-Eclipse Integration for Seamless Development
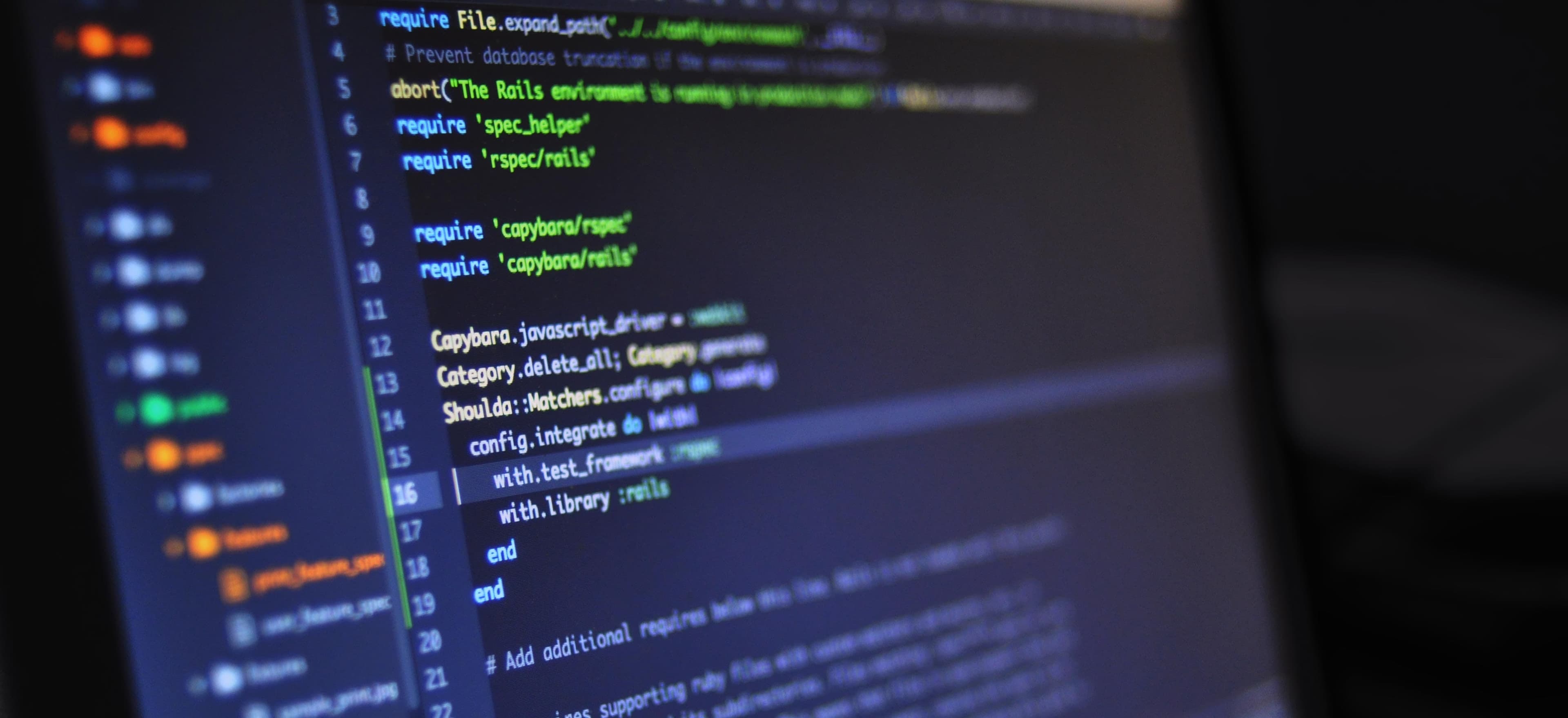
- Published on
Troubleshooting Jetty-Eclipse Integration for Seamless Development
Integrating Jetty with Eclipse can significantly enhance your development workflow, particularly if you’re building Java web applications. However, like any technical setup, you might encounter some bumps along the road. In this blog post, we’ll explore common issues and their solutions while providing insights into best practices to ensure a smooth integration process.
Understanding Jetty and Eclipse
Jetty is a lightweight and highly scalable web server and servlet container in Java, primarily used for running Java web applications. Eclipse, on the other hand, is a popular Integrated Development Environment (IDE) that provides functionalities to develop Java applications efficiently.
Combining the two allows developers to run web applications directly from the IDE without needing a separate server. However, misconfigurations can lead to frustrating debugging sessions and workflow interruptions.
Common Issues in Jetty-Eclipse Integration
1. Server Doesn't Start
Issue
After following the setup process, attempting to start Jetty may result in an error indicating the server cannot start.
Solution
Ensure that your project is correctly configured. Here’s how to verify:
-
Check Your Configuration.
- Right-click on your project in Eclipse, select "Properties," and navigate to "Targeted Runtimes."
- Ensure that Jetty is selected as the runtime for the project.
-
Proper Installation of Jetty Plugins.
- Double-check whether you installed Jetty’s Eclipse plugin correctly. For steps, consider referring to the Jetty documentation.
-
Review Console Output.
- Often, Jetty will provide error messages in the Eclipse console. Look for stack traces or specific messages indicating what’s going wrong.
2. Port Already in Use
Issue
If you've run other servers or applications, starting Jetty might return an error indicating that the desired port is already in use.
Solution
You can change the port Jetty uses by doing the following:
-
Open your configuration file. If you are using a Jetty project structure, you should find a file named
start.ini
orjetty.xml
. -
Locate the connector section, which typically looks like this:
<Call name="addConnector"> <Arg> <New class="org.eclipse.jetty.server.ServerConnector"> <Arg><Ref refid="Server"/></Arg> <Set name="port">8080</Set> </New> </Arg> </Call>
-
Change the port number to an available port:
<Set name="port">8081</Set>
By changing the port, you can avoid conflicts and run multiple servers.
3. Missing Web App Dependencies
Issue
You may find that the application starts but fails to load certain resources, leading to 404 errors for JavaScript, CSS, or image files.
Solution
Make sure that all resources are included in the project build path:
-
Check Build Path:
- Right-click on your project and go to "Build Path" > "Configure Build Path."
- Ensure all libraries and dependencies are included.
-
Ensure WEB-INF folder is configured properly.
- The
WEB-INF
folder is crucial for web applications, containing theweb.xml
deployment descriptor and the classes directory.
- The
-
Clean and rebuild your project.
- In Eclipse, go to "Project" > "Clean…" to remove existing builds and ensure everything compiles fresh.
4. Configuration Misalignment
Issue
After updating your code, you may notice changes not reflecting when you run the server.
Solution
This issue is often due to Eclipse not syncing your changes properly with the Jetty server.
-
Automatic Publishing:
- Right-click your server in the "Servers" view, and choose "Open."
- In the server settings, select "Automatically publish when resources change."
-
Manually publishing.
- If you prefer the manual approach, make sure to right-click the server and select "Publish" after changes. This step can save you from frustration over unseen changes.
Example of how to set up server configurations in Eclipse:
public class MyJettyServer {
public static void main(String[] args) throws Exception {
Server server = new Server(8080);
// Setting up a context for the web application.
WebAppContext webApp = new WebAppContext();
webApp.setContextPath("/");
webApp.setWar("src/main/webapp"); // Assuming that's the directory of your web app.
server.setHandler(webApp);
server.start();
server.join();
}
}
In the code above, we start Jetty on port 8080 and set the web application context. Ensure your directory paths correspond correctly, or you'll end up with further resource misallocation errors.
5. Debugging Features Not Working
Issue
You might find that the debugging features of Eclipse do not work correctly with Jetty.
Solution
Make sure the Jetty server run configuration is set up for debugging:
-
Create a new Run Configuration.
- Go to “Run” > “Run Configurations…”, then select “Java Application” and create a new configuration for your Jetty server.
-
Add Debug Options.
- Ensure you’ve included the VM arguments needed for debugging, such as:
-Xdebug -Xrunjdwp:transport=dt_socket,server=y,suspend=n,address=5005
These settings will allow you to attach the Eclipse debugger to Jetty's running process.
Wrapping Up
Integrating Jetty with Eclipse presents an excellent opportunity for developers to enhance their Java web application development. While challenges may appear, the solutions discussed here will allow for seamless troubleshooting and debugging.
Being aware of how configurations work, managing server settings, and keeping an eye on the correct library dependencies will not only help resolve issues but also contribute to a more productive development environment.
For further reading, explore the official Jetty documentation or specific Eclipse Jetty Plugin resources for more advanced settings and features.
Happy coding!
Checkout our other articles