Troubleshooting JMS Resource Provisioning Failures in Java EE 7
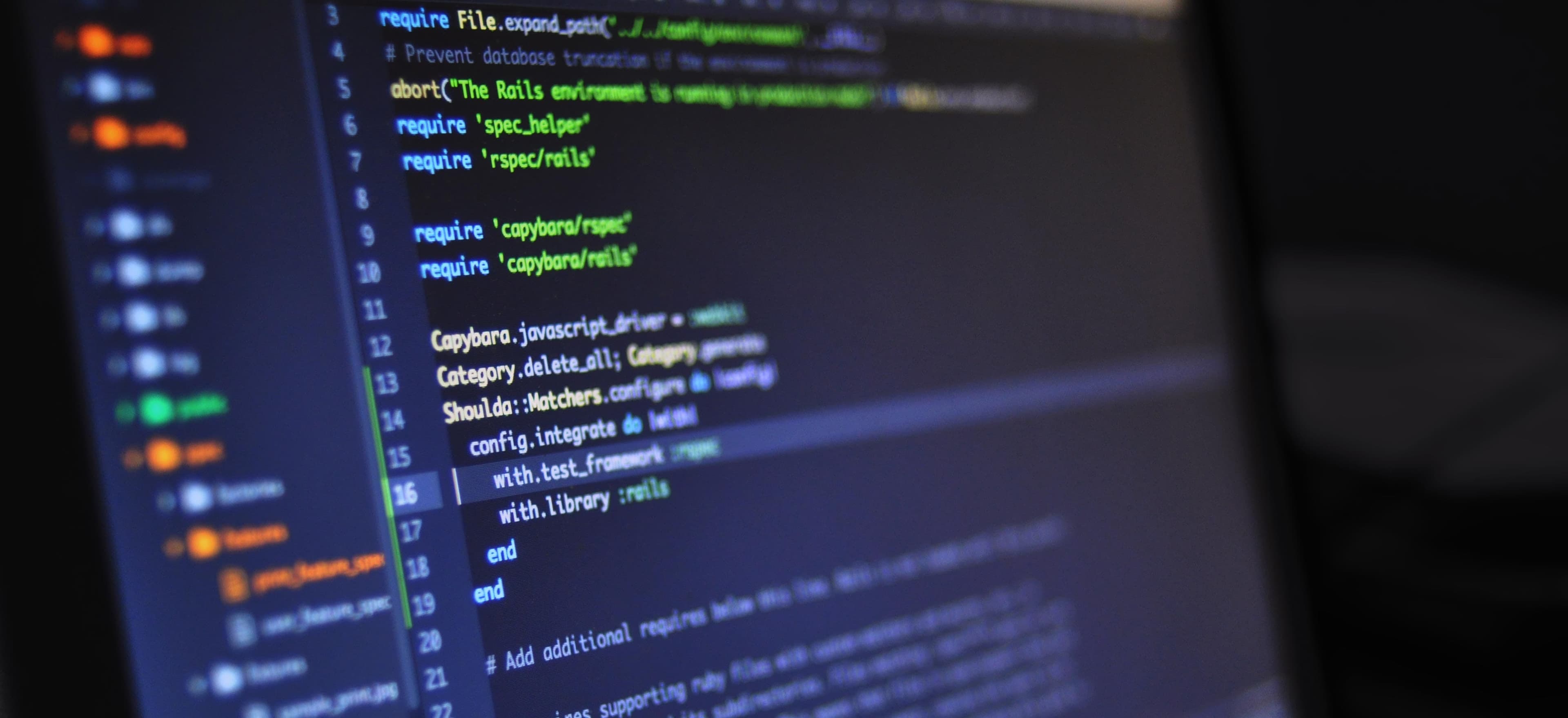
- Published on
Troubleshooting JMS Resource Provisioning Failures in Java EE 7
Java EE (Enterprise Edition) has long been recognized for its capability to build robust enterprise applications. One of the standout features of Java EE is its support for Java Message Service (JMS), which enables asynchronous communication between different components of an application. However, provisioning JMS resources can sometimes lead to failures. In this blog post, we will explore common issues that may arise during JMS resource provisioning in Java EE 7 and how to troubleshoot them effectively.
Understanding JMS in Java EE 7
Before diving into troubleshooting, let’s quickly recap what JMS is and why it matters. JMS provides a way for applications to create, send, receive and read messages, playing a crucial role in enabling loosely-coupled communication between applications. This is particularly important for enterprise systems that need to handle large volumes of transactions efficiently.
Key JMS Concepts
- Message: The data that is sent between clients.
- Queue: A point-to-point messaging model where a message is sent to a specific queue.
- Topic: A publish-subscribe model where messages are delivered to one or more subscribers.
- Connection Factory: A factory for creating connections to a JMS provider.
Common Scenarios Leading to Failures
- Configuration Issues: Incorrect settings in the configuration files can lead to failures.
- Resource Availability: Missing or unavailable JMS resources can cause connection issues.
- Connection Problems: Network issues can affect the connectivity between the JMS client and server.
Step-by-Step Troubleshooting
Step 1: Verify Configuration Files
Configuration issues are the first things you should check when troubleshooting JMS resource provisioning failures.
Example Configuration
Here’s a simplified example of what your persistence.xml
or resource definition could look like:
<resource-ref>
<description>Queue Connection Factory</description>
<res-ref-name>jms/ConnectionFactory</res-ref-name>
<res-type>javax.jms.ConnectionFactory</res-type>
<res-link>jms/ConnectionFactory</res-link>
</resource-ref>
<resource-ref>
<description>Queue</description>
<res-ref-name>jms/Queue</res-ref-name>
<res-type>javax.jms.Queue</res-type>
<res-link>jms/Queue</res-link>
</resource-ref>
Why This Matters
Always ensure that the JNDI (Java Naming and Directory Interface) names used in your application code match the resources defined in your configuration files. Mismatches can lead to NamingException
, which hinders your ability to look up JMS resources.
Step 2: Check Resource Availability
Once your configurations are verified, the next step is to ensure the JMS resources you are attempting to use are available.
Example Snippet for Checking JMS Connection
import javax.jms.Connection;
import javax.jms.ConnectionFactory;
import javax.naming.Context;
import javax.naming.InitialContext;
public class JMSUtil {
public static Connection createConnection() throws Exception {
Context ctx = new InitialContext();
ConnectionFactory factory = (ConnectionFactory) ctx.lookup("jms/ConnectionFactory");
return factory.createConnection();
}
}
Why This Matters
Using the above code snippet, you can attempt to create a connection. If the factory is missing or not configured properly, this line will throw an exception. It's critical to check server logs for configuration errors.
Step 3: Confirm JMS Provider Status
Check the status of your JMS provider. Is it running? Are there any network issues? You can typically find this information in server logs or by using monitoring tools.
Example of Logging Errors
try (Connection connection = createConnection()) {
System.out.println("Connection established successfully!");
} catch (Exception e) {
System.err.println("Failed to establish JMS connection: " + e.getMessage());
}
Why This Matters
Always log error messages. They usually contain critical clues for diagnosing the problem.
Step 4: Review Application Server Logs
Your application server (e.g., WildFly, GlassFish, TomEE) usually maintains logs that can provide valuable insights into JMS-related errors. Look for logs specific to JMS provisioning.
Common Logging Issues to Look For:
- Connection refused exceptions.
- Naming exceptions.
- Timeouts during resource lookups.
Step 5: Test Connectivity
Sometimes, the problem can be a network issue. Use tools like ping
or telnet
to check if the JMS server is reachable.
Example of Telnet Command
telnet <JMS_SERVER_IP> <JMS_PORT>
Why This Matters
A failure here indicates a network-related issue, unlike previous checks that related directly to your Java EE application.
Step 6: Assess Resource Adapter Configuration
Ensure that your JMS resource adapter is properly configured. If you are using an enterprise application, it might involve deployments and libraries that need to be bundled in a specific way.
Example Assessment Checklist
- Ensure the JCA (Java Connector Architecture) is correctly set up.
- Validate that the resource adapter JARs are included in your
WEB-INF/lib
orEAR/lib
. - Check the Java EE container's support for the JMS specification.
Common Errors with Resource Adapters:
- Invalid connection settings (username/password).
- Incorrect or missing JNDI bindings.
To Wrap Things Up
Troubleshooting JMS resource provisioning failures in Java EE 7 can be quite challenging, but by following a systematic approach, you can quickly identify and remedy the issues at hand. Start with configuration verification, then proceed through resource availability, connection testing, and finally, application server and networking considerations.
For further reading on JMS concepts, you can refer to the Java EE Documentation.
By understanding how JMS operates within Java EE, you can ensure that your enterprise applications communicate effectively and reliably—providing the back-end support that modern businesses depend on. Remember, persistence and thoroughness are key to effective troubleshooting. Happy coding!