Troubleshooting Common JSoup HTML Table Parsing Errors
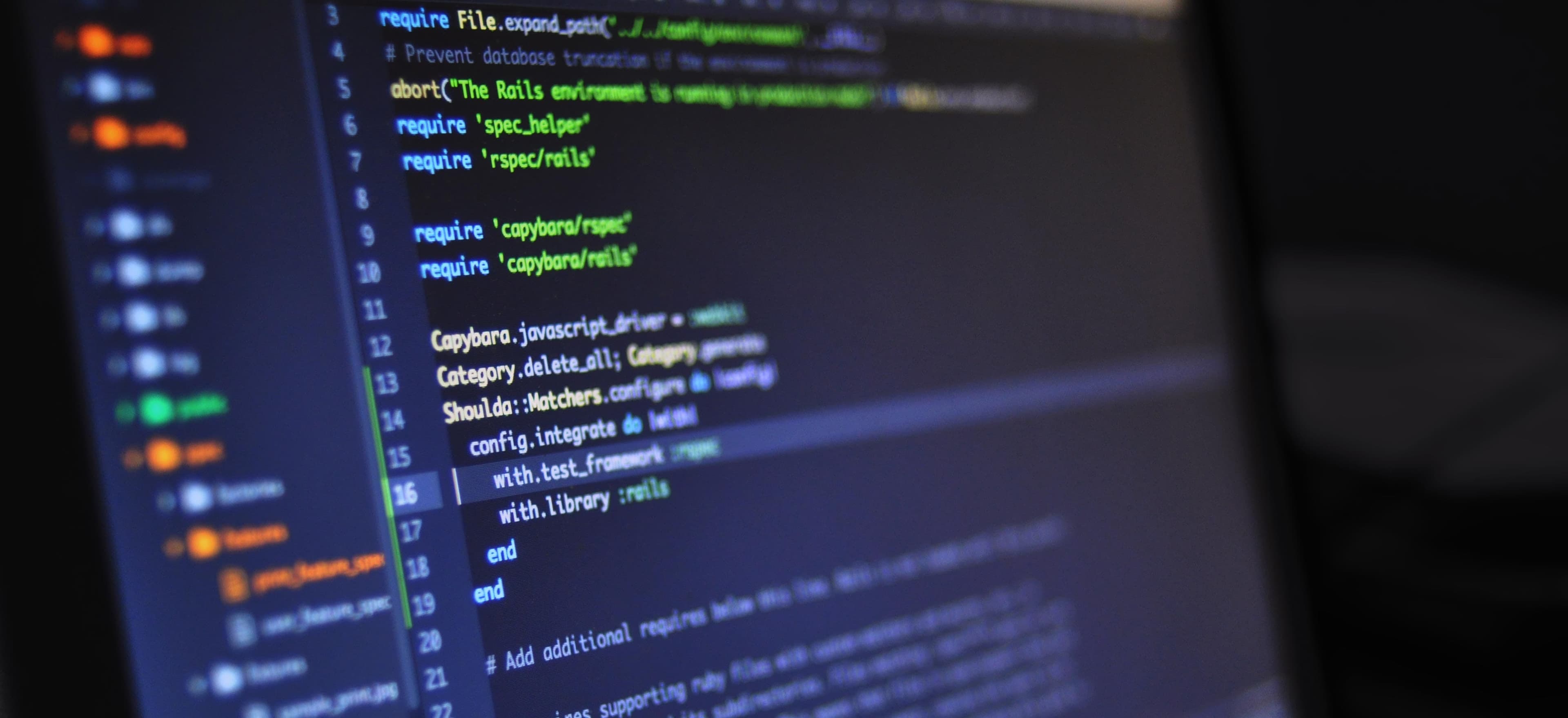
- Published on
Troubleshooting Common JSoup HTML Table Parsing Errors
Web scraping can be an invaluable skill in the data-driven world of today. As a Java developer, if you’re looking to extract data from the web, JSoup is one of the most popular libraries to achieve this. However, like any powerful tool, it can come with its share of challenges, especially when parsing HTML tables. In this blog post, we’ll discuss some common JSoup HTML table parsing errors, how to troubleshoot them, and provide examples with explanations for better understanding.
What is JSoup?
JSoup is a Java library that enables developers to work with real-world HTML. It provides a powerful API for retrieving and manipulating data, offering an easy way to parse, clean, and analyze HTML documents. Whether you're handling structured data or scraping web content, JSoup can simplify your tasks.
For more details on JSoup, check out the official JSoup documentation.
Common HTML Table Parsing Errors
1. Missing Table Elements
One common issue when working with HTML tables is that the expected <table>
, <tr>
, and <td>
elements may not be present. This can happen due to dynamic content loading or poorly structured HTML.
How to Identify
When you attempt to parse the table, you will find that your selectors return null or empty results.
Example Code
Document doc = Jsoup.connect("http://example.com").get();
Element table = doc.select("table").first();
if (table == null) {
System.out.println("Table not found!");
} else {
// Code to parse the table
}
Why it Matters: Ensuring that the table exists is crucial for avoiding null pointer exceptions during parsing.
2. Misplaced or Missing Closing Tags
HTML tables can sometimes have misplaced or missing closing tags for rows and data cells. This could lead to parsing errors, where JSoup might not recognize the intended structure.
How to Fix
If you encounter inconsistencies in the HTML structure, consider using methods to tidy up the HTML:
String html = "<table><tr><td>Data<!-- missing closing tags -->";
html += "<tr><td>More Data</td></tr></table>";
Document doc = Jsoup.parse(html);
// Ensure the document understands the structure
Element table = doc.select("table").first();
Why it Matters: Cleaning up the HTML allows JSoup to accurately interpret the table structure and parse it correctly.
3. Nested Tables
Sometimes, tables may contain nested tables, leading to confusion in parsing. JSoup will treat all tables independently unless specified.
How to Handle Nested Tables
You can use recursive methods to extract data from nested tables:
public void parseTable(Element table) {
for (Element row : table.select("tr")) {
for (Element cell : row.select("td")) {
System.out.println(cell.text());
}
// Check for nested tables
for (Element nestedTable : row.select("table")) {
parseTable(nestedTable);
}
}
}
// Usage
Element table = doc.select("table").first();
parseTable(table);
Why it Matters: Handling nested tables requires a clear strategy. Recursive parsing ensures that all levels are accounted for.
4. Incorrect CSS Selector Usage
JSoup uses CSS selectors to identify elements. If you're not familiar with CSS selectors, it can lead to not retrieving the desired data.
Common Pitfalls
- Forgetting to specify index when selecting items in a collection.
- Using the wrong selector syntax can lead to missed elements.
Example
// Incorrectly trying to select the first row
Element firstRow = doc.select("tr").first(); // This works correctly
// Make sure you are selecting the right elements
List<Element> rows = doc.select("table tr");
for (Element row : rows) {
// Do something with each row
}
Why it Matters: Understanding CSS selectors is critical to efficiently parse HTML content.
5. Handling Errors from External Sources
When scraping data, the structure of the external HTML can change at any time. If the website is redesigned or the table data is moved, your code may break.
Best Practice
Implement error handling to manage such changes gracefully.
try {
Document doc = Jsoup.connect("http://reliable-website.com").get();
Element table = doc.select("table").first(); // Adjust selector
// process table
} catch (IOException e) {
System.out.println("Error during connection: " + e.getMessage());
}
Why it Matters: Implementing error handling helps you to manage unexpected changes and maintain a robust scraper.
The Bottom Line
As you can see, troubleshooting common JSoup HTML table parsing errors can involve a range of strategies. In summary, it is essential to:
- Ensure your HTML documents are structured properly.
- Use proper CSS selectors.
- Handle nested tables.
- Implement error handling.
Armed with this knowledge, you can tackle table parsing projects with confidence. Happy coding with JSoup!
For further reading on best practices for web scraping, consider exploring this web scraping tutorial.
Further Resources
By following the strategies outlined above and utilizing JSoup effectively, your HTML table parsing tasks can go from tedious to effortless. Embrace the power of JSoup and transform the way you interact with data on the web!