Streamlining Loan Processing with JBoss on OpenShift
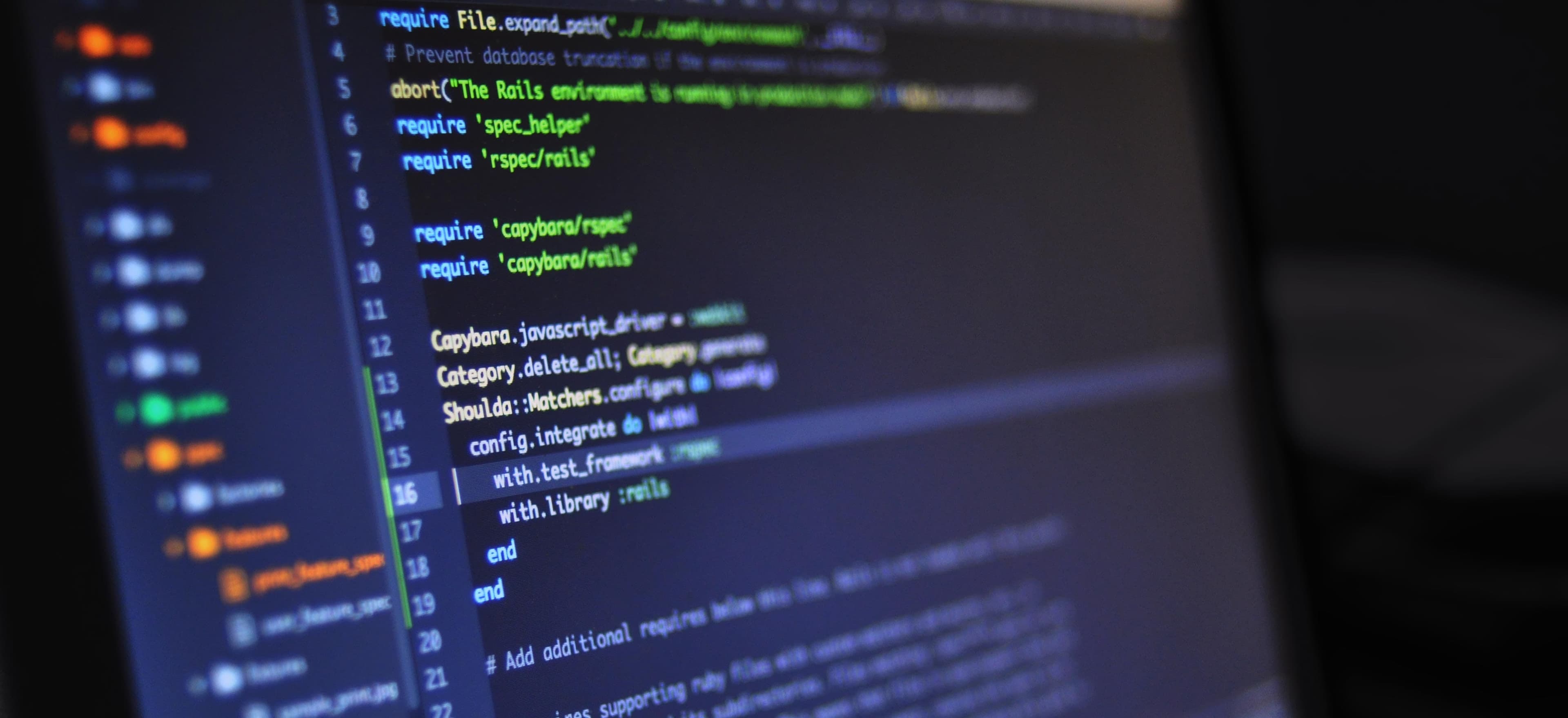
- Published on
Streamlining Loan Processing with JBoss on OpenShift
Loan processing can often be a cumbersome and time-consuming task. With the rise of digital banking and micro-lending platforms, financial institutions are looking for ways to streamline their loan processing systems. In this blog post, we will explore how to leverage JBoss on OpenShift to create a more efficient loan processing service.
Understanding JBoss and OpenShift
JBoss is a widely used application server that provides the necessary environment to run Java EE applications. It is known for its modular architecture and extensive features that cater to enterprise-grade applications.
OpenShift, on the other hand, is a Kubernetes-based platform as a service (PaaS) offered by Red Hat. It allows developers to build, deploy, and manage applications seamlessly in containers. Combining JBoss with OpenShift gives developers an edge in scalability and reliability, making it an excellent choice for loan processing applications.
Benefits of Using JBoss on OpenShift
-
Scalability: OpenShift supports automatic scaling, which is essential for handling varying workloads in loan processing, especially during peak application usage.
-
Easy Deployment: The integration of JBoss and OpenShift allows for rapid deployment of new features and patches, reducing downtime.
-
Cost-Effectiveness: Using containers can significantly lower resource expenses by optimizing the use of underlying infrastructure.
-
Improved Performance: JBoss has robust features for managing transactions and managing in-memory data, which boosts the performance of your application.
Designing the Loan Processing Application
Core Functionality
The loan processing application we will develop will include the following core functionalities:
- Loan Application Submission
- Application Review and Evaluation
- Approval and Rejection Processes
- Notifications to Applicants
Tech Stack
- Frontend: React.js (JavaScript)
- Backend: Java with the JBoss WildFly server
- Database: PostgreSQL
- Containerization: Docker
- Orchestration: OpenShift
Setting Up Your Development Environment
1. Installing JBoss and OpenShift
First, you need to install the following:
-
JBoss (WildFly) application server
-
You can download WildFly from WildFly's official site.
-
OpenShift CLI (oc)
You can get the installation instructions from the OpenShift documentation.
2. Configuring WildFly
After downloading WildFly, extract it and navigate to the bin
directory.
To start the server locally, you can use the command:
./standalone.sh
Make sure it is running by navigating to http://localhost:8080
.
3. Setting Up OpenShift
Create your OpenShift project:
oc new-project loan-processing
4. Deploying PostgreSQL on OpenShift
Deploy a PostgreSQL database to your project:
oc new-app postgresql --name=loan-db \
-e POSTGRESQL_USER=user -e POSTGRESQL_PASSWORD=pass -e POSTGRESQL_DATABASE=loan_db
This command creates a PostgreSQL instance named loan-db
, which is essential for managing incoming loan data.
Developing the Loan Processing Application
1. Setting Up the Database Model
Define your data model as follows:
@Entity
public class LoanApplication {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String applicantName;
private Double loanAmount;
private String status;
// getters and setters omitted for brevity
}
Why: Using JPA for object-relational mapping simplifies data manipulation and enhances database interactions.
2. Creating RESTful Services
Using JAX-RS, we can set up RESTful endpoints for processing loan applications.
@Path("/loans")
public class LoanApplicationResource {
@Inject
private LoanApplicationService loanApplicationService;
@POST
@Consumes(MediaType.APPLICATION_JSON)
@Produces(MediaType.APPLICATION_JSON)
public Response createApplication(LoanApplication application) {
loanApplicationService.submit(application);
return Response.status(Response.Status.CREATED).entity(application).build();
}
}
Why: This structure allows for straightforward operation management through HTTP methods, which aligns with REST principles.
3. Business Logic for Loan Approval
Implement the business logic to evaluate applications.
@Stateless
public class LoanApplicationService {
@Inject
private LoanApplicationRepository loanApplicationRepository;
public void submit(LoanApplication application) {
// Simple business logic for approval
if (application.getLoanAmount() < 50000) {
application.setStatus("Approved");
} else {
application.setStatus("Pending Review");
}
loanApplicationRepository.save(application);
}
}
Why: The logic ensures that submissions are processed and appropriately categorized, which is essential for streamlining operations.
4. Frontend Integration
Utilize React on the frontend to allow users to submit applications.
import React, { useState } from 'react';
import axios from 'axios';
const LoanApplicationForm = () => {
const [formData, setFormData] = useState({ name: '', amount: '' });
const handleSubmit = async (e) => {
e.preventDefault();
await axios.post('/api/loans', formData);
alert("Application submitted!");
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Applicant Name"
onChange={(e) => setFormData({ ...formData, name: e.target.value })}
/>
<input
type="number"
placeholder="Loan Amount"
onChange={(e) => setFormData({ ...formData, amount: e.target.value })}
/>
<button type="submit">Submit Application</button>
</form>
);
};
export default LoanApplicationForm;
Why: This React component enables dynamic user interaction while interacting with backend APIs seamlessly.
Deploying on OpenShift
Building and Deploying the Application
- Create a Dockerfile in your project root.
FROM jboss/wildfly
COPY target/loan-processing.war /opt/jboss/wildfly/standalone/deployments/
- Build the Docker image.
docker build -t loan-processing .
- Push the image to your OpenShift registry.
oc login <your-openshift-cluster>
docker tag loan-processing <your-registry>/<your-namespace>/loan-processing
docker push <your-registry>/<your-namespace>/loan-processing
- Deploy your application:
oc new-app <your-registry>/<your-namespace>/loan-processing
Monitoring and Future Enhancements
Monitoring application health and usage is vital for a loan processing system. OpenShift provides various tools for monitoring performance and usage statistics.
Future enhancements might include:
- Implementing Machine Learning algorithms for loan risk assessments.
- Incorporating a notification service using Kafka or ActiveMQ.
- Building a mobile app for loan applications.
To Wrap Things Up
Streamlining loan processing with JBoss on OpenShift presents financial institutions with a robust solution to modernize their operations. By leveraging cloud-native architecture, businesses can enhance their service offerings, improve user experiences, and significantly reduce processing times.
For further reading on JBoss and OpenShift, check out the official JBoss Documentation and OpenShift Documentation.
Your feedback and questions are welcome—let’s continue the conversation in the comments below!