Navigating Challenges in Scatter-Gather with Spring Reactor
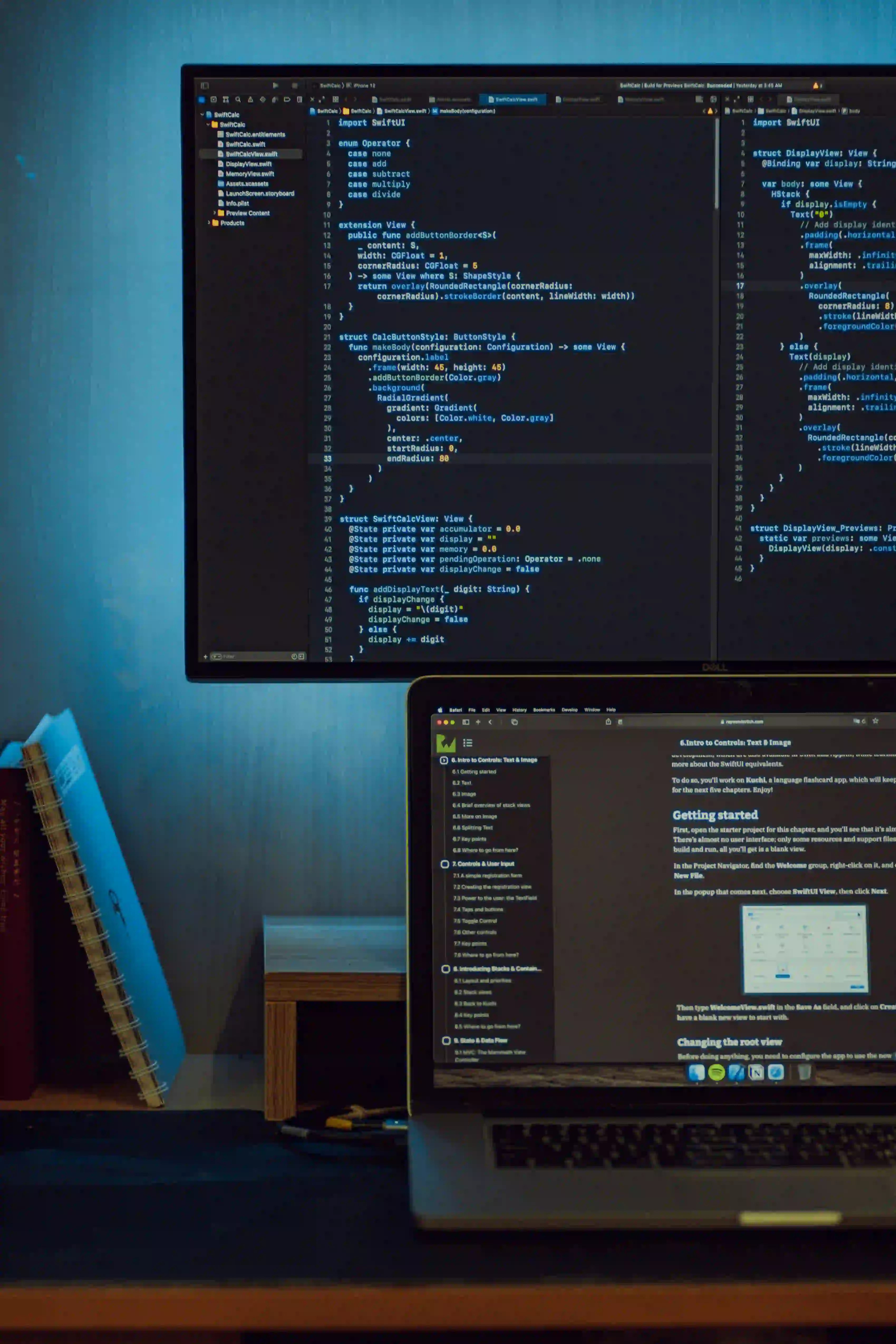
Navigating Challenges in Scatter-Gather with Spring Reactor
In the realm of reactive programming, handling asynchronous data streams can sometimes prove daunting. One such pattern that arises frequently is the Scatter-Gather pattern, an effective approach to distributing tasks across services and gathering their responses. This blog post delves into Scatter-Gather, focusing on its implementation using Spring Reactor while addressing the potential challenges you may face along the way.
Understanding Scatter-Gather
The Scatter-Gather pattern is a messaging pattern where a request is broadcast to several services (Scatter), and their responses are collected (Gather) for further processing. It's particularly useful when you want to parallelize requests while maintaining a robust architecture.
Why Use Scatter-Gather?
-
Efficiency: By distributing tasks across multiple services or threads, applications can significantly reduce response time.
-
Scalability: It facilitates the scaling of services independently, allowing for better resource usage.
-
Fault Tolerance: The pattern inherently contributes to developing resilient applications. If one service fails, it does not necessarily incapacitate the others.
Spring Reactor Overview
Before jumping into implementing Scatter-Gather, let's briefly discuss Spring Reactor. Spring Reactor is a fully non-blocking reactive programming framework built around the Reactive Streams specification. This framework is integral for building reactive applications with Spring's capabilities.
Setting Up Your Spring Reactor Project
To get started, you’ll need the following dependencies in your Maven pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>io.projectreactor</groupId>
<artifactId>reactor-core</artifactId>
</dependency>
This setup enables the use of web client and reactor core functionalities.
Implementing Scatter-Gather with Spring Reactor
Let’s dive into the code. We will create a simple Scatter-Gather implementation where we invoke multiple external APIs, gather their responses, and combine them.
Sample Service Implementation
In this example, we will build a service that fetches user details from multiple sources.
import org.springframework.stereotype.Service;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.util.List;
@Service
public class UserService {
private final WebClient webClient;
public UserService(WebClient.Builder webClientBuilder) {
this.webClient = webClientBuilder.baseUrl("https://api.example.com").build();
}
public Mono<List<User>> getUserDetails(List<String> userIds) {
return Flux.fromIterable(userIds)
.flatMap(userId -> webClient.get()
.uri("/users/{id}", userId)
.retrieve()
.bodyToMono(User.class)
.onErrorResume(e -> {
// Handle service failure gracefully
System.err.println("Failed to fetch user: " + userId + " due to " + e.getMessage());
return Mono.empty(); // Returning empty Mono instead of throwing an error
})
)
.collectList();
}
}
Breaking Down the Code
-
WebClient: This is a non-blocking client used to make HTTP calls. We build it with a base URL to streamline requests.
-
Flux.fromIterable: This allows us to create a Flux stream from a list of user IDs. Here, each ID will be processed concurrently.
-
flatMap: This operator allows parallel requests. Each service call returns a
Mono<User>
, and we utilize flatMap to execute these calls asynchronously. -
onErrorResume: This method enhances fault tolerance. If a call fails for any reason, we log the error and return an empty Mono instead of terminating the entire process.
-
collectList(): Finally, we gather all results into a list. This brings us back to a Mono, which can be easily handled downstream.
Example API Call
Let’s simulate calling our service:
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping
public Mono<List<User>> getUsers(@RequestParam List<String> ids) {
return userService.getUserDetails(ids);
}
}
Why This Structure?
The delineation between your service layer and controller promotes clean architecture. The service is reusable and can be tested independently without worrying about HTTP-specific concerns.
Challenges in Scatter-Gather
-
Handling Backpressure: In high-load scenarios, you may encounter backpressure issues when dealing with numerous concurrent requests. Spring WebFlux provides built-in strategies to handle this by using operators such as
limitRate
. -
Error Management: As showcased in the
onErrorResume
method, handling errors appropriately is critical. You must decide whether to log, ignore, or aggregate errors based on your application requirements. -
Aggregation of Responses: When gathering results, make sure to account for the possibility of empty results or errors. This often requires additional logic to filter and validate the collected data.
-
Performance Considerations: While scatter-gather is efficient, you must ensure that the number of threads handling requests does not overwhelm the system, leading to DDoS-like scenarios.
A Final Look
The Scatter-Gather pattern is an invaluable strategy in modern reactive applications. Utilizing Spring Reactor can greatly simplify the implementation while providing powerful functionalities for handling asynchronous data streams. Always be mindful of the challenges that accompany it. Error handling, backpressure, and response aggregation are key areas to watch.
For further reading, check out these official Spring documentation resources or dive into the specifics of Reactive Programming.
As you venture into developing reactive applications, remember: every challenge is a stepping stone to crafting more resilient software. Happy coding!