Mastering String Manipulation with Apache Commons Lang
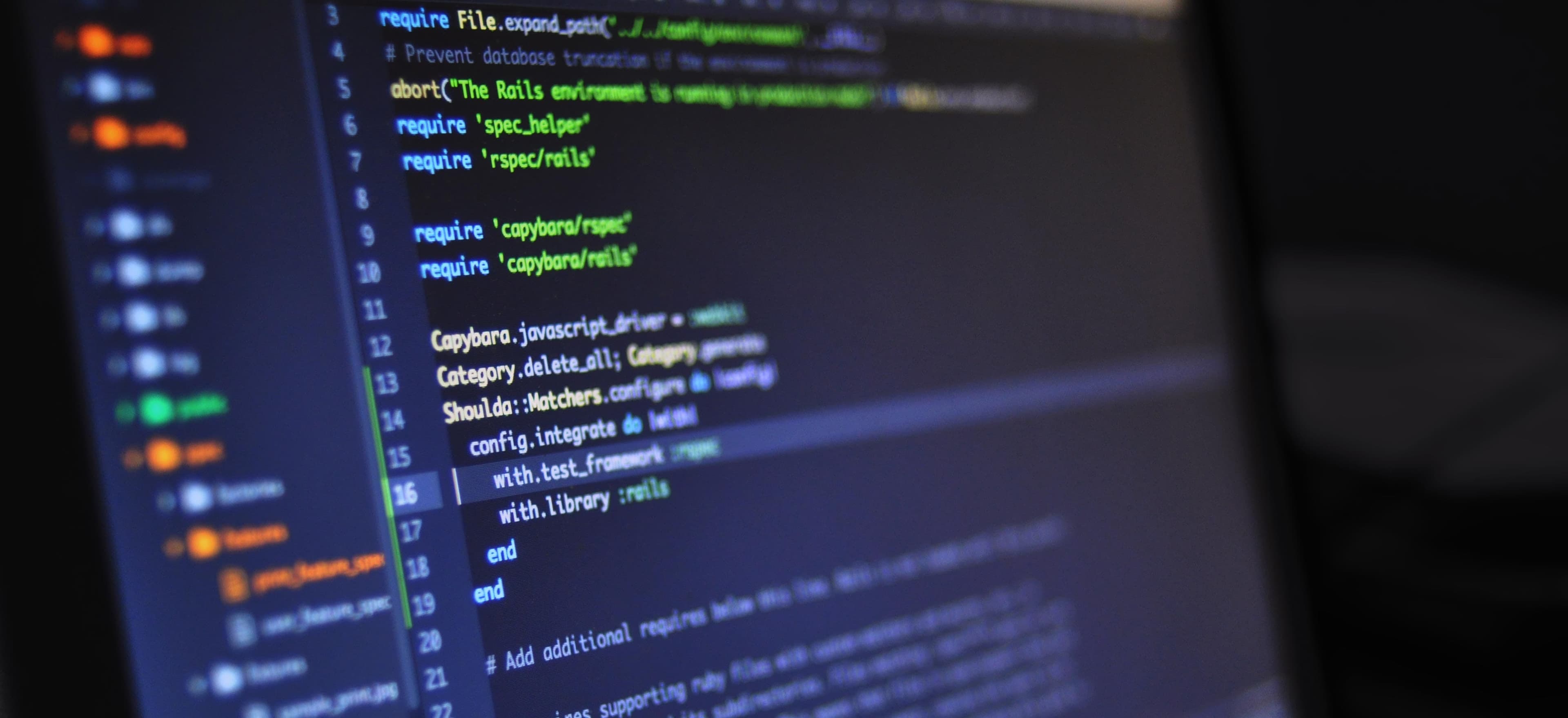
- Published on
Mastering String Manipulation with Apache Commons Lang
String manipulation is a fundamental operation in any programming language, and in Java, it's no different. While the Java Standard Library offers robust methods to handle strings, the Apache Commons Lang library elevates string manipulation into a more refined and efficient craft. In this post, we will delve into various string manipulation techniques provided by Apache Commons Lang, focusing on how these tools can enhance your coding experience.
Why Use Apache Commons Lang?
Before we dive into specific string manipulation techniques, let’s explore why Apache Commons Lang is essential for Java developers. Here are a few compelling reasons:
- Enhanced Functionality: While Java includes
String
andStringBuilder
, the Apache Commons Lang library extends this functionality with numerous utility methods. - Code Readability and Maintenance: Using well-named, dedicated utility methods can make your code cleaner and easier to understand.
- Performance Optimizations: Many of the methods in Apache Commons Lang are optimized for speed and performance, making them suitable for applications that do extensive string manipulations.
To get started, you need to include the Apache Commons Lang library in your project. If you're using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
Let's explore the powerful features of Apache Commons Lang for string manipulation.
1. StringUtils: The Core of String Manipulation
The StringUtils
class provides methods for string manipulation that cover a wide range of utilities. Here are some of the most commonly used methods:
a. Checking for Empty Strings
One of the common tasks in string manipulation is checking whether a string is empty or not. Instead of using string.length() == 0
, you can use:
import org.apache.commons.lang3.StringUtils;
public class StringUtilsExample {
public static void main(String[] args) {
String str = "";
if (StringUtils.isEmpty(str)) {
// Using StringUtils to check if the string is empty
System.out.println("The string is empty.");
}
}
}
Why Use StringUtils.isEmpty()
?
This method returns true
for both null
and empty strings, which can save you from null pointer exceptions and make your code safer.
b. Trimming and Padding
StringUtils also provides methods to trim strings of whitespace and to pad them as needed.
public class TrimAndPadExample {
public static void main(String[] args) {
String original = " Java Programming ";
// Trimming whitespace
String trimmed = StringUtils.trim(original);
System.out.println("Trimmed: '" + trimmed + "'");
// Padding the string
String padded = StringUtils.center(trimmed, 30, '*');
System.out.println("Padded: '" + padded + "'");
}
}
Why Trim and Pad?
Trimming removes unwanted whitespace, making user inputs cleaner. Centering can be helpful for formatting output for better readability, especially in command-line applications.
2. String Conversion
The library offers some utilities to convert strings easily:
a. Case Conversion
You can convert strings to different cases easily using StringUtils
:
public class CaseConversionExample {
public static void main(String[] args) {
String original = "java programming";
String upperCase = StringUtils.upperCase(original);
String lowerCase = StringUtils.lowerCase(original);
String capitalizedCase = StringUtils.capitalize(original);
System.out.println("Upper Case: " + upperCase);
System.out.println("Lower Case: " + lowerCase);
System.out.println("Capitalized: " + capitalizedCase);
}
}
Why Use Case Conversion?
This is particularly important in processing user input or filenames, where you may want a consistent format for comparisons or storage.
3. String Comparison
Comparing strings efficiently is another area where Apache Commons Lang shines.
a. Case-Insensitive Comparison
Two strings might differ in cases but represent the same meaning. For such cases, StringUtils
provides a case-insensitive comparison:
public class ComparisonExample {
public static void main(String[] args) {
String str1 = "JAVA";
String str2 = "java";
boolean equalsIgnoreCase = StringUtils.equalsIgnoreCase(str1, str2);
System.out.println("Strings are equal (ignore case): " + equalsIgnoreCase);
}
}
Why Use Case-Insensitive Comparisons?
This is crucial for user authentication or search functionalities where the case should not affect the results.
4. String Splitting and Joining
Splitting strings into arrays and joining them back together is another common task in string manipulation.
a. Splitting Strings
You can split strings by delimiters using StringUtils
:
public class SplitExample {
public static void main(String[] args) {
String csv = "apple,banana,orange";
String[] fruits = StringUtils.split(csv, ',');
for (String fruit : fruits) {
System.out.println("Fruit: " + fruit);
}
}
}
b. Joining Strings
Similarly, joining strings is just as easy:
public class JoinExample {
public static void main(String[] args) {
String[] fruits = {"apple", "banana", "orange"};
String joined = StringUtils.join(fruits, ", ");
System.out.println("Joined Fruits: " + joined);
}
}
Why Split and Join?
These methods facilitate handling CSV data, lists, or any other such formats where data is structured in strings.
5. String Manipulation in Practice
Let’s put all of these techniques together in a practical example: Processing a user input string into a standardized format suitable for storage in a database.
import org.apache.commons.lang3.StringUtils;
public class UserInputProcessor {
public static void main(String[] args) {
String userInput = " Example User@Email.Com ";
// Step 1: Trim whitespace
String cleanedInput = StringUtils.trim(userInput);
// Step 2: Normalize case
cleanedInput = StringUtils.lowerCase(cleanedInput);
// Step 3: Simple validation
if (StringUtils.contains(cleanedInput, "@")) {
System.out.println("Valid input: " + cleanedInput);
} else {
System.out.println("Invalid input. Please enter a valid email.");
}
}
}
Why This Example?
In a real-world application, user inputs must be thoroughly cleaned and processed to prevent errors later in the data handling pipeline, especially before database storage.
Closing the Chapter
Apache Commons Lang is a powerful library that can substantially enhance string manipulation in Java. Its StringUtils
class allows for clean, efficient, and readable code that addresses many common string-related challenges. Whether you're doing basic trimming or complex string comparisons, you'll find the tools you need at your fingertips.
For more information on the capabilities of Apache Commons Lang, refer to the official documentation.
Make string manipulation a breeze with Apache Commons Lang and improve your productivity as a Java developer!