Understanding Text Blocks: Common Pitfalls in JDK 13
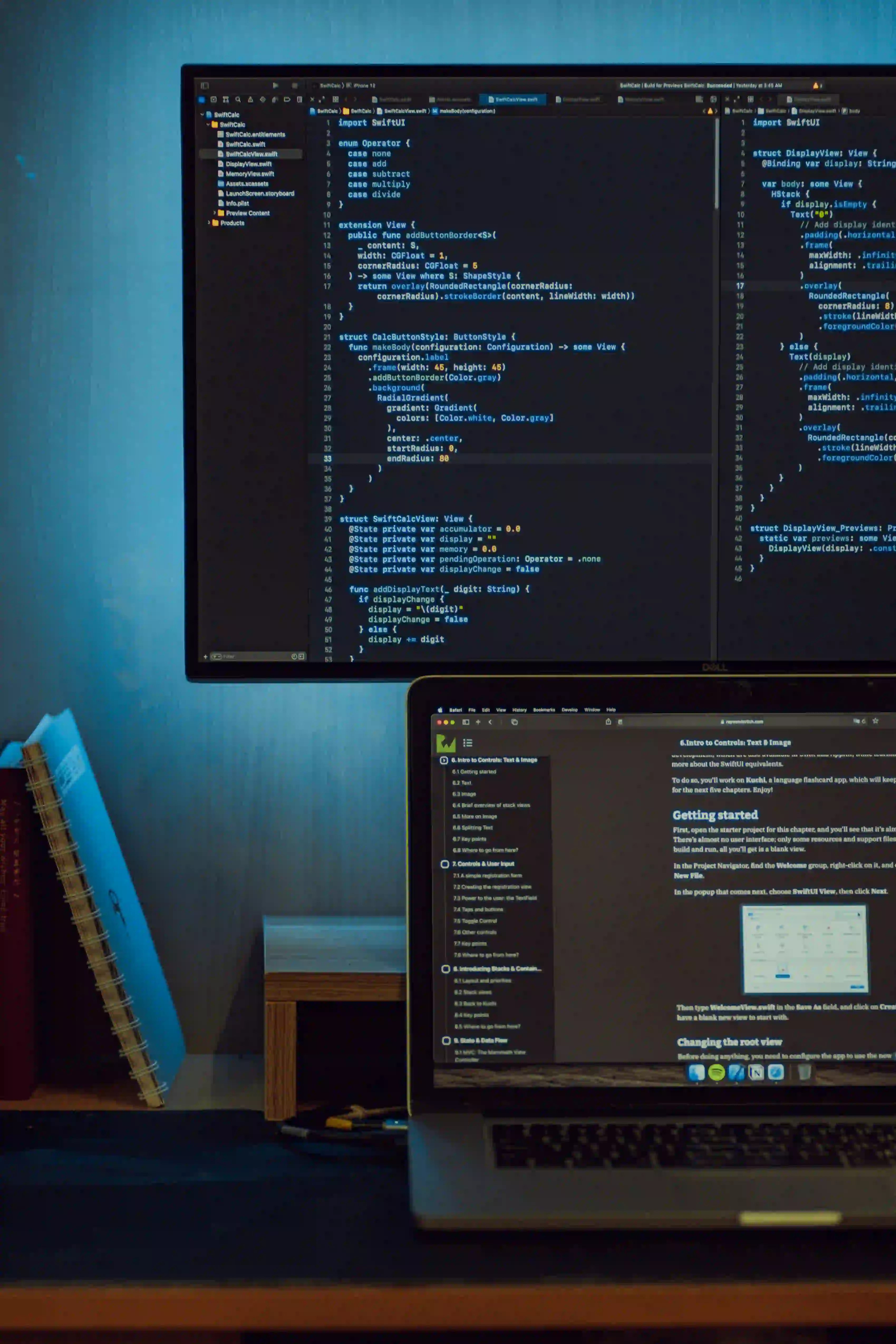
Understanding Text Blocks: Common Pitfalls in JDK 13
In Java 13, the introduction of Text Blocks revolutionized the way we handle multi-line string literals. Designed to enhance the readability of strings that span multiple lines, Text Blocks provide a streamlined approach to defining rich text content like SQL queries, JSON, or HTML. However, transitioning to this feature is not without its challenges. In this blog post, we will explore some common pitfalls that developers encounter when working with Text Blocks in JDK 13 and how to effectively navigate them.
What are Text Blocks?
Before diving into pitfalls, it is essential to understand what Text Blocks are. A Text Block is defined using three double-quote characters ("""
). Here’s a basic example:
String textBlock = """
Hello, World!
This is a Text Block.
It can span multiple lines.
""";
The above example is straightforward. The content inside the triple double quotes will be preserved exactly as shown, including line breaks and spaces, making it significantly easier to read compared to traditional string concatenation techniques.
Common Pitfalls with Text Blocks
1. Indentation and Whitespace Issues
One of the most common pitfalls with Text Blocks is managing indentation and leading whitespace. Developers may find that the indentation of the Text Block content can lead to unexpected leading spaces in the output. Java will preserve any space before the first non-whitespace character in each line.
For example:
String textBlock = """
Line one
Line two
""";
This would create a string with leading spaces:
Line one
Line two
Solution
To address this, you can use the stripMargin()
method to remove the leading whitespace effectively. Here’s how:
String textBlock = """
|Line one
|Line two
""".stripMargin();
By prefixing each line with a pipe (|
), you can strip the margin, leading to a nicely formatted string without unwanted whitespace.
2. Unexpected Line Breaks
Another critical issue newcomers face involves unwanted line breaks in their output. While Text Blocks allow you to create multi-line strings, a line break in your Text Block will actually insert a newline in the string.
Consider this example:
String json = """
{
"key": "value"
}
""";
The output will include line breaks, which may not always be suitable for JSON or similar formats.
Solution
If you need the string to remain continuous, you can concatenate the lines instead of breaking them:
String json = "{\n" +
" \"key\": \"value\"\n" +
"}";
Alternatively, using the replaceAll()
method can also clean up unwanted line breaks effectively.
3. Escape Sequences
When using Text Blocks, developers might mistakenly believe they are free from managing escape sequences. However, to include special characters like quotes within Text Blocks, you still need to escape them, which may lead to confusion.
Example:
String textBlock = """
"This is quoted text"
""";
You must escape inner quotes:
String textBlock = """
\"This is quoted text\"
""";
Solution
Always remember that while Text Blocks reduce the need for much escaping, they cannot eliminate it entirely. Keeping your quotes escaped will ensure the string compiles correctly.
4. Using Non-Standard Characters
When working with Text Blocks, developers may inadvertently include non-standard characters that can cause issues. For example, if you are not careful, you might end up with a string containing invalid characters that do not display as intended.
Solution
To prevent such errors, it is best practice to validate the content of your Text Block, especially if it includes data from external sources or user input. Implementing thorough input validation can save you from unexpected behaviors down the line.
5. Formatting Quirks
The formatting behavior of Text Blocks can sometimes achieve unexpected results based on how the code is laid out. Despite the benefits, you may find that formats such as JSON are not as clean or compact due to how strings are composed.
Solution
When writing JSON or XML, consider string manipulation functions to format the string as needed. For instance, you might want to remove unnecessary whitespace before parsing JSON data:
String jsonData = """
{
"key": "value"
}
""".replaceAll("[\n\r]", "");
To Wrap Things Up
Text Blocks introduced in JDK 13 provide a powerful tool for managing large strings with multiple lines more elegantly. However, they do come with their set of common pitfalls that can confound even experienced Java developers. By understanding these issues—whitespace management, line breaks, escape sequences, character validation, and formatting quirks—you can effectively leverage Text Blocks in your Java applications without encountering frustrating bugs.
For further reading, you can explore the official Java SE Documentation or delve deeper into the Java Enhancement Proposals related to Text Blocks.
Feel free to share your experiences with Text Blocks or any other common pitfalls you have faced while transitioning to JDK 13 in the comments below!